


What are the different types of testing that you can perform in a Vue.js application (e.g., unit testing, component testing, end-to-end testing)?
Mar 27, 2025 pm 05:21 PMWhat are the different types of testing that you can perform in a Vue.js application (e.g., unit testing, component testing, end-to-end testing)?
In a Vue.js application, several types of testing can be performed to ensure the quality and reliability of the application. These include:
- Unit Testing: This type of testing focuses on individual units or components of the application in isolation. In Vue.js, unit tests are typically written to test the logic within components, methods, computed properties, and other small, isolated pieces of code. Unit testing helps in identifying bugs at the earliest stage of development.
- Component Testing: Also known as integration testing in the context of Vue.js, component testing involves testing the Vue components in a more realistic environment. This type of testing checks how components interact with each other and how they behave when rendered in the DOM. It's crucial for ensuring that the components work correctly when integrated into the larger application.
- End-to-End (E2E) Testing: This type of testing simulates real user scenarios and tests the application from start to finish. E2E testing in Vue.js involves automating interactions with the application as a user would, checking if the application behaves as expected from a user's perspective. It helps in identifying issues that might not be caught by unit or component testing, such as navigation flows and integration with backend services.
Each type of testing plays a crucial role in the development lifecycle of a Vue.js application, ensuring that the application is robust, reliable, and user-friendly.
Which tools are recommended for unit testing in Vue.js applications?
For unit testing in Vue.js applications, several tools are recommended due to their compatibility and effectiveness:
- Jest: Jest is a popular choice for unit testing in Vue.js applications. It's a JavaScript testing framework developed by Facebook that provides a zero-configuration setup, fast execution, and a rich set of features like mocking, code coverage, and snapshot testing. Vue.js officially recommends Jest for unit testing.
- Mocha: Mocha is another widely used JavaScript test framework that can be used for unit testing in Vue.js applications. It's flexible and can be paired with assertion libraries like Chai. While it requires more setup compared to Jest, it's a robust choice for developers who prefer a more customizable testing environment.
- Vue Test Utils: This is a set of utility functions provided by the Vue.js team specifically designed to simplify the process of testing Vue components. It's often used in conjunction with Jest or Mocha to provide a more seamless testing experience for Vue.js applications.
These tools, when used effectively, can significantly enhance the unit testing process in Vue.js applications, ensuring that individual components and functions are thoroughly tested and reliable.
How can component testing improve the development process of a Vue.js application?
Component testing can significantly improve the development process of a Vue.js application in several ways:
- Early Detection of Bugs: By testing components in isolation and in a more realistic environment, developers can catch bugs early in the development cycle. This reduces the likelihood of these issues propagating to other parts of the application, making it easier and less costly to fix them.
- Improved Code Quality: Component testing encourages developers to write more modular and reusable code. Knowing that components will be tested individually motivates developers to keep their components clean, well-structured, and focused on a single responsibility.
- Enhanced Collaboration: When components are thoroughly tested, it becomes easier for different team members to work on different parts of the application without fear of breaking existing functionality. This fosters better collaboration and allows for parallel development, speeding up the overall development process.
- Confidence in Refactoring: With a robust set of component tests, developers can refactor code with confidence, knowing that any unintended changes will be caught by the tests. This encourages continuous improvement and optimization of the codebase.
- Better User Experience: By ensuring that components work correctly when integrated into the application, component testing helps in delivering a more reliable and user-friendly application. This can lead to higher user satisfaction and better overall performance of the application.
What are the best practices for setting up end-to-end testing in a Vue.js project?
Setting up end-to-end (E2E) testing in a Vue.js project involves several best practices to ensure that the tests are effective and maintainable:
- Choose the Right Tool: For Vue.js projects, Cypress and Nightwatch.js are popular choices for E2E testing. Cypress is particularly recommended due to its ease of use, fast execution, and rich set of features tailored for modern web applications.
- Set Up a Testing Environment: Ensure that your testing environment closely mimics the production environment. This includes using the same browser versions, operating systems, and network conditions. Tools like Docker can help in setting up consistent testing environments.
- Write Clear and Concise Tests: E2E tests should be written to simulate real user interactions. Keep the tests focused on user flows and critical functionalities. Use clear and descriptive names for your tests to make them easy to understand and maintain.
- Use Page Object Model (POM): Implement the Page Object Model pattern to abstract the structure and behavior of your application's pages. This makes your tests more readable and easier to maintain, as changes to the UI can be updated in one place rather than across multiple tests.
- Run Tests Regularly: Integrate E2E tests into your CI/CD pipeline to run them automatically on every code commit or pull request. This ensures that any issues are caught early and can be addressed before they reach production.
- Monitor Test Performance: Keep an eye on the performance of your E2E tests. Slow tests can become a bottleneck in your development process. Optimize your tests to run quickly and efficiently, and consider running them in parallel to save time.
- Review and Refactor Tests: Regularly review your E2E tests to ensure they remain relevant and effective. Refactor tests as needed to keep them aligned with the evolving application and to remove any redundant or obsolete tests.
By following these best practices, you can set up a robust E2E testing framework for your Vue.js project, ensuring that your application is thoroughly tested and reliable from a user's perspective.
The above is the detailed content of What are the different types of testing that you can perform in a Vue.js application (e.g., unit testing, component testing, end-to-end testing)?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
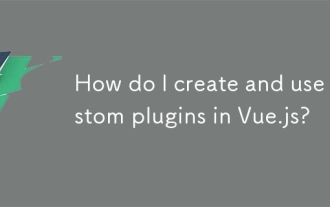
How do I create and use custom plugins in Vue.js?
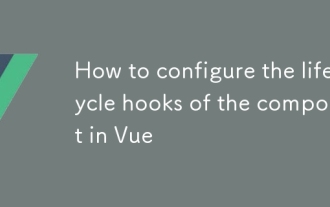
How to configure the lifecycle hooks of the component in Vue

How to configure the watch of the component in Vue export default
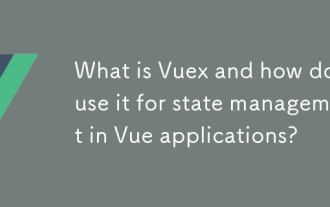
What is Vuex and how do I use it for state management in Vue applications?
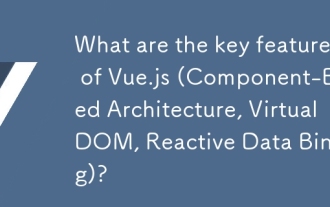
What are the key features of Vue.js (Component-Based Architecture, Virtual DOM, Reactive Data Binding)?
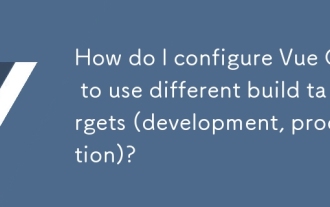
How do I configure Vue CLI to use different build targets (development, production)?

How do I implement advanced routing techniques with Vue Router (dynamic routes, nested routes, route guards)?
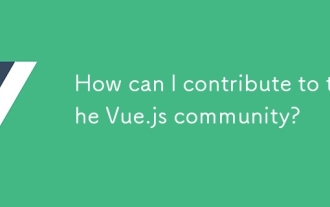
How can I contribute to the Vue.js community?
