


What testing libraries are you familiar with (e.g., Vue Test Utils, Testing Library)?
Mar 27, 2025 pm 05:23 PMWhat testing libraries are you familiar with (e.g., Vue Test Utils, Testing Library)?
I am familiar with several testing libraries that are commonly used in front-end development, particularly for Vue.js projects. Here are some of the most notable ones:
- Vue Test Utils: This is the official testing utility library for Vue.js. It provides a set of methods to mount and interact with Vue components in a test environment. It's particularly useful for unit testing Vue components and allows for deep manipulation of the component's internals.
-
Testing Library: This library is not specific to Vue.js but can be used with Vue through the
@testing-library/vue
package. It emphasizes testing components in a way that closely mimics how users interact with them, promoting more accessible and user-centric tests. - Jest: While not a testing library per se, Jest is a popular JavaScript testing framework that can be used in conjunction with Vue Test Utils or Testing Library. It provides a zero-configuration testing experience and includes features like mocking, code coverage, and snapshot testing.
- Cypress: This is an end-to-end testing framework that can be used to test Vue.js applications. It runs in the browser and provides a rich set of commands to interact with the application as a user would.
- Mocha: Another popular JavaScript test framework that can be used with Vue.js. It's often used with Chai for assertions and Sinon for spies, stubs, and mocks.
Can you recommend the best testing library for a Vue.js project?
For a Vue.js project, I would recommend using @testing-library/vue in conjunction with Jest. Here's why:
- User-Centric Testing: @testing-library/vue encourages testing components from the perspective of the user, which leads to more robust and maintainable tests. It helps ensure that your tests are aligned with how users interact with your application.
- Integration with Jest: Jest is a powerful and easy-to-use testing framework that integrates well with @testing-library/vue. It provides features like snapshot testing, which can be particularly useful for ensuring UI consistency in Vue components.
- Community Support and Documentation: Both @testing-library/vue and Jest have strong community support and extensive documentation, making it easier to find resources and solutions to common testing challenges.
- Flexibility: This combination allows for both unit and integration testing, giving you the flexibility to test at different levels of your application.
How do Vue Test Utils and Testing Library differ in their approach to testing?
Vue Test Utils and Testing Library differ significantly in their approach to testing Vue.js components:
-
Focus on User Interaction: Testing Library focuses on testing components from the user's perspective. It encourages querying the DOM in the same way a user would, using methods like
getByText
,getByRole
, etc. This approach promotes more accessible and user-centric tests. -
Component Internals: Vue Test Utils, on the other hand, allows for more direct manipulation of component internals. It provides methods like
setProps
,setData
, andsetMethods
, which allow you to directly modify the state and props of a component. This can be useful for unit testing but may lead to tests that are tightly coupled to the implementation details of the component. - Testing Philosophy: Testing Library follows the philosophy of "the more your tests resemble the way your software is used, the more confidence they can give you." It discourages testing implementation details and encourages testing the behavior of the component. Vue Test Utils, while not discouraging this approach, provides the tools to test implementation details if needed.
- Accessibility: Testing Library has a strong focus on accessibility, providing utilities to test for accessibility issues. Vue Test Utils does not have built-in accessibility testing features.
What are the key features to look for in a testing library for front-end development?
When choosing a testing library for front-end development, consider the following key features:
- Ease of Use: The library should have a straightforward API and be easy to set up and use. This reduces the learning curve and makes it more likely that developers will write and maintain tests.
- User-Centric Testing: Look for libraries that encourage testing from the user's perspective. This leads to tests that are more aligned with how the application is used and can help catch issues that affect the user experience.
- Accessibility Testing: The ability to test for accessibility issues is crucial, especially for web applications. Libraries that provide built-in accessibility testing utilities can help ensure your application is accessible to all users.
- Integration with Testing Frameworks: Compatibility with popular testing frameworks like Jest or Mocha can enhance the testing experience by providing additional features like code coverage and snapshot testing.
- Mocking and Stubbing: The ability to mock dependencies and stub out external services is essential for isolating the component or function being tested.
- Community and Documentation: A strong community and comprehensive documentation can be invaluable resources when you encounter issues or need to learn new testing techniques.
- Flexibility: The library should be flexible enough to support different types of testing, such as unit, integration, and end-to-end testing, depending on your project's needs.
- Performance: The library should not significantly slow down your development workflow. Fast test execution times are important for maintaining a smooth development process.
The above is the detailed content of What testing libraries are you familiar with (e.g., Vue Test Utils, Testing Library)?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
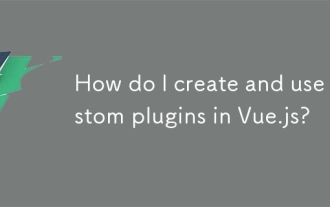
How do I create and use custom plugins in Vue.js?
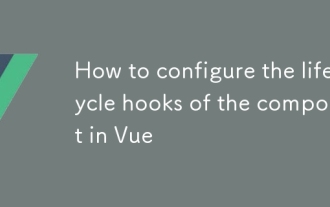
How to configure the lifecycle hooks of the component in Vue

How to configure the watch of the component in Vue export default
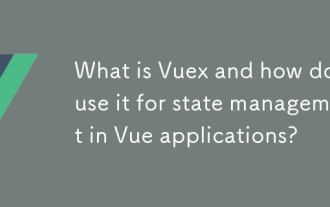
What is Vuex and how do I use it for state management in Vue applications?
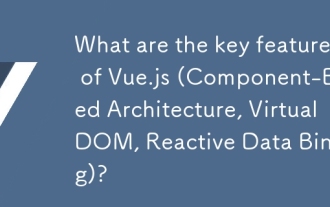
What are the key features of Vue.js (Component-Based Architecture, Virtual DOM, Reactive Data Binding)?
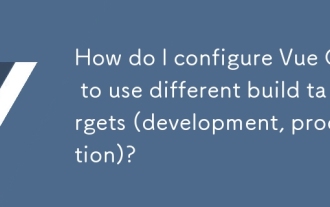
How do I configure Vue CLI to use different build targets (development, production)?

How do I implement advanced routing techniques with Vue Router (dynamic routes, nested routes, route guards)?
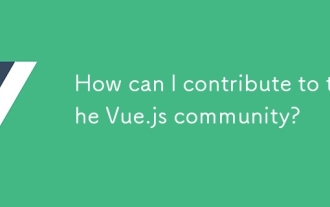
How can I contribute to the Vue.js community?
