Is Laravel a frontend or backend?
Mar 27, 2025 pm 05:31 PMLaravel is a backend framework built on PHP, designed for web application development. It focuses on server-side logic, database management, and application structure, and can be integrated with frontend technologies like Vue.js or React for full-stack development.
Laravel is primarily a backend framework. It's built on PHP and designed to facilitate the development of web applications, focusing on the server-side logic, database management, and application structure. While Laravel itself doesn't handle frontend development directly, it can be paired with frontend technologies like Vue.js or React to create full-stack applications.
Laravel: A Deep Dive into Backend Mastery
When I first stumbled upon Laravel, I was captivated by its elegance and power. As a backend framework, Laravel has transformed the way I approach web development, offering a symphony of tools and features that make server-side coding not just efficient, but enjoyable. Let's embark on a journey to explore Laravel's backend prowess, sharing insights and experiences along the way.
Laravel's Backend Charm
Laravel isn't just another PHP framework; it's a philosophy. It embraces the Model-View-Controller (MVC) pattern, which I've found to be a game-changer for organizing code. The elegance of Laravel lies in its ability to handle complex backend tasks with simplicity. From routing to database migrations, Laravel streamlines the process, making it a joy to work with.
Here's a snippet of how Laravel's routing system works, which I find incredibly intuitive:
Route::get('/users', function () { return 'Welcome to the users page!'; });
This simple route definition showcases Laravel's clean syntax, allowing you to map URLs to specific actions effortlessly.
The Art of Eloquent ORM
One of Laravel's crown jewels is Eloquent ORM. It's not just an ORM; it's a way of thinking about data. Eloquent allows you to interact with your database using an object-oriented approach, which I've found to be both intuitive and powerful. Here's how you can define a model and retrieve data:
class User extends Model { protected $fillable = ['name', 'email']; } $users = User::all();
This code snippet demonstrates how Eloquent simplifies database interactions. However, it's worth noting that while Eloquent is incredibly convenient, it can lead to performance issues if not used judiciously. I've learned to be cautious with eager loading to avoid the N 1 query problem.
Blade: The Artisan's Template Engine
While Laravel is a backend framework, it comes with Blade, a templating engine that blurs the line between backend and frontend. Blade allows you to inject backend logic into your views, which I've found to be a powerful tool for maintaining consistency across your application. Here's a simple example:
<!-- resources/views/welcome.blade.php --> @if($users->count()) <ul> @foreach($users as $user) <li>{{ $user->name }}</li> @endforeach </ul> @endif
Blade's syntax is clean and expressive, but it's important to keep your views lean. Overloading them with logic can lead to a messy frontend, which I've learned the hard way.
Laravel's Ecosystem: A Developer's Playground
Laravel's ecosystem is vast and vibrant. From Laravel Mix for asset compilation to Laravel Horizon for queue monitoring, the tools at your disposal are endless. I've found Laravel Mix particularly useful for managing frontend assets, even though Laravel itself is backend-focused:
// webpack.mix.js mix.js('resources/js/app.js', 'public/js') .sass('resources/sass/app.scss', 'public/css');
This snippet shows how Laravel Mix simplifies asset management, allowing you to focus on your backend logic while still maintaining a polished frontend.
Performance Optimization: The Backend's Achilles' Heel
While Laravel offers a plethora of features, performance optimization is an area where it can be challenging. I've encountered situations where eager loading and complex queries slowed down my applications. Here's a tip I've learned to optimize database queries:
$users = User::with('posts')->get();
This eager loading technique can significantly reduce the number of queries, but it's crucial to profile your application and understand where bottlenecks occur. I've used Laravel's built-in debugging tools like dd()
and dump()
to identify performance issues, which has been invaluable.
Best Practices: Crafting Elegant Backend Code
Over the years, I've developed a set of best practices that have helped me harness Laravel's full potential. Here are a few insights:
Modularize Your Code: Laravel's service providers and facades allow you to break down your application into manageable pieces. I've found this approach to be crucial for maintaining large projects.
Use Artisan Commands: Laravel's Artisan CLI is a powerful tool for automating tasks. I've written custom commands to streamline my development process, which has saved me countless hours.
Embrace Testing: Laravel's testing suite is robust. I've learned to write tests before writing code, which has improved the quality and reliability of my applications.
In conclusion, Laravel is a backend framework that offers a rich set of tools and features to build robust web applications. Its elegance, coupled with its powerful ecosystem, makes it a favorite among developers. However, like any tool, it requires careful handling to avoid common pitfalls. By understanding its strengths and weaknesses, you can leverage Laravel to create backend masterpieces that are both efficient and enjoyable to develop.
The above is the detailed content of Is Laravel a frontend or backend?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
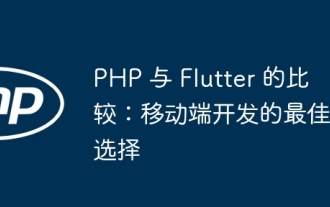
PHP vs. Flutter: The best choice for mobile development

Analysis of the advantages and disadvantages of PHP unit testing tools
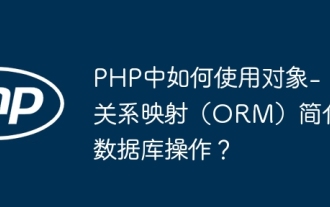
How to use object-relational mapping (ORM) in PHP to simplify database operations?
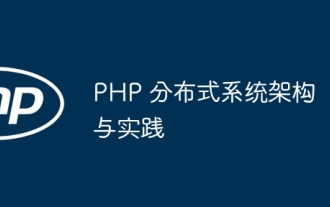
PHP distributed system architecture and practice
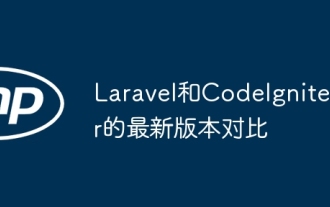
Comparison of the latest versions of Laravel and CodeIgniter
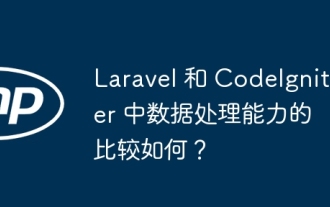
How do the data processing capabilities in Laravel and CodeIgniter compare?
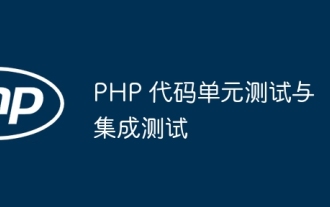
PHP code unit testing and integration testing
