Laravel/Symfony Middleware: Creating and using middleware.
Mar 28, 2025 pm 05:08 PMLaravel/Symfony Middleware: Creating and using middleware.
Middleware in Laravel and Symfony frameworks serves as an intermediary layer for handling requests entering your application. It allows you to perform actions before and after the execution of a route or controller action. This concept is vital for maintaining the modularity and reusability of your code.
Middleware can be used for a wide range of functionalities such as authentication, logging, data transformation, and more. In Laravel, middleware can be applied to specific routes, route groups, or globally to all routes. Similarly, in Symfony, middleware is often implemented through event listeners or subscribers, allowing fine-grained control over the request-response cycle.
What are the key steps to create a new middleware in Laravel or Symfony?
Laravel:
-
Generate Middleware: Use the
make:middleware
Artisan command to create a new middleware. For instance, to create a middleware namedCheckAge
, you would run:php artisan make:middleware CheckAge
Copy after loginThis command generates a new file in the
app/Http/Middleware
directory. Define Logic: Open the newly created file and implement the logic within the
handle
method. For example:public function handle(Request $request, Closure $next) { if ($request->input('age') < 18) { return redirect('home'); } return $next($request); }
Copy after loginRegister Middleware: Register the middleware in the
app/Http/Kernel.php
file within the$routeMiddleware
array. For example:protected $routeMiddleware = [ // ... other middleware ... 'age' => \App\Http\Middleware\CheckAge::class, ];
Copy after loginApply Middleware: Finally, apply the middleware to routes or controllers. For instance:
Route::get('user/profile', function () { // ... })->middleware('age');
Copy after login
Symfony:
- Create a Listener: Create a service class to act as a listener. For example, you might create a
CheckAgeListener.php
in thesrc/EventListener
directory. Implement Listener Logic: The listener class should implement an event listener. For example:
use Symfony\Component\HttpKernel\Event\RequestEvent; class CheckAgeListener { public function onKernelRequest(RequestEvent $event) { $request = $event->getRequest(); if ($request->get('age') < 18) { $event->setResponse(new RedirectResponse('/home')); } } }
Copy after loginRegister the Listener: Register your listener in the
config/services.yaml
file. For example:services: App\EventListener\CheckAgeListener: tags: - { name: kernel.event_listener, event: kernel.request, method: onKernelRequest }
Copy after login- Apply Listener: The listener will now be automatically invoked for each request, and you can further control its scope using event priorities and configurations as needed.
How can middleware be effectively utilized to enhance security in web applications?
Middleware plays a crucial role in enhancing the security of web applications. Here are some ways it can be utilized effectively:
-
Authentication and Authorization: Middleware can check whether a user is authenticated before allowing access to certain routes or functionalities. For example, in Laravel, you can use the
auth
middleware to ensure that only authenticated users can access certain parts of your application. - Input Validation and Sanitization: Middleware can inspect incoming data to validate and sanitize it, helping to prevent common security threats like SQL injection and cross-site scripting (XSS). This can be achieved by creating custom middleware that uses validation libraries or rules.
- CSRF Protection: Both Laravel and Symfony include built-in middleware for Cross-Site Request Forgery (CSRF) protection. This middleware adds a token to all outgoing requests and verifies it on incoming requests, enhancing the security of your application against CSRF attacks.
- Rate Limiting: Middleware can be used to implement rate limiting, which helps protect against brute-force attacks by limiting the number of requests a user can make within a certain timeframe.
- IP Whitelisting/Blacklisting: Middleware can enforce IP-based access control, allowing or denying requests based on the client's IP address. This can be used to protect administrative routes or limit access to specific parts of your application.
- Logging and Monitoring: Middleware can log requests and responses, which can be used for monitoring suspicious activities and detecting potential security breaches. This is essential for incident response and forensic analysis.
What are some common use cases for middleware in Laravel and Symfony frameworks?
Middleware in both Laravel and Symfony can be used for a variety of tasks. Here are some common use cases:
-
Authentication and Authorization:
- In Laravel, middleware like
auth
andguest
are used to manage user sessions and access controls. - In Symfony, similar functionality can be achieved with event listeners that check authentication status.
- In Laravel, middleware like
-
Logging:
- Middleware can be used to log requests and responses for debugging and auditing purposes. For example, Laravel has a built-in
log
middleware, while Symfony can achieve similar functionality through custom event listeners.
- Middleware can be used to log requests and responses for debugging and auditing purposes. For example, Laravel has a built-in
-
Input Validation and Transformation:
- Middleware can be used to validate incoming request data before it reaches the controller. In Laravel, you might use a custom middleware to validate and possibly transform data.
- Symfony can use request listeners to validate and transform data before it reaches the controller action.
-
CSRF Protection:
- Both frameworks offer built-in middleware for CSRF protection, ensuring that only legitimate requests are processed.
-
Localization and Internationalization:
- Middleware can be employed to set the correct locale based on user preferences or browser settings. Laravel’s
locale
middleware and Symfony’s locale listeners serve this purpose.
- Middleware can be employed to set the correct locale based on user preferences or browser settings. Laravel’s
-
Maintenance Mode and Redirects:
- Middleware can be used to redirect users to a maintenance page or handle redirects based on certain conditions. Laravel’s
maintenance
middleware and Symfony’s event listeners can be configured for this.
- Middleware can be used to redirect users to a maintenance page or handle redirects based on certain conditions. Laravel’s
-
Rate Limiting:
- Middleware can enforce rate limits to prevent abuse of your application’s API or web services. Laravel’s
throttle
middleware and Symfony’s custom listeners can be used to implement this.
- Middleware can enforce rate limits to prevent abuse of your application’s API or web services. Laravel’s
-
Caching:
- Middleware can be used to cache responses, improving the performance of your application. Laravel’s
cache.headers
middleware and Symfony’s response listeners can be configured to handle caching.
- Middleware can be used to cache responses, improving the performance of your application. Laravel’s
By leveraging middleware effectively, developers can create more robust, secure, and efficient web applications using Laravel and Symfony frameworks.
The above is the detailed content of Laravel/Symfony Middleware: Creating and using middleware.. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
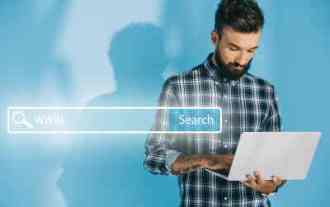
11 Best PHP URL Shortener Scripts (Free and Premium)
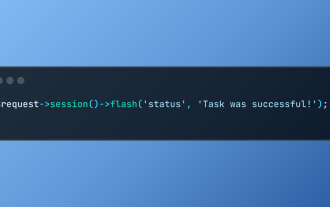
Working with Flash Session Data in Laravel
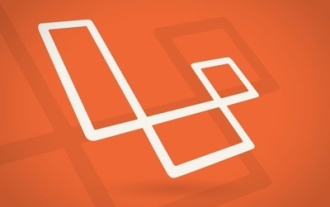
Build a React App With a Laravel Back End: Part 2, React
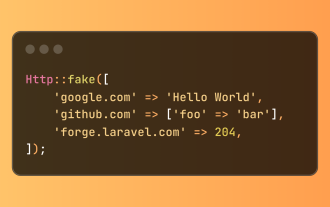
Simplified HTTP Response Mocking in Laravel Tests
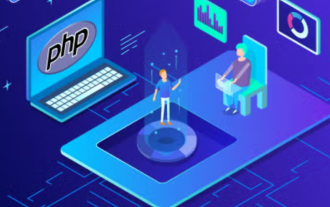
cURL in PHP: How to Use the PHP cURL Extension in REST APIs
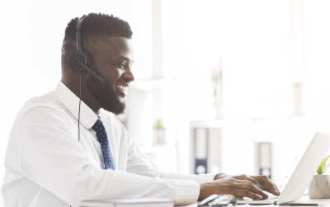
12 Best PHP Chat Scripts on CodeCanyon
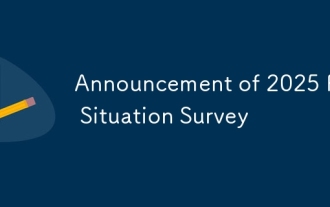
Announcement of 2025 PHP Situation Survey
