Service Container in Laravel/Symfony: How it enables DI.
Mar 28, 2025 pm 05:13 PMService Container in Laravel/Symfony: How it enables DI
Dependency Injection (DI) is a design pattern that allows the removal of hard-coded dependencies and makes it possible to change them, whether at runtime or compile time. In Laravel and Symfony, the Service Container is a powerful tool that facilitates Dependency Injection by managing class dependencies and injecting them where needed.
The Service Container acts as a registry for services, which can be any object that provides a specific functionality. It instantiates and configures these services as needed. In both frameworks, it's known as the IoC (Inversion of Control) container, and it plays a crucial role in promoting loose coupling between classes.
The Service Container in Laravel and Symfony enables DI by allowing developers to define services and their dependencies in configuration files or directly in code. When a class needs a dependency, it can be requested from the container rather than being instantiated directly. This not only decouples the dependent class from the specifics of the dependency but also allows for easy swapping of different implementations of the same interface, thereby enhancing testability and maintainability.
What are the benefits of using a service container for dependency injection in Laravel/Symfony?
Using a service container for dependency injection in Laravel and Symfony offers several key benefits:
- Loose Coupling: By injecting dependencies through the service container, classes are decoupled from the concrete implementation of their dependencies. This makes the system more modular and easier to maintain.
- Testability: With DI enabled by the service container, it's easier to write unit tests. You can easily swap out real implementations with mock objects for testing purposes.
- Flexibility and Configurability: The service container allows for easy configuration of services and their dependencies. You can define different behaviors for different environments (development, testing, production) without changing the application code.
- Performance: The service container in Laravel and Symfony caches configurations and instantiated services, reducing the overhead of creating and configuring objects repeatedly.
- Centralized Management: All services and their dependencies are defined in a centralized location, making it easier to manage complex dependency graphs and understand the overall structure of the application.
How does the service container in Laravel/Symfony simplify the management of dependencies?
The service container in Laravel and Symfony simplifies the management of dependencies in several ways:
- Automatic Instantiation: The service container automatically instantiates objects and injects their dependencies. This reduces the boilerplate code needed to create and configure objects manually.
- Dependency Graph Management: It manages the complex graph of dependencies, ensuring that all dependencies are correctly instantiated and configured before they are injected into other services.
- Lazy Loading: The container can lazy-load services, meaning that they are only instantiated when they are actually needed. This can improve the initial load time of an application.
- Configuration and Binding: Developers can bind interfaces to their implementations and configure how services are created and how their dependencies are resolved, all in a centralized configuration.
- Error Handling: The container can throw meaningful exceptions when dependencies cannot be resolved, helping developers identify and fix configuration issues more easily.
Can you explain how to configure and use the service container for dependency injection in Laravel/Symfony?
In both Laravel and Symfony, configuring and using the service container for dependency injection involves a few key steps:
Laravel:
-
Defining Services: In Laravel, you can define services in the
App\Providers\AppServiceProvider.php
file, particularly within theregister
method. For example:public function register() { $this->app->bind('App\Contracts\PaymentGateway', function ($app) { return new \App\Services\StripePaymentGateway(); }); }
Copy after login Using Services: You can then inject this service into a class using constructor injection:
class OrderController extends Controller { private $paymentGateway; public function __construct(PaymentGateway $paymentGateway) { $this->paymentGateway = $paymentGateway; } // Use $this->paymentGateway in your methods }
Copy after login
Symfony:
Defining Services: In Symfony, services are typically defined in YAML, XML, or PHP configuration files under the
config/services
directory. For example, inconfig/services.yaml
:services: App\Service\StripePaymentGateway: public: false autowire: true autoconfigure: true
Copy after loginUsing Services: Similar to Laravel, you can inject services into your classes using constructor injection:
use App\Service\StripePaymentGateway; class OrderController extends AbstractController { private $paymentGateway; public function __construct(StripePaymentGateway $paymentGateway) { $this->paymentGateway = $paymentGateway; } // Use $this->paymentGateway in your methods }
Copy after login
In both frameworks, the service container manages the lifecycle of the services and ensures that dependencies are correctly injected. This setup allows for a clean, maintainable architecture where dependencies are managed centrally and injected automatically where needed.
The above is the detailed content of Service Container in Laravel/Symfony: How it enables DI.. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
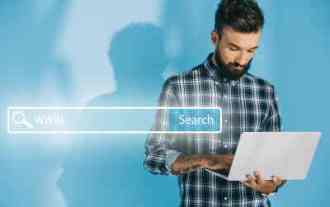
11 Best PHP URL Shortener Scripts (Free and Premium)
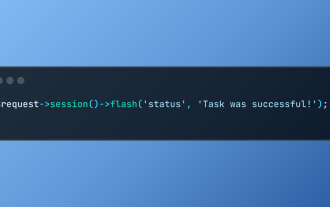
Working with Flash Session Data in Laravel
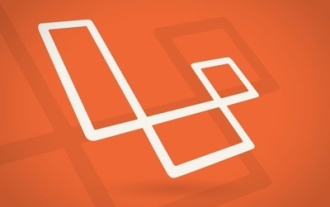
Build a React App With a Laravel Back End: Part 2, React
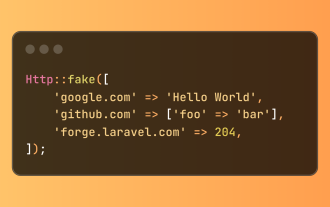
Simplified HTTP Response Mocking in Laravel Tests
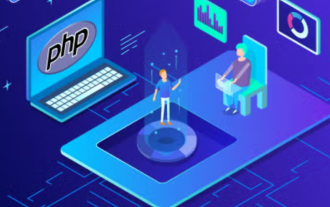
cURL in PHP: How to Use the PHP cURL Extension in REST APIs
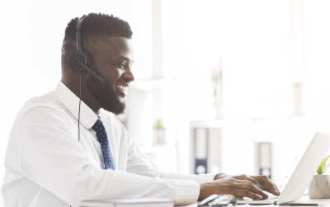
12 Best PHP Chat Scripts on CodeCanyon
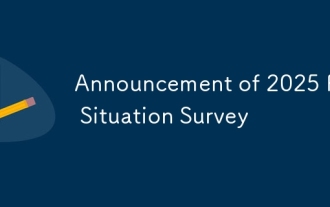
Announcement of 2025 PHP Situation Survey
