How do you handle legacy code in Python?
How do you handle legacy code in Python?
Handling legacy code in Python requires a strategic approach to ensure that it can be maintained and improved over time. Here are some steps to effectively manage legacy Python code:
- Understand the Codebase: Start by thoroughly reviewing the existing codebase to understand its structure, dependencies, and functionality. This can be aided by creating documentation or comments if they are lacking.
- Set Up a Safe Environment: Use version control systems like Git to create branches where you can safely test changes without affecting the main codebase.
-
Write Tests: If the legacy code lacks tests, start by writing unit tests or integration tests to ensure that any changes made do not break existing functionality. Tools like
unittest
orpytest
can be instrumental here. - Refactor Gradually: Instead of attempting a large-scale rewrite, refactor the code incrementally. Focus on small, manageable sections that can be improved without causing widespread disruption.
- Use Modern Python Features: Where possible, update the code to use more recent Python features and best practices, but be mindful of compatibility issues.
- Document Changes: Keep detailed records of changes made to the codebase, including why changes were necessary and how they affect the system.
- Engage the Team: If working in a team, ensure that everyone understands the legacy code and the refactoring process. Regular code reviews can help maintain code quality and share knowledge.
By following these steps, you can handle legacy Python code more effectively, making it easier to maintain and extend in the future.
What are the best practices for refactoring legacy Python code?
Refactoring legacy Python code is crucial for improving its maintainability and performance. Here are some best practices to follow:
- Incremental Refactoring: Tackle refactoring in small, manageable chunks. Focus on one module or function at a time to minimize the risk of introducing bugs.
- Preserve Functionality: Ensure that the refactored code behaves exactly as the original code did. Use automated tests to verify this.
- Improve Code Readability: Use clear and descriptive naming conventions, add comments where necessary, and break down complex functions into smaller, more manageable ones.
-
Eliminate Code Smells: Look for and address common code smells such as duplicated code, long methods, and large classes. Tools like
pylint
can help identify these issues. - Adopt Modern Python Constructs: Update the code to use more recent Python features and idioms, such as list comprehensions, context managers, and type hints, where appropriate.
-
Refactor with Tests: Write tests before refactoring to ensure that the code's behavior remains unchanged. Use tools like
pytest
to create and run these tests. - Use Version Control: Commit changes frequently and use branches to isolate refactoring efforts. This allows you to revert changes if something goes wrong.
- Code Review: Have peers review your refactored code to catch any issues you might have missed and to share knowledge about the codebase.
By adhering to these best practices, you can effectively refactor legacy Python code, making it more maintainable and efficient.
How can you ensure compatibility when updating legacy Python code?
Ensuring compatibility when updating legacy Python code is essential to prevent disruptions in existing systems. Here are some strategies to achieve this:
-
Use Virtual Environments: Create isolated environments using tools like
venv
orconda
to test updates without affecting the production environment. -
Maintain Multiple Python Versions: If the legacy code runs on an older version of Python, ensure that you can test it on both the old and new versions. Tools like
tox
can help automate this process. - Backward Compatibility: When updating code, ensure that it remains compatible with the older version of Python it was originally written for. This might involve using conditional imports or feature detection.
-
Dependency Management: Use tools like
pip
andrequirements.txt
to manage dependencies. Ensure that any updated dependencies are compatible with the existing codebase. - Automated Testing: Implement a robust suite of tests that cover the existing functionality. Run these tests on both the old and new versions of the code to ensure that updates do not break anything.
-
Code Analysis Tools: Use tools like
pylint
ormypy
to analyze the code for potential compatibility issues before deploying updates. - Incremental Updates: Update the code incrementally, testing each change thoroughly before moving on to the next. This helps isolate any compatibility issues that arise.
- Documentation and Communication: Keep detailed documentation of changes and communicate with stakeholders about any potential impacts on compatibility.
By following these strategies, you can ensure that updates to legacy Python code maintain compatibility with existing systems.
What tools are most effective for analyzing legacy Python code?
Analyzing legacy Python code is crucial for understanding its structure and identifying areas for improvement. Here are some of the most effective tools for this purpose:
- Pylint: Pylint is a static code analysis tool that checks for errors, enforces a coding standard, and looks for code smells. It's particularly useful for identifying issues in legacy code.
- Pyflakes: Pyflakes is a lightweight tool that checks Python source code for errors. It's fast and can be integrated into development environments to provide real-time feedback.
- Mypy: Mypy is a static type checker for Python. It can help identify type-related issues in legacy code, especially if you're planning to add type hints during refactoring.
- Bandit: Bandit is a tool designed to find common security issues in Python code. It's useful for ensuring that legacy code does not contain vulnerabilities.
- Radon: Radon is a Python tool that computes various metrics from the source code, such as cyclomatic complexity, raw metrics, and maintainability index. It's helpful for assessing the complexity of legacy code.
- Pytest: While primarily a testing framework, Pytest can be used to write and run tests for legacy code, helping to ensure that any changes do not break existing functionality.
- Coverage.py: This tool measures code coverage during testing. It's useful for identifying parts of the legacy code that are not covered by tests, which can be risky areas for refactoring.
- Sourcery: Sourcery is an AI-powered tool that provides refactoring suggestions. It can be particularly useful for identifying quick wins in legacy code.
By using these tools, you can gain a comprehensive understanding of your legacy Python code and make informed decisions about how to improve it.
The above is the detailed content of How do you handle legacy code in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


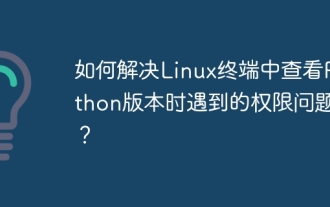
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
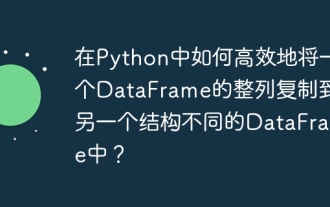
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
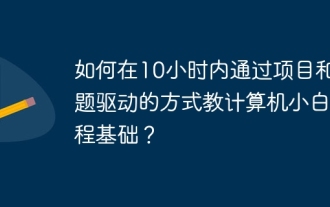
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
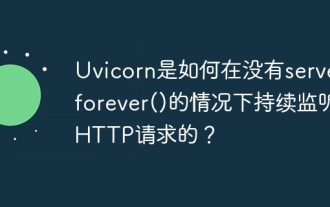
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
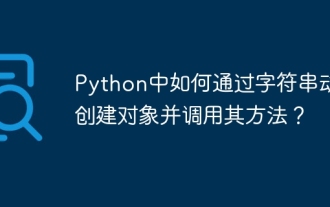
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
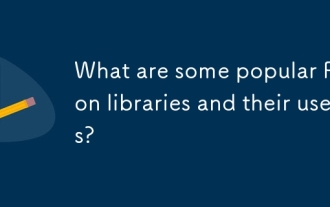
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
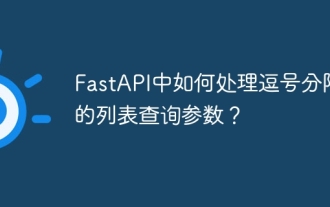
Fastapi ...
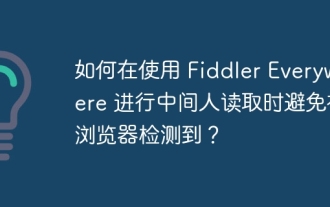
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
