


Design a system for managing user sessions in a web application.
Design a system for managing user sessions in a web application.
Designing a system for managing user sessions in a web application involves creating a structured approach to handle user authentication, session data storage, and session management. Here’s a step-by-step guide to designing such a system:
-
Authentication Mechanism:
- Implement a secure login system where users can authenticate themselves using credentials like username and password, or other methods like social login or multi-factor authentication.
- Use industry-standard protocols like OAuth, OpenID Connect, or SAML for single sign-on (SSO) capabilities.
-
Session Creation:
- Upon successful authentication, generate a unique session ID. This ID should be cryptographically secure and difficult to guess or predict.
- Store the session ID in a cookie on the user's browser and keep a reference on the server side, often in a session store or database.
-
Session Data Management:
- Decide on the data to be stored in sessions. This may include user preferences, temporary data, or other relevant information.
- Implement a session store, which could be in-memory, database-backed, or a distributed cache like Redis or Memcached.
-
Session Validation and Renewal:
- Validate the session ID with each request to ensure it's still valid.
- Implement mechanisms for session renewal or timeout to manage session length and security. Set appropriate timeout periods and refresh them based on user activity.
-
Session Termination:
- Provide options for users to log out, which should invalidate the session on both client and server sides.
- Implement automatic session termination upon inactivity or when suspicious activities are detected.
-
Scalability and Performance:
- Ensure the session management system can scale with your application. Use distributed session stores if necessary to handle high loads.
- Optimize session data storage and retrieval to minimize latency.
-
Monitoring and Logging:
- Implement logging to track session creation, renewal, and termination events.
- Monitor session-related metrics to detect anomalies and potential security issues.
By following these steps, you can design a robust and secure system for managing user sessions in a web application.
What are the key features to consider when designing a user session management system?
When designing a user session management system, several key features should be considered to ensure it is effective, secure, and user-friendly:
-
Security:
- Implement strong encryption for session IDs and data.
- Use secure protocols for session transmission (e.g., HTTPS).
- Implement session fixation and hijacking prevention measures.
-
Scalability:
- Design the system to handle a growing number of users without performance degradation.
- Use distributed session stores to manage load across multiple servers.
-
Performance:
- Optimize session data storage and retrieval to minimize latency.
- Implement caching mechanisms to reduce database load.
-
User Experience:
- Ensure seamless session management that doesn’t interrupt user activities.
- Provide clear options for session management, such as logout and session renewal.
-
Session Timeout and Renewal:
- Set appropriate session timeout periods to balance security and user convenience.
- Implement automatic session renewal based on user activity.
-
Data Integrity and Consistency:
- Ensure session data is consistent across different parts of the application.
- Implement mechanisms to handle concurrent session updates.
-
Monitoring and Logging:
- Log session-related events for auditing and troubleshooting.
- Monitor session metrics to detect and respond to security threats.
-
Flexibility and Customization:
- Allow for customization of session management policies to fit different use cases.
- Support integration with various authentication mechanisms and third-party services.
By focusing on these key features, you can create a user session management system that meets the needs of both the application and its users.
How can you ensure the security of user sessions in a web application?
Ensuring the security of user sessions in a web application is crucial to protect user data and prevent unauthorized access. Here are several strategies to enhance session security:
-
Use HTTPS:
- Always use HTTPS to encrypt data transmitted between the client and server, including session IDs.
-
Secure Session IDs:
- Generate session IDs using cryptographically secure random number generators.
- Ensure session IDs are long enough to prevent brute-force attacks.
-
Session Fixation Protection:
- Regenerate session IDs after successful authentication to prevent session fixation attacks.
- Use the
HttpOnly
andSecure
flags on session cookies to prevent client-side script access and ensure transmission over HTTPS.
-
Session Timeout and Inactivity:
- Implement session timeouts to automatically terminate inactive sessions.
- Set appropriate timeout periods and renew sessions based on user activity.
-
IP and User Agent Checking:
- Validate the user’s IP address and user agent with each request to detect session hijacking attempts.
- Be cautious with this approach as legitimate users may change IP addresses or user agents.
-
Data Encryption:
- Encrypt sensitive session data stored on the server side.
- Use secure encryption algorithms and key management practices.
-
Session Termination:
- Provide clear options for users to log out, which should invalidate the session on both client and server sides.
- Implement automatic session termination upon detecting suspicious activities.
-
Regular Security Audits:
- Conduct regular security audits and penetration testing to identify and fix vulnerabilities.
- Monitor session-related logs for unusual patterns that may indicate security breaches.
-
Implement Multi-Factor Authentication (MFA):
- Use MFA to add an extra layer of security, making it harder for attackers to gain unauthorized access.
By implementing these security measures, you can significantly enhance the security of user sessions in your web application.
What methods can be used to optimize the performance of session handling in a web application?
Optimizing the performance of session handling in a web application is essential for ensuring a smooth user experience and efficient resource utilization. Here are several methods to achieve this:
-
Use of In-Memory Session Stores:
- Store session data in memory (e.g., using Redis or Memcached) to reduce database load and improve access times.
- Ensure the in-memory store is properly configured for high availability and data persistence.
-
Session Data Minimization:
- Store only essential data in sessions to reduce the size of session data and improve retrieval times.
- Use other storage mechanisms for non-essential data that doesn’t need to be accessed frequently.
-
Caching:
- Implement caching mechanisms to store frequently accessed session data, reducing the need to fetch data from the session store.
- Use distributed caching solutions to handle high loads and ensure data consistency across multiple servers.
-
Asynchronous Session Handling:
- Use asynchronous programming techniques to handle session operations without blocking the main application thread.
- Implement non-blocking I/O operations for session data retrieval and storage.
-
Session Clustering:
- Use session clustering to distribute session data across multiple servers, improving scalability and fault tolerance.
- Ensure session data synchronization across the cluster to maintain data consistency.
-
Optimized Session Serialization:
- Use efficient serialization formats (e.g., Protocol Buffers, MessagePack) to reduce the overhead of storing and retrieving session data.
- Implement compression techniques for session data to reduce storage and transmission overhead.
-
Load Balancing:
- Implement load balancing to distribute session requests evenly across multiple servers, preventing any single server from becoming a bottleneck.
- Use sticky sessions or session replication to ensure session continuity across load-balanced servers.
-
Session Timeout Optimization:
- Set appropriate session timeout periods to balance security and performance.
- Implement session renewal based on user activity to prevent unnecessary session terminations.
-
Monitoring and Profiling:
- Use monitoring tools to track session-related performance metrics and identify bottlenecks.
- Conduct regular performance profiling to optimize session handling code and data structures.
By applying these methods, you can significantly improve the performance of session handling in your web application, leading to a better user experience and more efficient resource utilization.
The above is the detailed content of Design a system for managing user sessions in a web application.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


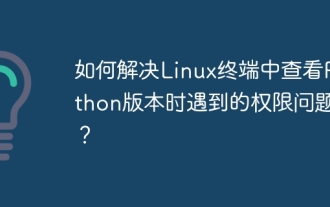
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
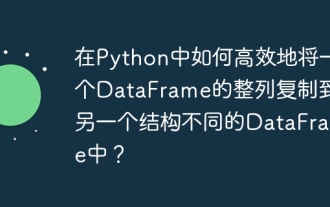
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
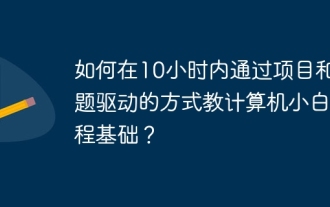
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
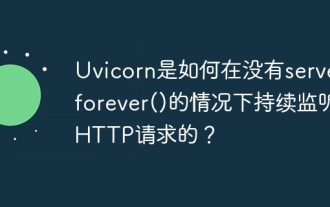
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
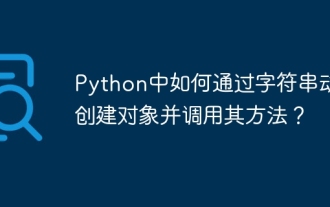
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
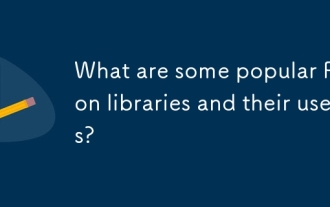
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
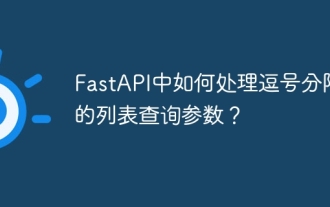
Fastapi ...
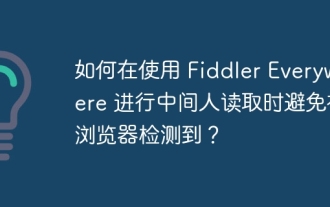
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
