


How do you use linters and code formatters (e.g., ESLint, Prettier) to enforce code style?
How do you use linters and code formatters (e.g., ESLint, Prettier) to enforce code style?
Linters and code formatters such as ESLint and Prettier are essential tools in modern software development, used to enforce and maintain a consistent code style across a project. Here’s a detailed explanation of how to use these tools:
ESLint: ESLint is a static code analysis tool used to identify problematic patterns and enforce coding standards in JavaScript and TypeScript projects. To use ESLint, follow these steps:
-
Installation: Install ESLint as a development dependency in your project using npm or yarn:
<code>npm install eslint --save-dev</code>
Copy after loginor
<code>yarn add eslint --dev</code>
Copy after login -
Configuration: Create an
.eslintrc
file to define your coding standards and rules. This file can be in JSON, YAML, or JavaScript format. You can either manually create this file or use the ESLint CLI to initialize it:<code>npx eslint --init</code>
Copy after login -
Integration: Integrate ESLint into your development workflow. This can be done by running ESLint manually via the command line or integrating it into your IDE/editor for real-time feedback. You can add ESLint to your
package.json
scripts for easy running:<code>"scripts": { "lint": "eslint ." }</code>
Copy after login - Automating: To automate ESLint checks, incorporate it into your CI/CD pipeline to ensure that all code pushed to your repository meets the defined standards.
Prettier: Prettier is an opinionated code formatter that supports various programming languages including JavaScript, TypeScript, and CSS. Here’s how to use Prettier:
-
Installation: Install Prettier as a development dependency:
<code>npm install prettier --save-dev</code>
Copy after loginor
<code>yarn add prettier --dev</code>
Copy after login -
Configuration: While Prettier is opinionated and requires minimal configuration, you can still create a
.prettierrc
file to specify your formatting options. For example:{ "semi": false, "singleQuote": true }
Copy after login Integration: Similar to ESLint, integrate Prettier into your development environment. Many IDEs support Prettier out of the box, or you can use plugins/extensions. Add Prettier to your
package.json
scripts:<code>"scripts": { "format": "prettier --write ." }</code>
Copy after login- Automating: Run Prettier automatically by including it in pre-commit hooks or CI/CD pipelines to ensure consistent formatting before code is merged.
Both tools help enforce code style by catching and correcting deviations from the defined standards, thus maintaining a uniform codebase.
What are the best practices for configuring ESLint and Prettier to work together seamlessly?
To ensure ESLint and Prettier work together seamlessly, follow these best practices:
Disable Formatting Rules in ESLint: Since Prettier will handle code formatting, disable any ESLint rules that overlap with formatting to avoid conflicts. Use the
eslint-config-prettier
package to turn off all rules that are unnecessary or might conflict with Prettier:<code>npm install eslint-config-prettier --save-dev</code>
Copy after loginThen, extend it in your
.eslintrc
:{ "extends": ["eslint:recommended", "prettier"] }
Copy after loginRun Prettier Before ESLint: Prettier should run before ESLint to format the code first. This can be automated in your
package.json
scripts:<code>"scripts": { "lint": "prettier --write . && eslint ." }</code>
Copy after loginUse a Pre-Commit Hook: Utilize tools like
lint-staged
andhusky
to run both Prettier and ESLint as a pre-commit hook. This ensures your code is formatted and linted before it reaches the repository:<code>npm install lint-staged husky --save-dev</code>
Copy after loginThen, configure in
package.json
:"lint-staged": { "*.{js,ts,tsx}": [ "prettier --write", "eslint --fix" ] }, "husky": { "hooks": { "pre-commit": "lint-staged" } }
Copy after login-
Consistent Configuration Across Team: Ensure all team members use the same configuration files (
.eslintrc
,.prettierrc
) to maintain consistency. Keep these configuration files in version control. - Education and Training: Educate team members on the importance of these tools and how to use them. Regularly review and update the configurations to adapt to evolving project needs.
How can linters and code formatters improve code quality and maintainability in a development team?
Linters and code formatters significantly enhance code quality and maintainability in a development team through several mechanisms:
- Enforcing Consistency: By automating style checks and formatting, these tools ensure that all code follows the same standards. This reduces disputes over style and makes the codebase easier to navigate and understand.
- Reducing Errors: Linters like ESLint can detect potential errors and problematic patterns before they reach production. This helps prevent bugs and reduces the time spent on debugging.
- Improving Readability: Well-formatted code is easier to read and understand. Prettier helps maintain a clean and consistent code structure, which is crucial for code reviews and onboarding new team members.
- Saving Time: Automating the formatting process saves developers time, allowing them to focus more on logic and functionality rather than worrying about style. Additionally, integrating these tools into CI/CD pipelines can automate quality checks, reducing manual effort.
- Enhancing Collaboration: Consistent code style fosters better collaboration among team members. When everyone adheres to the same standards, code reviews become more efficient, and developers can more easily understand and contribute to different parts of the project.
- Facilitating Onboarding: New team members can quickly adapt to the codebase when it follows a uniform style. This reduces the learning curve and accelerates productivity.
- Maintaining Code Health: Regular use of linters and formatters encourages developers to continuously improve and maintain code quality, leading to a healthier, more maintainable codebase over time.
Can you explain the differences between ESLint and Prettier and when to use each tool?
ESLint and Prettier serve different purposes in the development process, though they complement each other well:
ESLint:
- Purpose: ESLint is primarily a linter used for identifying and reporting on patterns in JavaScript and TypeScript code. It focuses on code quality, best practices, and catching potential errors.
- Features: ESLint can be configured to enforce coding standards, detect problematic patterns, and suggest code improvements. It also has the ability to auto-fix certain issues.
- When to Use: Use ESLint throughout your development process for continuous code analysis. It's particularly useful during code reviews and as part of CI/CD pipelines to ensure code quality.
- Configuration: Highly configurable with extensive rule sets, allowing you to tailor it to your project's specific needs.
Prettier:
- Purpose: Prettier is a code formatter that focuses on the aesthetic aspect of code. It standardizes code formatting, making it consistent and readable.
- Features: Prettier automatically formats code according to its opinionated style, reducing debates over code style and ensuring uniformity.
- When to Use: Use Prettier as a pre-commit hook or as part of your development workflow to automatically format code before committing or pushing changes. It’s especially beneficial for ensuring that code submitted to the repository is consistently formatted.
- Configuration: Minimal configuration is required as Prettier enforces a set style, but you can customize some options if needed.
When to Use Each:
- Use ESLint for static code analysis, to catch errors, enforce best practices, and improve overall code quality.
- Use Prettier to automatically format code, ensuring it adheres to a consistent style across the codebase.
- Use Both Together to benefit from comprehensive code quality checks and consistent formatting. This combination ensures that your code is not only stylistically uniform but also adheres to high-quality standards.
By understanding and leveraging the strengths of both tools, you can significantly enhance your development process and maintain a high-quality codebase.
The above is the detailed content of How do you use linters and code formatters (e.g., ESLint, Prettier) to enforce code style?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.

The React ecosystem includes state management libraries (such as Redux), routing libraries (such as ReactRouter), UI component libraries (such as Material-UI), testing tools (such as Jest), and building tools (such as Webpack). These tools work together to help developers develop and maintain applications efficiently, improve code quality and development efficiency.
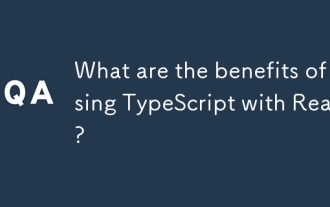
TypeScript enhances React development by providing type safety, improving code quality, and offering better IDE support, thus reducing errors and improving maintainability.

React is a front-end framework for building user interfaces; a back-end framework is used to build server-side applications. React provides componentized and efficient UI updates, and the backend framework provides a complete backend service solution. When choosing a technology stack, project requirements, team skills, and scalability should be considered.

The advantages of React are its flexibility and efficiency, which are reflected in: 1) Component-based design improves code reusability; 2) Virtual DOM technology optimizes performance, especially when handling large amounts of data updates; 3) The rich ecosystem provides a large number of third-party libraries and tools. By understanding how React works and uses examples, you can master its core concepts and best practices to build an efficient, maintainable user interface.
