What are the benefits of using React 18's automatic batching?
What are the benefits of using React 18's automatic batching?
React 18 introduced automatic batching, a feature that significantly enhances the way state updates are handled in React applications. The primary benefits of using automatic batching include:
- Improved Performance: Automatic batching groups multiple state updates into a single re-render, reducing the number of re-renders and thus improving the overall performance of the application. This is particularly beneficial in scenarios where multiple state updates occur in quick succession, such as within event handlers or asynchronous operations.
-
Simplified Code: Developers no longer need to manually wrap state updates in
unstable_batchedUpdates
to ensure efficient rendering. This simplification reduces the cognitive load on developers and makes the codebase cleaner and easier to maintain. - Consistency Across Platforms: Automatic batching ensures that state updates are batched consistently across different platforms, including the browser and server-side rendering. This consistency helps in creating more predictable and reliable applications.
- Better Handling of Asynchronous Operations: With automatic batching, state updates triggered by asynchronous operations, such as API calls or timeouts, are batched together, leading to fewer re-renders and a smoother user experience.
How does automatic batching in React 18 improve application performance?
Automatic batching in React 18 improves application performance in several ways:
- Reduced Number of Re-renders: By grouping multiple state updates into a single re-render, automatic batching minimizes the number of times the component tree needs to be re-rendered. This reduction in re-renders leads to faster execution and a more responsive user interface.
- Efficient Use of Resources: Fewer re-renders mean less work for the browser's rendering engine, resulting in more efficient use of CPU and memory resources. This is particularly important for applications running on resource-constrained devices or those with complex UI components.
- Smoother User Experience: With fewer re-renders, the application feels smoother and more responsive to user interactions. This is especially noticeable in scenarios where multiple state updates occur in quick succession, such as form submissions or data updates.
- Optimized Asynchronous Operations: Automatic batching ensures that state updates triggered by asynchronous operations are handled efficiently. This optimization is crucial for applications that rely heavily on asynchronous data fetching or processing, as it helps maintain a smooth and consistent user experience.
Can automatic batching in React 18 simplify state management in my app?
Yes, automatic batching in React 18 can simplify state management in your app in several ways:
-
Elimination of Manual Batching: Before React 18, developers had to manually use
unstable_batchedUpdates
to batch state updates, especially in event handlers or asynchronous operations. Automatic batching removes this need, simplifying the state management process. - Reduced Complexity: With automatic batching, developers can focus more on the logic of their application rather than worrying about optimizing state updates. This reduction in complexity makes it easier to manage state, especially in larger and more complex applications.
- Consistency Across Different Scenarios: Automatic batching ensures that state updates are handled consistently, whether they occur synchronously or asynchronously. This consistency simplifies state management by reducing the need for special handling of different types of state updates.
- Easier Debugging: With fewer re-renders and a more predictable state update process, debugging state-related issues becomes easier. Developers can more easily trace the flow of state updates and identify any unexpected behavior.
What specific scenarios benefit most from React 18's automatic batching feature?
React 18's automatic batching feature is particularly beneficial in the following scenarios:
- Event Handlers: When multiple state updates occur within a single event handler, such as a button click that updates multiple pieces of state, automatic batching ensures that these updates are grouped into a single re-render, improving performance and user experience.
- Asynchronous Operations: Applications that rely heavily on asynchronous operations, such as API calls or timeouts, benefit greatly from automatic batching. It ensures that state updates triggered by these operations are batched together, leading to fewer re-renders and a smoother user experience.
- Complex Forms: In applications with complex forms where multiple fields need to be updated simultaneously, automatic batching helps in reducing the number of re-renders, making the form interactions smoother and more responsive.
- Data-Driven Applications: Applications that frequently update based on incoming data, such as real-time dashboards or live updates, can leverage automatic batching to handle multiple state updates efficiently, ensuring a consistent and smooth user experience.
- Server-Side Rendering: For applications that use server-side rendering, automatic batching ensures consistent behavior across different rendering environments, simplifying the development and maintenance of such applications.
The above is the detailed content of What are the benefits of using React 18's automatic batching?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


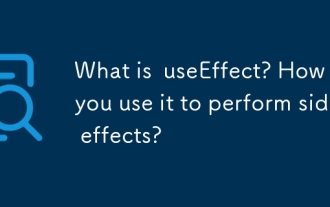
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
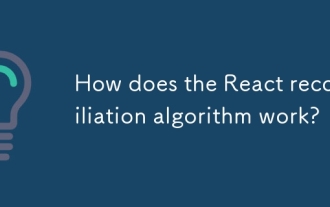
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
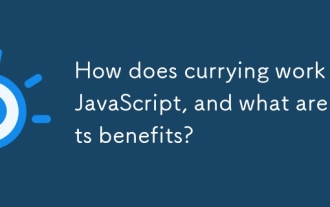
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
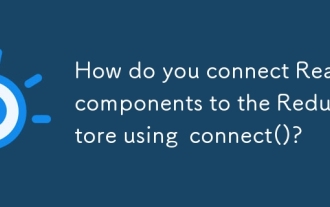
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
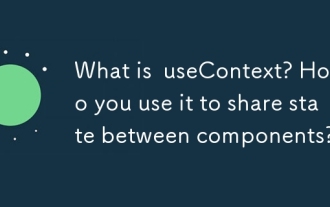
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
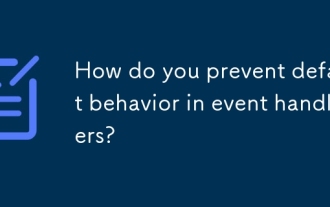
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
