


How do you use server-side rendering (SSR) with Vue.js? What are the benefits?
How do you use server-side rendering (SSR) with Vue.js? What are the benefits?
To use server-side rendering (SSR) with Vue.js, you'll need to create a server that can handle the rendering of Vue components on the server side before sending the fully rendered HTML to the client. Here’s a step-by-step approach to implementing SSR in Vue.js:
- Set up a Node.js server: Use a server framework like Express.js or Koa to handle HTTP requests and responses.
- Bundle your application: Use a tool like Webpack to create a server bundle that can be used for server-side rendering.
-
Create the renderer: Utilize Vue Server Renderer (
vue-server-renderer
) to convert your Vue app into a string of HTML. - Handle the request: When a request comes in, create a new Vue instance, render it on the server, and send the rendered HTML to the client.
- Client-side hydration: Once the HTML is loaded on the client, Vue.js will attach event listeners and make the app interactive.
The benefits of using SSR with Vue.js include:
- Improved SEO: Search engines can crawl the rendered HTML more effectively since the content is already available when the page is loaded.
- Faster initial load time: Users see a fully rendered page immediately, which can be especially beneficial for users on slower internet connections.
- Better social media sharing: When sharing links, social media platforms can correctly preview the content because the HTML is available immediately.
- Enhanced user experience: Users can start interacting with the page content sooner, as the initial load is faster.
What are the steps to set up SSR in a Vue.js application?
Setting up SSR in a Vue.js application involves several steps:
-
Install Node.js and Vue CLI: Ensure you have Node.js installed and use Vue CLI to create a new project with SSR support.
vue create my-ssr-app cd my-ssr-app vue add ssr
Copy after login Configure the server: Set up a server using a framework like Express.js. Create a
server.js
file to handle HTTP requests.const express = require('express') const { createRenderer } = require('vue-server-renderer') const server = express() const renderer = createRenderer() server.get('*', (req, res) => { const context = { url: req.url } const app = require('./src/entry-server.js').default renderer.renderToString(app, context, (err, html) => { if (err) { if (err.code === 404) { res.status(404).end('Page not found') } else { res.status(500).end('Internal Server Error') } } else { res.end(html) } }) }) server.listen(8080)
Copy after loginCreate entry points: You need two entry points: one for the server (
entry-server.js
) and one for the client (entry-client.js
).entry-server.js
:import { createApp } from './main' export default context => { const { app, router } = createApp() router.push(context.url) return new Promise((resolve, reject) => { router.onReady(() => { const matchedComponents = router.getMatchedComponents() if (!matchedComponents.length) { return reject({ code: 404 }) } resolve(app) }, reject) }) }
Copy after loginentry-client.js
:import { createApp } from './main' const { app, router } = createApp() router.onReady(() => { app.$mount('#app') })
Copy after login
Modify
main.js
: Ensure yourmain.js
can be used to create the app for both server and client.import Vue from 'vue' import App from './App.vue' import { createRouter } from './router' export function createApp () { const router = createRouter() const app = new Vue({ router, render: h => h(App) }) return { app, router } }
Copy after login- Build and run: Use the build scripts provided by Vue CLI to build your application and run the server.
How does SSR improve the SEO of a Vue.js website?
Server-side rendering (SSR) improves the SEO of a Vue.js website in several ways:
- Immediate Content Availability: With SSR, the HTML content is generated on the server and sent to the client immediately. Search engine crawlers can index this content more effectively because they don't have to wait for JavaScript to load and execute.
- Better Crawling: Search engines can crawl and index the content more accurately since the initial HTML contains all the necessary content and metadata. This is particularly important for dynamic content that might be difficult for crawlers to access in a client-side rendered application.
- Improved Metadata: SSR allows for dynamic generation of meta tags, titles, and descriptions, which are crucial for SEO. These can be tailored for each page and updated dynamically based on the content being served.
- Reduced Bounce Rates: Faster initial load times can lead to lower bounce rates, which indirectly improves SEO as search engines consider user engagement metrics in their ranking algorithms.
What performance advantages does SSR offer for Vue.js applications?
Server-side rendering (SSR) offers several performance advantages for Vue.js applications:
- Faster Time to Content: SSR allows the server to send a fully rendered HTML page to the client, which means users can see the content much faster than if they had to wait for the JavaScript to load and render the page.
- Reduced Client-Side Load: By offloading the initial rendering to the server, the client's device has less work to do, which can be particularly beneficial for users on mobile devices or with slower internet connections.
- Improved Perceived Performance: Users perceive the application as faster because they can interact with the page content sooner. This can lead to a better user experience and potentially higher engagement.
- Efficient Resource Utilization: SSR can help in managing server resources more efficiently, especially when combined with caching strategies. The server can cache rendered pages and serve them quickly to subsequent requests, reducing the load on both the server and the client.
- Better Handling of Large Datasets: For applications that need to display large datasets, SSR can render the initial view of the data on the server, reducing the amount of data that needs to be processed on the client side initially.
The above is the detailed content of How do you use server-side rendering (SSR) with Vue.js? What are the benefits?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


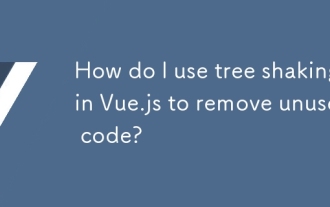
The article discusses using tree shaking in Vue.js to remove unused code, detailing setup with ES6 modules, Webpack configuration, and best practices for effective implementation.Character count: 159
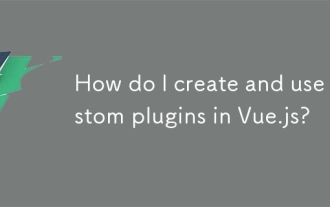
Article discusses creating and using custom Vue.js plugins, including development, integration, and maintenance best practices.

Vue.js is mainly used for front-end development. 1) It is a lightweight and flexible JavaScript framework focused on building user interfaces and single-page applications. 2) The core of Vue.js is its responsive data system, and the view is automatically updated when the data changes. 3) It supports component development, and the UI can be split into independent and reusable components.
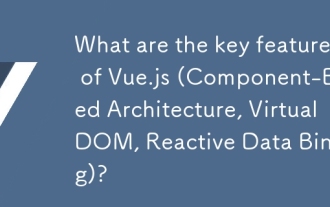
Vue.js enhances web development with its Component-Based Architecture, Virtual DOM for performance, and Reactive Data Binding for real-time UI updates.
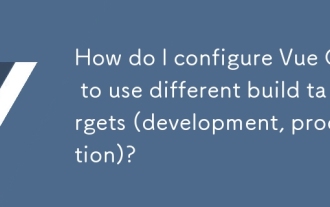
The article explains how to configure Vue CLI for different build targets, switch environments, optimize production builds, and ensure source maps in development for debugging.

The article discusses using Vue with Docker for deployment, focusing on setup, optimization, management, and performance monitoring of Vue applications in containers.
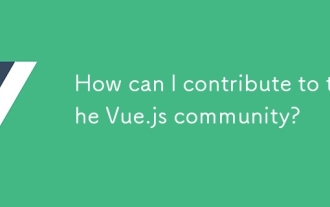
The article discusses various ways to contribute to the Vue.js community, including improving documentation, answering questions, coding, creating content, organizing events, and financial support. It also covers getting involved in open-source proje

Vue.js is not difficult to learn, especially for developers with a JavaScript foundation. 1) Its progressive design and responsive system simplify the development process. 2) Component-based development makes code management more efficient. 3) The usage examples show basic and advanced usage. 4) Common errors can be debugged through VueDevtools. 5) Performance optimization and best practices, such as using v-if/v-show and key attributes, can improve application efficiency.
