


How does singleton mode behave in multi-threaded and multi-process environments?
Analysis of multithreading and multiprocess behavior of Python singleton pattern
Singleton pattern is designed to ensure that a class has only one instance and provides a global access point. But in Python's multi-threaded and multi-process environments, its performance is different. This article will explore this difference in depth and illustrate it with code examples.
First, let's look at a simple singleton pattern implementation:
import multiprocessing import threading import time def singleton(cls): _instance = {} def inner(): if cls not in _instance: _instance[cls] = cls() return _instance[cls] Return inner @singleton class SingletonClass: count = 0 def __init__(self): SingletonClass.count = 1 def worker(name): for _ in range(10): instance = SingletonClass() instance.count = 1 time.sleep(0.1) print(f"{name}: count = {SingletonClass.count}, id = {id(instance)}") if __name__ == '__main__': # Multithreaded test threads = [threading.Thread(target=worker, args=(f"Thread-{i}",)) for i in range(2)] for thread in threads: thread.start() for thread in threads: thread.join() # Multi-process testing (comment out the multi-threaded part and run it) # processes = [multiprocessing.Process(target=worker, args=(f"Process-{i}",)) for i in range(2)] # for process in processes: # process.start() # for process in processes: # process.join()
In this example, the singleton
decorator ensures SingletonClass
' singleton property. worker
function simulates access to a singleton object by multiple threads or processes.
Run the multithreaded part and you will find that all threads share the same SingletonClass
instance, the value of id(instance)
is always the same, and the count
variable is incremented correctly.
However, if you uncomment the multi-threaded part and run the multi-process part, you will observe that each process creates its own SingletonClass
instance, the value of id(instance)
varies in different processes, and count
variable increments independently in each process.
This is because:
- Multithreading: All threads share the memory space of the same process, so the global variable
_instance
of the singleton pattern is visible to all threads, thus ensuring the uniqueness of the singleton. - Multi-process: Each process has an independent memory space, so each process has its own independent copy of the
_instance
variable, resulting in each process creating a new instance ofSingletonClass
.
Therefore, in a multi-process environment, the above simple singleton pattern implementation cannot guarantee the uniqueness of singletons. If you need to implement a true singleton pattern in a multiprocessing environment, you need to adopt more advanced techniques, such as using multiprocessing.Manager
to create shared memory or use inter-process communication mechanisms.
This modified example more clearly demonstrates the behavioral differences of singleton pattern in multi-threaded and multi-process environments and explains its root cause. It avoids redundant code from the original example and illustrates key concepts more concisely.
The above is the detailed content of How does singleton mode behave in multi-threaded and multi-process environments?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


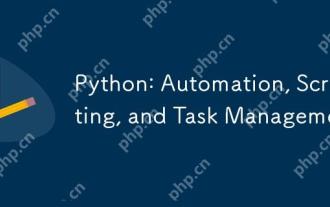
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
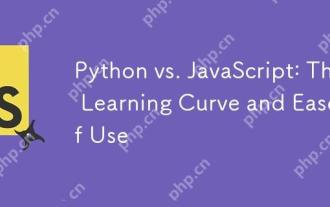
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
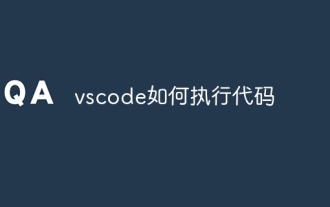
Executing code in VS Code only takes six steps: 1. Open the project; 2. Create and write the code file; 3. Open the terminal; 4. Navigate to the project directory; 5. Execute the code with the appropriate commands; 6. View the output.
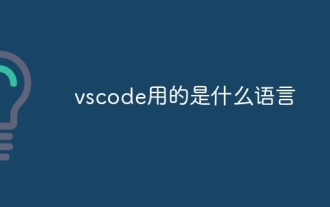
Visual Studio Code (VSCode) is developed by Microsoft, built using the Electron framework, and is mainly written in JavaScript. It supports a wide range of programming languages, including JavaScript, Python, C, Java, HTML, CSS, etc., and can add support for other languages through extensions.

The main differences between VS Code and PyCharm are: 1. Extensibility: VS Code is highly scalable and has a rich plug-in market, while PyCharm has wider functions by default; 2. Price: VS Code is free and open source, and PyCharm is paid for professional version; 3. User interface: VS Code is modern and friendly, and PyCharm is more complex; 4. Code navigation: VS Code is suitable for small projects, and PyCharm is more suitable for large projects; 5. Debugging: VS Code is basic, and PyCharm is more powerful; 6. Code refactoring: VS Code is basic, and PyCharm is richer; 7. Code
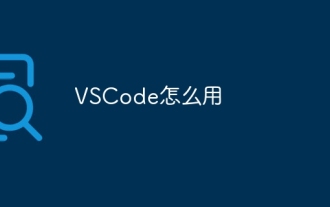
Visual Studio Code (VSCode) is a cross-platform, open source and free code editor developed by Microsoft. It is known for its lightweight, scalability and support for a wide range of programming languages. To install VSCode, please visit the official website to download and run the installer. When using VSCode, you can create new projects, edit code, debug code, navigate projects, expand VSCode, and manage settings. VSCode is available for Windows, macOS, and Linux, supports multiple programming languages and provides various extensions through Marketplace. Its advantages include lightweight, scalability, extensive language support, rich features and version
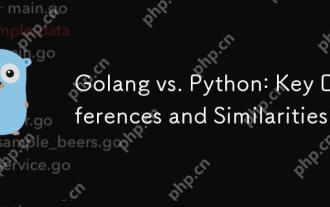
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
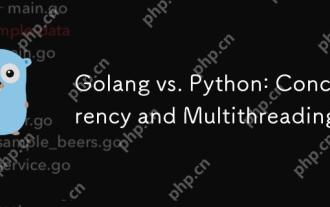
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
