


How does Flask streaming simulate real-time response of ChatGPT?
Simulate ChatGPT real-time response using Flask streaming
Many applications, such as real-time chats that simulate ChatGPT or large file downloads, need to generate and transmit data while avoiding long waits on the client. This article demonstrates how to implement this streaming in the Python Flask framework and corrects flaws in the original code.
The original code tried to use yield
to implement streaming, but since the response
object returned only after the generate()
function ended, the browser must wait for all data to be generated before the content is displayed, which does not match the real-time response expectations.
Problem code:
from time import sleep from flask import Flask, Response, stream_with_context app = Flask(__name__) @app.route('/stream', methods=['GET']) def stream(): def generate(): for i in range(1, 21): print(i) yield f'this is item {i}\n' sleep(0.5) return Response(generate(), mimetype='text/plain') if __name__ == '__main__': app.run(debug=True)
Workaround: Use Flask's stream_with_context
decorator correctly. This decorator ensures that data is returned to the client immediately every time yield
is generated, enabling true streaming. Improved code:
from flask import stream_with_context, request, jsonify @app.route('/stream') def streamed_response(): def generate(): yield 'Hello' yield request.args.get('name', 'World') # Use get() to avoid KeyError yield '!' return jsonify({'message': list(stream_with_context(generate()))}) # Return to JSON format
stream_with_context
wraps the generate
function, causing data to be sent immediately every yield
. In the example, data generation is simple. In actual applications, generate
function may contain more complex logic (such as database queries or complex calculations), but the function of stream_with_context
is still to ensure timely transmission of data. request.args.get('name', 'World')
obtains data from request parameters, implements more flexible streaming, and uses the get()
method to deal with missing parameters to avoid KeyError
errors. Finally, using jsonify
to wrap the result into JSON format, which is more suitable for front-end processing.
Through the above improvements, the real-time response effect of ChatGPT can be effectively simulated.
The above is the detailed content of How does Flask streaming simulate real-time response of ChatGPT?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


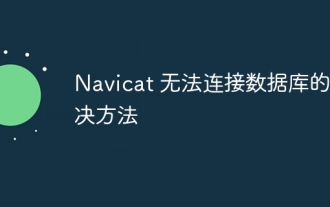
The following steps can be used to resolve the problem that Navicat cannot connect to the database: Check the server connection, make sure the server is running, address and port correctly, and the firewall allows connections. Verify the login information and confirm that the user name, password and permissions are correct. Check network connections and troubleshoot network problems such as router or firewall failures. Disable SSL connections, which may not be supported by some servers. Check the database version to make sure the Navicat version is compatible with the target database. Adjust the connection timeout, and for remote or slower connections, increase the connection timeout timeout. Other workarounds, if the above steps are not working, you can try restarting the software, using a different connection driver, or consulting the database administrator or official Navicat support.
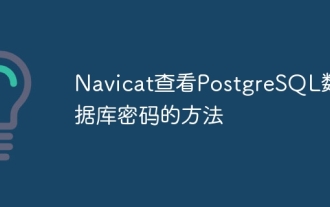
It is impossible to view PostgreSQL passwords directly from Navicat, because Navicat stores passwords encrypted for security reasons. To confirm the password, try to connect to the database; to modify the password, please use the graphical interface of psql or Navicat; for other purposes, you need to configure connection parameters in the code to avoid hard-coded passwords. To enhance security, it is recommended to use strong passwords, periodic modifications and enable multi-factor authentication.
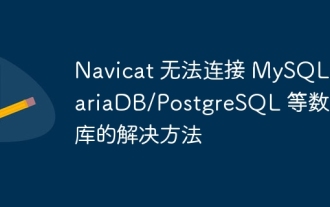
Common reasons why Navicat cannot connect to the database and its solutions: 1. Check the server's running status; 2. Check the connection information; 3. Adjust the firewall settings; 4. Configure remote access; 5. Troubleshoot network problems; 6. Check permissions; 7. Ensure version compatibility; 8. Troubleshoot other possibilities.
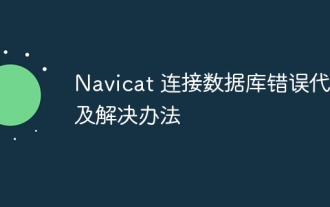
Common errors and solutions when connecting to databases: Username or password (Error 1045) Firewall blocks connection (Error 2003) Connection timeout (Error 10060) Unable to use socket connection (Error 1042) SSL connection error (Error 10055) Too many connection attempts result in the host being blocked (Error 1129) Database does not exist (Error 1049) No permission to connect to database (Error 1000)
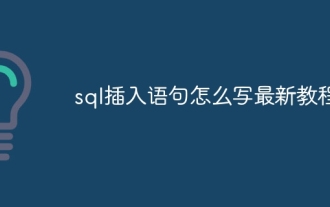
The SQL INSERT statement is used to add new rows to a database table, and its syntax is: INSERT INTO table_name (column1, column2, ..., columnN) VALUES (value1, value2, ..., valueN);. This statement supports inserting multiple values and allows NULL values to be inserted into columns, but it is necessary to ensure that the inserted values are compatible with the column's data type to avoid violating uniqueness constraints.
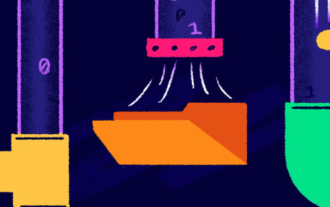
As a data professional, you need to process large amounts of data from various sources. This can pose challenges to data management and analysis. Fortunately, two AWS services can help: AWS Glue and Amazon Athena.

Add new columns to an existing table in SQL by using the ALTER TABLE statement. The specific steps include: determining the table name and column information, writing ALTER TABLE statements, and executing statements. For example, add an email column to the Customers table (VARCHAR(50)): ALTER TABLE Customers ADD email VARCHAR(50);
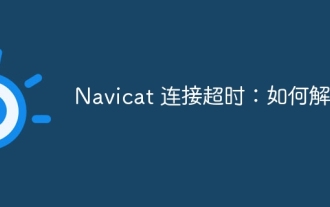
Reasons for Navicat connection timeout: network instability, busy database, firewall blocking, server configuration problems, and improper Navicat settings. Solution steps: Check network connection, database status, firewall settings, adjust server configuration, check Navicat settings, restart the software and server, and contact the administrator for help.
