Create a Tag Cloud with some Simple CSS and even Simpler JavaScript
I have always loved the tag cloud. I like to see which tags are the most popular user experience on the website by looking at the relative font sizes of tags, and the popular tags are bigger. They seem to be outdated, although you often see their versions in illustrations of tools like Wordle.
How difficult is it to create a tag cloud? Not difficult at all. let's see!
Let's start with the mark
For our HTML, we put each tag in a list,<ul class="tags"></ul>
. We will inject it into it using JavaScript. It would be great if your tag cloud already exists in HTML and you just want to do something relatively font size! Gradually enhanced! You should be able to tweak JavaScript later to do just that part, but not necessarily build and inject the tag itself.
I marked each attribute with a certain number of articles, mocking some JSON data. Let's write some JavaScript to get that JSON feed and do three things.
First, we will create one for each entry in the list<li>
. Imagine HTML so far is like this:
We then place the number of articles each attribute has in brackets next to each list item. Now, the tag looks like this:
Third and final step, we will create a link around each tag that points to the correct location. Here we can set the font size attribute for each item based on the number of articles marked for each attribute, so an animation with 13 articles will be much larger than a background-color with only 1 article.
JavaScript Part
Let's take a look at the JavaScript code that does this.
const dataURL = "https://gist.githubusercontent.com/markconroy/536228ed416a551de8852b74615e55dd/raw/9b96c9049b10e7e18ee922b4caf9167acb4efdd6/tags.json"; const tags = document.querySelector(".tags"); const fragment = document.createDocumentFragment(); const maxFontSizeForTag = 6; fetch(dataURL) .then(function (res) { return res.json(); }) .then(function (data) { // 1. Create a new array from data let orderedData = data.map((x) => x); // 2. Sort by the number of articles per tag orderedData.sort(function(a, b) { return a.tagged_articles.length - b.tagged_articles.length; }); orderedData = orderedData.reverse(); // 3. Get the value of the tag with the largest number of tags const highestValue = orderedData[0].tagged_articles.length; // 4. Create a list item for each result in data. data.forEach((result) => handleResult(result, highestValue)); // 5. Attach the complete tag list to tags element tags.appendChild(fragment); // Fixed: fragment should be attached here, not tags });
The JavaScript above uses the Fetch API to get the URL of the managed tags.json. Once this data is fetched, it returns it as JSON. Here we go on to a new array called orderedData (so we won't change the original array) and find the tags with the most posts. We will use this value later in the font scale so that all other labels have the font size associated with it. Then, for each result in the response, we call the function I named handleResult() and pass the result and the highestValue as arguments to the function. It also creates:
-
<li> A variable named tags that we will use to inject each list item created from the result,
<li> A variable for fragments to hold the result of each iteration of the loop, which we will append to tags later, as well
<li> A variable for maximum font size, which we will use later in the font scale.
Next is the handleResult(result) function:
function handleResult(result, highestValue) { // Set the variable const name = result.title; const link = result.href; const numberOfArticles = result.tagged_articles.length; let fontSize = numberOfArticles/highestValue * maxFontSizeForTag; fontSize = fontSize.toFixed(2); const fontSizeProperty = `${fontSize}em`; // Create a list element for each tag and inline the font size const tag = document.createElement("li"); tag.classList.add("tag"); tag.innerHTML = ` <a href="https://www.php.cn/link/72085ca61c54cb3316dcf4b61e0b198d" class="tag__link" style="font-size: ${fontSizeProperty}">${name} (${numberOfArticles})</a> `; // Attach each tag to the fragment fragment.appendChild(tag); }
The code is easier to read by setting some variables before we start creating HTML. It also makes our code more DRY, as we can use the numberOfArticles variable in multiple places.
Once this .forEach loop returns each tag, they are collected into the fragment. After that, we use appendChild() to add them to the tags element. This means that the DOM is operated only once, not every time the loop runs, which is a good performance boost if we happen to have a lot of tags.
Font zoom
What we have now works great for us and we can start writing CSS. However, our formula for fontSize variable means that the most tags (i.e. "flex" with 25) will be 6em (25 / 25 6 = 6), but only 1 post will have a tag size of 1/25 (1 / 25 6 = 0.24), making the content difficult to read. If we had a tag with 100 posts, smaller tags would be worse (1 / 100 * 6 = 0.06).
To solve this I added a simple if statement that sets fontSize to 1 if the returned fontSize is less than 1. If not, keep it at the current size. Now all tags have font sizes between 1em and 6em, rounded to two decimal places. To increase the size of the maximum tag, just change the value of maxFontSizeForTag. You can decide which method is best for you based on the amount of content you are dealing with.
function handleResult(result, highestValue) { // Set the variable const numberOfArticles = result.tagged_articles.length; const name = result.title; const link = result.href; let fontSize = numberOfArticles/highestValue * maxFontSizeForTag; fontSize = fontSize.toFixed(2); // Make sure the font size is at least 1em if (fontSize ${name} (${numberOfArticles}) `; // Attach each tag to the fragment fragment.appendChild(tag); }
It's CSS now!
We use flexbox for layout because each label may have different width. Then we center them with justify-content: center and remove the list bullets.
.tags { display: flex; flex-wrap: wrap; justify-content: center; max-width: 960px; margin: auto; padding: 2rem 0 1rem; list-style: none; border: 2px solid white; border-radius: 5px; }
We will also use flexbox for each tag. This allows us to align them vertically using the align-items: center, because their height varies according to the font size.
.tag { display: flex; align-items: center; margin: 0.25rem 1rem; }
Each link in the Tag Cloud has some padding, just to make it slightly beyond its strict size to click.
.tag__link { padding: 5px 5px 0; transition: 0.3s; text-decoration: none; }
I find this especially convenient on small screens, especially for those who may have difficulty clicking on links. The initial text-decoration was removed because I think we can assume that each text item in the tag cloud is a link, so there is no need to have special decoratives for them.
I'll add some colors to make the styles a little more beautiful:
.tag:nth-of-type(4n 1) .tag__link { color: https://www.php.cn/link/93ac0c50dd620dc7b88e5fe05c70e15bffd560; } .tag:nth-of-type(4n 2) .tag__link { color: https://www.php.cn/link/93ac0c50dd620dc7b88e5fe05c70e15bee4266; } .tag:nth-of-type(4n 3) .tag__link { color: https://www.php.cn/link/93ac0c50dd620dc7b88e5fe05c70e15b9e88f7; } .tag:nth-of-type(4n 4) .tag__link { color: https://www.php.cn/link/93ac0c50dd620dc7b88e5fe05c70e15b54d0ff; }
This color scheme is directly stolen from Chris' blog link, where each fourth tag from the beginning of tag one is yellow, each fourth tag from the beginning of tag two is red, each fourth tag from the beginning of tag three is purple, and each fourth tag from the beginning of tag four is blue.
Then we set the focus and hover states for each link:
.tag:nth-of-type(4n 1) .tag__link:focus, .tag:nth-of-type(4n 1) .tag__link:hover { box-shadow: inset 0 -1.3em 0 0 https://www.php.cn/link/93ac0c50dd620dc7b88e5fe05c70e15bffd560; } .tag:nth-of-type(4n 2) .tag__link:focus, .tag:nth-of-type(4n 2) .tag__link:hover { box-shadow: inset 0 -1.3em 0 0 https://www.php.cn/link/93ac0c50dd620dc7b88e5fe05c70e15bee4266; } .tag:nth-of-type(4n 3) .tag__link:focus, .tag:nth-of-type(4n 3) .tag__link:hover { box-shadow: inset 0 -1.3em 0 0 https://www.php.cn/link/93ac0c50dd620dc7b88e5fe05c70e15b9e88f7; } .tag:nth-of-type(4n 4) .tag__link:focus, .tag:nth-of-type(4n 4) .tag__link:hover { box-shadow: inset 0 -1.3em 0 0 https://www.php.cn/link/93ac0c50dd620dc7b88e5fe05c70e15b54d0ff; }
At this stage, I may have created a custom variable for the color - for example --yellow: https://www.php.cn/link/93ac0c50dd620dc7b88e5fe05c70e15bffd560 etc - but decided to use a lengthy approach to support IE 11. I like the box-shadow hover effect. This is a small piece of code that achieves a more visually appealing effect than a standard underscore or bottom border. Using em units here means we can control the size of the shadow relative to the text that needs to be covered.
OK, let's end up by setting each tag link to black:
.tag:nth-of-type(4n 1) .tag__link:focus, .tag:nth-of-type(4n 1) .tag__link:hover, .tag:nth-of-type(4n 2) .tag__link:focus, .tag:nth-of-type(4n 2) .tag__link:hover, .tag:nth-of-type(4n 3) .tag__link:focus, .tag:nth-of-type(4n 3) .tag__link:hover, .tag:nth-of-type(4n 4) .tag__link:focus, .tag:nth-of-type(4n 4) .tag__link:hover { color: black; }
We're done! Here is the final result:
(Since I don't have the actual running code, I can't provide the final tag cloud screenshot. Please create and view the results by yourself based on the provided code and CSS.)
The above is the detailed content of Create a Tag Cloud with some Simple CSS and even Simpler JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


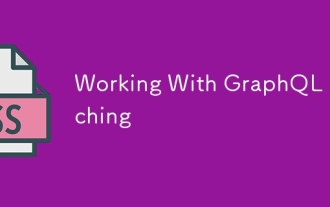
If you’ve recently started working with GraphQL, or reviewed its pros and cons, you’ve no doubt heard things like “GraphQL doesn’t support caching” or
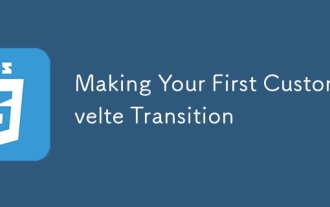
The Svelte transition API provides a way to animate components when they enter or leave the document, including custom Svelte transitions.
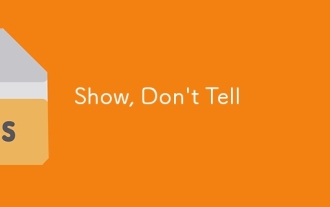
How much time do you spend designing the content presentation for your websites? When you write a new blog post or create a new page, are you thinking about
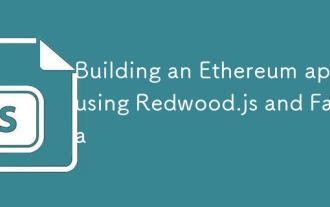
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
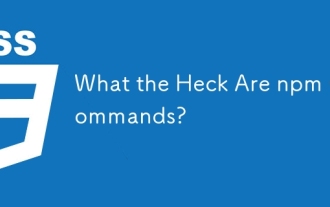
npm commands run various tasks for you, either as a one-off or a continuously running process for things like starting a server or compiling code.
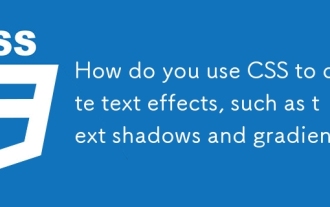
The article discusses using CSS for text effects like shadows and gradients, optimizing them for performance, and enhancing user experience. It also lists resources for beginners.(159 characters)
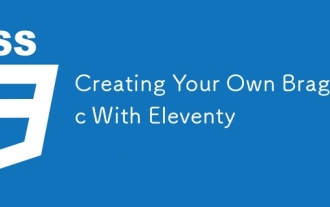
No matter what stage you’re at as a developer, the tasks we complete—whether big or small—make a huge impact in our personal and professional growth.
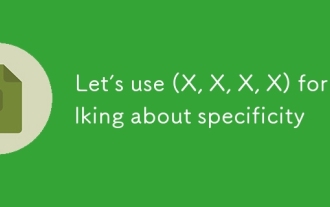
I was just chatting with Eric Meyer the other day and I remembered an Eric Meyer story from my formative years. I wrote a blog post about CSS specificity, and
