CSS Media Queries Guide
CSS media query is a technique that locates a browser based on specific features, functions, and user preferences, and then applies styles or runs other code based on these features. Perhaps the most common media queries in the world are those that target specific viewport ranges and apply custom styles, which gave birth to the entire concept of responsive design.
/* When the browser width is at least 600px or above*/ @media screen and (min-width: 600px) { .element { /* Apply some styles*/ } }
In addition to viewport width, we can position many other aspects. This could be screen resolution, device orientation, operating system preferences, and even a set of other factors we can query and use to style content.
Want to quickly view a list of media queries based on the viewport of standard devices such as mobile phones, tablets, and laptops? Please check out our set of code snippets.
Using media query
Media queries are usually associated with CSS, but can also be used in HTML and JavaScript.
HTML
There are several ways we can use media queries directly in HTML.
<link>
Elements can be placed directly in the document. In this example, we tell the browser that we want to use different stylesheets for different viewport sizes:
<link href="all.css" media="all" rel="stylesheet"> <link href="small.css" media="(min-width: 20em)" rel="stylesheet"> <link href="medium.css" media="(min-width: 64em)" rel="stylesheet"> <link href="large.css" media="(min-width: 90em)" rel="stylesheet"> <link href="extra-large.css" media="(min-width: 120em)" rel="stylesheet"> <link href="print.css" media="print" rel="stylesheet">
Why do this? This is a great way to fine-tune the performance of a website by splitting the styles apart in a way that fits the device that needs them download and service.
But it is clear that this does not always prevent stylesheet downloads that do not match these media queries, it simply assigns them a lower loading priority.
So if a small screen device like a phone visits the website, it will only download the stylesheets in the media query that matches its viewport size. However, if a larger desktop screen appears, it will download all the stylesheets because it matches all these queries (in this particular example, subtract the print query).
This is just<link>
element. As our responsive image guide explains, we can use media queries on elements, which tells the element browser which version of the image should be used from a set of image options.
<img src="/static/imghw/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/174353857438221.png" class="lazy" alt="CSS Media Queries Guide">
Again, this can improve performance well, as we can provide smaller images for smaller devices—this is probably (but not always) a low-power device that may be limited by data planning.
And let's not forget, we can also be directly in<img src="/static/imghw/default1.png" data-src="cat.png" class="lazy" alt="CSS Media Queries Guide" >
Use media queries on elements:
<img src="/static/imghw/default1.png" data-src="cat.png" class="lazy" alt="A calico cat with dark aviator sunglasses." srcset="cat-small.png 300w, cat-medium.png 600w, cat-large.png 1200w" sizes="(max-width: 600px) 300px, (max-width: 1200px) 600px, 1200px">
CSS
Similarly, CSS is the most common place to find media queries in practical applications. They are directly located in the @media
rule in the stylesheet, which wraps elements using conditions when the browser matches these conditions to determine when and where a set of styles is applied.
/* Viewport with width between 320px and 480px*/ @media only screen and (min-device-width: 320px) and (max-device-width: 480px) { .card { background: #bada55; } }
You can also scope the imported stylesheet, but as a general rule, you should avoid using @import
because it performs poorly.
/* Avoid using @import as much as possible! */ /* Basic styles of all screens*/ @import url("style.css") screen; /* Style of the portrait (narrow) direction screen*/ @import url('landscape.css') screen and (orientation: portrait); /* Print style*/ @import url("print.css") print;
JavaScript
We can also use media queries in JavaScript! Guess what? They work very similarly to those in CSS. The difference is? We first use window.matchMedia()
method to define the condition.
So, suppose we want to log messages to the console when the browser width is at least 768px. We can create a constant that calls matchMedia()
and defines the screen width:
// Create a media condition for viewports with a width of at least 768px const mediaQuery = window.matchMedia('(min-width: 768px)');
Then, when this condition is met, we can trigger the record to the console:
// Create a media condition for viewports with a width of at least 768px const mediaQuery = window.matchMedia('(min-width: 768px)'); // Note the `matches` property if (mediaQuery.matches) { console.log('Media Query Match!'); }
Unfortunately this will only fire once, so if the alarm is turned off, it won't fire again if we change the screen width and try again without refreshing. This is why it is best to use a listener to check for updates.
// Create a condition that is for viewports with a width of at least 768px const mediaQuery = window.matchMedia('(min-width: 768px)'); function handleTabletChange(e) { // Check if the media query is true if (e.matches) { // Then log the following message to console.log('Media Query Match!'); } } // Register the event listener mediaQuery.addListener(handleTabletChange); // Initial check handleTabletChange(mediaQuery);
Check out Marko Ilic's full article on "Using JavaScript Media Query" for a more in-depth look at this, including using media queries to comparisons of older JavaScript methods (binding resize
event listener, checking window.innerWidth
or window.innerHeight
to trigger changes).
### The structure of media query
Now that we've seen several examples of where media queries can be used, let's break them down and see what they are actually doing.
@media
@media [media-type] ([media-feature]) { /* Style! */ }
The first ingredient in the media query recipe is the @media
rule itself, which is one of many CSS at-rules. Why does @media
get so much attention? Because it targets the type of media used for the website viewing, the features supported by that media type, and operators that can be combined to mix and match simple and complex conditions.
Media Type
@media screen { /* Style! */ }
What type of media are we trying to locate? In many, if not most, cases you will see a screen
value here, which makes sense, because many of the media types we are trying to match are devices with screen connections.
But the screen is certainly not the only media type we can locate. We have some, including:
-
all
: match all devices -
print
: Matches the document viewed in the print preview or any media that breaks the content into pages designed to be printed. -
screen
: Match devices with screens -
speech
: Matches devices that read content in a sound manner, such as screen readers. Since self-media query level 4, this has replaced the now deprecatedaural
type.
To preview print styles on the screen, all major browsers can use DevTools to simulate the output of print style sheets. Other media types (such as tty
, tv
, projection
, handheld
, braille
, embossed
, and aural
) have been deprecated, and while the specification continues to recommend that browsers recognize them, they must be evaluated as empty. If you are using one of these, consider changing it to a modern approach.
Media Features
Once we define the media type to match, we can start defining the features to match it. We've looked at many examples of matching screens to widths, where screen
is type , and min-width
and max-width
are properties with specific values.
But there are many, many (many) "features" that we can match! Media Query Level 5 Classify media features into 6 categories.
Viewport/page features
Display quality
color
Interaction
Video prefix
The specification references user agents, including television, that present video and graphics on two planes each with their own characteristics. The following features describe these planes.
script
User preferences
Deprecated
Operators
Media queries support logical operators in many programming languages so that we can match media types based on certain conditions. The @media
rule itself is a logical operator that basically declares that "if" the following types and attributes match, then perform some operations.
and
However, if we want to locate screens within a certain width range, we can use and
operator:
/* Match screens with widths between 320px and 768px*/ @media screen (min-width: 320px) and (max-width: 768px) { .element { /* Style! */ } }
or (or comma separated)
We can also use comma-separated features as the or
operator to match different features:
/* Match the user's preference for dark mode or screen width of at least 1200px*/ @media screen (prefers-color-scheme: dark), (min-width: 1200px) { .element { /* Style! */ } }
Not
Maybe we want to locate the device by what the device does not support or does not match . This statement removes the background color of the body when the device is a printer and can only display one color.
@media print and (not(color)) { body { background-color: none; } }
Want to have a deeper understanding? Check out the DigitalOcean community's "CSS Media Query: Quick References and Guides" for more examples that follow the media query syntax.
Do you really need CSS media queries?
Media Query is a powerful tool in the CSS toolbox with exciting hidden gems. But if you adapt to the design of each situation, you end up with a code base that is overly complex to maintain, and, as we all know, CSS is like a bear cub: cute and harmless, but it eats you when it grows up.
That's why I recommend following Ranald Mace's universal design philosophy, which is to " design products to be used by everyone as much as possible without adapting or designing specifically. "
In "Accessible to Everyone," Laura Kalbag explains that the difference between accessibility and universal design is subtle but important. Accessible designers create a large-sized door for wheelchair users to access, while general designers create an entrance that anyone can adapt, regardless of their abilities.
I know it's hard to talk about universal design on the web and it sounds almost utopian, but think about it, there are about 150 different browsers, about 50 different combinations of user preferences, and as we mentioned earlier, there are over 24,000 different and unique devices for Android devices alone.
This means at least 18 million possible scenarios that may display your content. In the words of the wonderful Miriam Suzanne, “ CSS is here trying to graphic design of unknown content across operating systems, interfaces, and languages on an infinite and unknown canvas. None of us may know what we are doing. ”
This is why assumptions are very dangerous, so when you design, develop and consider your product, leave assumptions aside and use media queries to make sure your content is displayed correctly in front of any contact and any user.
Match range of values
Many media features outlined in the previous section, including width
, height
, color
and color-index
, can be prefixed with min-
or max-
to represent minimum or maximum constraints. We've seen these usages in many examples, but the key is that we can create a range of values to match without having to declare a specific value.
In the following code snippet, when the viewport width is greater than 30em and less than 80em, we set the background color of the body to purple. If the viewport width does not match the value range, it falls back to white.
body { background-color: #fff; } @media (min-width: 30em) and (max-width: 80em) { body { background-color: purple; } }
Media Query Level 4 specifies a new simplified syntax using less than( and <code>equals(=)
operators. Therefore, the last example can be converted to a new syntax, like so:
@media (width >= 30em) and (width <p></p><h3 id="Nested-and-complex-decision-making"> Nested and complex decision making</h3><p></p><p> CSS allows you to nest at-rules or group statements using parentheses, allowing us to deeply evaluate complex operations.</p><pre class="brush:php;toolbar:false"> @media (min-width: 20em), not all and (min-height: 40em) { @media not all and (pointer: none) { ... } @media screen and ( (min-width: 50em) and (orientation: landscape) ), print and (not (color)) { ... } }
careful! Even if you can create powerful and complex expressions, you may end up with a very arbitrary, hard to maintain query. As Brad Frost puts it, “The more complex our interfaces are, the more we have to think to properly maintain them.”
Auxiliary functions
Many of the features added in Media Query Level 4 focus on accessibility.
prefers-reduced-motion
prefers-reduced-motion
detects whether the user has enabled Reduced Motion Preference to minimize the number of movement and animations. It takes two values:
<code>- no-preference:指示用户没有向系统告知任何偏好。 - reduce:指示用户已通知系统他们更喜欢最大限度地减少移动或动画数量的界面,最好是消除所有非必要的移动。此偏好通常由患有前庭障碍或眩晕症的人使用,其中不同的运动会导致失去平衡、偏头痛、恶心或听力损失。如果您曾经尝试快速旋转并感到头晕,您就会知道感觉如何。在Eric Bailey 的一篇精彩文章中,他建议使用以下代码停止所有动画: ```css @media screen and (prefers-reduced-motion: reduce) { * { /* 非常短的持续时间意味着依赖事件的JavaScript 仍然有效*/ animation-duration: 0.001ms !important; animation-iteration-count: 1 !important; transition-duration: 0.001ms !important; } }</code>
Popular frameworks such as Bootstrap have this feature by default. In my opinion, there is no reason not to use prefers-reduced-motion
- just use it.
prefers-contrast
The prefers-contrast
feature tells the user whether the preferences and browser settings have been selected to increase or decrease contrast. It takes three values:
- no-preference: When the user does not inform the system of any preferences. If you use it as a boolean, it will evaluate to
false
. - high: When the user selects to display a higher contrast level.
- low: When the user selects to display a lower contrast level.
At the time of writing, this feature is not supported by any browser. Microsoft uses non-standard early implementations to work with -ms-high-contrast
feature, which is only available for Microsoft Edge v18 or earlier (but does not include Chromium-based versions).
.button { background-color: #0958d8; color: #ffff; } @media (prefers-contrast: high) { .button { background-color: #0a0db7; } }
This example raises the contrast of the button
class from AA to AA when the user turns on high contrast.
inverted-colors
The inverted-colors
feature tells the user whether the color has been selected to be reversed in their system preferences or browser settings. Sometimes this option is used as an alternative to high contrast. It takes two values:
- none: When the color is displayed normally
- inverted: When the user selects the invert color option
The problem with inverting the color is that it also inverts the colors of the image and video, making them look like X-ray images. By using the CSS invert
filter, you can select all images and videos and invert them back.
@media (inverted-colors) { img, video { filter: invert(100%); } }
At the time of writing, this feature is supported only by Safari.
prefers-color-scheme
Today, we are increasingly seeing “dark mode” color schemes, and thanks to the prefers-color-scheme
feature, we can leverage the user’s system or browser preferences to determine whether we should provide “dark” or “light” themes based on their preferences.
It takes two values:
- light: When users choose they prefer light themes or have no activity preferences
- dark: When the user selects a dark display in its settings
body { --bg-color: white; --text-color: black; background-color: var(--bg-color); color: var(--text-color); } @media screen and (prefers-color-scheme: dark) { body { --bg-color: black; --text-color: white; } }
As Adhuham explains in his complete guide on dark mode, it's more than just changing the background color. Before you start using dark mode, remember that if you don't have a very smart implementation strategy, you may end up with a very difficult code base that is difficult to maintain. CSS variables can do wonders for this, but this will be the subject of another post.
Future Outlook
Media Query Level 5 is currently in working draft state, which means a lot of changes can occur between now and it becomes a suggestion. But it contains some interesting features worth mentioning as they open up new ways to position the screen and adapt the design to very specific conditions.
User preference media features
Hey, we just introduced these in the last section! All right. These features are exciting because they are informed by the user's actual settings, whether they come from the user agent or operating system level.
Detect mandatory color palette
This is very simple. Some browsers limit the number of available colors available to render styles. This is called "forced color mode", and if enabled in browser settings, users can choose to use a limited set of color on the page. Therefore, users can define color combinations and contrast, making the content easier to read.
The forced-colors
feature allows us to use active
values to detect whether a mandatory color palette is being used. If it matches, the browser must provide the required palette through the CSS system colors. The browser can also decide whether the background color of the page is light or dark, and if appropriate, trigger the corresponding prefers-color-scheme
value so that we can adjust the page.
Detect maximum brightness, color depth and contrast ratio
Some devices (and browsers) are able to display super bright displays, present a wide range of color and high contrast ratios between colors. We can detect these devices using the dynamic-range
feature, where high
keyword matches these devices and standard
matches all other devices.
We may see changes to this, as as of now, there is still uncertainty about the measurements that make up high brightness and contrast. The browser may make this decision.
Video prefix characteristics
The specification discusses some screens (such as TVs) that are able to display video and graphics on separate "planes", which may be a way to differentiate video frames from other elements on the screen. Therefore, Media Query Level 5 proposes a new set of media features designed to detect video features, including color gamut and dynamic range.
There are also suggestions on detecting video height, width, and resolution, but the jury is still discussing whether these are the correct way to solve the video.
Browser support
Browsers are constantly evolving because browser support for this feature may change as you read this article, please check the MDN updated browser compatibility table.
Instructions for container query
Wouldn't that be cool if components can be adjusted according to their own size rather than the browser's own size? This is the concept of CSS container query. We currently only have the browser screen to make these changes through media queries.
This is unfortunate because the viewport is not always directly related to the size of the element itself. Imagine a widget rendered in many different contexts on a website: sometimes in the sidebar, sometimes in the full width footer, sometimes in the grid with unknown columns.
This is the problem that container query is trying to solve. Ideally, we can adjust the style of the element according to the size of the element itself rather than the size of the viewport. Chrome 105 releases support for CSS container queries. The same goes for Safari 16.1. At the time of writing, what we really wait for is Firefox’s extensive support.
This browser supports data from Caniuse, which contains more details. The number indicates that the browser supports this feature in this version and later.
desktop
Mobile/Tablet PC
### Example
Let's look at some media query examples. With so many combinations of media types, features, and operators, the number of possibilities we can display will be exhaustive. Instead, we will highlight some based on specific media features.
Adjust layout at different viewport widths
More information
This is probably the most widely used media feature. It tells the browser the width of the viewport, including the scrollbar. It unlocks the CSS implementation of Ethan Marcotte's famous responsive design : a design process that responds to viewport size by combining fluid mesh, flexible images and responsive typography.
Later, Luke Wroblewski developed the concept of responsive design by introducing the term mobile priority , encouraging designers and developers to start with a small screen experience first and then gradually enhance the experience as screen width and device functionality expand.
Mobile priority can usually be found by using min-width
instead of max-width
. If we start with min-width
, we're basically saying, "Hey, browser, work up from here." On the other hand, max-width
is like prioritizing larger screens.
One way to define breakpoints by width is to use the size of a standard device, such as the exact pixel width of an iPhone. But there are many, many (many) different phones, tablets, laptops and desktops. Looking at Android alone, as of August 2015, there were more than 24,000 variants of viewport sizes, resolutions, operating systems and browsers.
So while the exact width for a particular device may be helpful for troubleshooting or one-time fixes, this may not be the most reliable solution for maintaining a responsive architecture. This is by no means a new idea. Brad Frost has already promoted the advantages of letting content (not devices) determine breakpoints in his 2013 post, “7 Habits of Efficient Media Query.”
Even though media queries are still an effective tool for creating responsive interfaces, in many cases, width can be completely avoided. Modern CSS allows us to create flexible layouts using CSS grids and flex that adapt our content to viewport size without adding breakpoints. For example, this is a grid layout that adjusts how many columns it will have without any media queries.
.container { display: grid; grid-template-columns: repeat(auto-fill, minmax(200px, 1fr)); }
There are a lot of articles about thinking beyond widths, I wrote this a few years ago and I suggest checking out an article by Una Kravet called "Ten Modern Layouts in One Line of CSS."
#### Dark Mode
More information
This example is taken directly from our guide on dark mode on the network. The idea is that we can use prefers-color-scheme
feature to detect whether the user's system settings are configured in light or dark mode, and then define a set of alternative colors for the rendered UI.
Combining this technique with CSS custom properties makes things easier because they are like variables that we only need to define once and then use throughout our code. Need to exchange colors? Change the custom property value and it will be updated anywhere. This is exactly prefers-color-scheme
does. We define a set of colors as custom properties and then redefine them in media queries using the prefer-color-scheme
attribute to change colors based on user settings.
#### Detect direction, hover, and motion on responsive card library
More information
This gallery is responsive and does not require the use of width features.
It detects the orientation of the viewport. If it is a portrait viewport, the sidebar will become the title; if it is landscape, it will remain on the side.
Using the pointer
media feature, it determines whether the main input device is rough (such as finger) or fine (such as mouse cursor) to set the size of the clickable area of the checkbox.
Then, by using the hover
media feature, the example checks if the device is able to hover (such as the mouse cursor) and displays check boxes in each card.
When prefers-reduced-motion
is set to reduce
, the animation will be removed.
Have you noticed something? We don't actually use media queries for the actual layout and size of the card! This is handled using the minmax()
function on the .container
element to show that responsive design does not always mean using media queries.
In short, this is a fully responsive application without measuring width or making any assumptions.
#### Positioning iPhone in landscape mode
/* iPhone X landscape mode*/ @media only screen and (min-device-width: 375px) and (max-device-width: 812px) and (-webkit-min-device-pixel-ratio: 3) and (orientation: landscape) { /* Style! */ }
More information
orientation
media characteristic tests whether the device rotates in the wide direction (lateral direction) or in the high direction (vertical direction).
While media queries don't know exactly which device is being used, we can use the exact size of a particular device. The above code snippet is aimed at iPhone X.
#### Apply sticky titles for large viewports
More information
In the example above, we use height
to separate fixed elements and avoid taking up too much screen space when the screen is too short. When the screen is high, the horizontal navigation bar is in a fixed position, but separates itself on a shorter screen.
Like the width
feature, height
detects the height of the viewport, including the scrollbar. Many of us browse web pages on small devices with narrow viewports, which makes designing for different heights more important than ever. Anthony Colangelo describes how Apple uses the height
media feature in a meaningful way to handle the size of a hero image when viewport height changes.
#### Responsive (Fluid) Typesetting
More information
The font may look too big or too small, depending on the screen size of the screen displayed. If we work on small screens, we will most likely want to use fonts that are smaller than those used on larger screens.
The idea here is that we use the width of the browser to scale the font size. We're in Set a default font size on it as the "small" font size, and then set another font size using the media query as the "large" font size. In the middle? We set the font size again, but in another media query that calculates the size based on the browser width.
The beauty of this is that it allows the font size to be adjusted according to the browser width, but never exceeds or falls below a specific size. However, thanks to newer CSS features like min()
, max()
, and clamp()
, there is an easier way to do this without any media queries.
#### Provides larger touch targets when the device has thick pointers
More information
Have you ever visited a website with super small buttons? Some of us have thick fingers and it's hard to accurately click on an object without accidentally clicking on something else.
Of course, we can rely on the width
feature to tell if we are working on small screens, but we can also detect if the device can hover over the element. If not, then it could be a touch device, or it could be a device that supports both devices, such as Microsoft Surface.
The above demonstration uses check boxes as an example. When viewing checkboxes on small screens, the checkbox can be hard to click, so if the device fails to perform hover events, we will increase the size and do not need to hover.
Again, this approach is not always accurate. Please see Patrick Lauke's detailed article on issues that may arise when using hover
, pointer
, any-hover
, and any-pointer
.
### Specification
- Media Query Level 4 (Cannotated Recommendation)
- Media query level 5 (working draft)
Special thanks to Sarah Rambacher for helping review this guide.
The above is the detailed content of CSS Media Queries Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


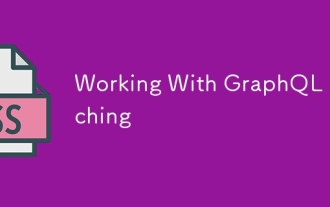
If you’ve recently started working with GraphQL, or reviewed its pros and cons, you’ve no doubt heard things like “GraphQL doesn’t support caching” or
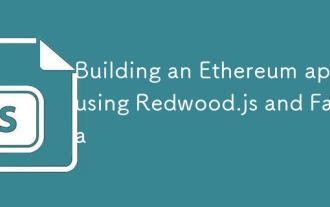
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
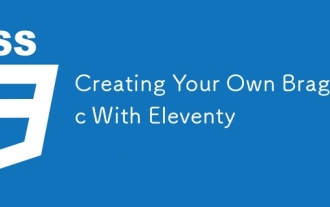
No matter what stage you’re at as a developer, the tasks we complete—whether big or small—make a huge impact in our personal and professional growth.
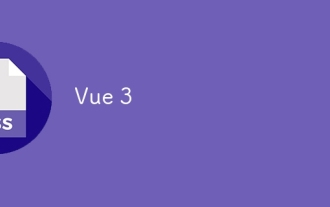
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
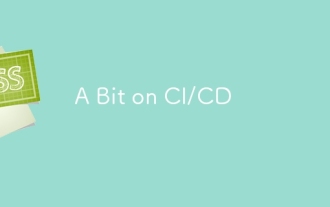
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
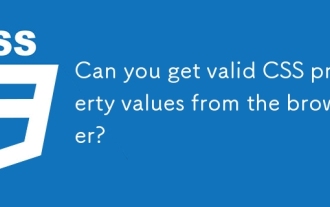
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
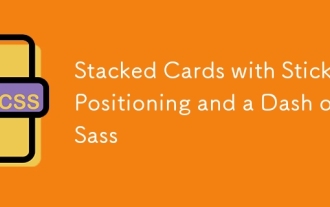
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
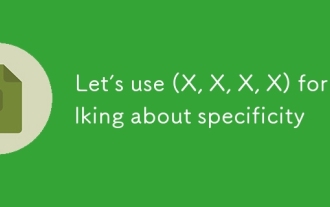
I was just chatting with Eric Meyer the other day and I remembered an Eric Meyer story from my formative years. I wrote a blog post about CSS specificity, and
