Advanced C Tutorial: Crack Your Next Coding Interview
C Interview preparation requires mastering advanced features such as smart pointers, templates, and mobile semantics. 1) Smart pointers such as std::unique_ptr and std::shared_ptr are used for memory management to avoid leakage. 2) The template supports generic programming to improve code reusability. 3) Moving semantics and rvalue references improve performance, and you need to pay attention to the use of noexcept.
introduction
In the programming world, C is a powerful and complex language, especially in interviews, which often serves as a touchstone for testing programmers’ abilities. This article is designed to help you master the advanced features of C and stand out in your next coding interview. By reading this article, you will gain insight into the complexity of C, master key programming skills, and learn how to deal with common interview questions.
Review of basic knowledge
C is an object-oriented programming language that combines the ease of use of high-level languages and the performance of underlying languages. It supports a variety of programming paradigms, including object-oriented programming, generic programming, and functional programming. During an interview, you may need to demonstrate an understanding of these concepts and how to apply them in real-life programming.
C's standard library provides a wealth of containers and algorithms, which are also frequently examined during interviews. Understanding the use of containers such as vector, list, map, and the application of functions such as sort and find in the algorithm library is the key to preparing for an interview.
Core concept or function analysis
Smart pointer and memory management
C's memory management has always been the focus of interviews. Smart pointers such as std::unique_ptr
and std::shared_ptr
are important tools in modern C, which help developers avoid memory leaks and dangling pointers.
#include <memory> #include <iostream> class MyClass { public: void doSomething() { std::cout << "Doing something...\n"; } }; int main() { // Use std::unique_ptr std::unique_ptr<MyClass> uniquePtr(new MyClass()); uniquePtr->doSomething(); // Use std::shared_ptr std::shared_ptr<MyClass> sharedPtr(new MyClass()); sharedPtr->doSomething(); return 0; }
Smart pointers work by managing the life cycle of an object through reference counting or exclusive ownership. std::unique_ptr
ensures that the object is deleted when it is no longer needed, while std::shared_ptr
allows multiple pointers to share the same object until the last reference is released.
Template and generic programming
C's template system is one of its powerful features, allowing for the writing of common code to process different types of data. During an interview, you may be asked to write a template function or class.
template<typename T> T max(T a, T b) { return (a > b) ? a : b; } int main() { std::cout << max(5, 10) << std::endl; // Output 10 std::cout << max(3.14, 2.71) << std::endl; // Output 3.14 return 0; }
The implementation principle of templates involves compile-time code generation, which makes template code almost no extra overhead at runtime. However, abuse of templates can lead to too long compilation times and bloat code, so trade-offs are needed when using them.
Move semantics and rvalue references
C 11 introduces mobile semantics and rvalue references, greatly improving the performance of the program. Understanding and applying these concepts is very important in interviews.
#include <iostream> #include <vector> class MyClass { public: MyClass() { std::cout << "Constructor\n"; } MyClass(MyClass&& other) noexcept { std::cout << "Move Constructor\n"; } MyClass& operator=(MyClass&& other) noexcept { std::cout << "Move assignment operator\n"; return *this; } }; int main() { std::vector<MyClass> vec; vec.push_back(MyClass()); // Use the move constructor MyClass obj = std::move(MyClass()); // Use the move assignment operator to return 0; }
Move semantics improve efficiency by avoiding unnecessary copy operations. Rvalue references ( &&
) allow functions to accept temporary objects, thus implementing mobile constructors and mobile assignment operators. However, writing correct movement semantics requires attention to the use of noexcept
keywords to ensure exception security.
Example of usage
Basic usage
During the interview, you may need to show how to use C's standard library to solve the problem. For example, use std::vector
and std::algorithm
to implement a simple sorting algorithm.
#include <vector> #include <algorithm> #include <iostream> int main() { std::vector<int> numbers = {3, 1, 4, 1, 5, 9, 2, 6, 5, 3}; std::sort(numbers.begin(), numbers.end()); for (int num : numbers) { std::cout << num << " "; } std::cout << std::endl; return 0; }
This code shows how to sort an array of integers using std::vector
and std::sort
. Understanding the use of these standard library functions is a common requirement in interviews.
Advanced Usage
In more advanced interviews, you may need to show how to use the advanced features of C to solve complex problems. For example, use lambda expressions and std::function
to implement a general callback mechanism.
#include <functional> #include <iostream> void execute(std::function<void()> callback) { callback(); } int main() { auto lambda = []() { std::cout << "Lambda executed\n"; }; execute(lambda); return 0; }
This example shows how to implement a general callback mechanism using lambda expressions and std::function
. This technique is very common in modern C and can demonstrate your advanced understanding of the language.
Common Errors and Debugging Tips
Understanding common mistakes and debugging skills is also very important in interviews. For example, avoiding frequent allocation and freeing of memory in loops is a common optimization point.
#include <vector> void inefficientFunction() { std::vector<int> vec; for (int i = 0; i < 10000; i) { vec.push_back(i); // Each push_back may cause memory reallocation} } void efficientFunction() { std::vector<int> vec; vec.reserve(10000); // Preallocate memory to avoid frequent re-allocation for (int i = 0; i < 10000; i) { vec.push_back(i); } }
In inefficientFunction
, each push_back
may cause vector to reallocate memory, degrading performance. This is avoided efficientFunction
preallocating memory through reserve
. Understanding these optimization points and showing them in the interview can greatly improve your performance.
Performance optimization and best practices
In practical applications, optimizing the performance of C code is a key skill. Comparing the performance differences between different methods and showing optimization effects is a common requirement in interviews. For example, compare the performance of std::vector
and std::list
.
#include <vector> #include <list> #include <chrono> #include <iostream> void benchmarkVector() { std::vector<int> vec; auto start = std::chrono::high_resolution_clock::now(); for (int i = 0; i < 1000000; i) { vec.push_back(i); } auto end = std::chrono::high_resolution_clock::now(); auto duration = std::chrono::duration_cast<std::chrono::microseconds>(end - start); std::cout << "Vector push_back time: " << duration.count() << " microseconds\n"; } void benchmarkList() { std::list<int> lst; auto start = std::chrono::high_resolution_clock::now(); for (int i = 0; i < 1000000; i) { lst.push_back(i); } auto end = std::chrono::high_resolution_clock::now(); auto duration = std::chrono::duration_cast<std::chrono::microseconds>(end - start); std::cout << "List push_back time: " << duration.count() << " microseconds\n"; } int main() { benchmarkVector(); benchmarkList(); return 0; }
This code shows how to compare the performance differences between std::vector
and std::list
in push_back
operation. Understanding these performance differences and showing optimizations in the interview can help you better deal with performance-related questions in the interview.
It is very important to keep the code readable and maintained in terms of programming habits and best practices. For example, using meaningful variable names, adding comments, and following a consistent code style are all great ways to show your professionalism in an interview.
In short, mastering the advanced features and best practices of C will not only help you perform well in interviews, but also improve your efficiency and code quality in actual programming. I hope this article can provide you with valuable guidance and wish you success in your next coding interview!
The above is the detailed content of Advanced C Tutorial: Crack Your Next Coding Interview. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
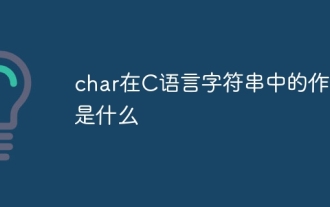
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
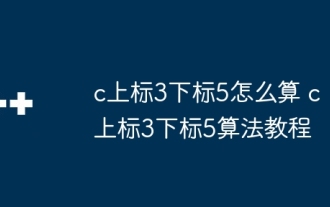
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
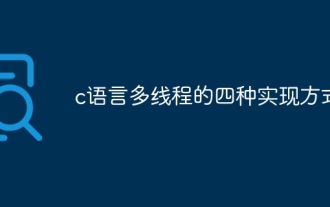
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
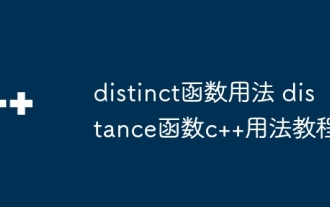
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
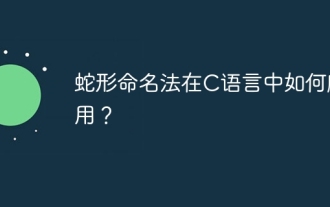
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
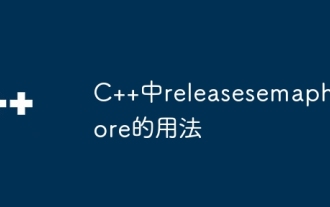
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
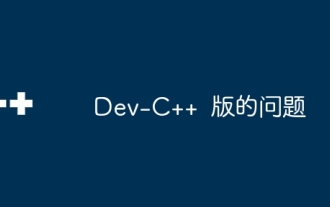
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
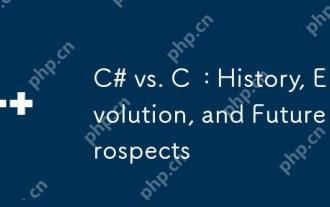
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
