


XML/RSS Data Integration: Practical Guide for Developers & Architects
XML/RSS data integration can be achieved by parsing and generating XML/RSS files. 1) Use Python's xml.etree.ElementTree or feedparser library to parse XML/RSS files and extract data. 2) Use ElementTree to generate XML/RSS files and gradually add nodes and data.
introduction
In this data-driven world, XML and RSS remain an important part of many applications, especially in content aggregation and data exchange. As a developer or architect, understanding how to effectively integrate XML/RSS data will not only improve work efficiency, but also bring more flexibility and scalability to your project. This article will take you into a hands-on guide to XML/RSS data integration to help you master this key skill.
By reading this article, you will learn how to parse and generate XML/RSS data, understand their application scenarios in modern applications, and master some practical best practices and performance optimization techniques. I will combine my experience to share some problems and solutions encountered in actual projects to help you avoid common pitfalls.
Review of basic knowledge
XML (Extensible Markup Language) and RSS (Really Simple Syndication) are two common data formats. XML is used for the storage and transmission of structured data, while RSS is a standard format for content distribution and aggregation. Understanding the basics of these two formats is the first step in integrating them.
XML files are composed of tags that can be nested to form a tree-like structure. RSS is a specific format based on XML, used to publish frequently updated content, such as blog posts, news, etc. RSS files usually contain fields such as title, link, description, etc., which facilitates content aggregation for other applications.
When processing XML/RSS data, we usually use some libraries or tools, such as Python's xml.etree.ElementTree
or feedparser
. These tools can help us parse XML/RSS files and extract the data in it.
Core concept or function analysis
XML/RSS parsing and generation
Parsing XML/RSS data is one of the core tasks that integrate them. Let's look at a simple example, using Python's xml.etree.ElementTree
to parse an RSS file:
import xml.etree.ElementTree as ET # Read RSS file tree = ET.parse('example.rss') root = tree.getroot() # traverse RSS items for item in root.findall('./channel/item'): title = item.find('title').text link = item.find('link').text print(f'Title: {title}, Link: {link}')
This code shows how to read an RSS file and iterate through the items in it, extracting title and link information. Similarly, we can use ElementTree
to generate XML/RSS files:
import xml.etree.ElementTree as ET # Create root element root = ET.Element('rss') channel = ET.SubElement(root, 'channel') item = ET.SubElement(channel, 'item') # Add child element ET.SubElement(item, 'title').text = 'Example Title' ET.SubElement(item, 'link').text = 'https://example.com' # Generate XML file tree = ET.ElementTree(root) tree.write('output.rss', encoding='utf-8', xml_declaration=True)
How it works
The core of XML/RSS parsing is the traversal of tree structures and node operations. The parser will read the XML file into a tree structure, and we can then access and manipulate the nodes in it by traversing the tree. For RSS files, channel
node is usually first found, and then iterates over item
nodes and extracts the data.
To generate XML/RSS files, on the contrary, we start from the root node, gradually add child nodes and data, and finally generate a complete XML tree structure, and then write it to the file.
In terms of performance, the efficiency of XML/RSS parsing and generation mainly depends on the file size and parser implementation. For large files, you may want to consider using a streaming parser to reduce memory footprint.
Example of usage
Basic usage
Let's look at a more practical example, using Python's feedparser
library to parse an RSS feed and extract the contents:
import feedparser # parse RSS feeds feed = feedparser.parse('https://example.com/feed') # traverse RSS items for entry in feed.entries: print(f'Title: {entry.title}, Link: {entry.link}, Published: {entry.published}')
This code shows how to use the feedparser
library to parse RSS feeds and extract the title, link, and publish time information. feedparser
is a very convenient tool that can handle feeds in various RSS and Atom formats, simplifying the parsing process.
Advanced Usage
In some complex scenarios, we may need to handle RSS feeds more deeply. For example, we could write a script that automatically extracts content from multiple RSS feeds and generates a summary report:
import feedparser from collections import defaultdict # Define RSS feeds list feeds = [ 'https://example1.com/feed', 'https://example2.com/feed', ] # Initialize the data structure data = defaultdict(list) # traversal RSS feeds for feed_url in feeds: feed = feedparser.parse(feed_url) for entry in feed.entries: data[feed_url].append({ 'title': entry.title, 'link': entry.link, 'published': entry.published, }) # Generate summary report for feed_url, entries in data.items(): print(f'Feed: {feed_url}') for entry in entries: print(f' - Title: {entry["title"]}, Link: {entry["link"]}, Published: {entry["published"]}')
This example shows how to extract content from multiple RSS feeds and generate a summary report. It shows how to use defaultdict
to organize data, and how to iterate through multiple feeds and process the data in it.
Common Errors and Debugging Tips
Common problems when processing XML/RSS data include:
- XML format error : The format of the XML file must strictly comply with the specifications, otherwise the parser will report an error. This type of problem can be avoided using XML verification tools or format checks before parsing.
- Coding issues : XML/RSS files may use different encodings and need to make sure that the parser handles these encodings correctly. When using
xml.etree.ElementTree
, you can specify the file encoding throughencoding
parameter. - Data Loss : During parsing, some fields may not exist or be empty, and appropriate error handling and default value settings are required.
When debugging these problems, you can use the following tips:
- Using debugging tools : Many IDEs and debugging tools can help you gradually track code execution, view variable values, and find out what the problem is.
- Logging : Adding logging to the code can help you track the execution process of the program and find out the specific location where the exception occurs.
- Unit Testing : Writing unit tests can help you verify the correctness of your code and ensure that no new problems are introduced when modifying your code.
Performance optimization and best practices
In practical applications, it is very important to optimize the performance of XML/RSS data integration. Here are some optimization tips and best practices:
- Using Streaming Parser : For large XML/RSS files, using Streaming Parser can reduce memory usage and improve parsing speed. Python's
xml.sax
module provides a method to stream parse XML files. - Cache results : If you need to frequently parse the same XML/RSS file, you can consider cache the parsing results to avoid the performance overhead caused by repeated parsing.
- Parallel processing : If you need to process multiple RSS feeds, you can consider using multi-threaded or multi-process technology to process these feeds in parallel to improve the overall processing speed.
There are some best practices to note when writing code:
- Code readability : Use meaningful variable names and comments to improve the readability of the code and facilitate subsequent maintenance.
- Error handling : Add appropriate error handling to the code to ensure that the program can handle exceptions gracefully instead of crashing directly.
- Modular design : divide the code into multiple modules or functions to improve the reusability and maintainability of the code.
Through these tips and practices, you can integrate XML/RSS data more effectively to improve the performance and reliability of your project.
Summarize
XML/RSS data integration is an important part of many applications. Through this article, you should have mastered how to parse and generate XML/RSS data, understand their application scenarios, and learn some practical best practices and performance optimization techniques. I hope this knowledge and experience can help you better process XML/RSS data in actual projects and improve your development efficiency and project quality.
The above is the detailed content of XML/RSS Data Integration: Practical Guide for Developers & Architects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


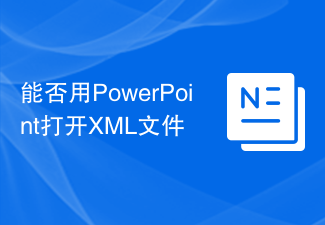
Can XML files be opened with PPT? XML, Extensible Markup Language (Extensible Markup Language), is a universal markup language that is widely used in data exchange and data storage. Compared with HTML, XML is more flexible and can define its own tags and data structures, making the storage and exchange of data more convenient and unified. PPT, or PowerPoint, is a software developed by Microsoft for creating presentations. It provides a comprehensive way of
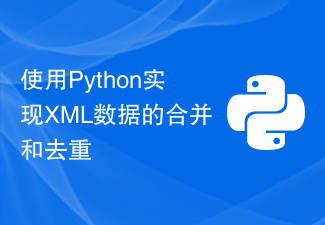
Using Python to merge and deduplicate XML data XML (eXtensibleMarkupLanguage) is a markup language used to store and transmit data. When processing XML data, sometimes we need to merge multiple XML files into one, or remove duplicate data. This article will introduce how to use Python to implement XML data merging and deduplication, and give corresponding code examples. 1. XML data merging When we have multiple XML files, we need to merge them
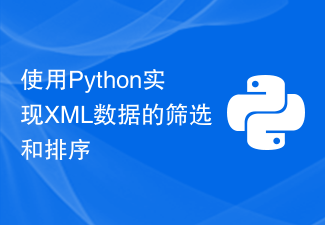
Implementing filtering and sorting of XML data using Python Introduction: XML is a commonly used data exchange format that stores data in the form of tags and attributes. When processing XML data, we often need to filter and sort the data. Python provides many useful tools and libraries to process XML data. This article will introduce how to use Python to filter and sort XML data. Reading the XML file Before we begin, we need to read the XML file. Python has many XML processing libraries,
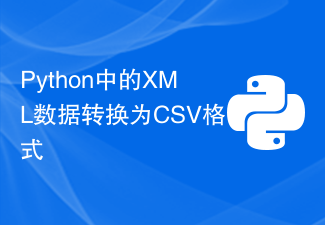
Convert XML data in Python to CSV format XML (ExtensibleMarkupLanguage) is an extensible markup language commonly used for data storage and transmission. CSV (CommaSeparatedValues) is a comma-delimited text file format commonly used for data import and export. When processing data, sometimes it is necessary to convert XML data to CSV format for easy analysis and processing. Python is a powerful
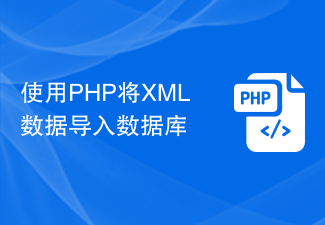
Importing XML data into the database using PHP Introduction: During development, we often need to import external data into the database for further processing and analysis. As a commonly used data exchange format, XML is often used to store and transmit structured data. This article will introduce how to use PHP to import XML data into a database. Step 1: Parse the XML file First, we need to parse the XML file and extract the required data. PHP provides several ways to parse XML, the most commonly used of which is using Simple
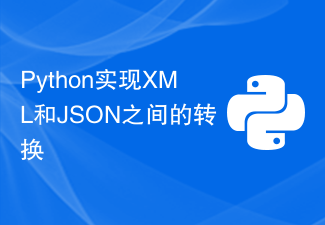
Python implements conversion between XML and JSON Introduction: In the daily development process, we often need to convert data between different formats. XML and JSON are common data exchange formats. In Python, we can use various libraries to convert between XML and JSON. This article will introduce several commonly used methods, with code examples. 1. To convert XML to JSON in Python, we can use the xml.etree.ElementTree module
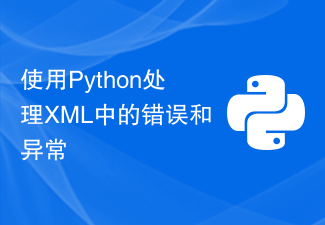
Handling Errors and Exceptions in XML Using Python XML is a commonly used data format used to store and represent structured data. When we use Python to process XML, sometimes we may encounter some errors and exceptions. In this article, I will introduce how to use Python to handle errors and exceptions in XML, and provide some sample code for reference. Use try-except statement to catch XML parsing errors When we use Python to parse XML, sometimes we may encounter some
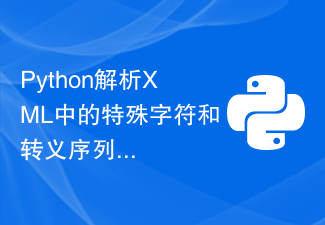
Python parses special characters and escape sequences in XML XML (eXtensibleMarkupLanguage) is a commonly used data exchange format used to transfer and store data between different systems. When processing XML files, you often encounter situations that contain special characters and escape sequences, which may cause parsing errors or misinterpretation of the data. Therefore, when parsing XML files using Python, we need to understand how to handle these special characters and escape sequences. 1. Special characters and
