Designing a JavaScript Plugin System
Many popular libraries and frameworks use plug-in systems such as WordPress, jQuery, Gatsby, Eleventy, and Vue. The plug-in system allows developers to add features in a secure and scalable way, enhancing the value of core projects and building communities while reducing maintenance burdens. This article will explain its design concept by building a JavaScript plug-in system.
We will take an example of a simple JavaScript calculator called BetaCalc, with the goal of allowing other developers to add a "button" feature to it.
Initial calculator code:
// Calculator const betaCalc = { currentValue: 0, setValue(newValue) { this.currentValue = newValue; console.log(this.currentValue); }, plus(addend) { this.setValue(this.currentValue addend); }, minus(subtrahend) { this.setValue(this.currentValue - subtrahend); } }; // Use calculator betaCalc.setValue(3); // => 3 betaCalc.plus(3); // => 6 betaCalc.minus(2); // => 4
This calculator has limited functionality and can only display results through console.log
. Next, we will add the plugin system.
The simplest plug-in system:
We first create a register
method that allows developers to register plugins. This method takes the plugin, gets its exec
function, and attaches it to the calculator as a new method:
// Calculator const betaCalc = { // ...Other calculator code register(plugin) { const { name, exec } = plugin; this[name] = exec; } };
An example of a simple "square" button plugin:
// Define the plugin const squaredPlugin = { name: 'squared', exec: function() { this.setValue(this.currentValue * this.currentValue); } }; // Register plugin betaCalc.register(squaredPlugin);
Plugins usually contain two parts: code and metadata (name, description, version number, dependencies, etc.). In this example, the exec
function contains code and name
is metadata. After registration, exec
function is directly attached to betaCalc
object as a method.
Now, BetaCalc has a new "squared" button:
betaCalc.setValue(3); // => 3 betaCalc.plus(2); // => 5 betaCalc.squared(); // => 25 betaCalc.squared(); // => 625
This method is simple and easy to use, and the plug-in can be easily distributed and imported through npm. But it also has flaws: the plugin directly accesses this
in betaCalc
, has permission to read and write all code, and violates the principle of opening and closing. In addition, the squared
function works through side effects and is not ideal.
Improved plug-in architecture:
To solve these problems, we improve the plug-in architecture:
// Calculator const betaCalc = { currentValue: 0, setValue(value) { this.currentValue = value; console.log(this.currentValue); }, core: { 'plus': (currentVal, addend) => currentVal addend, 'minus': (currentVal, subtrahend) => currentVal - subtrahend }, plugins: {}, press(buttonName, newVal) { const func = this.core[buttonName] || this.plugins[buttonName]; this.setValue(func(this.currentValue, newVal)); }, register(plugin) { const { name, exec } = plugin; this.plugins[name] = exec; } }; // Plugin const squaredPlugin = { name: 'squared', exec: function(currentValue) { return currentValue * currentValue; } }; betaCalc.register(squaredPlugin); // Use calculator betaCalc.setValue(3); // => 3 betaCalc.press('plus', 2); // => 5 betaCalc.press('squared'); // => 25 betaCalc.press('squared'); // => 625
Improvements:
- Separate the plugin from the core method and store it in the
plugins
object to improve security. - Use the
press
method to find functions and call them by name, convert buttons into pure functions, simplifying API, convenient testing, and reducing coupling.
This architecture is more limited, but safer and more reliable. It may be too strict, limiting the functionality extension of the plugin. The permissions of plug-in authors need to be carefully weighed, which not only ensures the stability of the project, but also allows them to solve problems.
Further improvements:
It can be further improved: add error handling, extend plug-in functions (such as registration lifecycle callbacks, storage status, etc.), and improve plug-in registration mechanism (supports batch registration, configuration settings, etc.).
Summarize:
Building an excellent plug-in system is not easy, and requires careful consideration of various factors. Only by referring to the plug-in system of existing projects (such as jQuery, Gatsby, etc.) and JavaScript design patterns, and following software development principles (such as open and shutdown principles, loose coupling, Dimitter rules, dependency injection), can we build a plug-in system that meets all user needs in the long term. A good plug-in system can promote the prosperity of the developer community and achieve a win-win situation.
The above is the detailed content of Designing a JavaScript Plugin System. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


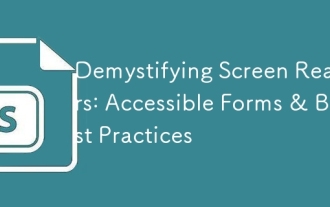
This is the 3rd post in a small series we did on form accessibility. If you missed the second post, check out "Managing User Focus with :focus-visible". In
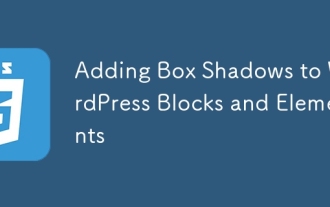
The CSS box-shadow and outline properties gained theme.json support in WordPress 6.1. Let's look at a few examples of how it works in real themes, and what options we have to apply these styles to WordPress blocks and elements.
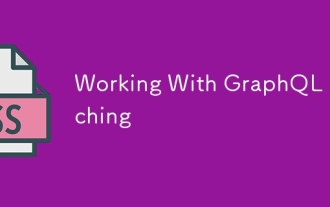
If you’ve recently started working with GraphQL, or reviewed its pros and cons, you’ve no doubt heard things like “GraphQL doesn’t support caching” or
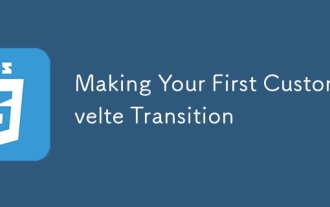
The Svelte transition API provides a way to animate components when they enter or leave the document, including custom Svelte transitions.
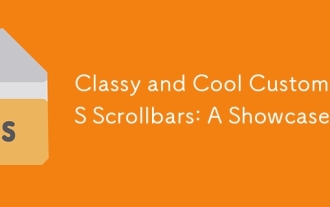
In this article we will be diving into the world of scrollbars. I know, it doesn’t sound too glamorous, but trust me, a well-designed page goes hand-in-hand
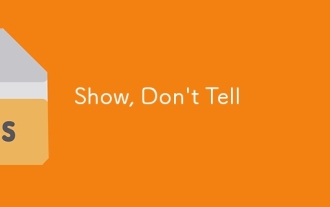
How much time do you spend designing the content presentation for your websites? When you write a new blog post or create a new page, are you thinking about
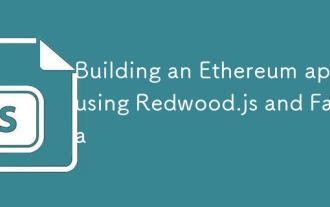
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
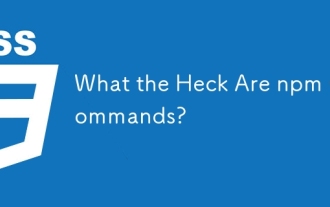
npm commands run various tasks for you, either as a one-off or a continuously running process for things like starting a server or compiling code.
