How to modify content using Java in XML
When Java modify XML, you need to select the parsing library according to the XML file size and modification complexity: DOM (small file, simple modification), SAX/StAX (large file, complex modification). When using DOM parsing, first use the factory class to create a DocumentBuilder, load and parse XML files, and then use the DOM API to operate the node; when using SAX parsing, you need to record the node information and rebuild the XML fragment when modifying. In addition, pay attention to common pitfalls such as exception handling, coding issues, XPath usage and performance optimization, and follow best practices such as using appropriate libraries, writing clear code, fully testing and considering XML Schema validation to write efficient, maintainable XML modification code.
Java Modification of XML: In-depth Analysis and Best Practices
Have you ever thought about how to efficiently modify XML files in Java? This is not a simple string replacement, and the structure, normativeness and efficiency of XML need to be considered. This article will take you into the tips for Java XML modification and share some of the experience I have accumulated over the years and the pitfalls I have stepped on. After reading it, you will be able to write XML modification code that is both elegant and efficient.
Basics: XML and Java
Let's quickly review the basics related to XML and Java. XML is a markup language used to store and transfer data. Java provides a variety of libraries to process XML, the most commonly used ones include DOM and SAX. DOM (Document Object Model) loads the entire XML document into memory, which is convenient for modification, but for large XML files, memory consumption may be a big problem. SAX (Simple API for XML) is an event-based parser that parses XML line by line, has a small memory footprint, is suitable for processing large files, but the modification operations are relatively complex.
DOM analysis and modification: step by step
DOM is the most intuitive way to modify XML. We use the class under javax.xml.parsers
package to parse and modify XML.
<code class="java">import javax.xml.parsers.*; import org.w3c.dom.*; import javax.xml.transform.*; import javax.xml.transform.dom.*; import javax.xml.transform.stream.*; public class ModifyXML { public static void main(String[] args) { try { // 解析XML文档DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document doc = builder.parse("input.xml"); // 找到需要修改的节点(假设我们要修改名为'name'的节点) NodeList nodeList = doc.getElementsByTagName("name"); Node node = nodeList.item(0); // 获取第一个'name'节点node.setTextContent("New Name"); // 修改节点内容// 将修改后的文档写入文件TransformerFactory transformerFactory = TransformerFactory.newInstance(); Transformer transformer = transformerFactory.newTransformer(); DOMSource source = new DOMSource(doc); StreamResult result = new StreamResult("output.xml"); transformer.transform(source, result); System.out.println("XML修改成功!"); } catch (Exception e) { e.printStackTrace(); } } }</code>
This code shows how to parse XML using DOM, find the specified node and modify its contents, and finally write the modified contents to a new XML file. Note that this is just the simplest example, and more complex XML structures and exceptions may be required in practical applications. For example, if the target node is not found, the program should handle it gracefully instead of throwing an exception termination.
SAX parsing and modification: efficiently process large files
For large XML files, SAX is a better choice. SAX does not load the entire document into memory, but parses it line by line, making the memory footprint smaller. However, modifying XML with SAX is more complicated. It requires recording node information during parsing and rebuilding XML fragments when modification is needed. This requires a deeper understanding of the XML structure and the code will be much more complex than the DOM. I personally recommend using StAX (Streaming API for XML), which combines the advantages of DOM and SAX, which can process large files efficiently and is relatively easy to use.
Avoid common pitfalls
Probably encountered problems when dealing with XML include:
- Exception handling: Various exceptions may occur during XML parsing and modification, such as file failure, format errors, etc. Be sure to write complete exception handling code to avoid program crashes.
- Coding issues: Make sure that the XML files and Java code use the same encoding to avoid garbled code.
- Use of XPath: For complex XML structures, XPath expressions can conveniently locate nodes, improve code efficiency and readability.
- Performance optimization: For frequent XML modification operations, consider using memory pools or other optimization techniques to improve performance.
Best Practices and Recommendations
To write efficient and maintainable XML modification code, it is recommended:
- Use the appropriate XML parsing library: Select DOM or SAX/StAX according to the XML file size and modification complexity.
- Write clear code: use meaningful variable names and comments to improve code readability.
- Perform adequate testing: Make sure the code can handle various situations correctly, including exceptions.
- Consider using XML Schema Verification: Make sure the XML file is formatted correctly.
In short, it is not easy for Java to modify XML, and requires a deep understanding of XML and Java. Only by choosing the right tools, writing clear code, and paying attention to potential pitfalls can you write efficient and reliable XML modification programs. Remember, the elegance and efficiency of the code are equally important. Only by continuing to learn and practice can you become a true programming expert.
The above is the detailed content of How to modify content using Java in XML. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


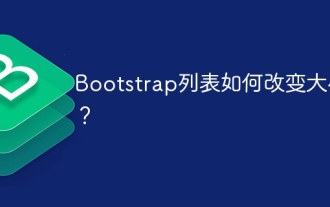
The size of a Bootstrap list depends on the size of the container that contains the list, not the list itself. Using Bootstrap's grid system or Flexbox can control the size of the container, thereby indirectly resizing the list items.
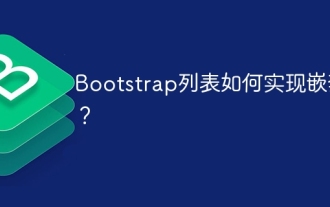
Nested lists in Bootstrap require the use of Bootstrap's grid system to control the style. First, use the outer layer <ul> and <li> to create a list, then wrap the inner layer list in <div class="row> and add <div class="col-md-6"> to the inner layer list to specify that the inner layer list occupies half the width of a row. In this way, the inner list can have the right one
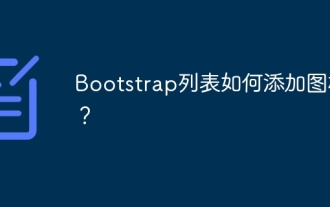
How to add icons to the Bootstrap list: directly stuff the icon into the list item <li>, using the class name provided by the icon library (such as Font Awesome). Use the Bootstrap class to align icons and text (for example, d-flex, justify-content-between, align-items-center). Use the Bootstrap tag component (badge) to display numbers or status. Adjust the icon position (flex-direction: row-reverse;), control the style (CSS style). Common error: The icon does not display (not
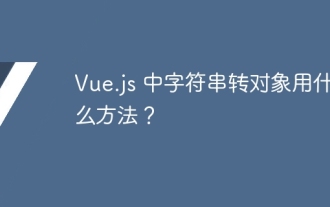
When converting strings to objects in Vue.js, JSON.parse() is preferred for standard JSON strings. For non-standard JSON strings, the string can be processed by using regular expressions and reduce methods according to the format or decoded URL-encoded. Select the appropriate method according to the string format and pay attention to security and encoding issues to avoid bugs.
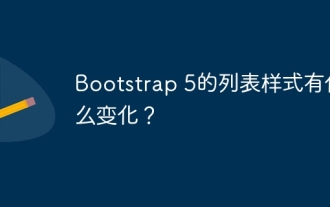
Bootstrap 5 list style changes are mainly due to detail optimization and semantic improvement, including: the default margins of unordered lists are simplified, and the visual effects are cleaner and neat; the list style emphasizes semantics, enhancing accessibility and maintainability.
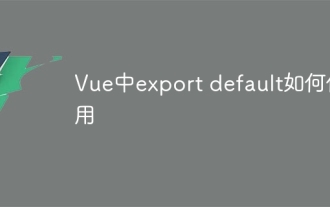
Export default in Vue reveals: Default export, import the entire module at one time, without specifying a name. Components are converted into modules at compile time, and available modules are packaged through the build tool. It can be combined with named exports and export other content, such as constants or functions. Frequently asked questions include circular dependencies, path errors, and build errors, requiring careful examination of the code and import statements. Best practices include code segmentation, readability, and component reuse.
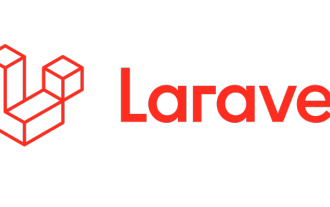
Efficiently process 7 million records and create interactive maps with geospatial technology. This article explores how to efficiently process over 7 million records using Laravel and MySQL and convert them into interactive map visualizations. Initial challenge project requirements: Extract valuable insights using 7 million records in MySQL database. Many people first consider programming languages, but ignore the database itself: Can it meet the needs? Is data migration or structural adjustment required? Can MySQL withstand such a large data load? Preliminary analysis: Key filters and properties need to be identified. After analysis, it was found that only a few attributes were related to the solution. We verified the feasibility of the filter and set some restrictions to optimize the search. Map search based on city
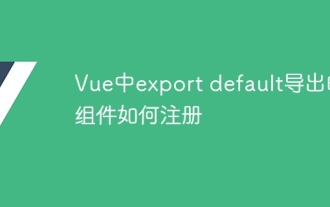
Question: How to register a Vue component exported through export default? Answer: There are three registration methods: Global registration: Use the Vue.component() method to register as a global component. Local Registration: Register in the components option, available only in the current component and its subcomponents. Dynamic registration: Use the Vue.component() method to register after the component is loaded.
