Does XML modification require programming?
Modifying XML content requires programming, because it requires accurate finding of the target nodes to add, delete, modify and check. The programming language has corresponding libraries to process XML and provides APIs to perform safe, efficient and controllable operations like operating databases.
XML modification content is necessary. Don't think about using any fool-like tool to solve all situations with one click, that's unrealistic. This article will talk about why and how to do it.
Think about it, XML is structured data, not as casual as text files. If you want to modify it, you have to accurately find the target node, and then you can add, delete, modify and check it. Manually change? Unless your XML file has only a few lines and is as simple as a diary written by a primary school student. If you are a little more complicated, you will find yourself trapped in the quagmire of code - manual modification is inefficient, prone to errors, and easily destroy the XML structure, causing the program to crash. Don't believe it? Try it, and you can make sure you are crying without tears.
Therefore, using programming languages to manipulate XML is the right way. Different languages have different libraries to handle XML, Python has xml.etree.ElementTree
, Java has javax.xml.parsers
, C# has System.Xml
, etc. They provide a set of APIs that allow you to operate XML like a database, which is safe, efficient and controllable.
Let's use Python's xml.etree.ElementTree
to demonstrate it. Suppose we have a simple XML file with the following content:
<code class="xml"><bookstore> <book category="cooking"> <title lang="en">Everyday Italian</title> <author>Giada De Laurentiis</author> <year>2005</year> <price>30.00</price> </book> <book category="children"> <title lang="en">Harry Potter</title> <author>J K. Rowling</author> <year>2005</year> <price>29.99</price> </book> </bookstore></code>
Now, we are going to change the price of Harry Potter to 35.00. The code is as follows:
<code class="python">import xml.etree.ElementTree as ET tree = ET.parse('books.xml') # 解析XML文件root = tree.getroot() # 获取根节点for book in root.findall('book'): # 遍历所有book节点if book.find('title').text == 'Harry Potter': # 找到Harry Potter price = book.find('price') # 找到price节点price.text = '35.00' # 修改price的值break # 找到就退出循环,避免重复修改tree.write('books_modified.xml') # 写入修改后的XML文件</code>
This code is concise and clear, but it only handles simple modifications. In practical applications, the XML structure may be very complex. You need to deal with namespaces, CDATA segments, properties, etc. This requires more advanced skills, and even requires XPath to locate nodes.
In addition, parsing and modification of XML can also bring performance problems, especially when dealing with large XML files. At this point, you need to consider using more advanced XML processing libraries, or optimizing your code, such as using an iterator to avoid loading the entire XML document into memory. Choosing the right library and algorithm is crucial, depending on the size and complexity of your XML file, as well as your performance requirements. Blind choices can lead to inefficiency and even program crashes.
In short, programming is indispensable to modifying XML content. Only by choosing the right tools and methods can you complete tasks efficiently and safely. Don’t underestimate the complexity of XML, and don’t expect to achieve it overnight. Only by practicing and learning more can you become an expert in XML modification.
The above is the detailed content of Does XML modification require programming?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










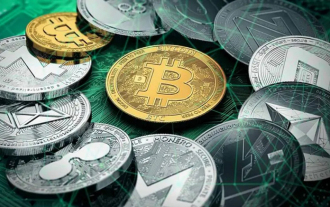
Factors of rising virtual currency prices include: 1. Increased market demand, 2. Decreased supply, 3. Stimulated positive news, 4. Optimistic market sentiment, 5. Macroeconomic environment; Decline factors include: 1. Decreased market demand, 2. Increased supply, 3. Strike of negative news, 4. Pessimistic market sentiment, 5. Macroeconomic environment.

IIS and PHP are compatible and are implemented through FastCGI. 1.IIS forwards the .php file request to the FastCGI module through the configuration file. 2. The FastCGI module starts the PHP process to process requests to improve performance and stability. 3. In actual applications, you need to pay attention to configuration details, error debugging and performance optimization.
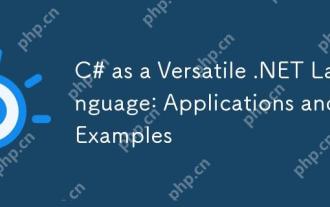
C# is widely used in enterprise-level applications, game development, mobile applications and web development. 1) In enterprise-level applications, C# is often used for ASP.NETCore to develop WebAPI. 2) In game development, C# is combined with the Unity engine to realize role control and other functions. 3) C# supports polymorphism and asynchronous programming to improve code flexibility and application performance.
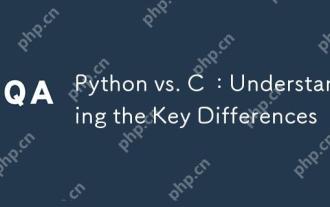
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
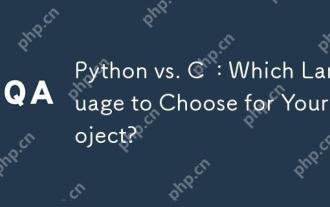
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.

Laravel is suitable for projects that teams are familiar with PHP and require rich features, while Python frameworks depend on project requirements. 1.Laravel provides elegant syntax and rich features, suitable for projects that require rapid development and flexibility. 2. Django is suitable for complex applications because of its "battery inclusion" concept. 3.Flask is suitable for fast prototypes and small projects, providing great flexibility.
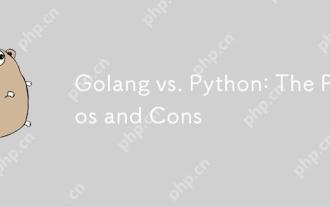
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
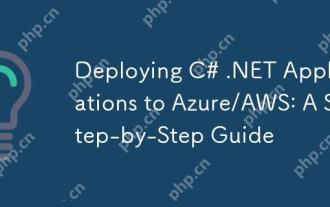
How to deploy a C# .NET app to Azure or AWS? The answer is to use AzureAppService and AWSElasticBeanstalk. 1. On Azure, automate deployment using AzureAppService and AzurePipelines. 2. On AWS, use Amazon ElasticBeanstalk and AWSLambda to implement deployment and serverless compute.
