How to add new nodes in XML
XML node addition tips: Create a new node using the SubElement function of the ElementTree library by understanding the tree structure and finding the appropriate insertion point. More complex scenarios require selective insertion or batch addition based on node attributes or content, which requires logical judgment and looping. For large files, consider using a faster lxml library. Following a good code style, clear annotations help the readability and maintainability of the code.
XML adds branches and leaves: elegant addition of nodes
Have you ever been troubled by the structure of XML documents? Want to insert a new node into it, but it feels like adding bricks and tiles to a precise gear device, be careful but unable to do it? Don't worry, this article will take you to appreciate the exquisiteness of XML node addition, helping you easily control this seemingly complex structure.
The purpose of this article is to let you fully master the various techniques of adding new nodes to XML documents, from the most basic insertion to advanced batch operations, so that you no longer have to worry about modification of XML documents. After reading, you will be able to confidently handle various XML node addition scenarios and write efficient and elegant code.
The core of XML is tree-like structures, and understanding this is crucial. Each node has its parent node (except the root node), and possibly child nodes. Adding a new node is essentially finding the right insertion point in this tree structure, then creating a new node and connecting it to the tree.
We demonstrate it in Python because it is concise and clear, and has a powerful XML processing library xml.etree.ElementTree
. This library comes with Python and does not require additional installation, which is really good news.
Let's first take a simple example. Suppose you have an XML document with the following content:
<code class="xml"><bookstore> <book category="cooking"> <title lang="en">Everyday Italian</title> <author>Giada De Laurentiis</author> <year>2005</year> <price>30.00</price> </book> </bookstore></code>
Now you want to add a new <description></description>
node inside <book></book>
node. The code is as follows:
<code class="python">import xml.etree.ElementTree as ET tree = ET.parse('bookstore.xml') root = tree.getroot() for book in root.findall('book'): description = ET.SubElement(book, 'description') description.text = 'A great book for learning Italian cooking.' tree.write('bookstore_updated.xml')</code>
This code first parses the XML file and then finds all <book></book>
nodes. The ET.SubElement
function is the key, which creates a new child node within the specified parent node (here is <book></book>
) and returns the object of this new node. We set the text content of the new node and finally write the modified XML to the new file.
This is just the most basic usage. In actual applications, you may need to select the insertion location based on the node's properties or text content, or you may need to add multiple nodes in batches. This requires more complex logical judgments and loop operations.
For example, you may need to decide whether to add a <description></description>
node based on category
attribute of <book></book>
node, or you need to control the number of added nodes based on the number of existing nodes. All of these require you to have a deeper understanding of XML structure and Python programming.
In addition, efficiency is crucial when dealing with large XML files. The xml.etree.ElementTree
library performs well when dealing with medium-sized XML files, but for super-large files you may want to consider using more efficient libraries such as lxml
. The lxml
library is faster and has lower memory footprint, especially when dealing with large XML files, which has obvious advantages. But it requires additional installation.
Finally, remember that a good code style and comments are essential for the readability and maintainability of your code. Clear code is not only convenient for one's own understanding, but also for others to read and modify.
Adding XML nodes is not a difficult task. By mastering its core principles and techniques, you can easily deal with various scenarios. Remember, practice to truly master this skill. I wish you a happy programming!
The above is the detailed content of How to add new nodes in XML. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


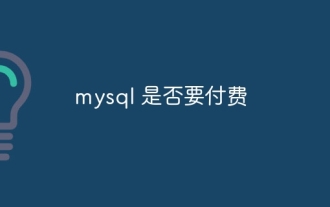
MySQL has a free community version and a paid enterprise version. The community version can be used and modified for free, but the support is limited and is suitable for applications with low stability requirements and strong technical capabilities. The Enterprise Edition provides comprehensive commercial support for applications that require a stable, reliable, high-performance database and willing to pay for support. Factors considered when choosing a version include application criticality, budgeting, and technical skills. There is no perfect option, only the most suitable option, and you need to choose carefully according to the specific situation.

HadiDB: A lightweight, high-level scalable Python database HadiDB (hadidb) is a lightweight database written in Python, with a high level of scalability. Install HadiDB using pip installation: pipinstallhadidb User Management Create user: createuser() method to create a new user. The authentication() method authenticates the user's identity. fromhadidb.operationimportuseruser_obj=user("admin","admin")user_obj.
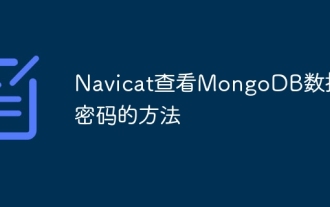
It is impossible to view MongoDB password directly through Navicat because it is stored as hash values. How to retrieve lost passwords: 1. Reset passwords; 2. Check configuration files (may contain hash values); 3. Check codes (may hardcode passwords).
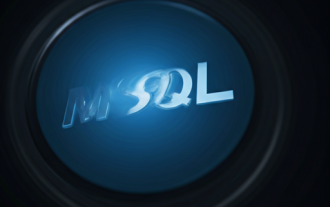
MySQL database performance optimization guide In resource-intensive applications, MySQL database plays a crucial role and is responsible for managing massive transactions. However, as the scale of application expands, database performance bottlenecks often become a constraint. This article will explore a series of effective MySQL performance optimization strategies to ensure that your application remains efficient and responsive under high loads. We will combine actual cases to explain in-depth key technologies such as indexing, query optimization, database design and caching. 1. Database architecture design and optimized database architecture is the cornerstone of MySQL performance optimization. Here are some core principles: Selecting the right data type and selecting the smallest data type that meets the needs can not only save storage space, but also improve data processing speed.
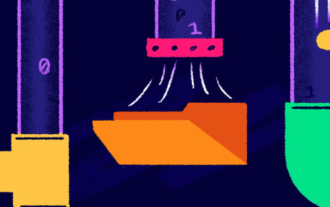
As a data professional, you need to process large amounts of data from various sources. This can pose challenges to data management and analysis. Fortunately, two AWS services can help: AWS Glue and Amazon Athena.
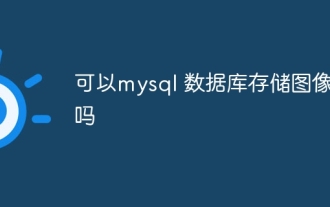
Storing images in a MySQL database is feasible, but not best practice. MySQL uses the BLOB type when storing images, but it can cause database volume swell, query speed and complex backups. A better solution is to store images on a file system and store only image paths in the database to optimize query performance and database volume.
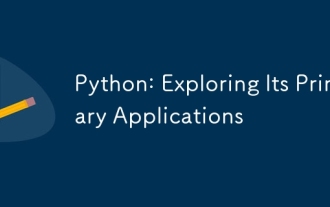
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
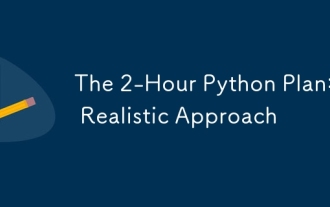
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
