Is Vue used for frontend or backend?
Vue.js is mainly used for front-end development. 1) It is a lightweight and flexible JavaScript framework focused on building user interfaces and single-page applications. 2) The core of Vue.js is its responsive data system, and the view is automatically updated when the data changes. 3) It supports component development, and the UI can be split into independent and reusable components.
Vue.js is primarily used for frontend development. It's a progressive JavaScript framework that focuses on building user interfaces and single-page applications. While Vue itself is not used for backend development, it can be integrated with various backend technologies to create full-stack applications.
Vue.js: Explore the art of front-end development
introduction
Vue.js, this is a JavaScript framework that makes front-end development more interesting and efficient. Why choose Vue.js? Because it is not only lightweight, but also extremely flexible, allowing developers to build complex user interfaces at the lowest cost. Today, we will explore the front-end development art of Vue.js, from basic to advanced usage, to performance optimization and best practices, to take you into a comprehensive understanding of the charm of Vue.js.
Review of basic knowledge
At the heart of Vue.js is its responsive data system, which means that the view will automatically update when the data changes. Understanding the responsive principle of Vue.js is the key to mastering its foundation. In addition, Vue.js also provides the concept of component development, allowing us to split the UI into independent and reusable components.
Before using Vue.js, understanding the basic syntax of JavaScript, DOM operations, and the new features of ES6 will be of great help to learn. The design concept of Vue.js is progressive, you can start with simplicity and gradually penetrate into more complex application development.
Core concept or function analysis
Vue.js' responsive system
Vue.js' responsive system is one of its core, and it implements data monitoring through Object.defineProperty
or Proxy
(in Vue 3). When the data changes, Vue.js automatically detects and updates the view. This mechanism allows developers to focus on business logic without having to manually operate the DOM.
// Simple responsive example const vm = new Vue({ el: '#app', data: { message: 'Hello Vue!' } }) // When vm.message changes, the view will automatically update vm.message = 'Hello World!'
Component development
Componentization is another core concept of Vue.js. Through components, we can split the UI into separate, reusable parts. Each component has its own logic and templates, which makes organizing and maintaining code easier.
// Define a simple component Vue.component('my-component', { template: '<div>A custom component!</div>' })
How it works
The working principle of Vue.js can be understood from three aspects: its life cycle, virtual DOM and responsive system. The life cycle hook function allows us to execute specific logic at different stages of the component; the virtual DOM improves rendering performance by building a lightweight DOM tree in memory; and the responsive system ensures that the view is automatically updated when data changes.
Example of usage
Basic usage
Let's start with a simple Vue.js application:
// Create a Vue instance new Vue({ el: '#app', data: { message: 'Hello Vue.js!' } })
This example shows how to create a Vue instance and mount it onto a DOM element. message
attribute in data
object will automatically become responsive, and the view will be automatically updated when its value changes.
Advanced Usage
What makes Vue.js powerful is its flexibility and scalability. Let's look at a more complex example using computed properties and methods to process data:
new Vue({ el: '#app', data: { firstName: 'John', lastName: 'Doe' }, computed: { fullName() { return this.firstName ' ' this lastName } }, methods: { updateName() { this.firstName = 'Jane' } } })
In this example, we use the calculation property fullName
to calculate the full name dynamically and define a method updateName
to update the data. Computed properties are automatically updated when their dependencies change, while methods need to be called manually.
Common Errors and Debugging Tips
When using Vue.js, common errors include data not being correctly bound, components not being registered correctly, etc. Here are some debugging tips:
- Using the
Vue Devtools
browser plug-in, you can view and modify the status of Vue applications in real time. - Check the console error message, Vue.js will provide detailed error prompts.
- When using
v-if
andv-show
, pay attention to their differences and usage scenarios.
Performance optimization and best practices
In practical applications, it is very important to optimize the performance of Vue.js applications. Here are some optimization tips and best practices:
- When using
v-for
, remember to addkey
attributes to each item to improve rendering efficiency. - For large applications, consider using
Vuex
to manage state and avoid data transfer confusion between components. - Use
keep-alive
components to cache infrequently changing components and reduce unnecessary re-rendering.
When writing Vue.js code, it is also very important to keep the code readable and maintainable. Here are some best practices:
- Component naming should be clear and meaningful, following the single responsibility principle.
- Use
props
andevents
to communicate between components to avoid directly modifying the data of the parent component. - Use the life cycle hook function reasonably and execute logic at the right time.
Through these tips and practices, you can better utilize Vue.js to build efficient and maintainable front-end applications. I hope this article can help you understand the front-end development art of Vue.js and flexibly use it in actual projects.
The above is the detailed content of Is Vue used for frontend or backend?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


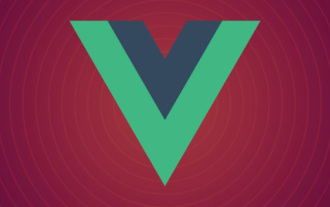
When using the Vue framework to develop front-end projects, we will deploy multiple environments when deploying. Often the interface domain names called by development, testing and online environments are different. How can we make the distinction? That is using environment variables and patterns.
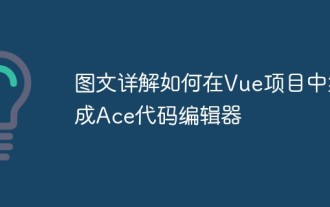
Ace is an embeddable code editor written in JavaScript. It matches the functionality and performance of native editors like Sublime, Vim, and TextMate. It can be easily embedded into any web page and JavaScript application. Ace is maintained as the main editor for the Cloud9 IDE and is the successor to the Mozilla Skywriter (Bespin) project.
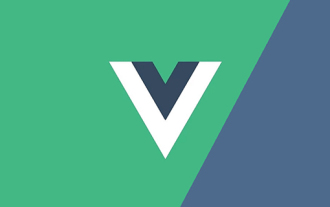
Foreword: In the development of vue3, reactive provides a method to implement responsive data. This is a frequently used API in daily development. In this article, the author will explore its internal operating mechanism.
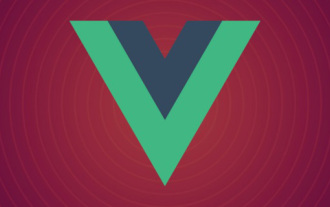
Vue.js has become a very popular framework in front-end development today. As Vue.js continues to evolve, unit testing is becoming more and more important. Today we’ll explore how to write unit tests in Vue.js 3 and provide some best practices and common problems and solutions.
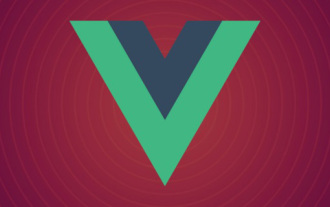
In Vue.js, developers can use two different syntaxes to create user interfaces: JSX syntax and template syntax. Both syntaxes have their own advantages and disadvantages. Let’s discuss their differences, advantages and disadvantages.
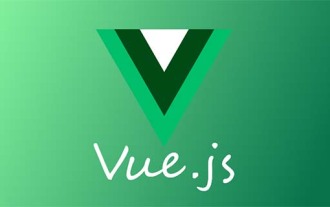
In the actual development project process, sometimes it is necessary to upload relatively large files, and then the upload will be relatively slow, so the background may require the front-end to upload file slices. It is very simple. For example, 1 A gigabyte file stream is cut into several small file streams, and then the interface is requested to deliver the small file streams respectively.
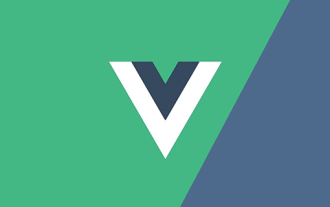
Since the release of Vue3, the word composition API has entered the field of vision of students who write Vue. I believe everyone has always heard how much better the composition API is than the previous options API. Now, due to the release of the @vue/composition-api plug-in, Vue2 Students can also get on the bus. Next, we will mainly use responsive ref and reactive to conduct an in-depth analysis of how this plug-in achieves this.
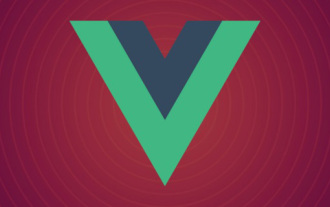
There are two ways to query the current Vue version: 1. In the cmd console, execute the "npm list vue" command to query the version. The output result is the version number information of Vue; 2. Find and open the package.json file in the project and search You can see the version information of vue in the "dependencies" item.
