Is NULL a value in C?
NULL is a macro in C language, usually defined as 0 or null pointer constant (void *)0. It means that the pointer does not point to a valid memory address, and access to the memory referenced by the pointer is prohibited to avoid causing the program to crash. It is crucial to use NULL correctly (such as checking if the pointer is empty) to avoid pointer errors and improve program robustness.
What is NULL in C?
Many beginners, even some programmers with certain experience, have their understanding of NULL
at the level of "null pointer". This is actually not in-depth enough and may even lead to some difficult-to-know bugs. So, let's talk about this seemingly simple NULL
today.
The purpose of this article is to let you fully understand the essence of NULL
in C language and how to use it safely and effectively, so as to avoid crazy pointer errors. After reading, you will have a deeper understanding of NULL
and be able to write more robust and less error-prone C code.
First of all, we need to be clear: NULL
itself is not a value, but a macro. It is usually defined as 0, or a null pointer constant. It depends on the compiler implementation. This subtle difference is crucial! Only by understanding this can you avoid many misunderstandings.
Let's take a look at a typical NULL
definition:
<code class="c">#ifdef __cplusplus #define NULL 0 #else #define NULL ((void *)0) #endif</code>
This code shows two common ways of defining NULL
. In C, NULL
is usually defined as 0, while in C, to avoid type conversion problems, it is usually defined as (void *)0
. This represents a null pointer to void
type. void
type means there is no type, and it is safe to convert it to any pointer type.
Why is it defined like this? Because the pointer type in C language is strongly typed. If you directly assign a pointer variable with 0
, the compiler may issue a warning or even report an error. (void *)0
eliminates this ambiguity and ensures type compatibility. This reflects the importance C language attaches to type safety and explains why the definition of NULL
is not static.
So, how exactly does NULL
work? When we assign NULL
to a pointer, it actually means that the pointer does not point to any valid memory address. Trying to access memory through this pointer will lead to undefined behavior, at the least the program crashes, at the worst the system crashes, and even security vulnerabilities!
Let's take a look at an example and feel the power of NULL
:
<code class="c">#include <stdio.h> #include <stdlib.h> int main() { int *ptr = NULL; if (ptr == NULL) { printf("指针ptr为空指针\n"); } else { printf("指针ptr指向的内存值为:%d\n", *ptr); //千万别运行这行! } return 0; }</stdlib.h></stdio.h></code>
This code shows how to check if a pointer is NULL
. The if (ptr == NULL)
statement is a standard way to check for null pointers. Never try to uncomment the code in the else
block, otherwise your program will hang up directly.
More advanced usage, such as checking whether the return value of functions such as malloc
, calloc
is NULL
after dynamic memory allocation, is crucial in avoiding memory leaks and segfaults. Forgot to check the return value is a common cause of crashes in many C programs.
Let’s talk about performance optimization. NULL
itself does not directly affect program performance, but using NULL
incorrectly will cause program crashes, thereby indirectly affecting performance. Therefore, developing good programming habits and always checking whether the pointer is NULL
before using it is the key to improving program robustness and performance.
Finally, remember that NULL
is an important concept in C, but it is not a value itself, but a macro, and its definition may also vary from compiler to compiler. Only by understanding its essence and developing good programming habits can we avoid various problems caused by NULL
pointers. Using NULL
safely is one of the keys to writing high-quality C code.
The above is the detailed content of Is NULL a value in C?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


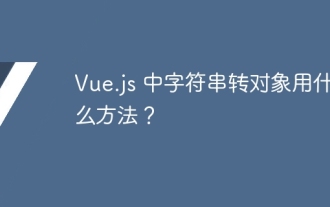
When converting strings to objects in Vue.js, JSON.parse() is preferred for standard JSON strings. For non-standard JSON strings, the string can be processed by using regular expressions and reduce methods according to the format or decoded URL-encoded. Select the appropriate method according to the string format and pay attention to security and encoding issues to avoid bugs.
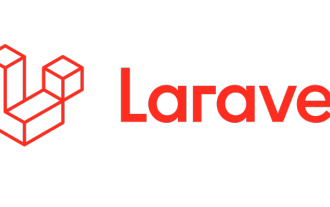
Efficiently process 7 million records and create interactive maps with geospatial technology. This article explores how to efficiently process over 7 million records using Laravel and MySQL and convert them into interactive map visualizations. Initial challenge project requirements: Extract valuable insights using 7 million records in MySQL database. Many people first consider programming languages, but ignore the database itself: Can it meet the needs? Is data migration or structural adjustment required? Can MySQL withstand such a large data load? Preliminary analysis: Key filters and properties need to be identified. After analysis, it was found that only a few attributes were related to the solution. We verified the feasibility of the filter and set some restrictions to optimize the search. Map search based on city
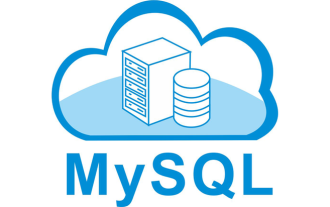
MySQL performance optimization needs to start from three aspects: installation configuration, indexing and query optimization, monitoring and tuning. 1. After installation, you need to adjust the my.cnf file according to the server configuration, such as the innodb_buffer_pool_size parameter, and close query_cache_size; 2. Create a suitable index to avoid excessive indexes, and optimize query statements, such as using the EXPLAIN command to analyze the execution plan; 3. Use MySQL's own monitoring tool (SHOWPROCESSLIST, SHOWSTATUS) to monitor the database health, and regularly back up and organize the database. Only by continuously optimizing these steps can the performance of MySQL database be improved.
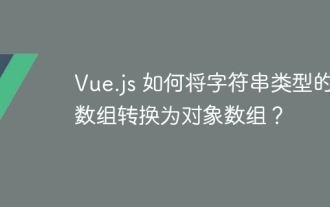
Summary: There are the following methods to convert Vue.js string arrays into object arrays: Basic method: Use map function to suit regular formatted data. Advanced gameplay: Using regular expressions can handle complex formats, but they need to be carefully written and considered. Performance optimization: Considering the large amount of data, asynchronous operations or efficient data processing libraries can be used. Best practice: Clear code style, use meaningful variable names and comments to keep the code concise.
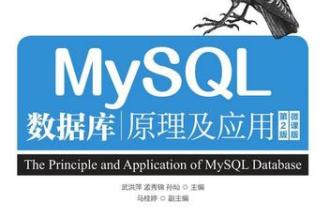
MySQL installation failure is usually caused by the lack of dependencies. Solution: 1. Use system package manager (such as Linux apt, yum or dnf, Windows VisualC Redistributable) to install the missing dependency libraries, such as sudoaptinstalllibmysqlclient-dev; 2. Carefully check the error information and solve complex dependencies one by one; 3. Ensure that the package manager source is configured correctly and can access the network; 4. For Windows, download and install the necessary runtime libraries. Developing the habit of reading official documents and making good use of search engines can effectively solve problems.
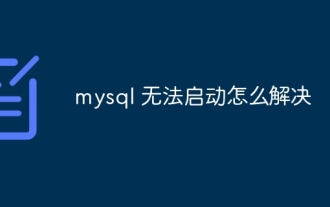
There are many reasons why MySQL startup fails, and it can be diagnosed by checking the error log. Common causes include port conflicts (check port occupancy and modify configuration), permission issues (check service running user permissions), configuration file errors (check parameter settings), data directory corruption (restore data or rebuild table space), InnoDB table space issues (check ibdata1 files), plug-in loading failure (check error log). When solving problems, you should analyze them based on the error log, find the root cause of the problem, and develop the habit of backing up data regularly to prevent and solve problems.
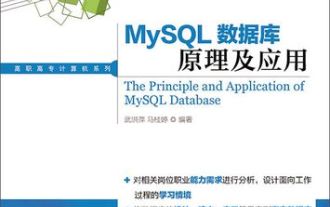
The article introduces the operation of MySQL database. First, you need to install a MySQL client, such as MySQLWorkbench or command line client. 1. Use the mysql-uroot-p command to connect to the server and log in with the root account password; 2. Use CREATEDATABASE to create a database, and USE select a database; 3. Use CREATETABLE to create a table, define fields and data types; 4. Use INSERTINTO to insert data, query data, update data by UPDATE, and delete data by DELETE. Only by mastering these steps, learning to deal with common problems and optimizing database performance can you use MySQL efficiently.
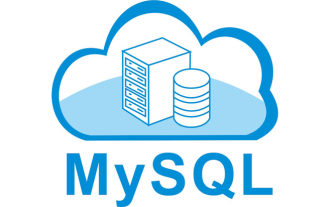
MySQL refused to start? Don’t panic, let’s check it out! Many friends found that the service could not be started after installing MySQL, and they were so anxious! Don’t worry, this article will take you to deal with it calmly and find out the mastermind behind it! After reading it, you can not only solve this problem, but also improve your understanding of MySQL services and your ideas for troubleshooting problems, and become a more powerful database administrator! The MySQL service failed to start, and there are many reasons, ranging from simple configuration errors to complex system problems. Let’s start with the most common aspects. Basic knowledge: A brief description of the service startup process MySQL service startup. Simply put, the operating system loads MySQL-related files and then starts the MySQL daemon. This involves configuration
