C# multi-threaded method to prevent jamming
The following ways to avoid "stuck" multithreading in C#: Avoid performing time-consuming operations on UI threads. Use Task and async/await to perform time-consuming operations asynchronously. Update the UI on the UI thread via Application.Current.Dispatcher.Invoke. Use CancellationToken to control task cancellation. Make rational use of thread pools to avoid excessive creation of threads. Pay attention to code readability and maintainability, making it easy to debug. Logs are recorded in each thread for easy debugging.
C# Multithreading: The Art of Elegantly Avoiding "Hard"
You must have had this experience: the program suddenly stops moving, the mouse pointer becomes an hourglass, and the world seems to be still... The concurrency ability brought by multi-threading programming is tempting, but if you are not careful, it may become a nightmare for your program, causing you to fall into the abyss of "stagnation". In this article, let’s talk about how to elegantly avoid this embarrassment in C#, so that your multi-threaded program can gallop like a wild horse without losing control.
First of all, you need to understand that the so-called "stuck" is usually not a problem with the thread itself, but the UI thread is blocked. Your program may have multiple hard-working threads, but if they are all waiting for the UI thread to process the results, once the UI thread is blocked, the entire program will be in a state of silence. Therefore, the key is to remove the burden on UI threads.
Let's review the basics first. C# provides Thread
class and Task
class for multi-thread programming. Task
is based on ThreadPool
, which is more convenient to manage and has higher resource utilization, so we mainly use it. In addition, the keywords async
and await
are powerful tools for asynchronous programming. They make the asynchronous code look as simple as synchronous code and are the key to avoid blocking UI threads.
Now, let's dive into the core: how to avoid UI thread blocking. The most common solution is to use BackgroundWorker
, but it is a bit outdated, and it is now more recommended to use Task
in combination with async/await
. Take a look at this example:
<code class="csharp">private async void Button_Click(object sender, RoutedEventArgs e) { // 禁用按钮,防止重复点击button1.IsEnabled = false; try { await Task.Run(() => { // 模拟耗时操作Thread.Sleep(5000); // 更新UI需要使用Dispatcher Application.Current.Dispatcher.Invoke(() => { label1.Content = "耗时操作完成!"; }); }); } catch (Exception ex) { // 处理异常Application.Current.Dispatcher.Invoke(() => { label1.Content = $"错误: {ex.Message}"; }); } finally { // 启用按钮button1.IsEnabled = true; } }</code>
In this code, the time-consuming operations are placed in Task.Run
and executed asynchronously. The key lies in Application.Current.Dispatcher.Invoke
, which ensures that UI update operations are executed on the UI thread, avoiding conflicts. try-catch-finally
block ensures the robustness of the program, and can be handled gracefully even if an exception occurs, and will not cause the program to crash.
To be more advanced, you can use CancellationToken
to control task cancellation to avoid unnecessary resource waste. For example, if the user clicks the "Cancel" button, you can cancel the executing task through CancellationTokenSource
.
Remember, don't perform time-consuming operations on UI threads! This is the golden rule of multi-threaded programming. Any operations that may block UI threads should be executed in a separate thread and then updated with Dispatcher
or similar mechanism.
Finally, regarding performance optimization, try to avoid overuse of locks, as locks will reduce concurrent performance. Use thread pools reasonably to avoid creating too many threads. The readability and maintainability of the code are also very important. Clear code is easier to debug and maintain, reducing the possibility of "stagnation". A good habit is to log in every thread so that problems can be quickly located during debugging. Remember, elegant multi-threading programming requires you to have a deep understanding of concepts such as concurrency, asynchronousness, thread safety, etc. This requires accumulation of experience and continuous learning and practice.
The above is the detailed content of C# multi-threaded method to prevent jamming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


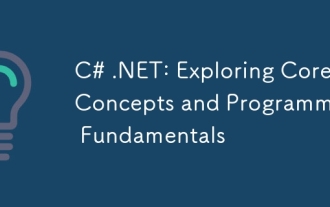
C# is a modern, object-oriented programming language developed by Microsoft and as part of the .NET framework. 1.C# supports object-oriented programming (OOP), including encapsulation, inheritance and polymorphism. 2. Asynchronous programming in C# is implemented through async and await keywords to improve application responsiveness. 3. Use LINQ to process data collections concisely. 4. Common errors include null reference exceptions and index out-of-range exceptions. Debugging skills include using a debugger and exception handling. 5. Performance optimization includes using StringBuilder and avoiding unnecessary packing and unboxing.

Yes, the DELETE statement can be used to clear a SQL table, the steps are as follows: Use the DELETE statement: DELETE FROM table_name; Replace table_name with the name of the table to be cleared.
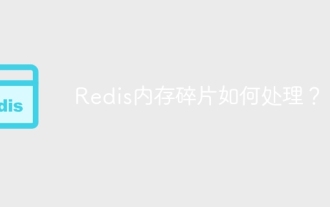
Redis memory fragmentation refers to the existence of small free areas in the allocated memory that cannot be reassigned. Coping strategies include: Restart Redis: completely clear the memory, but interrupt service. Optimize data structures: Use a structure that is more suitable for Redis to reduce the number of memory allocations and releases. Adjust configuration parameters: Use the policy to eliminate the least recently used key-value pairs. Use persistence mechanism: Back up data regularly and restart Redis to clean up fragments. Monitor memory usage: Discover problems in a timely manner and take measures.
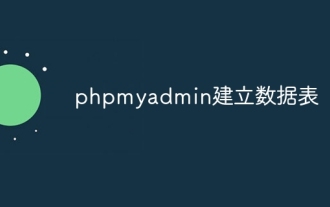
To create a data table using phpMyAdmin, the following steps are essential: Connect to the database and click the New tab. Name the table and select the storage engine (InnoDB recommended). Add column details by clicking the Add Column button, including column name, data type, whether to allow null values, and other properties. Select one or more columns as primary keys. Click the Save button to create tables and columns.
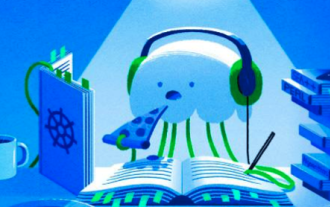
Effective monitoring of Redis databases is critical to maintaining optimal performance, identifying potential bottlenecks, and ensuring overall system reliability. Redis Exporter Service is a powerful utility designed to monitor Redis databases using Prometheus. This tutorial will guide you through the complete setup and configuration of Redis Exporter Service, ensuring you seamlessly build monitoring solutions. By studying this tutorial, you will achieve fully operational monitoring settings

Creating an Oracle database is not easy, you need to understand the underlying mechanism. 1. You need to understand the concepts of database and Oracle DBMS; 2. Master the core concepts such as SID, CDB (container database), PDB (pluggable database); 3. Use SQL*Plus to create CDB, and then create PDB, you need to specify parameters such as size, number of data files, and paths; 4. Advanced applications need to adjust the character set, memory and other parameters, and perform performance tuning; 5. Pay attention to disk space, permissions and parameter settings, and continuously monitor and optimize database performance. Only by mastering it skillfully requires continuous practice can you truly understand the creation and management of Oracle databases.
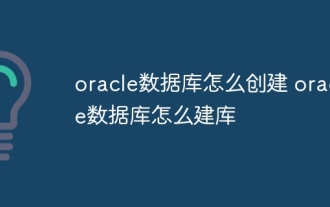
To create an Oracle database, the common method is to use the dbca graphical tool. The steps are as follows: 1. Use the dbca tool to set the dbName to specify the database name; 2. Set sysPassword and systemPassword to strong passwords; 3. Set characterSet and nationalCharacterSet to AL32UTF8; 4. Set memorySize and tablespaceSize to adjust according to actual needs; 5. Specify the logFile path. Advanced methods are created manually using SQL commands, but are more complex and prone to errors. Pay attention to password strength, character set selection, tablespace size and memory
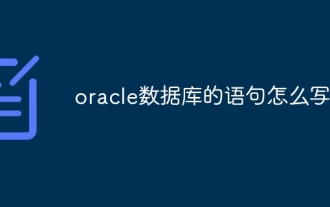
The core of Oracle SQL statements is SELECT, INSERT, UPDATE and DELETE, as well as the flexible application of various clauses. It is crucial to understand the execution mechanism behind the statement, such as index optimization. Advanced usages include subqueries, connection queries, analysis functions, and PL/SQL. Common errors include syntax errors, performance issues, and data consistency issues. Performance optimization best practices involve using appropriate indexes, avoiding SELECT *, optimizing WHERE clauses, and using bound variables. Mastering Oracle SQL requires practice, including code writing, debugging, thinking and understanding the underlying mechanisms.
