Data Structures and Algorithms in C : A Practical Implementation Guide
Implementing data structures and algorithms in C can be divided into the following steps: 1. Review the basic knowledge and understand the basic concepts of data structures and algorithms. 2. Implement basic data structures, such as arrays and linked lists. 3. Implement complex data structures, such as binary search trees. 4. Write common algorithms such as quick sorting and binary search. 5. Apply debugging skills to avoid common mistakes. 6. Perform performance optimization and select appropriate data structures and algorithms. Through these steps, you can build and apply data structures and algorithms from scratch to improve programming efficiency and problem-solving capabilities.
introduction
In the world of programming, data structures and algorithms are the core knowledge that every developer must master. They are not only hot topics during interviews, but also the basis for writing efficient and reliable code. Today, we will dive into how to implement these concepts in C and share some practical experiences and tips. Through this article, you will learn how to build common data structures and algorithms from scratch and learn how to apply them in real projects.
Review of basic knowledge
Before we begin our C journey, let’s review the basic concepts of data structures and algorithms. Data structures are the way to organize and store data, while algorithms are a series of steps to solve problems. As a powerful programming language, C provides a wealth of tools and libraries to implement these concepts.
Some basic data structures in C include arrays, linked lists, stacks, queues, trees and graphs, etc., while common algorithms cover sorting, searching, graph traversal, etc. Understanding these basic knowledge is the key to our further learning and realization.
Core concept or function analysis
Definition and function of data structure
Data structures are the cornerstone of programming, and they determine how data is organized and accessed in memory. Let's take an array as an example, an array is a linear data structure where elements are stored continuously in memory, which makes random access very efficient.
//Array example int arr[5] = {1, 2, 3, 4, 5}; std::cout << arr[2] << std::endl; // Output 3
How the algorithm works
Algorithms are specific steps to solve problems, and understanding how they work is crucial for optimization and debugging. Taking Quick Sort as an example, Quick Sort is used to select a benchmark value, divide the array into two parts, and then sort the two parts recursively.
// Quick sort example void quickSort(int arr[], int low, int high) { if (low < high) { int pi = partition(arr, low, high); quickSort(arr, low, pi - 1); quickSort(arr, pi 1, high); } } int partition(int arr[], int low, int high) { int pivot = arr[high]; int i = (low - 1); for (int j = low; j <= high - 1; j ) { if (arr[j] < pivot) { i ; std::swap(arr[i], arr[j]); } } std::swap(arr[i 1], arr[high]); return (i 1); }
The core of quick sorting is to select the appropriate benchmark value and efficient partitioning process, which makes its average time complexity O(n log n).
Example of usage
Basic usage
Let's see how to implement a simple linked list in C. A linked list is a dynamic data structure suitable for frequent insertion and deletion operations.
// Linked list node definition struct Node { int data; Node* next; Node(int val) : data(val), next(nullptr) {} }; // linked list class LinkedList { private: Node* head; public: LinkedList() : head(nullptr) {} void insert(int val) { Node* newNode = new Node(val); newNode->next = head; head = newNode; } void display() { Node* current = head; while (current != nullptr) { std::cout << current->data << " "; current = current->next; } std::cout << std::endl; } }; // Use example LinkedList list; list.insert(3); list.insert(2); list.insert(1); list.display(); // Output: 1 2 3
Advanced Usage
Now, let's implement a binary search tree (BST), a more complex data structure suitable for quick search and sorting.
// Binary search tree node definition struct TreeNode { int val; TreeNode* left; TreeNode* right; TreeNode(int x) : val(x), left(nullptr), right(nullptr) {} }; // BinarySearchTree { private: TreeNode* root; TreeNode* insertRecursive(TreeNode* node, int val) { if (node == nullptr) { return new TreeNode(val); } if (val < node->val) { node->left = insertRecursive(node->left, val); } else if (val > node->val) { node->right = insertRecursive(node->right, val); } return node; } void inorderTraversalRecursive(TreeNode* node) { if (node != nullptr) { inorderTraversalRecursive(node->left); std::cout << node->val << " "; inorderTraversalRecursive(node->right); } } public: BinarySearchTree() : root(nullptr) {} void insert(int val) { root = insertRecursive(root, val); } void inorderTraversal() { inorderTraversalRecursive(root); std::cout << std::endl; } }; // Use example BinarySearchTree bst; bst.insert(5); bst.insert(3); bst.insert(7); bst.insert(1); bst.insert(9); bst.inorderTraversal(); // Output: 1 3 5 7 9
Common Errors and Debugging Tips
Common errors include memory leaks, out-of-bounds access, and logical errors when implementing data structures and algorithms. Here are some debugging tips:
- Use smart pointers such as
std::unique_ptr
andstd::shared_ptr
) to manage memory and avoid memory leaks. - Write unit tests to verify the correctness of the code, especially the boundary situation.
- Use a debugger (such as GDB) to track program execution and find logical errors.
Performance optimization and best practices
Performance optimization and best practices are crucial in real-world projects. Here are some suggestions:
- Choose the right data structure and algorithm: For example, use a hash table for quick lookups and use a heap for priority queues.
- Time complexity of optimization algorithms: For example, dynamic programming is used to solve duplicate subproblems, and greedy algorithms are used to solve optimization problems.
- Improve code readability and maintainability: Use meaningful variable and function names, add comments and documentation, and follow the code style guide.
In terms of performance comparison, let's look at an example: suppose we need to find an element in a large array, the time complexity of linear search is O(n), and the time complexity of using binary search is O(log n). The following is the implementation of binary search:
// binary search example int binarySearch(int arr[], int left, int right, int x) { while (left <= right) { int mid = left (right - left) / 2; if (arr[mid] == x) { return mid; } if (arr[mid] < x) { left = mid 1; } else { right = mid - 1; } } return -1; // Not found} // Use example int arr[] = {2, 3, 4, 10, 40}; int n = sizeof(arr) / sizeof(arr[0]); int x = 10; int result = binarySearch(arr, 0, n - 1, x); (result == -1) ? std::cout << "Element is not present in array" : std::cout << "Element is present at index " << result;
By selecting the right algorithm, we can significantly improve the performance of the program.
In short, data structures and algorithms are the core of programming. Mastering them can not only help you write efficient code, but also improve your programming thinking and problem-solving ability. I hope this article can provide you with some practical guidance and inspiration for implementing data structures and algorithms in C.
The above is the detailed content of Data Structures and Algorithms in C : A Practical Implementation Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



01 Outlook Summary Currently, it is difficult to achieve an appropriate balance between detection efficiency and detection results. We have developed an enhanced YOLOv5 algorithm for target detection in high-resolution optical remote sensing images, using multi-layer feature pyramids, multi-detection head strategies and hybrid attention modules to improve the effect of the target detection network in optical remote sensing images. According to the SIMD data set, the mAP of the new algorithm is 2.2% better than YOLOv5 and 8.48% better than YOLOX, achieving a better balance between detection results and speed. 02 Background & Motivation With the rapid development of remote sensing technology, high-resolution optical remote sensing images have been used to describe many objects on the earth’s surface, including aircraft, cars, buildings, etc. Object detection in the interpretation of remote sensing images
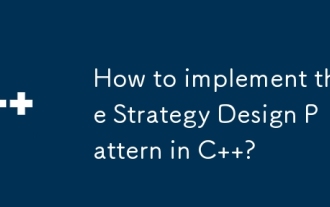
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
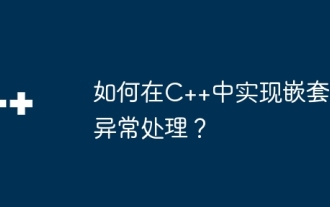
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
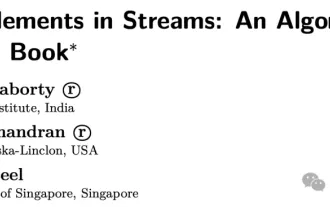
Counting sounds simple, but in practice it is very difficult. Imagine you are transported to a pristine rainforest to conduct a wildlife census. Whenever you see an animal, take a photo. Digital cameras only record the total number of animals tracked, but you are interested in the number of unique animals, but there is no statistics. So what's the best way to access this unique animal population? At this point, you must be saying, start counting now and finally compare each new species from the photo to the list. However, this common counting method is sometimes not suitable for information amounts up to billions of entries. Computer scientists from the Indian Statistical Institute, UNL, and the National University of Singapore have proposed a new algorithm - CVM. It can approximate the calculation of different items in a long list.
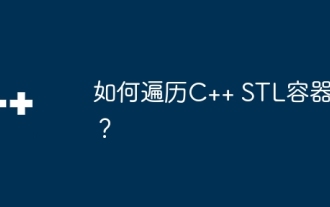
To iterate over an STL container, you can use the container's begin() and end() functions to get the iterator range: Vector: Use a for loop to iterate over the iterator range. Linked list: Use the next() member function to traverse the elements of the linked list. Mapping: Get the key-value iterator and use a for loop to traverse it.
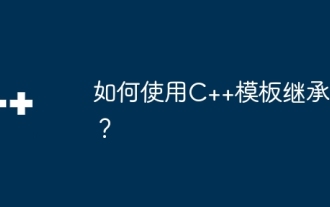
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.
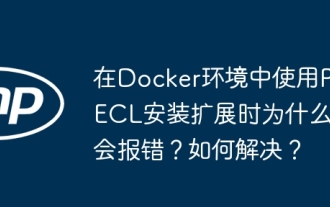
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
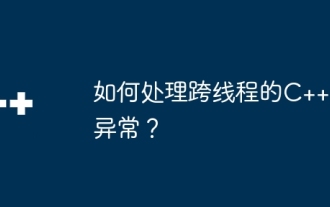
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
