How to define the call declaration format of c language function
C language functions include definitions, calls and declarations. Function definition specifies function name, parameters and return type, function body implements functions; function calls execute functions and provide parameters; function declaration tells the compiler the function type. Value pass is used for parameter pass, pay attention to the return type, maintain a consistent code style, and handle errors in functions. Mastering this knowledge can help write elegant, robust C code.
C language functions: things that define, call and declare
You must be thinking about what the definition, call and declaration of C language functions are, and how to write them to make the compiler obedient and the program not report errors? Don't worry, let's talk slowly. This article not only teaches you grammar, but also helps you understand the logic behind it and avoid those crazy pitfalls. After reading it, you can write elegant and efficient C functions instead of a bunch of headache-inducing code.
Let’s talk about the basics first. In C language, functions are the basic unit of organizing code, just like bricks that build blocks. You have to know how to define them and how to use them to build a beautiful "house".
Function definition is like giving shapes and functions to blocks. It contains function name, parameter list (input), return type (output), and function body (implementation).
<code class="c">// 函数定义:一个计算两个数平方和的函数int square_sum(int a, int b) { // 函数体:计算平方和int sum = a * ab * b; return sum; // 返回计算结果}</code>
Here, int square_sum(int a, int b)
is part of the function declaration, which tells the compiler: this function is called square_sum
, which receives two integers a
and b
as input and returns an integer. The function body is enclosed in curly braces {}
, which contains specific calculation logic.
Function calls are like using blocks of blocks you defined. You just need to provide the correct parameters and the function will execute and return the result.
<code class="c">#include <stdio.h> int main() { int x = 3, y = 4; int result = square_sum(x, y); // 调用square_sum函数printf("The square sum of %d and %d is: %d\n", x, y, result); // 打印结果return 0; }</stdio.h></code>
In the main
function, square_sum(x, y)
is a function call. The compiler will find the definition of the square_sum
function, execute the code inside, and assign the result result
. Note that the type of the parameter must match the parameter type in the function definition, otherwise the compiler will report an error.
Function declaration This is like putting a label on a building block and telling others what the building block does. Before calling a function, the compiler needs to know the return type and parameter type of the function, which is what the declaration does. If you do not declare it before calling the function and the compiler does not know what to do, an error will be reported. However, if the function definition is before being called, the declaration can be omitted.
<code class="c">// 函数声明int square_sum(int a, int b); int main() { // ... (调用square_sum函数的代码) ... } // 函数定义int square_sum(int a, int b) { // ... (函数体) ... }</code>
Here, int square_sum(int a, int b);
is the function declaration. It tells the compiler that the square_sum
function receives two integer parameters and returns an integer.
Some pitfalls and suggestions
- Parameter passing: C function parameter passing is value passing, that is, modifying the value of the parameter inside the function will not affect the value of the external variable. If you need to modify the value of an external variable, you can use a pointer.
- Return type: Be sure to pay attention to the return type of the function, especially the function of type void, which does not return any value. If you forget to write the return type, the compiler may return int by default, which may cause unexpected problems.
- Code style: Maintain the consistency of the code style, use meaningful variable names, and add necessary comments, which can improve the readability and maintainability of the code.
- Error handling: Handle possible errors in the function, such as invalid parameters, and return the corresponding error code or information.
In short, understanding function definitions, calls and declarations is the key to writing a good C program. By mastering this knowledge, you can write more elegant and robust C code. Don’t forget to practice more and practice more to truly master these skills. Remember, programming is a craft, and practice makes perfect!
The above is the detailed content of How to define the call declaration format of c language function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


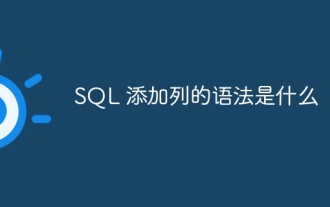
The syntax for adding columns in SQL is ALTER TABLE table_name ADD column_name data_type [NOT NULL] [DEFAULT default_value]; where table_name is the table name, column_name is the new column name, data_type is the data type, NOT NULL specifies whether null values are allowed, and DEFAULT default_value specifies the default value.
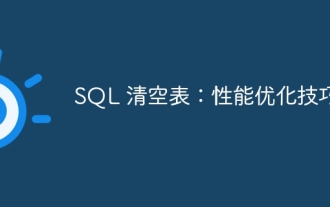
Tips to improve SQL table clearing performance: Use TRUNCATE TABLE instead of DELETE, free up space and reset the identity column. Disable foreign key constraints to prevent cascading deletion. Use transaction encapsulation operations to ensure data consistency. Batch delete big data and limit the number of rows through LIMIT. Rebuild the index after clearing to improve query efficiency.
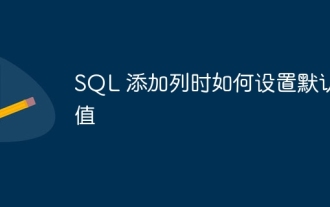
Set the default value for newly added columns, use the ALTER TABLE statement: Specify adding columns and set the default value: ALTER TABLE table_name ADD column_name data_type DEFAULT default_value; use the CONSTRAINT clause to specify the default value: ALTER TABLE table_name ADD COLUMN column_name data_type CONSTRAINT default_constraint DEFAULT default_value;

Yes, the DELETE statement can be used to clear a SQL table, the steps are as follows: Use the DELETE statement: DELETE FROM table_name; Replace table_name with the name of the table to be cleared.
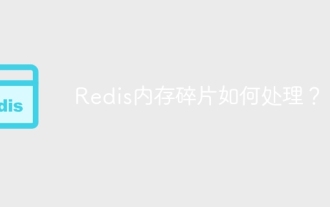
Redis memory fragmentation refers to the existence of small free areas in the allocated memory that cannot be reassigned. Coping strategies include: Restart Redis: completely clear the memory, but interrupt service. Optimize data structures: Use a structure that is more suitable for Redis to reduce the number of memory allocations and releases. Adjust configuration parameters: Use the policy to eliminate the least recently used key-value pairs. Use persistence mechanism: Back up data regularly and restart Redis to clean up fragments. Monitor memory usage: Discover problems in a timely manner and take measures.
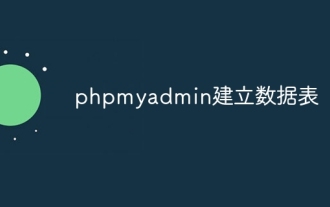
To create a data table using phpMyAdmin, the following steps are essential: Connect to the database and click the New tab. Name the table and select the storage engine (InnoDB recommended). Add column details by clicking the Add Column button, including column name, data type, whether to allow null values, and other properties. Select one or more columns as primary keys. Click the Save button to create tables and columns.

Creating an Oracle database is not easy, you need to understand the underlying mechanism. 1. You need to understand the concepts of database and Oracle DBMS; 2. Master the core concepts such as SID, CDB (container database), PDB (pluggable database); 3. Use SQL*Plus to create CDB, and then create PDB, you need to specify parameters such as size, number of data files, and paths; 4. Advanced applications need to adjust the character set, memory and other parameters, and perform performance tuning; 5. Pay attention to disk space, permissions and parameter settings, and continuously monitor and optimize database performance. Only by mastering it skillfully requires continuous practice can you truly understand the creation and management of Oracle databases.
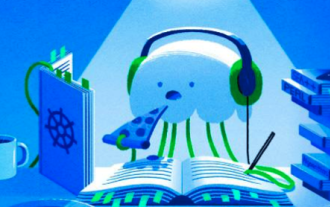
Effective monitoring of Redis databases is critical to maintaining optimal performance, identifying potential bottlenecks, and ensuring overall system reliability. Redis Exporter Service is a powerful utility designed to monitor Redis databases using Prometheus. This tutorial will guide you through the complete setup and configuration of Redis Exporter Service, ensuring you seamlessly build monitoring solutions. By studying this tutorial, you will achieve fully operational monitoring settings
