Building a Blog with Next.js
This article will guide you to build a static blog framework using Next.js, with design and structure inspired by Jekyll. Jekyll is known for its ease of access and high controllability, and this article aims to take advantage of Next.js and React to provide a new static blog building solution. Next.js is based on the file system and simplifies the construction process of static websites.
A typical Jekyll blog directory structure is as follows:
<code>. ├─── _posts/ ...Markdown 格式的博文├─── _layouts/ ...不同页面的布局├─── _includes/ ...可复用的组件├─── index.md ...主页└─── config.yml ...博客配置</code>
Our framework will follow this directory structure as much as possible to facilitate migration of blogs from Jekyll, simply reuse posts and configurations.
If you are not familiar with Jekyll, it is a static site generator that converts plain text into static websites and blogs. Please refer to the Jekyll Quick Start Guide for details.
This article assumes that you have the basics of React. If not, refer to the React Getting Started Guide.
Install
Next.js is based on React and written in Node.js. Therefore, you need to install npm before adding Next.js, React, and React-DOM.
<code>mkdir nextjs-blog && cd $_ npm init -y npm install next react react-dom --save</code>
In order to run the Next.js script on the command line, we need to add the next command to the scripts section of package.json.
<code>"scripts": { "dev": "next" }</code>
Now, run the npm run dev
command for the first time and see what happens.
<code>$ npm run dev > [email protected] dev /~user/nextjs-blog > next ready - started server on https://www.php.cn/link/4a914e5c38172ae9b61780ffbd0b2f90 Error: > Couldn't find a `pages` directory. Please create one under the project root</code>
The compiler prompts that the pages directory under the project root directory is missing. We will learn about the concept of pages in the next section.
pages concept
Next.js is built based on the pages concept. Each page is a React component (.js or .jsx) that is mapped to the route based on the file name. For example:
<code> 路由---- ----- /pages/about.js /about /pages/projects/work1.js /projects/work1 /pages/index.js /</code>
Let's create the pages directory in the project root directory and populate the first page index.js with the basic React component.
<code>// pages/index.js export default function Blog() { return</code> Welcome to Next.js blog }
Run npm run dev
again to start the server and visit https://www.php.cn/link/4a914e5c38172ae9b61780ffbd0b2f90 in your browser to view your blog.
Out of the box, we get:
- Hot reload, no need to refresh the browser for every code change.
- /pages/** Static generation of all pages in the directory.
- /public/** static file service for resources in the directory.
- 404 Error page.
Visit any path on localhost to see the actual effect of the 404 page. If you need to customize the 404 page, please refer to the Next.js documentation.
Dynamic page
Static routing pages are used to build home pages, about pages, etc. However, in order to build all blog posts dynamically, we will use Next.js' dynamic routing functionality. For example:
<code> 路由---- ----- /pages/posts/[slug].js /posts/1 /posts/abc /posts/hello-world</code>
Any routes, such as /posts/1, /posts/abc, etc., will match /posts/[slug].js, and the slug parameter will be sent to the page as a query parameter. This is especially useful for our blog posts, because we don't want to create a file for each blog post; instead, we can dynamically pass slugs to render the corresponding blog post.
(The following content is omitted. Because the length of the article is too long, the original text will remain unchanged, and the remaining parts will be simplified and the pictures will be retained)
Blog API, Includes, Layouts, Homepage, Blog Page, Production Environment Deployment, Improvement Suggestions
The subsequent part of the article details how to build a blog API (get all blog posts, get individual blog posts, and configure parsing), create reusable components (Includes), design page layout (Layouts), implement homepage blog post lists and individual blog post pages, as well as the final production environment deployment and some improvement suggestions, such as pagination, syntax highlighting, article classification labels and style beautification, etc. The implementation details of these parts are based on the features and functionality of Next.js and make full use of its static site generation capabilities.
The above is the detailed content of Building a Blog with Next.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


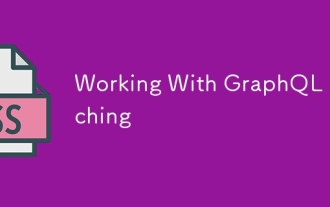
If you’ve recently started working with GraphQL, or reviewed its pros and cons, you’ve no doubt heard things like “GraphQL doesn’t support caching” or
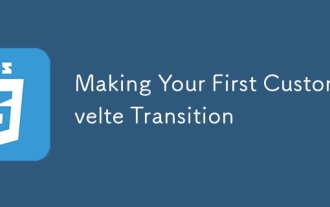
The Svelte transition API provides a way to animate components when they enter or leave the document, including custom Svelte transitions.
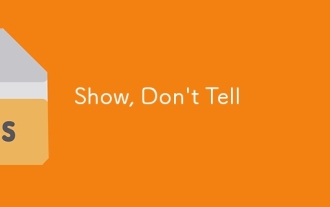
How much time do you spend designing the content presentation for your websites? When you write a new blog post or create a new page, are you thinking about
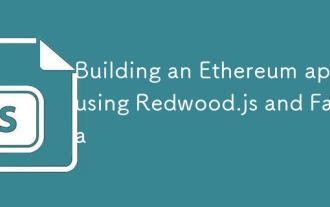
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
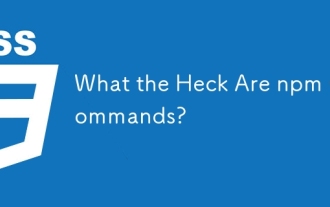
npm commands run various tasks for you, either as a one-off or a continuously running process for things like starting a server or compiling code.
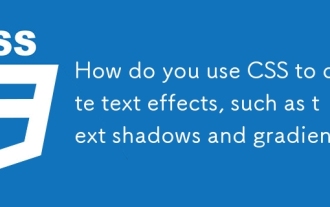
The article discusses using CSS for text effects like shadows and gradients, optimizing them for performance, and enhancing user experience. It also lists resources for beginners.(159 characters)
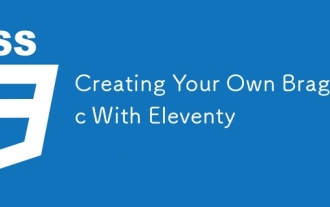
No matter what stage you’re at as a developer, the tasks we complete—whether big or small—make a huge impact in our personal and professional growth.
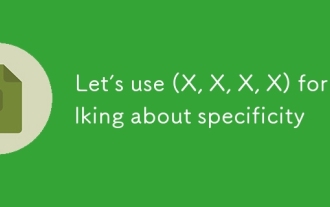
I was just chatting with Eric Meyer the other day and I remembered an Eric Meyer story from my formative years. I wrote a blog post about CSS specificity, and
