C language data structure: analysis of common interview questions
Data structures are key knowledge points in C language interviews: pointers and arrays: Understand pointers point to the array start address and are used to access and modify array elements. Linked List: Implement a one-way linked list to master the creation, insertion and deletion operations. Stack: Use arrays to build a stack, understand stack pressing, stacking and viewing stack top operations. Queue: Use arrays to implement queues to master the operations of joining, dequeuing and viewing team heads.
C Language Data Structure: Analysis of Common Interview Questions
In many programming interviews, data structures are inevitable topics. Mastering common data structures and their applications in C is crucial for job seekers.
1. Pointer and array
-
Understand the principle of pointers pointing to the starting address of an array.
int arr[] = {1, 2, 3, 4, 5}; int *ptr = arr; // Point to the first element of the array
Copy after login Use pointers to access and modify array elements.
printf("%d\n", *ptr); // Output 1 *ptr ; // Point to the next array element printf("%d\n", *ptr); // Output 2
Copy after login
2. Link list
Implement unidirectional linked lists and their basic operations (create, insert, delete).
struct node { int data; struct node *next; }; struct node *head = NULL; // header of linked list// create linked list void create_list(int data) { struct node *new_node = malloc(sizeof(struct node)); new_node->data = data; new_node->next = NULL; if (head == NULL) { head = new_node; } else { struct node *current = head; while (current->next != NULL) { current = current->next; } current->next = new_node; } } // Insert node to specific location of the linked list void insert_node(int data, int position) { struct node *new_node = malloc(sizeof(struct node)); new_node->data = data; if (position == 0) { new_node->next = head; head = new_node; } else { struct node *current = head; for (int i = 0; i < position - 1 && current != NULL; i ) { current = current->next; } if (current != NULL) { new_node->next = current->next; current->next = new_node; } } } // Delete node void delete_node(int position) { struct node *current = head; if (position == 0) { head = head->next; } else { for (int i = 0; i < position - 1 && current != NULL; i ) { current = current->next; } if (current != NULL && current->next != NULL) { struct node *temp = current->next; current->next = temp->next; free(temp); } } }
Copy after login
3. Stack
Implement the stack and use array simulation to understand the basic operations of the stack (press the stack, out the stack, view the top of the stack).
#define MAX_SIZE 100 int stack[MAX_SIZE]; int top = -1; // top pointer// stack push(int data) { if (top == MAX_SIZE - 1) { printf("Stack overflow\n"); } else { stack[top] = data; } } // Int pop() { if (top == -1) { printf("Stack underflow\n"); return -1; } else { return stack[top--]; } } // View the top element of the stack int peek() { if (top == -1) { printf("Empty stack\n"); return -1; } else { return stack[top]; } }
Copy after login
4. Queue
Use arrays to implement queues to understand the basic operations of queues (enter, dequeue, and view the team leader).
#define MAX_SIZE 100 int queue[MAX_SIZE]; int front = -1, rear = -1; // Enlist void enqueue(int data) { if ((front == 0 && rear == MAX_SIZE - 1) || (rear 1 == front)) { printf("Queue overflow\n"); } else if (front == -1) { front = rear = 0; queue[rear] = data; } else if (rear == MAX_SIZE - 1) { rear = 0; queue[rear] = data; } else { rear ; queue[rear] = data; } } // Dequeue int dequeue() { if (front == -1) { printf("Queue underflow\n"); return -1; } else if (front == rear) { int data = queue[front]; front = rear = -1; return data; } else { int data = queue[front]; front ; return data; } } // View the team's head element int peek() { if (front == -1) { printf("Queue empty\n"); return -1; } else { return queue[front]; } }
Copy after login
The above is the detailed content of C language data structure: analysis of common interview questions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
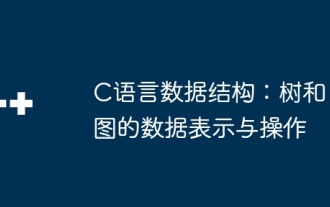
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
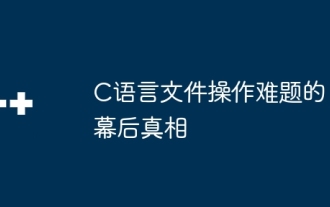
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
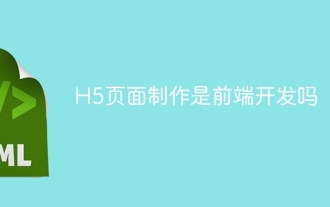
Yes, H5 page production is an important implementation method for front-end development, involving core technologies such as HTML, CSS and JavaScript. Developers build dynamic and powerful H5 pages by cleverly combining these technologies, such as using the <canvas> tag to draw graphics or using JavaScript to control interaction behavior.
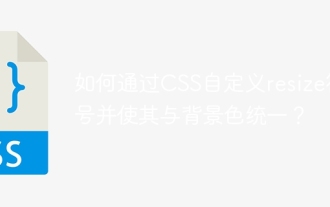
The method of customizing resize symbols in CSS is unified with background colors. In daily development, we often encounter situations where we need to customize user interface details, such as adjusting...
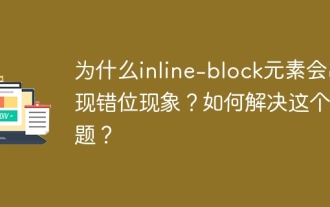
Regarding the reasons and solutions for misaligned display of inline-block elements. When writing web page layout, we often encounter some seemingly strange display problems. Compare...
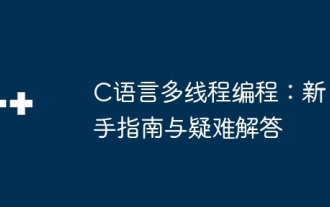
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
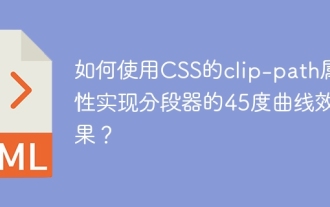
How to achieve the 45-degree curve effect of segmenter? In the process of implementing the segmenter, how to make the right border turn into a 45-degree curve when clicking the left button, and the point...
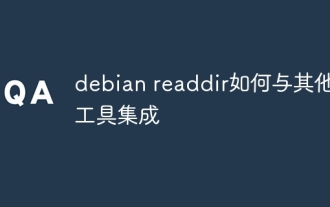
The readdir function in the Debian system is a system call used to read directory contents and is often used in C programming. This article will explain how to integrate readdir with other tools to enhance its functionality. Method 1: Combining C language program and pipeline First, write a C program to call the readdir function and output the result: #include#include#include#includeintmain(intargc,char*argv[]){DIR*dir;structdirent*entry;if(argc!=2){
