


When Vue and Mapbox combine Three.js, how do you ensure that the bottom of a 3D object is fixed on the map without changing with the perspective?
Implement perfect synchronization of 3D models and map viewing in Vue, Mapbox and Three.js
This article discusses how to combine Mapbox GL JS and Three.js in Vue.js application to achieve the effect of keeping the bottom fixed on the map when the map view angle changes. This is crucial for building 3D visualization applications based on geolocation.
Challenge: Change in perspective causes model shift
A common problem when overlaying Three.js models on Mapbox maps is that when you drag or zoom the map, the bottom of the model is offset instead of staying fixed on the map coordinates. This is different from using glTF models directly, which usually better maintain relative position to the map.
Solution: Accurate coordinate transformation and model positioning
Solving this problem requires precise coordinate transformation and model positioning strategies. The following steps and code examples will help you achieve your goals:
Correctness of coordinate system conversion: Make sure your coordinate conversion function (such as
calculatemodeltransform
) correctly converts the 3D model coordinates to the Mercator projection coordinate system used by Mapbox.Adjustment of model position and scaling: The key is to adjust the position and scaling of the Three.js model so that its bottom always corresponds to the map coordinates. The following code snippet shows how to modify the
createcustomlayer
function:
createCustomLayer(index, point, modelTransform) { let color; let altitude; // Set the color and height according to the PM2.5 value (assuming this logic already exists) // ... const customLayer = { id: `custom-layer-${index}`, type: 'custom', renderingMode: '3d', onAdd: (map, gl) => { console.log(`Custom layer added: ${customLayer.id}`); customLayer.camera = new THREE.Camera(); customLayer.scene = new THREE.Scene(); // Add light (ambient and directional light) // ... (assuming this logic already exists) const geometry = new THREE.BoxGeometry(20, altitude, 20); // Adjust the size const material = new THREE.MeshStandardMaterial({ color: color, transparent: true, opacity: 0.8 }); const cube = new THREE.Mesh(geometry, material); // Add contour lines (assuming this logic already exists) // ... // **Key steps: Adjust the position of the model so that the bottom of it is fixed to the map** cube.position.set(0, altitude / 2, 0); //The bottom is located at (0,0,0) customLayer.scene.add(cube); customLayer.map = map; customLayer.renderer = new THREE.WebGLRenderer({ canvas: map.getCanvas(), context: gl, antialias: true }); customLayer.renderer.autoClear = false; }, render: (gl, matrix) => { console.log(`Custom layer rendering: ${customLayer.id}`); const m = new THREE.Matrix4().fromArray(matrix); // ... (Computation of the model transformation matrix, assuming this logic already exists) customLayer.camera.projectionMatrix = m.multiply(l); customLayer.renderer.resetState(); customLayer.renderer.render(customLayer.scene, customLayer.camera); customLayer.map.triggerRepaint(); } }; return customLayer; }
In the code, cube.position.set(0, altitude / 2, 0);
make sure the bottom of the cube is at (0,0,0), and then adjust the height according to altitude
. This allows the bottom of the model to always align with the map coordinate system origin.
- Test and fine-tune: After completing the code modification, test your application and verify that the bottom of the model is always fixed on the map by dragging and zooming the map. It may need to be fine-tuned based on your specific coordinate transformation and model structure.
Through the above steps, you can effectively solve the problem of the Three.js model offset when the map view angle changes, thereby building a more stable and accurate three-dimensional geographic information visualization application. Remember, the key lies in precise coordinate transformation and relative position adjustment of the model.
The above is the detailed content of When Vue and Mapbox combine Three.js, how do you ensure that the bottom of a 3D object is fixed on the map without changing with the perspective?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


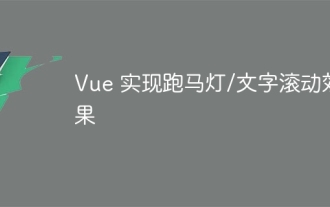
Implement marquee/text scrolling effects in Vue, using CSS animations or third-party libraries. This article introduces how to use CSS animation: create scroll text and wrap text with <div>. Define CSS animations and set overflow: hidden, width, and animation. Define keyframes, set transform: translateX() at the beginning and end of the animation. Adjust animation properties such as duration, scroll speed, and direction.
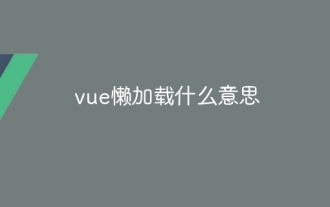
In Vue.js, lazy loading allows components or resources to be loaded dynamically as needed, reducing initial page loading time and improving performance. The specific implementation method includes using <keep-alive> and <component is> components. It should be noted that lazy loading can cause FOUC (splash screen) issues and should be used only for components that need lazy loading to avoid unnecessary performance overhead.
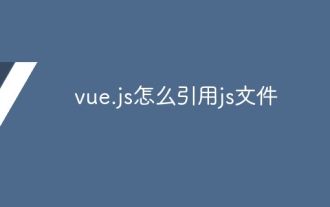
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
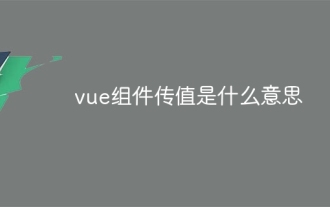
Vue component passing values is a mechanism for passing data and information between components. It can be implemented through properties (props) or events: Props: Declare the data to be received in the component and pass the data in the parent component. Events: Use the $emit method to trigger an event and listen to it in the parent component using the v-on directive.
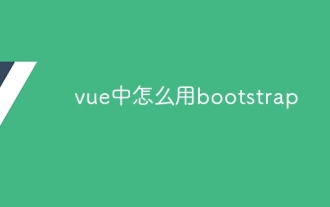
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
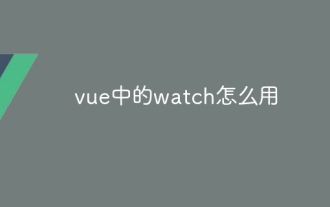
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
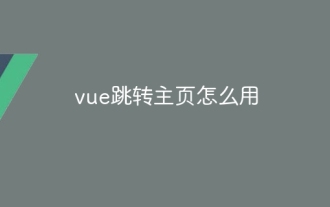
In Vue.js, you can jump to the home page through the router.push('/') method. The steps are as follows: introduce the Vue routing library; create a Vue router instance; configure the homepage routing; and jump to the homepage in the component.
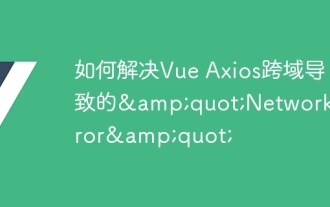
Methods to solve the cross-domain problem of Vue Axios include: Configuring the CORS header on the server side using the Axios proxy using JSONP using WebSocket using the CORS plug-in
