


How to merge array elements with the same ID into one object using JavaScript?
JavaScript array element merging: Merge elements with the same ID into one object
In data processing, it is often necessary to merge array elements with the same ID into a single object. This article provides a JavaScript solution to convert array elements with the same ID but different attributes into a new array format.
Original data format:
const list = [ { id: "202301", jine: 23, type: "Dinner" }, { id: "202301", jine: 87.5, type: "Breakfast" }, { id: "202301", jine: 1065.5, type: "Chinese food" }, { id: "202302", jine: 10, type: "Dinner" }, { id: "202302", jine: 181.5, type: "Breakfast" }, { id: "202302", jine: 633.5, type: "Chinese food" } ];
Target data format:
const targetList = [ { id: "202301", jine1: 87.5, jine2: 1065.5, jine3: 23 }, { id: "202302", jine1: 181.5, jine2: 633.5, jine3: 10 } ];
Solution:
We will use the reduce
method to implement data conversion. This method iterates over the array and accumulates each element into a result object.
const result = Object.values(list.reduce((acc, curr) => { const { id, jine, type } = curr; if (!acc[id]) { acc[id] = { id }; } switch (type) { case 'breakfast': acc[id].jine1 = jine; break; case 'Chinese food': acc[id].jine2 = jine; break; case 'dinner': acc[id].jine3 = jine; break; } return acc; }, {})); console.log(result); // Output target data format
Code explanation:
-
reduce
method: Thereduce
method iterates overlist
array and accumulates each element into an objectacc
. -
acc[id]
: Useid
as key to store data intoacc
object. Ifid
does not exist, a new object is created. -
switch
statement: Assign thejine
value tojine1
,jine2
orjine3
according to the value oftype
attribute. -
Object.values
: Finally, use theObject.values
method to convert theacc
object into an array to obtain the target data format.
This method is clear and concise, avoiding the use of external libraries, and directly using JavaScript built-in methods to implement data conversion. It's also easier to scale, if you need to deal with more types of dining, just add more case
in the switch
statement.
The above is the detailed content of How to merge array elements with the same ID into one object using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


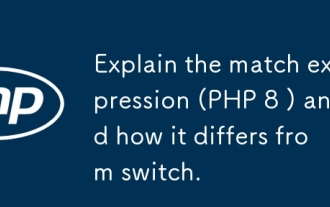
In PHP8, match expressions are a new control structure that returns different results based on the value of the expression. 1) It is similar to a switch statement, but returns a value instead of an execution statement block. 2) The match expression is strictly compared (===), which improves security. 3) It avoids possible break omissions in switch statements and enhances the simplicity and readability of the code.
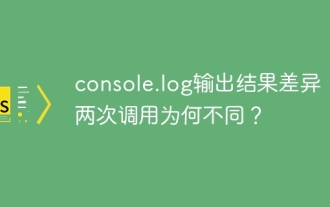
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
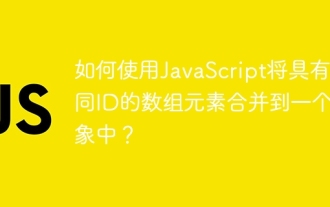
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
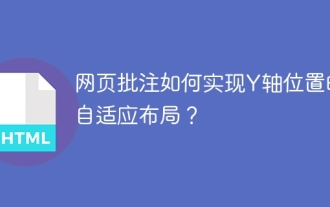
The Y-axis position adaptive algorithm for web annotation function This article will explore how to implement annotation functions similar to Word documents, especially how to deal with the interval between annotations...
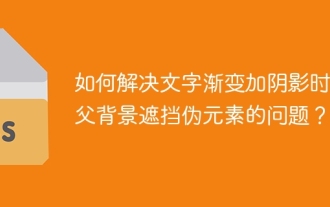
When adding shadows to text gradients, the solution to the grandfather background blocks pseudo-elements. When adding shadows to text gradients, pseudo-elements and absolute positioning are usually used to...
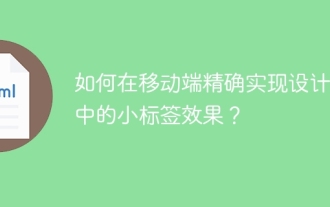
How to achieve the effect of small labels in the design draft on the mobile terminal? When designing mobile applications, it is common to find out how to accurately restore the small label effect in the design draft...
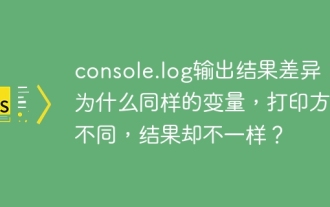
In-depth discussion of the differences in console.log output in this article will analyze the reasons why the output results of console.log function in a piece of code are different. Code snippets involve URL parameter resolution...
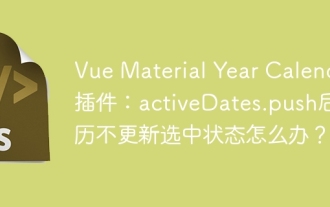
About VueMaterialYear...
