How to achieve segmentation effect with 45 degree curve border?
Create a 45-degree curve border segmenter: a clever combination of CSS and JavaScript
In modern user interface design, segmenters are a key navigation element to enhance user experience, especially in mobile applications and responsive websites. This article will demonstrate how to create a segmenter with a 45-degree angle curve border and dynamically switch the curve direction by clicking the button. This requires clever use of CSS' clip-path
attribute and JavaScript's interactive logic.
Steps detailed explanation
First, build the basic HTML structure:
<div class="wrap"> <div class="tabs"> <div class="tab" data-index="0">Tag 1</div> <div class="tab" data-index="1">Tag 2</div> </div> <div class="content-wrap"></div> </div>
Next, use CSS to define the style, clip-path
is the core:
.tabs { display: flex; width: 100%; overflow: hidden; border-radius: 8px 8px 0 0; /* top of rounded corners*/ background: linear-gradient(#cdd9fe, #e2e9fd); /* background gradient*/ } .tab { flex: 1 1 50%; /* Average distribution width*/ height: 50px; cursor: pointer; position: relative; text-align: center; line-height: 50px; transition: background-color 0.3s ease; /* Smooth transition*/ } .tab.active { background-color: #fff; color: #4185ef; } .tab.active::before { /* left curve*/ content: ''; position: absolute; top: 0; left: -50px; height: 100%; width: 50px; z-index: 2; background-color: #fff; clip-path: polygon(0 100%, 50% 100%, 50% 0, 100% 0); /* Simplify clip-path*/ } .tab.active::after { /* Right curve*/ content: ''; position: absolute; top: 0; right: -50px; height: 100%; width: 50px; z-index: 2; background-color: #fff; clip-path: polygon(0 0, 50% 0, 50% 100%, 100% 100%); /* Simplify clip-path */ }
In the code, we use the simpler polygon()
function to define clip-path
to make the code easier to read. The transition
property ensures a smooth transition of background colors.
Finally, JavaScript handles click events and activation status:
const tabs = document.querySelectorAll('.tab'); tabs.forEach(tab => { tab.addEventListener('click', () => { tabs.forEach(t => t.classList.remove('active')); tab.classList.add('active'); }); });
This JavaScript code adds a click event listener for each tag, removes the active
class of all tags when clicked, and then adds only active
classes for the clicked tags, thereby dynamically switching curve borders. You can use more complex JavaScript frameworks like Vue.js or React to manage state as needed, but for simple scenarios, this code is enough.
Through the above steps, a segmenter with a 45-degree curve border and dynamically responding to click events is completed. This design is both beautiful and practical, improving the user experience.
The above is the detailed content of How to achieve segmentation effect with 45 degree curve border?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


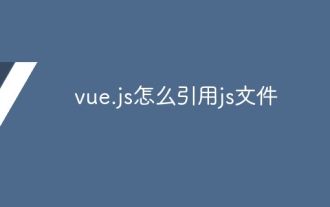
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
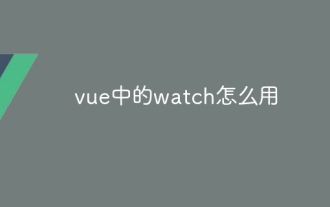
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
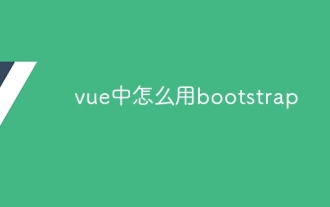
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
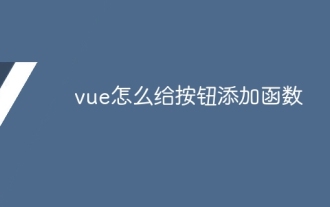
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
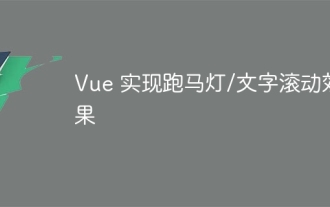
Implement marquee/text scrolling effects in Vue, using CSS animations or third-party libraries. This article introduces how to use CSS animation: create scroll text and wrap text with <div>. Define CSS animations and set overflow: hidden, width, and animation. Define keyframes, set transform: translateX() at the beginning and end of the animation. Adjust animation properties such as duration, scroll speed, and direction.
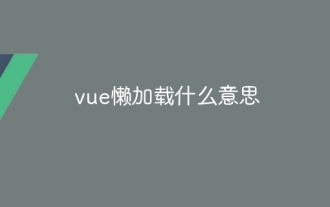
In Vue.js, lazy loading allows components or resources to be loaded dynamically as needed, reducing initial page loading time and improving performance. The specific implementation method includes using <keep-alive> and <component is> components. It should be noted that lazy loading can cause FOUC (splash screen) issues and should be used only for components that need lazy loading to avoid unnecessary performance overhead.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.
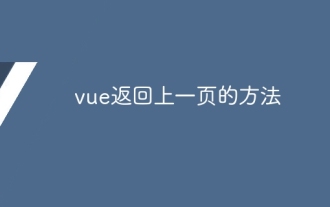
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.
