


From XML/RSS to JSON: Modern Data Transformation Strategies
Use Python to convert from XML/RSS to JSON. 1) parse source data, 2) extract fields, 3) convert to JSON, 4) output JSON. Use the xml.etree.ElementTree and feedparser libraries to parse XML/RSS, and use the json library to generate JSON data.
introduction
In today's data-driven world, conversion of data formats is becoming increasingly important. XML and RSS were once the standards for data exchange, but with the development of technology, JSON has gradually become the mainstream. So, how to convert from XML/RSS to JSON? This article will explore modern data transformation strategies to help you understand this process and provide practical code examples and experience sharing.
By reading this article, you will learn how to use Python for XML/RSS to JSON conversion, understand the problems you may encounter during the conversion process, and how to optimize the conversion process to improve efficiency.
Review of basic knowledge
XML (eXtensible Markup Language) and RSS (Really Simple Syndication) are common formats for early Internet data exchange. XML is known for its structure and scalability, while RSS is mainly used for content aggregation and subscription. In contrast, JSON (JavaScript Object Notation) has gradually become the first choice for modern APIs and data exchange due to its lightweight and easy to read and write.
In Python, we can use the xml.etree.ElementTree
module to parse XML files, use the feedparser
library to handle RSS feeds, and the json
module is used to generate JSON data.
Core concept or function analysis
Definition and function of XML/RSS to JSON conversion
XML/RSS to JSON conversion is essentially converting one data format to another to exchange data more efficiently between different systems or applications. JSON's simplicity and easy parsing properties make it more popular in modern web development.
For example, suppose we have an RSS feed that we can convert to JSON format to make it easier to handle in front-end applications:
import feedparser import json # parse RSS feed feed = feedparser.parse('https://example.com/rss') # Convert to JSON json_data = { 'title': feed.feed.title, 'entries': [{'title': entry.title, 'link': entry.link} for entry in feed.entries] } # Output JSON print(json.dumps(json_data, indent=2))
How it works
The conversion process usually includes the following steps:
- Parse source data : Use the appropriate library to parse XML or RSS data.
- Data extraction : Extract the required fields from the parsed data structure.
- Data conversion : Convert the extracted data to JSON format.
- Output JSON : Use
json.dumps()
method to serialize the data into a JSON string.
During the conversion process, it should be noted that the structure of XML and RSS can be very complex, so different tags and attributes need to be flexibly handled. In addition, the flattened structure of JSON may require special processing of nested data.
Example of usage
Basic usage
Let's look at a simple XML to JSON conversion example:
import xml.etree.ElementTree as ET import json # parse XML file tree = ET.parse('example.xml') root = tree.getroot() # Convert to JSON json_data = { 'root': { 'tag': root.tag, 'attributes': root.attrib, 'children': [ { 'tag': child.tag, 'attributes': child.attrib, 'text': child.text } for child in root ] } } # Output JSON print(json.dumps(json_data, indent=2))
This example shows how to convert a simple XML structure to JSON format. Each line of code has its specific function, for example, ET.parse()
is used to parse XML files, and json.dumps()
is used to convert Python dictionary to JSON strings.
Advanced Usage
When dealing with complex XML structures, we may need to recursively handle nested elements. Here is a more complex example:
import xml.etree.ElementTree as ET import json def xml_to_dict(element): result = {} result['tag'] = element.tag result['attributes'] = element.attrib if element.text and element.text.strip(): result['text'] = element.text.strip() children = list(element) if children: result['children'] = [xml_to_dict(child) for child in children] return result # parse XML file tree = ET.parse('complex_example.xml') root = tree.getroot() # Convert to JSON json_data = xml_to_dict(root) # Output JSON print(json.dumps(json_data, indent=2))
This example shows how to recursively process XML structures, convert them to JSON format. The recursive method xml_to_dict
can handle nested elements at any depth, making the conversion process more flexible and powerful.
Common Errors and Debugging Tips
Common errors during the conversion process include:
- Label or attribute loss : Make sure that no important tags or attributes are missing during the conversion process.
- Data type conversion error : For example, an error may occur when converting a string to a number, requiring type checking and conversion.
- Improper handling of nested structures : For complex nested structures, it is necessary to ensure that recursion is handled correctly.
Debugging skills include:
- Step by step debugging : Use the debugger to track the transformation process step by step to ensure that each step is executed correctly.
- Logging : Add logging in key steps to help track data flow and errors.
- Test cases : Write test cases to ensure that the conversion process works correctly under various inputs.
Performance optimization and best practices
In practical applications, it is very important to optimize the conversion process from XML/RSS to JSON. Here are some optimization strategies:
- Using efficient parsing libraries : For example, the
lxml
library is faster thanxml.etree.ElementTree
, which can significantly improve parsing speed. - Avoid unnecessary memory footprint : For large XML files, streaming parsing can be used to avoid loading the entire file into memory at once.
- Cache conversion results : If the conversion process occurs frequently, you can consider cache conversion results to reduce repeated calculations.
Compare performance differences between different methods, for example:
import time import xml.etree.ElementTree as ET from lxml import etree # Use xml.etree.ElementTree start_time = time.time() tree = ET.parse('large_example.xml') root = tree.getroot() end_time = time.time() print(f"xml.etree.ElementTree time: {end_time - start_time} seconds") # Use lxml start_time = time.time() tree = etree.parse('large_example.xml') root = tree.getroot() end_time = time.time() print(f"lxml time: {end_time - start_time} seconds")
This example shows the performance differences in parsing large XML files using different libraries. Through comparison, we can choose a more efficient analytical method.
In terms of programming habits and best practices, it is recommended:
- Code readability : Use meaningful variable names and comments to improve the readability of the code.
- Modularity : encapsulate conversion logic into functions or classes to improve the maintainability of the code.
- Error handling : Add appropriate error handling mechanisms to ensure the robustness of the conversion process.
Through these strategies and practices, you can perform XML/RSS to JSON more efficiently, improving the overall performance and reliability of data processing.
The above is the detailed content of From XML/RSS to JSON: Modern Data Transformation Strategies. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


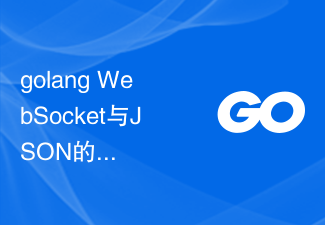
The combination of golangWebSocket and JSON: realizing data transmission and parsing In modern Web development, real-time data transmission is becoming more and more important. WebSocket is a protocol used to achieve two-way communication. Unlike the traditional HTTP request-response model, WebSocket allows the server to actively push data to the client. JSON (JavaScriptObjectNotation) is a lightweight format for data exchange that is concise and easy to read.
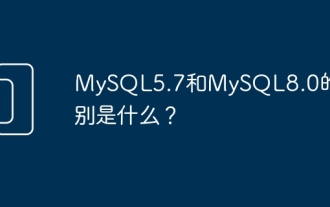
MySQL5.7 and MySQL8.0 are two different MySQL database versions. There are some main differences between them: Performance improvements: MySQL8.0 has some performance improvements compared to MySQL5.7. These include better query optimizers, more efficient query execution plan generation, better indexing algorithms and parallel queries, etc. These improvements can improve query performance and overall system performance. JSON support: MySQL 8.0 introduces native support for JSON data type, including storage, query and indexing of JSON data. This makes processing and manipulating JSON data in MySQL more convenient and efficient. Transaction features: MySQL8.0 introduces some new transaction features, such as atomic
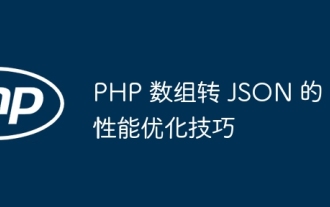
Performance optimization methods for converting PHP arrays to JSON include: using JSON extensions and the json_encode() function; adding the JSON_UNESCAPED_UNICODE option to avoid character escaping; using buffers to improve loop encoding performance; caching JSON encoding results; and considering using a third-party JSON encoding library.
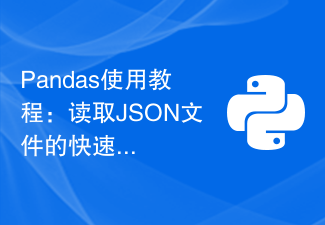
Quick Start: Pandas method of reading JSON files, specific code examples are required Introduction: In the field of data analysis and data science, Pandas is one of the important Python libraries. It provides rich functions and flexible data structures, and can easily process and analyze various data. In practical applications, we often encounter situations where we need to read JSON files. This article will introduce how to use Pandas to read JSON files, and attach specific code examples. 1. Installation of Pandas
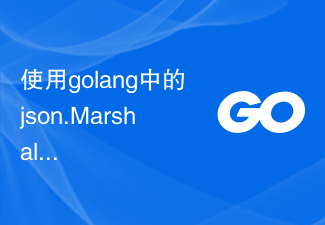
Use the json.MarshalIndent function in golang to convert the structure into a formatted JSON string. When writing programs in Golang, we often need to convert the structure into a JSON string. In this process, the json.MarshalIndent function can help us. Implement formatted output. Below we will explain in detail how to use this function and provide specific code examples. First, let's create a structure containing some data. The following is an indication
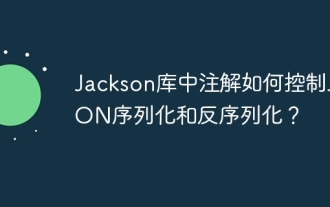
Annotations in the Jackson library control JSON serialization and deserialization: Serialization: @JsonIgnore: Ignore the property @JsonProperty: Specify the name @JsonGetter: Use the get method @JsonSetter: Use the set method Deserialization: @JsonIgnoreProperties: Ignore the property @ JsonProperty: Specify name @JsonCreator: Use constructor @JsonDeserialize: Custom logic
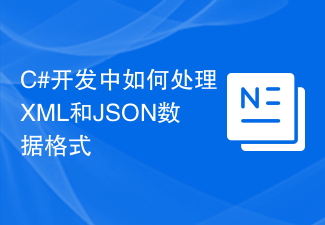
How to handle XML and JSON data formats in C# development requires specific code examples. In modern software development, XML and JSON are two widely used data formats. XML (Extensible Markup Language) is a markup language used to store and transmit data, while JSON (JavaScript Object Notation) is a lightweight data exchange format. In C# development, we often need to process and operate XML and JSON data. This article will focus on how to use C# to process these two data formats, and attach
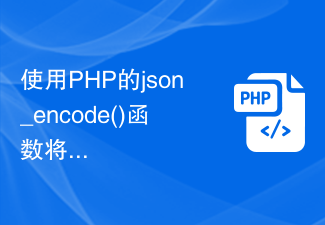
Using PHP's json_encode() function to convert an array or object into a JSON string and format the output can make it easier to transfer and exchange data between different platforms and languages. This article will introduce the basic usage of the json_encode() function and how to format and output a JSON string. 1. Basic usage of json_encode() function The basic syntax of json_encode() function is as follows: stringjson_encod
