


How to implement a single-table header and multi-body electronic quotation form in Vue and perform automatic calculation and summary?
Vue.js builds single-head multi-body electronic quotation form and automatic calculation summary
In modern business environments, electronic quotation forms are crucial, which can significantly improve efficiency and accuracy. This article will explain in detail how to use the Vue.js framework to build complex quotation forms with single headers and multiple table bodies, and realize automatic calculation and summary functions.
Requirements Analysis
The goal is to create a quote form with one header and multiple bodies. Each table body is a table whose row data calculates the recommended price based on the preset business logic and is automatically summarized to the total price of the table body. Unlike Excel, users cannot directly modify calculation formulas.
Implementation plan
We adopt the componentized development model of Vue.js, combine v-for
instructions to render tables dynamically, and use computational properties and methods to achieve automatic calculation and summary.
Data structure: Use an array to store the data of each table body. Each array element is an object that contains all rows of data of the table body.
Table rendering: Use Vue.js' template syntax to render the table header and table body.
v-for
instruction traverses the data array and dynamically generates each table body and its row. Consider using a custom component to encapsulate each watch body to improve code reusability.-
Recommended price calculation: define a function
calculateSuggestedPrice(item)
inmethods
of the Vue component to calculate the recommended price of each row based on the preset business logic. The parameteritem
of this function represents the data object of the current row.methods: { calculateSuggestedPrice(item) { // Calculate the suggested price based on actual business logic, for example: return item.quantity * item.unitPrice; } }
Copy after login -
Automatic summary: Use the computed attribute
computed
to calculate the total price of each body and the total of all body in real time.computed: { tableTotals() { return this.tableData.map(table => ({ total: table.reduce((sum, item) => sum this.calculateSuggestedPrice(item), 0) })); }, grandTotal() { return this.tableTotals.reduce((sum, table) => sum table.total, 0); } }
Copy after login User input limit: In order to prevent users from modifying the calculation logic, the calculation logic can be completely encapsulated inside the Vue component, allowing the user to enter only the original data (such as quantity, unit price, etc.). You can use
v-model
to bind data and add input verification as needed.
Through the above steps, a fully functional single-table multi-body electronic quotation form can be built in Vue.js to realize automatic calculation and summary, and effectively prevent users from misoperating or maliciously modifying calculation formulas. To enhance the user experience, consider adding data verification, form verification, and a more granular UI design.
The above is the detailed content of How to implement a single-table header and multi-body electronic quotation form in Vue and perform automatic calculation and summary?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


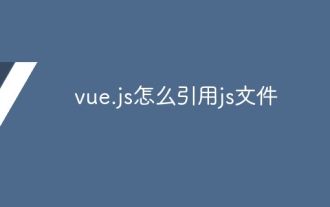
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
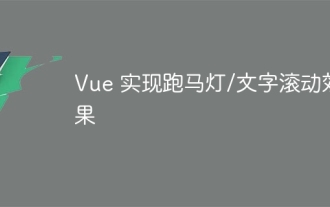
Implement marquee/text scrolling effects in Vue, using CSS animations or third-party libraries. This article introduces how to use CSS animation: create scroll text and wrap text with <div>. Define CSS animations and set overflow: hidden, width, and animation. Define keyframes, set transform: translateX() at the beginning and end of the animation. Adjust animation properties such as duration, scroll speed, and direction.
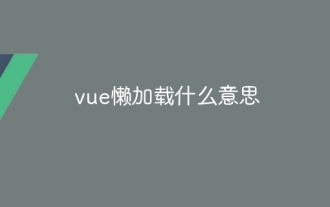
In Vue.js, lazy loading allows components or resources to be loaded dynamically as needed, reducing initial page loading time and improving performance. The specific implementation method includes using <keep-alive> and <component is> components. It should be noted that lazy loading can cause FOUC (splash screen) issues and should be used only for components that need lazy loading to avoid unnecessary performance overhead.
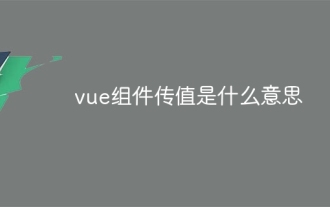
Vue component passing values is a mechanism for passing data and information between components. It can be implemented through properties (props) or events: Props: Declare the data to be received in the component and pass the data in the parent component. Events: Use the $emit method to trigger an event and listen to it in the parent component using the v-on directive.
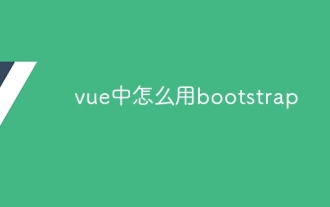
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
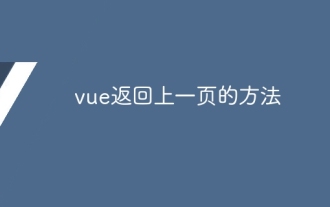
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.
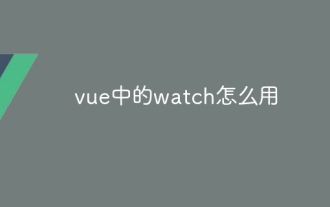
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
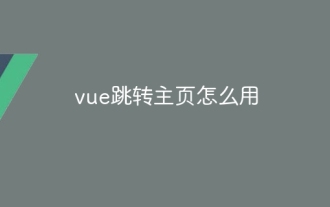
In Vue.js, you can jump to the home page through the router.push('/') method. The steps are as follows: introduce the Vue routing library; create a Vue router instance; configure the homepage routing; and jump to the homepage in the component.
