Easing Animations in Canvas
HTML5<canvas></canvas>
The elements combine with JavaScript's Canvas API to form one of the main raster graphics and animation functions on web pages. A common use case for Canvas is to generate images programmatically for websites (especially games). A card solitaire game website I built does exactly this, where all the cards and their movements are done using canvas.
This article will focus on animation in canvas and techniques to make animations look smoother. We will focus on easing transitions (such as "ease-in" and "ease-out") that are not available for free in canvas as in CSS.
Let's start with a static canvas. I've drawn a playing card from the DOM on canvas:
Let's start with a basic animation: move that card on canvas. Even a very basic thing, it needs to work from scratch in canvas, so we have to start building functions that we can use.
First, we will create some functions to help calculate X and Y coordinates:
function getX(params) { let distance = params.xTo - params.xFrom; let steps = params.frames; let progress = params.frame; return distance / steps * progress; } function getY(params) { let distance = params.yTo - params.yFrom; let steps = params.frames; let progress = params.frame; return distance / steps * progress; }
This will help us update the position value when the image is animate. We will then keep re-rendering the canvas until the animation is complete. We do this by adding the following code to our addImage()
method.
// ... (Omit some code) ... if (params.frame <p> Now we have animation! We are increasing 1 unit stably at each time, which we call <em>linear</em> animation.</p><p> You can see how the shape moves from point A to point B in a linear manner, maintaining the same constant velocity between the two points. It's fully functional, but lacks realism. The start and stop are abrupt.</p><p> What we want is the object to accelerate (ease-in) and deceleration (ease-out) so that it can simulate the movement of objects in the real world under the action of friction and gravity.</p><p></p><h2 id="JavaScript-easing-function"> JavaScript easing function</h2><p> We will use the "three times" ease-in and ease-out transitions to achieve this. We modified one of the equations found in Robert Penner's Flash easing function to fit what we want to do here.</p><pre class="brush:php;toolbar:false"> function getEase(currentProgress, start, distance, steps) { currentProgress /= steps/2; if (currentProgress <p> Insert it into our code (this is a <strong>three-easing</strong> ) and we will get a smoother result. Note how the card accelerates toward the center of the space and then slows down when it reaches the end.</p><p></p><h2 id="Advanced-easing-using-JavaScript"> Advanced easing using JavaScript</h2><p> We can use secondary or sinusoidal easing to get slower acceleration.</p><pre class="brush:php;toolbar:false"> function getQuadraticEase(currentProgress, start, distance, steps) { currentProgress /= steps/2; if (currentProgress <p> For faster acceleration, five or exponential easing can be used:</p><pre class="brush:php;toolbar:false"> function getQuinticEase(currentProgress, start, distance, steps) { currentProgress /= steps/2; if (currentProgress <p></p><h3 id="Use-GSAP-to-implement-more-complex-animations"> Use GSAP to implement more complex animations</h3><p> It's fun to write easing functions yourself, but what if you want more power and flexibility? You can go ahead and write custom code, or consider using more powerful libraries. Let's turn to GreenSock Animation Platform (GSAP) for this.</p><p> Using GSAP, the implementation of animations becomes much easier. Take this example as an example, the card will rebound at the end. Note that the GSAP library is included in the demo.</p><p> The key function is <code>moveCard</code> :</p><pre class="brush:php;toolbar:false"> function moveCard() { gsap.to(position, { duration: 2, ease: "bounce.out", x: position.xMax, y: position.yMax, onUpdate: function() { draw(); }, onComplete: function() { position.x = position.origX; position.y = position.origY; } }); }
The gsap.to
method is where all magic happens. For a duration of two seconds, the position object is updated and onUpdate
is called each time it is updated, triggering the redraw of canvas.
And we're not just talking about rebound. There are a large number of different easing options to choose from.
Summarize
Still not sure which animation style and method to use in canvas for easing? Here is an example demonstrating different easing animations, including those provided in GSAP.
Check out my Solitaire Game to see a live demo of non-GSAP animations. In this case, I have added animations so that the cards in the game ease-out and ease-in as they move between the heaps.
In addition to creating motion, the easing function can be applied to any other properties with a start and end state, such as changes in opacity, rotation, and scaling. I hope you can find many ways to use easing functions to make your application or game look smoother.
The above is the detailed content of Easing Animations in Canvas. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


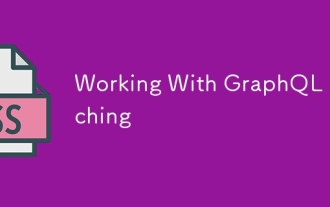
If you’ve recently started working with GraphQL, or reviewed its pros and cons, you’ve no doubt heard things like “GraphQL doesn’t support caching” or
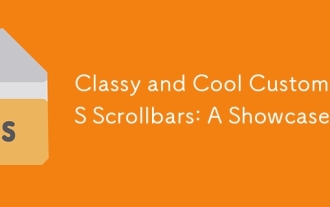
In this article we will be diving into the world of scrollbars. I know, it doesn’t sound too glamorous, but trust me, a well-designed page goes hand-in-hand
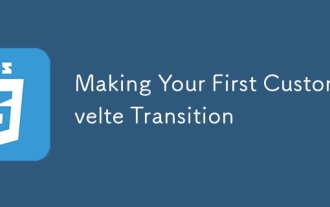
The Svelte transition API provides a way to animate components when they enter or leave the document, including custom Svelte transitions.
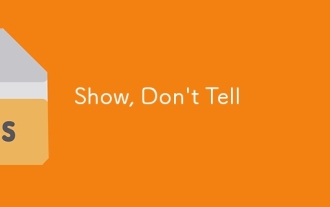
How much time do you spend designing the content presentation for your websites? When you write a new blog post or create a new page, are you thinking about
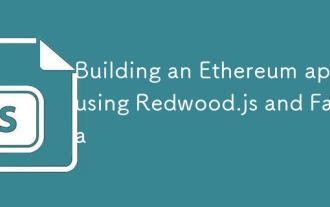
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
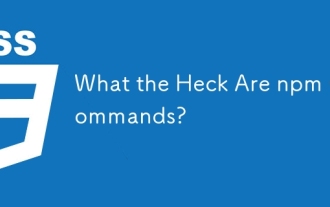
npm commands run various tasks for you, either as a one-off or a continuously running process for things like starting a server or compiling code.
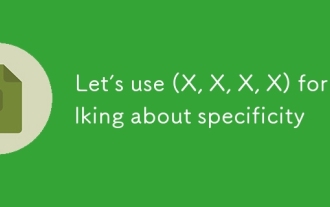
I was just chatting with Eric Meyer the other day and I remembered an Eric Meyer story from my formative years. I wrote a blog post about CSS specificity, and
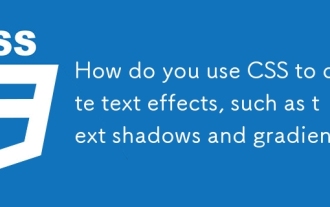
The article discusses using CSS for text effects like shadows and gradients, optimizing them for performance, and enhancing user experience. It also lists resources for beginners.(159 characters)
