Jumping Into Webmentions With NextJS (or Not)
Webmention, a W3C recommendation last updated January 12, 2017, is a simple mechanism for notifying a URL when it's mentioned on another site. Conversely, it allows a site to receive notifications when mentioned elsewhere. Essentially, it transforms your website into an active social media hub, facilitating communication from various platforms (Twitter, Instagram, Mastodon, etc.).
Implementing Webmentions varies; WordPress users can easily install plugins, while others may require more manual configuration. Let's explore the process.
Implementation Plan
- Establish a Webmention endpoint.
- Transform social media interactions into Webmentions.
- Integrate mentions into your website/app.
- Configure outbound Webmentions.
Fortunately, several services simplify this process. The third step requires more effort, but I'll detail my approach on atila.io.
My NextJS-based, server-side rendered blog uses client-side Webmention requests, easily adaptable to other React apps and JavaScript applications.
Step 1: Defining the Webmention Endpoint
To receive Webmentions, you can either create a custom script or use a service like Webmention.io (my chosen method).
Webmention.io is free; you only need to verify domain ownership. I used a rel="me"
attribute linking my website to my social media profiles. A single link suffices, but I included all my accounts.
<a href="https://www.php.cn/link/069802d489a7b73dd31fd13b0f2cd690" rel="me noopener noreferrer" target="_blank"> @AtilaFassina </a>
This requires a reciprocal link from my Twitter profile back to my website. After verification on Webmention.io, add your website URL. This provides your Webmention endpoint. Include these <link>
tags in your webpage's to collect mentions:
<link href="https://webmention.io/%7Buser%7D/webmention" rel="webmention"> <link href="https://webmention.io/%7Buser%7D/xmlrpc" rel="pingback">
Replace {user}
with your Webmention.io username.
Step 2: Processing Social Media Interactions
While Webmentions are ready, their usage is limited. Converting social media interactions is crucial. Bridgy, a free service, connects syndicated content and transforms it into Webmentions. Using SSO, connect your various profiles.
Step 3: Displaying Webmentions on Your Site
This step involves retrieving and displaying Webmention data. We'll break it down into stages: counting mentions, fetching mentions, and integrating with NextJS (optional).
Counting Webmentions
The Webmention.io API provides a count of mentions. The response is structured as follows:
type TMentionsCountResponse = { count: number; type: { like: number; mention: number; reply: number; repost: number; }; };
This is processed to:
type TMentionsCount = { mentions: number; likes: number; total: number; };
The endpoint is: https://webmention.io/api/count.json?target=${post_url}
. Max Böck and Swyx combine likes with reposts and mentions with replies.
const getMentionsCount = async (postURL: string): Promise<tmentionscount> => { const resp = await fetch(`https://webmention.io/api/count.json?target=${postURL}/`); const { type, count } = await resp.json(); return { likes: type.like type.repost, mentions: type.mention type.reply, total: count, }; };</tmentionscount>
Fetching Webmentions
The https://webmention.io/api/mentions
endpoint is paginated, accepting page
, per-page
, and target
parameters. A successful response includes a links
array of mentions:
type TMention = { source: string; verified: boolean; verified_date: string; id: number; private: boolean; data: { author: { name: string; url: string; photo: string; }; url: string; name: string; content: string; published: string; published_ts: number; }; activity: { type: 'link' | 'reply' | 'repost' | 'like'; sentence: string; sentence_html: string; }; target: string; };
The fetch request:
const getMentions = async ( page: string, postsPerPage: number, postURL: string ): Promise => { const resp = await fetch( `https://webmention.io/api/mentions?page=${page}&per-page=${postsPerPage}&target=${postURL}` ); const list = await resp.json(); return list.links; };
NextJS Integration (Optional)
NextJS offers getStaticProps
, getStaticPaths
, and getServerSideProps
for data fetching. I chose a client-side approach using SWR for caching and state management.
Displaying the Webmention Count
A MentionsCounter
component displays the count:
// ... (import statements and types) ... const MentionsCounter = ({ postUrl }) => { const { t } = useTranslation(); const { data = {}, error } = useSWR(postUrl, getMentionsCount); if (error) { return <errormessage>{t('common:errorWebmentions')}</errormessage>; } const { likes = '-', mentions = '-' } = data; return ( <mentioncounter> <li> <heart title="Likes"></heart> <counterdata>{Number.isNaN(likes) ? 0 : likes}</counterdata> </li> <li> <comment title="Mentions"></comment> <counterdata>{Number.isNaN(mentions) ? 0 : mentions}</counterdata> </li> </mentioncounter> ); };
Displaying the Mentions
A Webmentions
component displays the actual mentions, using state for pagination and useEffect
for fetching:
// ... (import statements and types) ... const Webmentions = ({ postUrl }) => { const { t } = useTranslation(); const [page, setPage] = useState(0); const [mentions, addMentions] = useState([]); useEffect(() => { const fetchMentions = async () => { const olderMentions = await getMentions(page, 50, postUrl); addMentions((mentions) => [...mentions, ...olderMentions]); }; fetchMentions(); }, [page]); return ( {mentions.map((mention, index) => ( <mention key="{mention.data.author.name}"> <authoravatar lazy src="%7Bmention.data.author.photo%7D"></authoravatar> <mentioncontent> <mentiontext activity="{mention.activity.type}" data="{mention.data}"></mentiontext> </mentioncontent> </mention> ))} {mentions.length > 0 && ( <morebutton onclick="{()"> setPage(page 1)} type="button"> {t('common:more')} </morebutton> )} > ); };
Step 4: Handling Outbound Mentions
Webmention.app, with an API token and webhook, simplifies outbound mentions. Alternatively, Remy Sharp's wm
CLI package can be used as a post-build script. Microformats (h-entry and h-card) are essential for richer outbound mentions.
Conclusion
This guide provides a comprehensive overview of Webmention implementation. Remember to share this post if it was helpful!
References
- Using Web Mentions on Static Sites (Max Böck)
- Client-side Webmentions (Swyx)
- Send outgoing Webmentions (Remy Sharp)
- Your first webmention (Aaron Parecki)
Further Reading
- Webmention W3C Specification (Recommendation)
- Webmention.io
- Webmention.App
- Outbound WebMentions CLI
- Bridgy
- Microformats.org
- IndieWeb
The above is the detailed content of Jumping Into Webmentions With NextJS (or Not). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


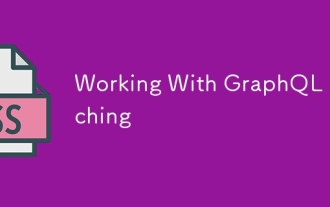
If you’ve recently started working with GraphQL, or reviewed its pros and cons, you’ve no doubt heard things like “GraphQL doesn’t support caching” or
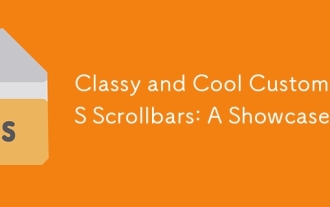
In this article we will be diving into the world of scrollbars. I know, it doesn’t sound too glamorous, but trust me, a well-designed page goes hand-in-hand
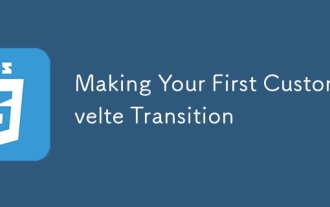
The Svelte transition API provides a way to animate components when they enter or leave the document, including custom Svelte transitions.
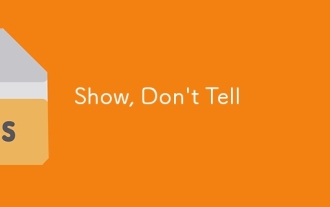
How much time do you spend designing the content presentation for your websites? When you write a new blog post or create a new page, are you thinking about
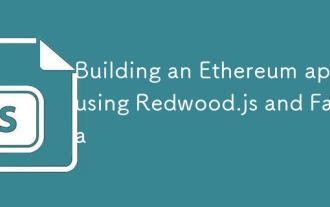
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
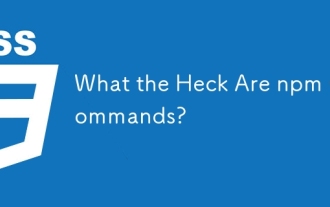
npm commands run various tasks for you, either as a one-off or a continuously running process for things like starting a server or compiling code.
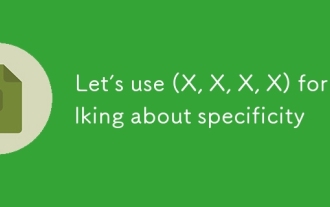
I was just chatting with Eric Meyer the other day and I remembered an Eric Meyer story from my formative years. I wrote a blog post about CSS specificity, and
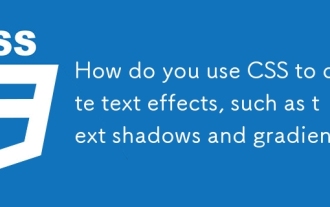
The article discusses using CSS for text effects like shadows and gradients, optimizing them for performance, and enhancing user experience. It also lists resources for beginners.(159 characters)
