What is a composer used for?
Composer is a dependency management tool for PHP. The core steps of using Composer include: 1) Declare dependencies in composer.json, such as "stripe/stripe-php": "^7.0"; 2) Run composer install to download and configure dependencies; 3) Manage versions and autoloads through composer.lock and autoload.php. Composer simplifies dependency management and improves project efficiency and maintainability.
introduction
In modern web development, managing project dependencies is an inevitable and crucial topic. Today, I want to talk to you about Composer, a dependency management tool designed specifically for PHP. Why do we need it? Because during the development process, dependency management is not only convenient, but also the key to improving project maintainability and efficiency. Through this article, you will learn about the core functions of Composer, how to use it, as well as my experience and experience using it in actual projects.
What is Composer?
Simply put, Composer is the package manager of the PHP world. It allows us to easily declare, install and update the libraries or packages required for our project. Imagine you are developing an e-commerce website that needs a library of payment gateways. Without Composer, you may need to manually download, decompress, and configure these libraries, which is not only cumbersome, but also prone to errors. Composer helps us automate these steps through a simple configuration file composer.json
.
For example, here is a simple composer.json
file:
{ "require": { "stripe/stripe-php": "^7.0" } }
Simply run composer install
and Stripe's payment library will be automatically downloaded and configured into your project.
How Composer works
When you run composer install
or composer update
, Composer does the following:
- Resolve dependencies : It will read
composer.json
and parse out the package you need and its version requirements. - Download packages : Download these packages from Packagist (Composer's central repository) or other designated repository based on the parsed results.
- Automatic loading : generate a
composer.lock
file, lock the current dependent version, and generateautoload.php
, so that you can use these libraries directly in your code.
This sounds simple, but in reality, Composer has complex algorithms for dealing with dependency conflicts and version management. For example, if package A depends on version 1.0 of package B and package C depends on version 2.0 of package B, Composer will try to find a compatible solution.
Example using Composer
Basic usage
Suppose you want to use Monolog, a popular log library in your project. You just need to add the following in composer.json
:
{ "require": { "monolog/monolog": "^2.0" } }
Then run composer install
and Monolog will be installed into your project. You can use it like this in your code:
<?php require 'vendor/autoload.php'; use Monolog\Logger; use Monolog\Handler\StreamHandler; $log = new Logger('name'); $log->pushHandler(new StreamHandler('app.log', Logger::WARNING)); $log->warning('Foo'); $log->error('Bar');
Advanced Usage
In more complex projects, you may need to customize the installation location of the package, or use a private repository. Here is an example of using a private Git repository:
{ "repositories": [ { "type": "vcs", "url": "git@github.com:yourorg/your-private-repo.git" } ], "require": { "yourorg/your-private-repo": "dev-master" } }
This way, you can easily integrate private libraries into your project.
Common Errors and Debugging Tips
Common problems when using Composer include dependency conflicts and version incompatibility. For example, if you encounter Your requirements could not be resolved to an installable set of packages.
Such errors are usually due to dependency version conflicts. At this time, you can try the following methods:
- Check
composer.lock
file : Make sure all developers use the same version. - Use
composer why-not
: This command can help you find out why some packages cannot be installed. - Adjust version requirements : Adjust the version range in
composer.json
, for example, change from^1.0
to~1.0
.
Performance optimization and best practices
In actual projects, there are a few points to note when using Composer:
- Lock version : Use the
composer.lock
file to ensure that all developers use the same dependency version to avoid problems caused by different versions. - Optimize automatic loading : Use
composer dump-autoload -o
to optimize automatic loading, which can reduce the startup time of the project. - Separate the development and production environment : Use
composer install --dev
in the development environment andcomposer install --no-dev
in the production environment to reduce the dependencies of the production environment.
In addition, there are some best practices that can improve the readability and maintenance of your code:
- Keep
composer.json
concise : only contain necessary dependencies and avoid unnecessary packages. - Regularly update dependencies : Use
composer outdated
to check whether the dependencies are updated and update them in time to avoid security vulnerabilities.
In general, Composer is not just a tool, but also a philosophy of managing project dependencies. By using it reasonably, you can greatly improve development efficiency and reduce confusion and mistakes in your project. I hope this article helps you better understand and use Composer to maximize its value in your project.
The above is the detailed content of What is a composer used for?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


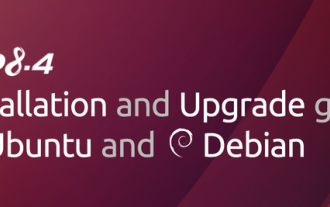
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
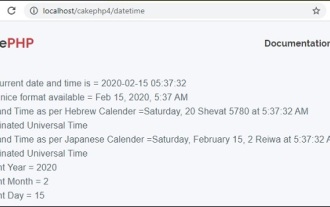
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
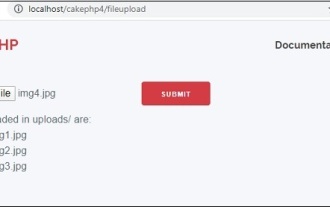
To work on file upload we are going to use the form helper. Here, is an example for file upload.
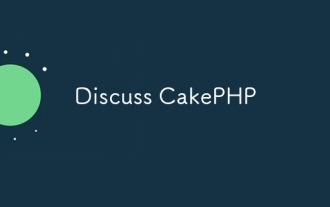
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
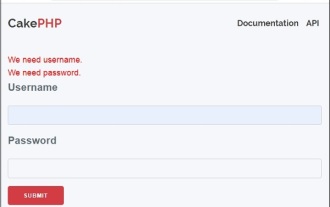
Validator can be created by adding the following two lines in the controller.
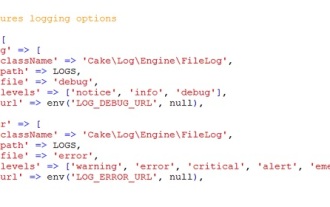
Logging in CakePHP is a very easy task. You just have to use one function. You can log errors, exceptions, user activities, action taken by users, for any background process like cronjob. Logging data in CakePHP is easy. The log() function is provide
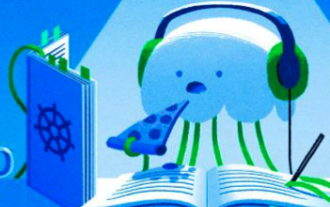
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
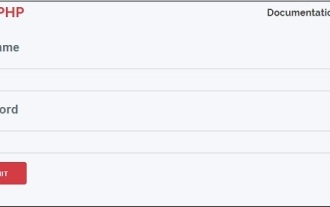
This chapter deals with the information about the authentication process available in CakePHP.
