


How does session hijacking work and how can you mitigate it in PHP?
Session hijacking can be achieved through the following steps: 1. Get the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted over HTTPS.
introduction
In the field of cybersecurity, session hijacking is a headache, which not only threatens user privacy, but can also lead to serious security vulnerabilities. Today we will dive into how session hijacking works and how to effectively prevent such attacks in PHP. Through this article, you will learn about the specific implementation of session hijacking, as well as some practical protection strategies and code examples.
Review of basic knowledge
The core of session hijacking is that the attacker acquires and utilizes the user's session ID (Session ID). In PHP, session management is implemented through the $_SESSION
hyperglobal variable, which allows developers to store and access data between different requests of users. The session ID is usually stored in a cookie or passed through a URL parameter.
Common methods of session hijacking include stealing cookies, man-in-the-middle attacks (MITM), XSS attacks, etc. Understanding these attack methods is the first step in preventing session hijacking.
Core concept or function analysis
The definition and function of session hijacking
Session hijacking refers to an attacker obtaining the user's session ID through illegal means, thereby impersonating the user for operations. The harm of this kind of attack lies in the fact that the attacker can access the user's sensitive information and even perform malicious operations.
The advantage of session hijacking lies in its concealment and efficiency. Attackers do not need to crack the user's password, but only need to obtain the session ID to achieve the attack.
How session hijacking works
The implementation of session hijacking usually includes the following steps:
Get session ID : The attacker obtains the user's session ID through various means, such as injecting malicious scripts into stealing cookies through XSS attacks, or intercepting network traffic through man-in-the-middle attacks.
Use Session ID : Once the session ID is obtained, an attacker can use this ID to access the victim's account and perform various operations.
Keep the session active : To extend the time of session hijacking, an attacker may regularly access the victim’s account through automation tools to keep the session active.
Example
Here is a simple PHP code example showing how to get and use the session ID:
<?php session_start(); // Get the session ID $sessionId = session_id(); // Use session ID echo "Current Session ID: " . $sessionId; //Storage some data into the session $_SESSION['username'] = 'exampleUser'; // Access session data echo "username: " . $_SESSION['username']; ?>
Example of usage
Basic usage
In PHP, basic session management can be implemented through the following code:
<?php session_start(); // Set session data $_SESSION['user_id'] = 123; // Access session data if (isset($_SESSION['user_id'])) { echo "User ID: " . $_SESSION['user_id']; } ?>
This code shows how to start a session, store data, and access data.
Advanced Usage
To enhance session security, some advanced tips can be used, such as session fixed protection and session regeneration:
<?php session_start(); // Check if the session is fixed if (isset($_SESSION['initiated'])) { if ($_SESSION['initiated'] != true) { session_regenerate_id(); $_SESSION['initiated'] = true; } } else { session_regenerate_id(); $_SESSION['initiated'] = true; } //Storing and accessing session data $_SESSION['user_id'] = 123; echo "User ID: " . $_SESSION['user_id']; ?>
This code shows how to regenerate the session ID through session_regenerate_id()
function to prevent session fixed attacks.
Common Errors and Debugging Tips
Common errors when using session management include:
- Session data loss : It may be caused by the deletion of the session file or the session timeout. This can be solved by increasing the session lifecycle or using a database to store session data.
- Session Fixed Attack : You can prevent it by regenerating the session ID regularly.
- XSS attacks lead to session hijacking : can be prevented by strictly filtering and validating user input.
Debugging skills include:
- Use
session_status()
function to check the session status. - Check the session file storage path through
session_save_path()
function to ensure that the path is correct and writable. - Use browser developer tools to view cookies to ensure that the session ID is delivered correctly.
Performance optimization and best practices
In practical applications, it is very important to optimize the performance and security of session management. Here are some suggestions:
- Use database to store session data : Databases are more secure and performant than file storage. You can use the
session_set_save_handler()
function to customize the session storage mechanism.
<?php class SessionHandler { private $db; public function __construct($db) { $this->db = $db; } public function open($save_path, $name) { return true; } public function close() { return true; } public function read($id) { $stmt = $this->db->prepare("SELECT data FROM sessions WHERE id = ?"); $stmt->execute([$id]); $result = $stmt->fetch(); return $result ? $result['data'] : ''; } public function write($id, $data) { $stmt = $this->db->prepare("REPLACE INTO sessions (id, data) VALUES (?, ?)"); return $stmt->execute([$id, $data]); } public function destroy($id) { $stmt = $this->db->prepare("DELETE FROM sessions WHERE id = ?"); return $stmt->execute([$id]); } public function gc($maxlifetime) { $stmt = $this->db->prepare("DELETE FROM sessions WHERE DATE_ADD(last_accessed, INTERVAL ? SECOND) < NOW()"); return $stmt->execute([$maxlifetime]); } } $db = new PDO('mysql:host=localhost;dbname=your_database', 'username', 'password'); $handler = new SessionHandler($db); session_set_save_handler($handler, true); session_start(); ?>
Regularly regenerate session ID : Regularly regenerate session ID through
session_regenerate_id()
function, which can effectively prevent session fixed attacks.Use HTTPS : Ensure that all session data is transmitted over HTTPS and prevent man-in-the-middle attacks.
Code readability and maintenance : When writing session management code, pay attention to the readability and maintenance of the code. Use meaningful variable names and comments to ensure that the code is easy to understand and maintain.
In-depth thinking and suggestions
When preventing session hijacking, the following points need to be considered:
Session ID security : The generation algorithm and storage method of session ID directly affect its security. Generate session IDs using an algorithm that is complex enough and ensure that they are not stolen during transmission.
User behavior monitoring : By monitoring user behavior, abnormal session activity can be detected. For example, if a session is accessed from a different IP address within a short period of time, it may indicate that the session is hijacked.
Multi-factor authentication : Multi-factor authentication (MFA) can provide additional security even if the session ID is stolen. Users need to provide additional verification information (such as SMS verification code) to access the account.
Session timeout settings : Set the session timeout reasonably to reduce the window period of session hijacking. Excessive session timeout increases the risk of being attacked.
With the above strategy and code examples, you can better understand how session hijacking works and effectively prevent such attacks in PHP. I hope this article will be helpful to you and I wish you continuous progress on the road to cybersecurity!
The above is the detailed content of How does session hijacking work and how can you mitigate it in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


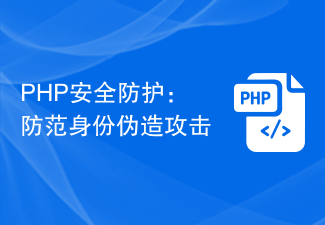
With the continuous development of the Internet, more and more businesses involve online interactions and data transmission, which inevitably causes security issues. One of the most common attack methods is identity forgery attack (IdentityFraud). This article will introduce in detail how to prevent identity forgery attacks in PHP security protection to ensure better system security. What is an identity forgery attack? Simply put, an identity forgery attack (IdentityFraud), also known as impersonation, refers to standing on the attacker’s side
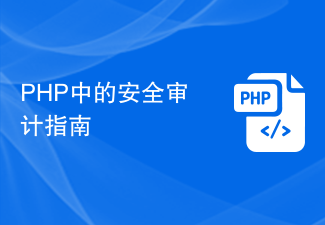
As web applications become more popular, security auditing becomes more and more important. PHP is a widely used programming language and the basis for many web applications. This article will introduce security auditing guidelines in PHP to help developers write more secure web applications. Input Validation Input validation is one of the most basic security features in web applications. Although PHP provides many built-in functions to filter and validate input, these functions do not fully guarantee the security of the input. Therefore, developers need
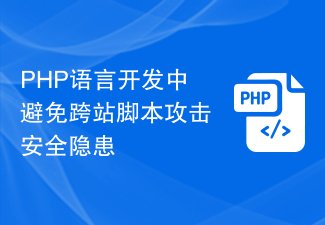
With the development of Internet technology, network security issues have attracted more and more attention. Among them, cross-site scripting (XSS) is a common network security risk. XSS attacks are based on cross-site scripting. Attackers inject malicious scripts into website pages to obtain illegal benefits by deceiving users or implanting malicious code through other methods, causing serious consequences. However, for websites developed in PHP language, avoiding XSS attacks is an extremely important security measure. because
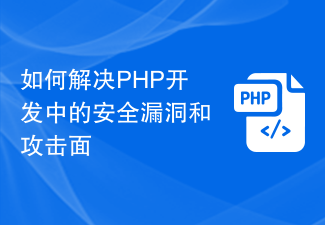
How to solve security vulnerabilities and attack surfaces in PHP development. PHP is a commonly used web development language. However, during the development process, due to the existence of security issues, it is easily attacked and exploited by hackers. In order to keep web applications secure, we need to understand and address the security vulnerabilities and attack surfaces in PHP development. This article will introduce some common security vulnerabilities and attack methods, and give specific code examples to solve these problems. SQL injection SQL injection refers to inserting malicious SQL code into user input to
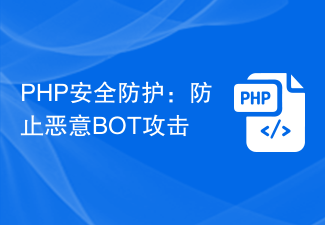
With the rapid development of the Internet, the number and frequency of cyber attacks are also increasing. Among them, malicious BOT attack is a very common method of network attack. It obtains website background login information by exploiting vulnerabilities or weak passwords, and then performs malicious operations on the website, such as tampering with data, implanting advertisements, etc. Therefore, for websites developed using PHP language, it is very important to strengthen security protection measures, especially in preventing malicious BOT attacks. 1. Strengthen password security. Password security is to prevent malicious BOT attacks.
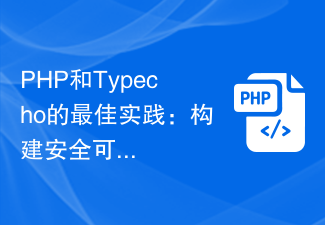
Best Practices for PHP and Typecho: Building a Safe and Reliable Website System [Introduction] Today, the Internet has become a part of people's lives. In order to meet user needs for websites, developers need to take a series of security measures to build a safe and reliable website system. PHP is a widely used development language, and Typecho is an excellent blogging program. This article will introduce how to combine the best practices of PHP and Typecho to build a safe and reliable website system. 【1.Input Validation】Input validation is built
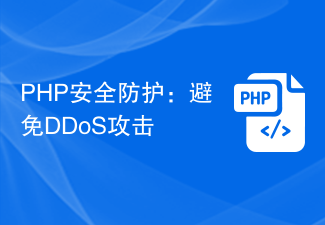
With the rapid development of Internet technology, website security issues are becoming more and more important. DDoS attacks are one of the most common security threats, especially for websites using PHP language, because PHP, as a dynamic language, is vulnerable to different forms of attacks. This article will introduce some PHP website protection techniques to help website administrators reduce the risk of DDoS attacks. 1. Using CDN (Content Delivery Network) CDN can help website administrators distribute website content and store static resources in the CDN cache to reduce
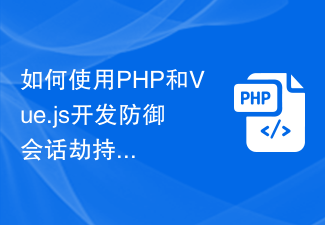
How to develop best practices for defense against session hijacking using PHP and Vue.js Session hijacking is an attack method in which an attacker obtains a user's session ID or token and gains unauthorized access to the user's session information. This kind of attack may lead to serious consequences such as user privacy leakage and identity forgery. To prevent session hijacking, we can develop some best practices using PHP and Vue.js. Strengthen back-end security First, we need to strengthen and protect the session on the back-end. Here are some common defenses: Use safe
