MongoDB Replication: Ensuring High Availability & Data Redundancy
MongoDB's replication set ensures high availability and redundancy of data through the following steps: 1) Data synchronization: the master node records write operations, and the replica node synchronizes data through oplog; 2) Heartbeat detection: the node regularly sends heartbeat signal detection status; 3) Failover: When the master node fails, the replica node elects a new master node to ensure that the service does not interrupt.
introduction
In modern application development, high availability and redundancy of data are no longer optional but essential. As a popular NoSQL database, MongoDB provides powerful replication capabilities to ensure high availability and redundancy of data. Today we will explore the replication mechanism of MongoDB in depth, understand how it works, and how it can be used to improve the reliability of the system in practical applications.
With this article, you will learn how to configure MongoDB's replication set, understand how it works, and master some best practices to ensure that your data is always available and secure.
Review of basic knowledge
MongoDB's Replica Set is the core of its high availability and data redundancy. Simply put, a replication set is a set of MongoDB instances, one of which is the primary node and the other is the secondary node. The master node is responsible for handling all write operations, while the replica node synchronizes data from the master node to ensure data consistency.
In MongoDB, data redundancy is achieved through replica nodes. If the master node fails, the replica node can automatically elect a new master node to ensure that the service is not interrupted.
Core concept or function analysis
Definition and function of copy set
MongoDB's replication set is a distributed system designed to provide high availability and data redundancy. Its main functions are:
- High Availability : Through automatic failover, ensure that the system can still operate normally even if the master node fails.
- Data redundancy : Prevent data loss by storing data on multiple nodes.
- Read and write separation : You can share read operations on the replica node to reduce the load on the primary node.
A simple replication set is configured as follows:
// Initialize the replication set rs.initiate({ _id: "myReplicaSet", Members: [ { _id: 0, host: "mongodb0.example.net:27017" }, { _id: 1, host: "mongodb1.example.net:27017" }, { _id: 2, host: "mongodb2.example.net:27017" } ] });
How it works
MongoDB's replication set works through the following steps:
- Data synchronization : The master node records all write operations in the operation log (oplog), and the replica node synchronizes data through oplog.
- Heartbeat detection : Each node will send a heartbeat signal regularly to detect the status of other nodes.
- Failover : If the master node fails, the replica node will select a new master node through the election mechanism.
In practical applications, it is very important to understand the size and synchronization delay of the oplog. The size of the oplog determines the amount of historical data that the replica node can trace back, while the synchronization delay affects the consistency of the data.
Example of usage
Basic usage
Configuring a basic replication set is very simple. Suppose you have three servers, namely mongodb0, mongodb1 and mongodb2, you can configure them as follows:
// Initialize the replication set rs.initiate({ _id: "myReplicaSet", Members: [ { _id: 0, host: "mongodb0:27017" }, { _id: 1, host: "mongodb1:27017" }, { _id: 2, host: "mongodb2:27017" } ] }); // Add the copy set rs.add("mongodb1:27017"); rs.add("mongodb2:27017");
Advanced Usage
In practical applications, you may need more complex configurations, such as priority settings, hidden nodes, etc. Here is a more advanced configuration example:
rs.initiate({ _id: "myReplicaSet", Members: [ { _id: 0, host: "mongodb0:27017", priority: 2 }, { _id: 1, host: "mongodb1:27017", priority: 1 }, { _id: 2, host: "mongodb2:27017", priority: 0, hidden: true } ] });
In this configuration, mongodb0 has the highest priority, mongodb2 is a hidden node that does not participate in the election.
Common Errors and Debugging Tips
Common errors when configuring and using MongoDB replication sets include:
- Synchronization failed : It may be caused by network problems or too small oplog. This can be solved by increasing the oplog size or checking the network connection.
- Election failure : It may be due to improper node priority settings or network partitioning. This can be resolved by adjusting priorities or checking network connections.
Debugging skills include:
- Use
rs.status()
command to view the status of the replication set. - Use
rs.printSlaveReplicationInfo()
command to view the synchronization information of the replica node.
Performance optimization and best practices
In practical applications, it is very important to optimize the performance of MongoDB replication sets. Here are some optimization suggestions:
- Adjust the oplog size : Adjust the oplog size appropriately according to your data volume and synchronization needs to ensure that the replica nodes can synchronize data in a timely manner.
- Read and write separation : share read operations on the replica node, reduce the load on the master node and improve the overall performance of the system.
- Priority setting : Set the priority of nodes reasonably according to your application needs to ensure that new master nodes can be quickly selected during failover.
When writing code, it is also very important to keep the code readable and maintained. Here is an example showing how to use MongoDB's replication set in your app:
const MongoClient = require('mongodb').MongoClient; const url = 'mongodb://mongodb0:27017,mongodb1:27017,mongodb2:27017/myReplicaSet'; MongoClient.connect(url, { replicaSet: 'myReplicaSet' }, function(err, client) { if (err) throw err; const db = client.db('mydb'); db.collection('mycollection').insertOne({ name: 'John Doe' }, function(err, result) { if (err) throw err; console.log('Document inserted'); client.close(); }); });
Through the above content, we have a deeper understanding of MongoDB's replication mechanism and mastered how to configure and optimize replication sets. In practical applications, flexibly applying this knowledge can greatly improve the reliability and performance of the system.
The above is the detailed content of MongoDB Replication: Ensuring High Availability & Data Redundancy. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



Introduction to how to implement load balancing and high availability in FastAPI: With the development of Internet applications, the requirements for system load balancing and high availability are getting higher and higher. FastAPI is a high-performance Python-based web framework that provides a simple and powerful way to build, deploy and scale web applications. This article will introduce how to implement load balancing and high availability in FastAPI and provide corresponding code examples. Using Nginx to achieve load balancingNginx is a popular
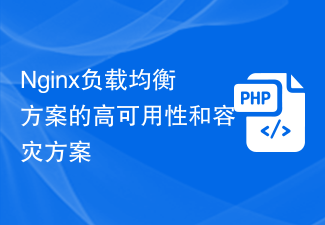
High Availability and Disaster Recovery Solution of Nginx Load Balancing Solution With the rapid development of the Internet, the high availability of Web services has become a key requirement. In order to achieve high availability and disaster tolerance, Nginx has always been one of the most commonly used and reliable load balancers. In this article, we will introduce Nginx’s high availability and disaster recovery solutions and provide specific code examples. High availability of Nginx is mainly achieved through the use of multiple servers. As a load balancer, Nginx can distribute traffic to multiple backend servers to
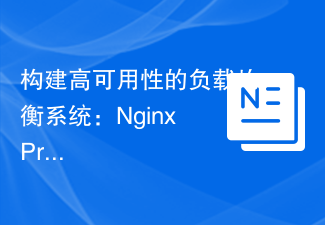
Building a high-availability load balancing system: Best practices for NginxProxyManager Introduction: In the development of Internet applications, the load balancing system is one of the essential components. It can achieve high concurrency and high availability services by distributing requests to multiple servers. NginxProxyManager is a commonly used load balancing software. This article will introduce how to use NginxProxyManager to build a high-availability load balancing system and provide
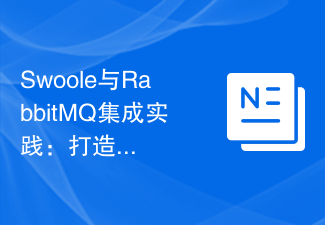
With the advent of the Internet era, message queue systems have become more and more important. It enables asynchronous operations between different applications, reduces coupling, and improves scalability, thereby improving the performance and user experience of the entire system. In the message queuing system, RabbitMQ is a powerful open source message queuing software. It supports a variety of message protocols and is widely used in financial transactions, e-commerce, online games and other fields. In practical applications, it is often necessary to integrate RabbitMQ with other systems. This article will introduce how to use sw
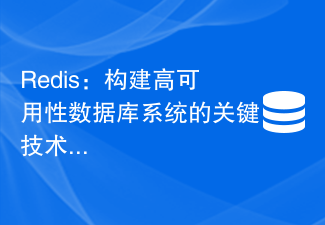
Redis: a key technology for building high-availability database systems. With the development of the Internet and the advent of the big data era, the need for high-availability database systems has become increasingly urgent. As an in-memory storage NoSQL database system, Redis has become one of the key technologies for building high-availability database systems with its excellent performance and flexible data model. This article will delve into the high availability technology of Redis and demonstrate it with specific code examples. 1. The high availability requirements of Redis in actual applications
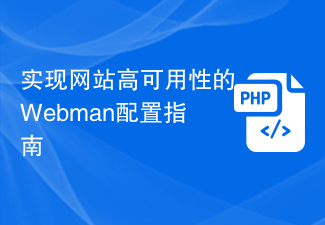
Introduction to Webman Configuration Guide for Implementing High Availability of Websites: In today's digital era, websites have become one of the important business channels for enterprises. In order to ensure the business continuity and user experience of enterprises and ensure that the website is always available, high availability has become a core requirement. Webman is a powerful web server management tool that provides a series of configuration options and functions that can help us achieve a high-availability website architecture. This article will introduce some Webman configuration guides and code examples to help you achieve the high performance of your website.
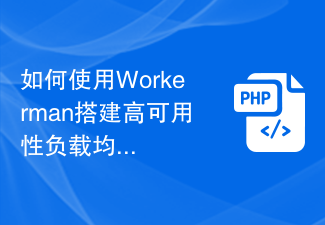
How to use Workerman to build a high-availability load balancing system requires specific code examples. In the field of modern technology, with the rapid development of the Internet, more and more websites and applications need to handle a large number of concurrent requests. In order to achieve high availability and high performance, the load balancing system has become one of the essential components. This article will introduce how to use the PHP open source framework Workerman to build a high-availability load balancing system and provide specific code examples. 1. Introduction to Workerman Worke
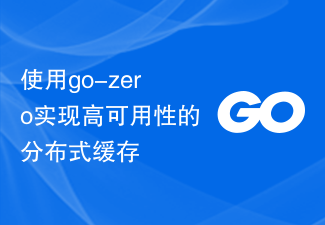
With the development of web applications, more and more attention is turning to how to improve application performance. The role of caching is to offset high traffic and busy loads and improve the performance and scalability of web applications. In a distributed environment, how to implement high-availability caching has become an important technology. This article will introduce how to use some tools and frameworks provided by go-zero to implement high-availability distributed cache, and briefly discuss the advantages and limitations of go-zero in practical applications. 1. What is go-
