Explain strict types (declare(strict_types=1);) in PHP.
Strict types in PHP are enabled by adding declare(strict_types=1); at the top of the file. 1) It forces type checking of function parameters and return values to prevent implicit type conversion. 2) Using strict types can improve the reliability and predictability of the code, reduce bugs, and improve maintainability and readability.
introduction
Have you ever encountered type-related troubles when writing PHP code? Maybe you've heard of strict types, but it's not clear about its specific uses and benefits. Today, let’s take a closer look at the strict types in PHP (declare(strict_types=1);). This article will not only explain the definition and role of strict types, but will also help you better understand and apply this feature through actual code examples and sharing of experience. After reading this article, you will be able to use strict types confidently in your projects, improving the quality and maintainability of your code.
Review of basic knowledge
In PHP, type systems have always been a relatively loose field. Traditionally, PHP uses a weak type system, which means that the type of variables can be changed dynamically at runtime. Although this flexibility is convenient, it can easily lead to some difficult to track errors. Strict types (strict_types) are a feature introduced in PHP 7, aiming to improve the reliability and predictability of your code by forcing type checking.
Strict type is enabled by adding declare(strict_types=1);
declaration at the top of the file. Once enabled, PHP performs strict type checks on function parameters and return values, and no longer allows implicit type conversions.
Core concept or function analysis
Definition and function of strict types
Strict type (strict_types) is a declaration used to enforce type checking in PHP. By adding declare(strict_types=1);
declaration at the top of the file, PHP performs strict type checks on function parameters and return values. This means that if the function expects to receive an integer, but is actually passing in a string, PHP will throw a TypeError exception instead of trying to perform an implicit type conversion.
The main function of strict types is to improve the reliability and predictability of the code. By mandatory type checking, potential type errors can be found at the development stage, rather than exposed at runtime. This not only helps reduce bugs, but also improves the maintainability and readability of the code.
How it works
When you enable strict type, PHP will strictly check the parameters and return values of the function. Let's look at a simple example:
<?php declare(strict_types=1); function add(int $a, int $b): int { return $a $b; } echo add(1, 2); // Output 3 echo add(1, '2'); // Throw TypeError
In this example, the add
function expects to receive two integer parameters and return an integer. If we try to pass in a string as an argument, PHP will throw a TypeError exception because strict types do not allow implicit type conversion.
The strict type implementation principle involves PHP's Zend engine. During the compilation phase, the Zend engine parses declare(strict_types=1);
declaration and performs type checking on the function call at runtime. If the types do not match, the Zend engine will throw a TypeError exception.
Example of usage
Basic usage
Let's look at a more complex example showing the basic usage of strict types:
<?php declare(strict_types=1); function calculateArea(float $radius): float { return pi() * $radius ** 2; } $radius = 5.0; $area = calculateArea($radius); echo "The area of the circle is: {$area}"; // The area of the output circle is: 78.539816339745
In this example, calculateArea
function expects to receive a floating point number as a parameter and returns a floating point number. Strict types ensure that we do not accidentally pass an integer or string, thus avoiding potential errors.
Advanced Usage
Strict types are also very useful when dealing with complex data structures. Let's look at an example of a class using strict types:
<?php declare(strict_types=1); class Rectangle { private float $width; private float $height; public function __construct(float $width, float $height) { $this->width = $width; $this->height = $height; } public function getArea(): float { return $this->width * $this->height; } } $rectangle = new Rectangle(10.0, 5.0); echo "The area of the rectangle is: {$rectangle->getArea()}"; // The area of the output rectangle is: 50
In this example, Rectangle
class uses strict types to ensure that width
and height
properties are floating-point numbers, and getArea
method returns a floating-point number. Strict types are here to help us ensure consistency and correctness of our data.
Common Errors and Debugging Tips
When using strict types, you may encounter some common errors. For example, if you try to pass a string into a function that expects to receive integers, PHP will throw a TypeError exception. Here are some debugging tips:
- Use
var_dump
orprint_r
functions to check the type and value of a variable. - Using type prompts and code checking tools in the IDE, you can discover potential type errors when writing code.
- Carefully check the function's parameters and return value types to make sure they are consistent with the function declaration.
Performance optimization and best practices
Strict typing not only improves code reliability, but also improves performance in some cases. By avoiding implicit type conversion, PHP can execute code faster. Here are some recommendations for performance optimization and best practices:
- In large projects, enabling strict types can significantly reduce type-related errors, thereby improving the overall quality of your code.
- Using strict types can improve the readability of the code because the parameters and return value types of functions are more explicit.
- In performance-sensitive code, strict type can reduce the time overhead of type checking, because PHP no longer requires implicit type conversion.
Overall, strict typing is a powerful tool in PHP that can help you write more reliable and efficient code. In actual projects, I found that after enabling strict types, the maintainability and readability of the code have been significantly improved. While there may be some challenges at the beginning, once you get used to the strict type, you will find that the benefits it brings are huge.
Hopefully this article helps you better understand and apply strict types in PHP. If you have any questions or experience sharing, please leave a message in the comment area to discuss!
The above is the detailed content of Explain strict types (declare(strict_types=1);) in PHP.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


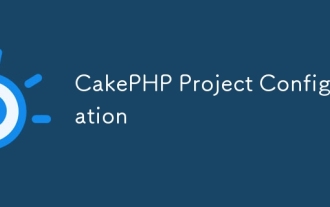
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
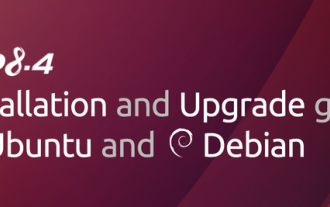
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
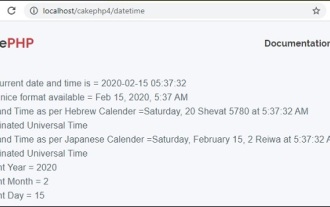
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
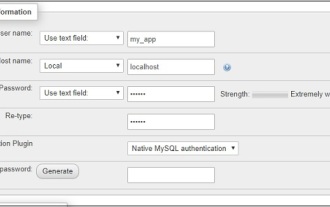
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
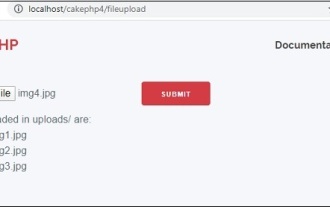
To work on file upload we are going to use the form helper. Here, is an example for file upload.
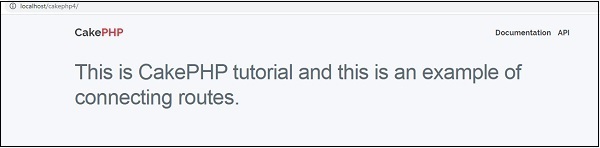
In this chapter, we are going to learn the following topics related to routing ?
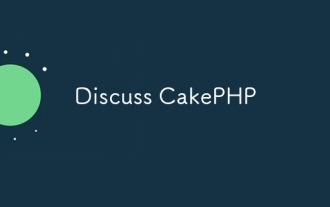
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
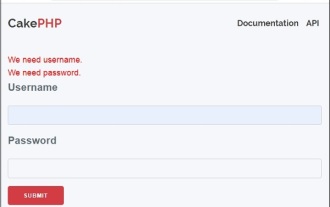
Validator can be created by adding the following two lines in the controller.
