Vue and Element-UI Cascading Pull-down Box Case Tutorial
The cascading selectors in Vue and Element-UI handle not only parent-child relationships, but a tree-shaped data structure. Through careful data design, complex scenarios such as multi-level linkage between provinces, cities and counties can be achieved. Pay attention to performance optimization when loading asynchronously, such as using virtual scrolling, caching and anti-shake/throttling technologies. For code quality, readability, maintainability and performance should be emphasized.
Vue and Element-UI cascade selectors: more than just a simple parent-son relationship
Have you ever wondered how to elegantly implement a cascading drop-down box in a Vue project to make the user experience smoother and the code simpler? This article takes you into the cascading selectors of Vue and Element-UI, which not only teaches you how to use them, but more importantly, understand the mechanisms behind them and how to avoid some common pitfalls. After reading, you will be able to independently complete various complex cascading selection scenarios and write efficient and maintainable code.
Let’s start with the basics. Element-UI's el-cascader
component looks like a multi-stage linkage selector on the surface, but in fact, behind it is a clever combination of data structures and event processing. You have to understand that it does not handle simple parent-son relationships, but a tree-shaped data. Each node of this tree represents an option, and the parent and child nodes are connected through children
attribute. This is not a simple array set, but requires careful design of data structures to exert the power of el-cascader
.
Let’s take a simple example, suppose we want to conduct a three-level linkage between provinces, cities and counties:
<code class="javascript"><template> <el-cascader v-model="selectedOptions" :options="options" :props="{ label: 'label', value: 'value', children: 'children' }"></el-cascader> <p>Selected: {{ selectedOptions }}</p> </template> <script> export default { data() { return { selectedOptions: [], options: [ { value: '北京', label: '北京', children: [ { value: '朝阳', label: '朝阳' }, { value: '海淀', label: '海淀' } ] }, { value: '上海', label: '上海', children: [ { value: '浦东', label: '浦东' }, { value: '黄浦', label: '黄浦' } ] } ] }; }, methods: { handleChange(value) { console.log(value); // 处理选择的省市县} } }; </script></code>
This code shows the most basic usage. options
attribute defines the data structure, props
attribute specifies the names of label
, value
and children
fields, v-model
binds the selected value, and @change
event listens for the selection change. Note that value
field is what you use to uniquely identify each option, and it will be returned in the handleChange
function.
But this is just the tip of the iceberg. In actual applications, your data may come from the backend interface and need to be loaded asynchronously. At this time, you need to use a function in the options
property, which returns a Promise. After the Promise is resolved, el-cascader
will re-render. Remember that performance and user experience are crucial when loading asynchronously, avoiding unnecessary requests and redundant data.
Going deeper, you may encounter situations where you need to dynamically load children based on the selected parent node. This requires you to cleverly combine @change
events and asynchronous requests. Each time a change is selected, a new request is triggered to obtain the data of the corresponding child nodes. This part of the code will be more complicated and requires careful processing of the loading state to avoid users from seeing flickering or error prompts. A good practice is to use a loading status indicator to let users know that the data is loading.
Finally, some suggestions for performance optimization:
- Data structure optimization: If your data volume is large, consider using virtual scrolling or paging loading to avoid rendering all data at once.
- Cache: Caches loaded data to reduce duplicate requests.
- debounce/throttle: If your asynchronous requests are frequently triggered, use debounce or throttle technology to limit the frequency of requests.
Remember, the elegance of the code is not only reflected in the implementation of functions, but also in the readability, maintainability and performance. Don't sacrifice code quality in pursuit of fast implementation of features. A good cascading selector should be efficient, stable and easy to scale. Hopefully this article will help you better understand and use the cascading selectors of Vue and Element-UI and write better code.
The above is the detailed content of Vue and Element-UI Cascading Pull-down Box Case Tutorial. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
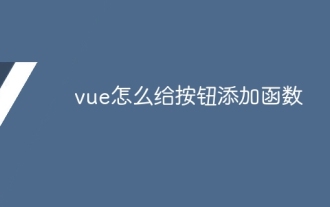
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
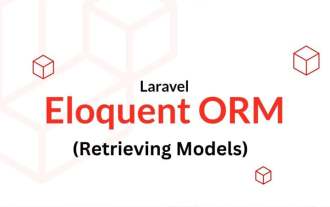
LaravelEloquent Model Retrieval: Easily obtaining database data EloquentORM provides a concise and easy-to-understand way to operate the database. This article will introduce various Eloquent model search techniques in detail to help you obtain data from the database efficiently. 1. Get all records. Use the all() method to get all records in the database table: useApp\Models\Post;$posts=Post::all(); This will return a collection. You can access data using foreach loop or other collection methods: foreach($postsas$post){echo$post->
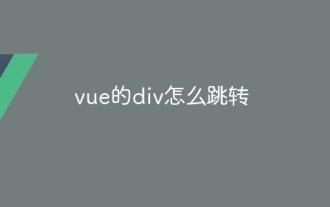
There are two ways to jump div elements in Vue: use Vue Router and add router-link component. Add the @click event listener and call this.$router.push() method to jump.
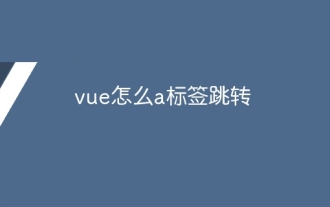
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
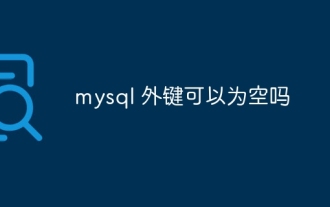
MySQL foreign keys can be empty, but be cautious. Allowing foreign keys to be empty is beneficial to booking systems, multi-stage processes and flexible business logic, but it also brings the risks of data redundancy, reduced data integrity and logical errors. Decisions depend on business needs, and need to weigh the pros and cons, improve error handling mechanisms, standardize data management, and select different ON DELETE options according to specific needs.

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema
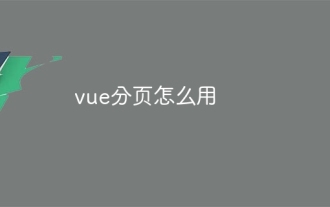
Pagination is a technology that splits large data sets into small pages to improve performance and user experience. In Vue, you can use the following built-in method to paging: Calculate the total number of pages: totalPages() traversal page number: v-for directive to set the current page: currentPage Get the current page data: currentPageData()

Function interception in Vue is a technique used to limit the number of times a function is called within a specified time period and prevent performance problems. The implementation method is: import the lodash library: import { debounce } from 'lodash'; Use the debounce function to create an intercept function: const debouncedFunction = debounce(() => { / Logical / }, 500); Call the intercept function, and the control function is called at most once in 500 milliseconds.
