Using Vue.js, how to convert strings into JavaScript objects?
When converting strings to JavaScript objects in Vue.js, the correct way is to use JSON.parse() to avoid using eval(). JSON.parse() is safe and reliable, but requires error handling because a format error will throw an exception. For large JSON strings, consider optimization strategies such as using faster parsing libraries or server-side preprocessing. Code readability and maintainability are also important, and clear and concise code helps to understand and maintain.
Vue.js string to JavaScript objects: those pitfalls and tricks
Many friends will encounter situations when using Vue.js that need to convert a JSON string into a JavaScript object. This looks simple, but there are many pits hidden inside, and you will fall in it if you are not careful. In this article, let’s talk about this and share some of my experiences to avoid detours.
Let’s talk about the conclusion first: Don’t use eval()
directly! This thing has extremely high risks and huge safety risks. Don't touch it unless you know what you are doing. Imagine if your string comes from user input, the consequences are unimaginable. Hackers can use eval()
to execute malicious code in minutes.
So how to convert? JSON.parse()
is your best choice. It is specially used to parse JSON strings, which are safe and reliable, and have good performance.
<code class="javascript">let jsonString = '{"name": "张三", "age": 30, "city": "北京"}'; let jsonObject = JSON.parse(jsonString); console.log(jsonObject.name); // 输出: 张三console.log(jsonObject.age); // 输出: 30</code>
Doesn't it seem very simple? But things didn't end that easily. JSON.parse()
also has its temper. If your JSON string is incorrect, it will directly throw an error, causing your application to crash. So, you need to handle errors well.
<code class="javascript">let jsonString = '{"name": "张三", "age": 30, "city": "北京"'; //故意弄错格式try { let jsonObject = JSON.parse(jsonString); console.log(jsonObject); } catch (error) { console.error("JSON 解析失败:", error); //优雅的错误处理//这里可以添加一些额外的处理逻辑,比如显示友好的错误提示给用户}</code>
Remember, good error handling is the key to high-quality code. Don't expect your JSON strings to be perfect forever. Network requests, user input, etc. will cause the JSON string format to be incorrect.
Let’s talk about performance. For large JSON strings, the performance of JSON.parse()
can become a bottleneck. At this time, you can consider some optimization strategies, such as using faster JSON parsing libraries, or preprocessing data on the server side. This needs to be weighed based on actual conditions.
Finally, let me mention one more thing: the readability and maintainability of the code are very important. Even if your code can run, it will fail if others (or you in the future) can't understand it. Therefore, be sure to write clear and concise code and add necessary comments. Don't sacrifice code readability in pursuit of so-called "skills".
This article is just a way to attract attention. In actual application, you may encounter more complicated situations. But as long as you master the usage methods and error handling skills of JSON.parse()
, you can easily deal with most situations. Remember, safety comes first, code quality comes first! Hope this article is helpful to you.
The above is the detailed content of Using Vue.js, how to convert strings into JavaScript objects?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


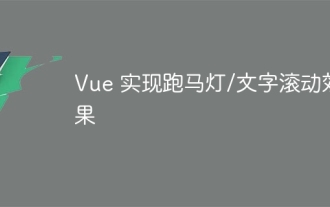
Implement marquee/text scrolling effects in Vue, using CSS animations or third-party libraries. This article introduces how to use CSS animation: create scroll text and wrap text with <div>. Define CSS animations and set overflow: hidden, width, and animation. Define keyframes, set transform: translateX() at the beginning and end of the animation. Adjust animation properties such as duration, scroll speed, and direction.
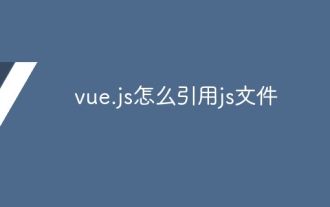
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
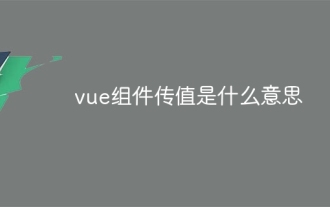
Vue component passing values is a mechanism for passing data and information between components. It can be implemented through properties (props) or events: Props: Declare the data to be received in the component and pass the data in the parent component. Events: Use the $emit method to trigger an event and listen to it in the parent component using the v-on directive.
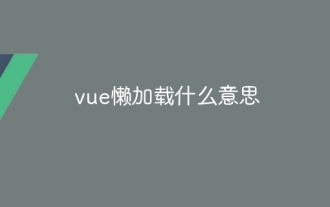
In Vue.js, lazy loading allows components or resources to be loaded dynamically as needed, reducing initial page loading time and improving performance. The specific implementation method includes using <keep-alive> and <component is> components. It should be noted that lazy loading can cause FOUC (splash screen) issues and should be used only for components that need lazy loading to avoid unnecessary performance overhead.
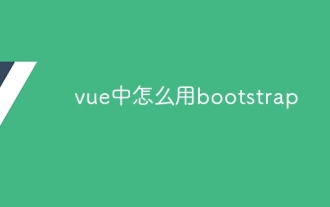
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
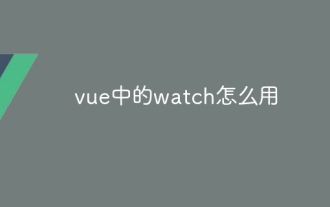
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
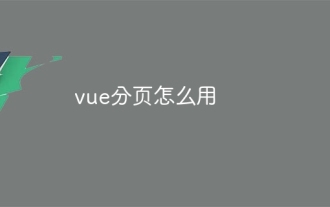
Pagination is a technology that splits large data sets into small pages to improve performance and user experience. In Vue, you can use the following built-in method to paging: Calculate the total number of pages: totalPages() traversal page number: v-for directive to set the current page: currentPage Get the current page data: currentPageData()

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.
