


How can you prevent a class from being extended or a method from being overridden in PHP? (final keyword)
In PHP, the final keyword is used to prevent classes from being inherited and methods being overwritten. 1) When the class is marked as final, the class cannot be inherited. 2) When the marker method is final, the method cannot be rewritten by the subclass. Using the final keyword ensures the stability and security of your code.
introduction
In PHP programming, the inheritance of control classes and the rewriting of methods are very important technical details. What we are going to explore today is how to use the final
keyword to achieve this. Through this article, you will learn how to prevent classes from being extended and methods from being rewritten. At the same time, I will share some experiences and precautions for using final
keywords in actual projects.
Review of basic knowledge
In PHP, inheritance of classes and rewriting methods are the core concepts of object-oriented programming. Inheritance allows one class to get properties and methods from another class, while overwriting allows the subclass to modify the method behavior of the parent class. However, sometimes we need to limit this behavior to ensure the stability and security of the code.
The final
keyword is a tool used to implement this limitation. It can be applied to classes and methods. When a class is marked as final
, it cannot be inherited; when a method is marked as final
, it cannot be overridden by subclasses.
Core concept or function analysis
Definition and function of final
keyword
The final
keyword is used in PHP to restrict inheritance of classes and rewrite methods. Its purpose is to ensure that certain classes or methods are not modified accidentally when used, thereby maintaining the expected behavior of the code.
For example, if we have a core feature class, we may not want it to be extended or modified by other developers, then we can use the final
keyword.
final class CoreFunctionality { public function doSomething() { // Core function implementation} }
In this example, the CoreFunctionality
class cannot be inherited, and doSomething
method cannot be rewritten.
How it works
When you use the final
keyword, the PHP compiler checks for violations of final
restrictions during the code parsing phase. If a class is found to try to inherit a final
class, or a method tries to override a final
method, PHP will throw a fatal error (Fatal Error).
This mechanism ensures the integrity and security of the code because it prevents illegal operations before it runs.
Example of usage
Basic usage
Let's look at a simple example showing how to use the final
keyword to prevent classes from being extended:
final class ImmutableClass { public function getValue() { return "This class cannot be extended."; } } // Attempting to inherit ImmutableClass will cause an error // class ExtendedClass extends ImmutableClass {}
In this example, ImmutableClass
is marked final
, so any attempt to inherit it will result in an error.
Advanced Usage
In more complex scenarios, we might want some methods to be final
, while others can be rewritten. Consider the following example:
class BaseClass { final public function criticalMethod() { // This method cannot be rewritten} public function flexibleMethod() { // This method can be rewritten} } class DerivedClass extends BaseClass { // Trying to rewrite criticalMethod will cause an error // public function criticalMethod() {} public function flexibleMethod() { // You can rewrite this method} }
In this example, criticalMethod
is final
and therefore cannot be rewritten, while flexibleMethod
can be rewritten by the subclass DerivedClass
.
Common Errors and Debugging Tips
Common errors when using final
keywords include:
- Try to inherit a
final
class - Try to rewrite a
final
method
These errors are caught by PHP before the code is executed and a fatal error is thrown. To debug these issues, make sure you double-check the class definition and method declarations and make sure there are no final
restrictions violated.
Performance optimization and best practices
There are a few things to note when using final
keyword:
- Performance impact : Using
final
keyword will not have a significant impact on the running performance of the code, because it mainly affects the inspection during the compilation phase. - Code readability : Using
final
keyword in code can improve the readability of the code because it expresses the design intention and tells other developers which parts are not modified. - Maintenance : The
final
keyword can help maintain the stability of the code and prevent unnecessary modifications and extensions.
In a real project, I found that one of the best practices for using final
keywords is to apply it to core functional classes and methods, which ensures that these critical parts are not modified unexpectedly. For example, in a payment processing system, the core payment logic class can be marked final
to prevent any modifications that may lead to security breaches.
In general, the final
keyword is a powerful tool in PHP for controlling the inheritance of classes and the rewriting of methods. By using it reasonably, you can ensure the stability and security of your code while improving the readability and maintenance of your code.
The above is the detailed content of How can you prevent a class from being extended or a method from being overridden in PHP? (final keyword). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


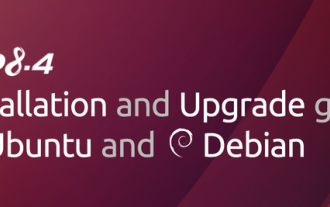
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
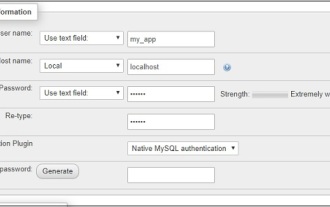
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
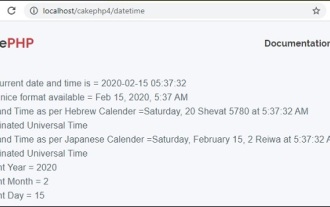
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
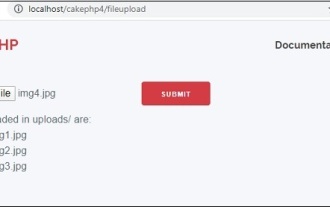
To work on file upload we are going to use the form helper. Here, is an example for file upload.
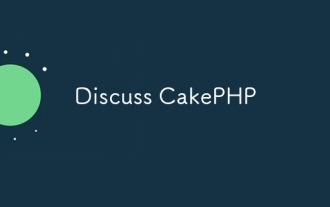
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
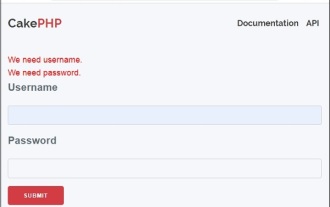
Validator can be created by adding the following two lines in the controller.
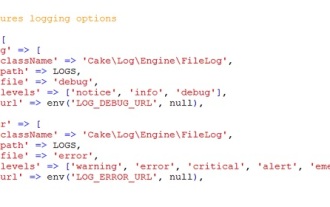
Logging in CakePHP is a very easy task. You just have to use one function. You can log errors, exceptions, user activities, action taken by users, for any background process like cronjob. Logging data in CakePHP is easy. The log() function is provide
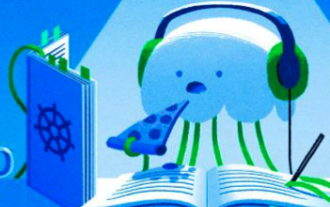
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
