Describe rate limiting techniques for PHP APIs.
PHP API current limiting can be achieved through fixed window counters, sliding window counters, leaky bucket algorithms and token bucket algorithms. 1. The fixed window counter limits the number of requests through the time window. 2. The sliding window counter refines the time window to provide more accurate current limiting. 3. The leaky bucket algorithm processes requests at a constant rate to prevent burst traffic. 4. The token bucket algorithm allows for a certain degree of burst traffic, and controls requests by consuming tokens.
introduction
Rate limiting is an indispensable part of building efficient and secure PHP APIs. Current limiting not only protects your API from abuse and DDoS attacks, but also ensures fair use of services and reasonable allocation of resources. This article will explore in-depth various technologies for PHP API current limiting to help you understand their implementation principles, advantages and disadvantages, and their application in actual projects.
By reading this article, you will learn how to implement different current limiting strategies in PHP, learn how to choose the appropriate current limiting method for your application scenario, and master some practical best practices and optimization techniques.
Review of basic knowledge
The core idea of current limiting is to limit the number of requests made by the client to the API within a certain period of time. Common current limiting algorithms include fixed window counters, sliding window counters, leaky bucket algorithms and token bucket algorithms. As a widely used server-side scripting language, PHP provides multiple ways to implement these algorithms.
In PHP, current limiting is usually achieved through middleware or standalone services. Middleware can intercept requests and execute current limiting logic, while independent services can provide greater flexibility and scalability.
Core concept or function analysis
Definition and function of current limiting algorithm
The purpose of the current limiting algorithm is to control the frequency of requests at the API level to prevent excessive requests from causing system crashes or performance degradation. Here are several common current limiting algorithms:
- Fixed window counter : divides time into fixed-sized windows, and the request count in each window does not exceed the set threshold.
- Sliding window counter : On the basis of fixed windows, further refine the time window to provide more accurate current limiting.
- Leak bucket algorithm : Requests to flow out at a constant rate, similar to funnel leakage, preventing burst flow.
- Token bucket algorithm : Add tokens to the bucket at a constant rate. The request needs to consume the token and allow a certain degree of burst traffic.
How it works
Fixed window counter
Fixed window counter is the simplest current limiting algorithm. Here is a simple PHP implementation:
class FixedWindowRateLimiter { private $limit; private $windowSize; private $requests; public function __construct($limit, $windowSize) { $this->limit = $limit; $this->windowSize = $windowSize; $this->requests = []; } public function allowRequest($clientId) { $now = time(); $windowStart = $now - ($now % $this->windowSize); if (!isset($this->requests[$clientId]) || $this->requests[$clientId]['start'] < $windowStart) { $this->requests[$clientId] = ['start' => $windowStart, 'count' => 1]; return true; } if ($this->requests[$clientId]['count'] < $this->limit) { $this->requests[$clientId]['count'] ; return true; } return false; } }
In this implementation, we use an array to record the number of requests and window start time for each client. Each time we request, we check whether the current time enters a new window, and if so, reset the counter; otherwise, check whether the number of requests in the current window exceeds the limit.
Sliding window counter
The sliding window counter further refines the time window based on the fixed window to provide more accurate current limiting. Here is a simple PHP implementation:
class SlidingWindowRateLimiter { private $limit; private $windowSize; private $requests; public function __construct($limit, $windowSize) { $this->limit = $limit; $windowSize = $windowSize; $this->requests = []; } public function allowRequest($clientId) { $now = time(); $this->requests[$clientId] = array_filter($this->requests[$clientId] ?? [], function($timestamp) use ($now, $windowSize) { return $timestamp > $now - $windowSize; }); if (count($this->requests[$clientId]) < $this->limit) { $this->requests[$clientId][] = $now; return true; } return false; } }
In this implementation, we use an array to record the request timestamp of each client. Each time we request, we filter out timestamps that are out of the window range and then check whether the number of requests in the current window exceeds the limit.
Leak bucket algorithm
The leaking bucket algorithm realizes current limit by simulating the process of water leakage in the funnel. Here is a simple PHP implementation:
class LeakyBucketRateLimiter { private $capacity; private $leakRate; private $currentAmount; private $lastLeakTime; public function __construct($capacity, $leakRate) { $this->capacity = $capacity; $this->leakRate = $leakRate; $this->currentAmount = 0; $this->lastLeakTime = time(); } public function allowRequest() { $now = time(); $leaked = ($now - $this->lastLeakTime) * $this->leakRate; $this->currentAmount = max(0, $this->currentAmount - $leaked); $this->lastLeakTime = $now; if ($this->currentAmount 1 <= $this->capacity) { $this->currentAmount ; return true; } return false; } }
In this implementation, we use a variable to record the amount of water in the current bucket. Each time we request, we first calculate the amount of water missed and then check if there is enough space to add a new request.
Token bucket algorithm
The token bucket algorithm implements current limit by simulating the process of adding tokens to the bucket. Here is a simple PHP implementation:
class TokenBucketRateLimiter { private $capacity; private $fillRate; private $tokens; private $lastFillTime; public function __construct($capacity, $fillRate) { $this->capacity = $capacity; $this->fillRate = $fillRate; $this->tokens = $capacity; $this->lastFillTime = time(); } public function allowRequest() { $now = time(); $tokensToAdd = ($now - $this->lastFillTime) * $this->fillRate; $this->tokens = min($this->capacity, $this->tokens $tokensToAdd); $this->lastFillTime = $now; if ($this->tokens >= 1) { $this->tokens--; return true; } return false; } }
In this implementation, we use a variable to record the number of tokens in the current bucket. Each time we request, we first calculate the number of tokens added and then check if there are enough tokens to handle the request.
Example of usage
Basic usage
Here is a simple example showing how to use fixed window counter current limiting in PHP API:
$limiter = new FixedWindowRateLimiter(10, 60); // Up to 10 requests per minute $clientId = 'user123'; if ($limiter->allowRequest($clientId)) { // Process the request echo "Request allowed"; } else { // Return error message echo "Rate limit exceeded"; }
Advanced Usage
In practical applications, you may need to combine multiple current limiting algorithms to implement more complex current limiting strategies. For example, you can use the token bucket algorithm to handle burst traffic while using a fixed window counter to limit the overall request frequency. Here is an example:
$tokenBucket = new TokenBucketRateLimiter(100, 1); // Up to 100 requests per second $fixedWindow = new FixedWindowRateLimiter(1000, 60); // Up to 1000 requests per minute $clientId = 'user123'; if ($tokenBucket->allowRequest() && $fixedWindow->allowRequest($clientId)) { // Process the request echo "Request allowed"; } else { // Return error message echo "Rate limit exceeded"; }
Common Errors and Debugging Tips
Common errors when implementing current limiting include:
- Time window calculation error : Ensure that the start and end time of the time window is correctly calculated, and avoid misjudging whether the request is in the same window.
- Concurrency problem : In a high concurrency environment, ensure that the current limit logic is thread-safe and avoid multiple requests passing the current limit check at the same time.
- Data persistence problem : If you use memory to store stream limiting data, make sure that data is not lost after the server restarts.
Debugging skills include:
- Logging : Records the current limit check results for each request to help analyze the effectiveness of the current limit strategy.
- Testing Tool : Use load testing tools to simulate high concurrent requests and verify the correctness and performance of the current limiting strategy.
Performance optimization and best practices
In practical applications, it is very important to optimize the performance and maintainability of the current limiting strategy. Here are some suggestions:
- Using Redis or other distributed caches : Using distributed caches such as Redis to store stream-limited data in high concurrency environments can improve performance and scalability.
- Asynchronous processing : asynchronize the current limiting logic to reduce blockage of request processing.
- Dynamically adjust the current limiting parameters : dynamically adjust the current limiting parameters according to the actual flow and system load to achieve a more flexible current limiting strategy.
When choosing a current limiting algorithm, the following factors need to be considered:
- Accuracy : Sliding window counters and token bucket algorithms are more accurate than fixed window counters, but also have higher implementation complexity.
- Burst traffic processing : The token bucket algorithm can better handle burst traffic, while the leak bucket algorithm is more suitable for smooth traffic.
- Implementation complexity : Fixed window counter is the simplest, but it may lead to inaccurate current limiting; the sliding window counter and token bucket algorithm implementation is more complex, but the current limiting effect is better.
Through the study of this article, you should have mastered the basic concepts and implementation methods of PHP API current limit. Hopefully this knowledge will help you better protect and optimize your API in real projects.
The above is the detailed content of Describe rate limiting techniques for PHP APIs.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


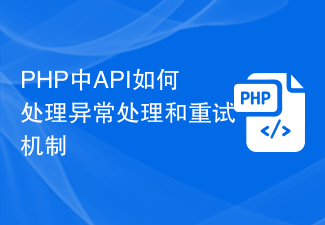
How APIs in PHP handle exception handling and retry mechanisms In PHP, APIs have become the core of many websites and applications as they provide various features and functions. However, when using APIs, we often encounter many problems, such as network connection problems, response timeouts, invalid requests, etc. In this case, we need to understand how to handle exceptions and retry mechanisms to ensure the reliability and stability of our applications. Exception handling In PHP, exception handling is a more elegant and readable error handling

PHP Kuaishou API Interface Development Guide: How to Build a Video Download and Upload System Introduction: With the booming development of social media, more and more people like to share their life moments on the Internet. Among them, short video platforms continue to grow in popularity and have become an important way for people to record and share their lives and entertainment. The PHP Kuaishou API interface is a powerful tool that can help developers build feature-rich video download and upload systems. In this article, we will explore how to use the PHP Kuaishou API interface to develop a
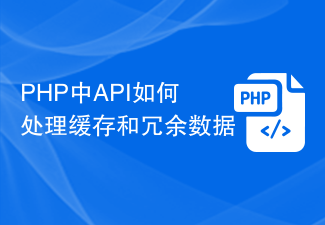
PHP is a very popular server-side scripting language that is widely used in web development. In web development, API is a very important component, responsible for communicating with the client. Among them, API performance and efficiency are very important to the user experience of an application. Caching and redundant data are two important concepts during API development. This article will introduce how to handle them in PHP to improve the performance and reliability of the API. 1. Caching concept Caching is an optimization technology widely used in web applications.
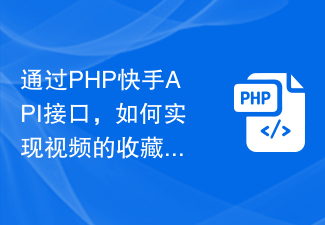
Through the PHP Kuaishou API interface, video collection and sharing can be realized. In the era of mobile Internet, short videos have become an indispensable part of people's lives. As China’s mainstream short video social platform, Kuaishou has a huge user base. In order to improve user experience, we can implement video collection and sharing functions through the PHP Kuaishou API interface, allowing users to more conveniently manage and share their favorite videos. 1. Use Kuaishou API Kuaishou provides a rich API interface, including video search, video details, video collection and video analysis.
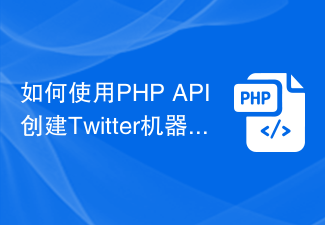
With the popularity of social media, more and more people are beginning to use social media platforms such as Twitter for marketing and promotion. This approach is effective, but requires a lot of time and effort to stay active. If you want to promote your brand or service on Twitter but don’t have enough time or resources to manage an active Twitter account, then you might consider using a Twitter bot. A Twitter bot is an automated tool that helps you create your own posts on Twitter
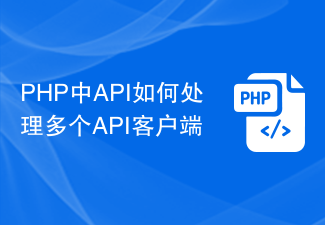
In modern web applications, API interfaces are usually a way to implement service interfaces. When implementing such an API interface in PHP language, you need to consider how to handle multiple API clients. Under normal circumstances, each API client request will be processed through the RESTful interface implemented by PHP. However, when a large number of API client requests need to be handled, how to improve the interface processing efficiency and reduce system overhead has become an urgent problem to be solved.
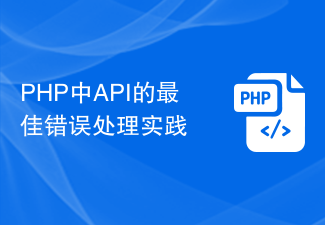
As a widely used programming language, PHP has become one of the main tools for web application development. When an application needs to interact with multiple external systems, such as databases, other web services, or third-party servers, using APIs is a popular implementation method. However, error handling is a very important part when writing APIs in PHP. Good error handling methods can not only continuously improve the reliability and robustness of the application, but also greatly improve the API usage experience. Here are some of the most

PHP Kuaishou API Interface Development Guide: How to Build a Video Playback and Comment System Introduction: With the rise of the Kuaishou platform, many developers have developed various applications through its API interface. This article will introduce how to use PHP to develop the API interface of the Kuaishou video playback and comment system to help readers quickly get started and build their own applications. 1. Preparation work Before starting, you need to ensure that you have completed the following preparation work: Install PHP environment: You need to set up PH in the local development environment
