


Bootstrap & JavaScript Integration: Dynamic Features & Functionality
Bootstrap and JavaScript can be seamlessly integrated to give web pages dynamic functionality. 1) Use JavaScript to manipulate Bootstrap components, such as modal boxes and navigation bars. 2) Ensure jQuery loads correctly and avoid common integration problems. 3) Achieve complex user interaction and dynamic effects through event monitoring and DOM operations.
introduction
In this era of rapid development of the Internet, building a website that is both beautiful and powerful has become the pursuit of every developer. As a popular front-end framework, Bootstrap provides us with rich components and styles, making interface design extremely simple. JavaScript is the soul of web page dynamic functions, which can turn static pages into an interactive experience. This article will take you into a deep understanding of how to seamlessly integrate Bootstrap and JavaScript to give your web page dynamic features and functions. By reading this article, you will learn how to use JavaScript to enhance Bootstrap components, enable complex user interactions, and avoid common integration problems.
Review of basic knowledge
Bootstrap is a front-end framework based on HTML and CSS. It provides a responsive mesh system, predefined styles and a rich component library, allowing you to quickly build beautiful interfaces. JavaScript is a dynamic scripting language in web development. It can operate DOM, process events, implement animations, etc., making the web page more vivid.
When integrating Bootstrap and JavaScript, you need to understand the component structure of Bootstrap and how to operate through JavaScript. For example, Bootstrap's modal box (Modal) can be displayed or hidden through JavaScript, and the Navbar can achieve dynamic expansion and contraction.
Core concept or function analysis
Integration of Bootstrap and JavaScript
Bootstrap itself contains some JavaScript plug-ins, such as modal boxes, tooltips, pop-up boxes, etc. These plug-ins are implemented through JavaScript. The key to integrating Bootstrap and JavaScript is how to manipulate these components with JavaScript, and how to add more dynamic capabilities to these components.
For example, suppose you want to display a modal box when the user clicks a button, you can do this:
<!-- HTML --> <button id="myBtn">Open the modal box</button> <!-- Modal --> <div class="modal fade" id="myModal" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog" role="document"> <div class="modal-content"> <div class="modal-header"> <h5 id="Modal-box-title">Modal box title</h5> <button type="button" class="close" data-dismiss="modal" aria-label="Close"> <span aria-hidden="true">×</span> </button> </div> <div class="modal-body"> Modal box content</div> <div class="modal-footer"> <button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button> <button type="button" class="btn btn-primary">Save changes</button> </div> </div> </div> </div> <!-- JavaScript --> <script> document.getElementById('myBtn').addEventListener('click', function() { $('#myModal').modal('show'); }); </script>
In this example, we use JavaScript to listen for button click events and call Bootstrap's modal box display method through jQuery. This shows how to integrate JavaScript and Bootstrap components.
How it works
Bootstrap's JavaScript plugin relies on jQuery, which means you need to make sure jQuery has been introduced into your project. The components of Bootstrap are defined by specific HTML structures and CSS classes, while JavaScript selects and manipulates them through these classes. For example, the display and hiding of modal boxes are implemented by operating the .modal
class.
In actual development, you may encounter some problems, such as JavaScript code execution order issues, component initialization failure, etc. One key to solving these problems is to make sure your JavaScript code is executed after the DOM is fully loaded and that all dependencies (such as jQuery) are loaded correctly.
Example of usage
Basic usage
The most common usage is to use JavaScript to control the expansion and contraction of Bootstrap's navigation bar:
<!-- HTML --> <nav class="navbar navbar-expand-lg navbar-light bg-light"> <a class="navbar-brand" href="#">Navbar</a> <button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation"> <span class="navbar-toggler-icon"></span> </button> <div class="collapse navbar-collapse" id="navbarSupportedContent"> <ul class="navbar-nav mr-auto"> <li class="nav-item active"> <a class="nav-link" href="#">Home <span class="sr-only">(current)</span></a> </li> <li class="nav-item"> <a class="nav-link" href="#">Link</a> </li> </ul> </div> </nav> <!-- JavaScript --> <script> document.querySelector('.navbar-toggler').addEventListener('click', function() { document.querySelector('.navbar-collapse').classList.toggle('show'); }); </script>
In this example, we listen to the click event of the navigation bar toggle button through JavaScript, and implement the expansion and contraction of the navigation bar by operating navbar-collapse
class.
Advanced Usage
In some complex scenarios, you may need to add more dynamic effects to the components of Bootstrap. For example, you can use JavaScript to implement a dynamic form verification function:
<!-- HTML --> <form id="myForm"> <div class="form-group"> <label for="email">Email address</label> <input type="email" class="form-control" id="email" aria-describedby="emailHelp" placeholder="Enter email"> <small id="emailHelp" class="form-text text-muted">We'll never share your email with anyone else.</small> </div> <button type="submit" class="btn btn-primary">Submit</button> </form> <!-- JavaScript --> <script> document.getElementById('myForm').addEventListener('submit', function(event) { event.preventDefault(); var email = document.getElementById('email').value; if (email === '') { alert('Please enter your email address'); } else if (!isValidEmail(email)) { alert('Please enter a valid email address'); } else { alert('Form submission successful'); } }); function isValidEmail(email) { var re = /^(([^<>()\[\]\\.,;:\s@"] (\.[^<>()\[\]\\.,;:\s@"] )*)|(". "))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9] \.) [a-zA-Z]{2,}))$/; return re.test(String(email).toLowerCase()); } </script>
In this example, we implement a simple form verification function through JavaScript, check whether the email address entered by the user is valid, and display different prompt information based on the verification results.
Common Errors and Debugging Tips
Common problems when integrating Bootstrap and JavaScript include JavaScript code execution order issues, component initialization failures, style conflicts, etc. Here are some debugging tips:
- Check the order in which JavaScript code is executed : Make sure your JavaScript code is executed after the DOM is fully loaded, you can use the
DOMContentLoaded
event to ensure this. - Make sure all dependencies are loaded : Bootstrap's JavaScript plugin relies on jQuery to make sure jQuery is loaded correctly.
- Developer Tools for Browser : The developer tools for Browser can help you view and debug JavaScript code, check console output to find error messages.
Performance optimization and best practices
In practical applications, it is very important to optimize the performance of Bootstrap and JavaScript integration. Here are some optimization suggestions:
- Reduce DOM operations : Frequent DOM operations can affect performance and minimize unnecessary DOM operations.
- Using Event Delegates : For dynamically added elements, using Event Delegates can reduce the number of event listeners and improve performance.
- Optimize JavaScript code : minimize unnecessary calculations and loops, and use efficient algorithms and data structures.
When writing code, it is also very important to keep the code readable and maintained. Here are some best practices:
- Use meaningful variable and function names : Clear naming can improve the readability of your code.
- Add comments : Proper comments can help other developers understand your code.
- Follow a consistent code style : Keeping a consistent code style can improve team collaboration efficiency.
Through the study of this article, you should have mastered how to integrate Bootstrap and JavaScript to achieve dynamic web page functions. Hope these knowledge and tips work in your project and have a great development!
The above is the detailed content of Bootstrap & JavaScript Integration: Dynamic Features & Functionality. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


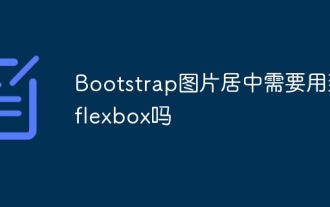
There are many ways to center Bootstrap pictures, and you don’t have to use Flexbox. If you only need to center horizontally, the text-center class is enough; if you need to center vertically or multiple elements, Flexbox or Grid is more suitable. Flexbox is less compatible and may increase complexity, while Grid is more powerful and has a higher learning cost. When choosing a method, you should weigh the pros and cons and choose the most suitable method according to your needs and preferences.
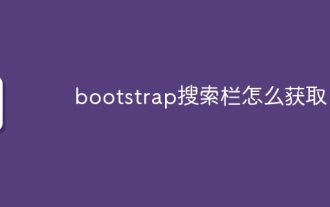
How to use Bootstrap to get the value of the search bar: Determines the ID or name of the search bar. Use JavaScript to get DOM elements. Gets the value of the element. Perform the required actions.
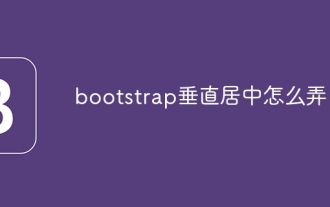
Use Bootstrap to implement vertical centering: flexbox method: Use the d-flex, justify-content-center, and align-items-center classes to place elements in the flexbox container. align-items-center class method: For browsers that do not support flexbox, use the align-items-center class, provided that the parent element has a defined height.

Article discusses customizing Bootstrap's appearance and behavior using CSS variables, Sass, custom CSS, JavaScript, and component modifications. It also covers best practices for modifying styles and ensuring responsiveness across devices.
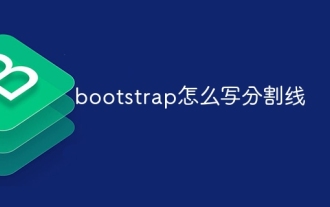
There are two ways to create a Bootstrap split line: using the tag, which creates a horizontal split line. Use the CSS border property to create custom style split lines.
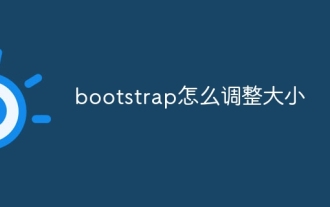
To adjust the size of elements in Bootstrap, you can use the dimension class, which includes: adjusting width: .col-, .w-, .mw-adjust height: .h-, .min-h-, .max-h-
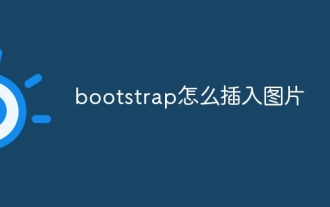
There are several ways to insert images in Bootstrap: insert images directly, using the HTML img tag. With the Bootstrap image component, you can provide responsive images and more styles. Set the image size, use the img-fluid class to make the image adaptable. Set the border, using the img-bordered class. Set the rounded corners and use the img-rounded class. Set the shadow, use the shadow class. Resize and position the image, using CSS style. Using the background image, use the background-image CSS property.
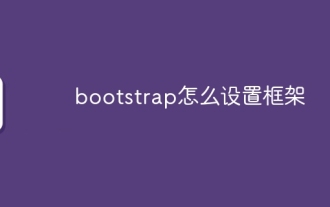
To set up the Bootstrap framework, you need to follow these steps: 1. Reference the Bootstrap file via CDN; 2. Download and host the file on your own server; 3. Include the Bootstrap file in HTML; 4. Compile Sass/Less as needed; 5. Import a custom file (optional). Once setup is complete, you can use Bootstrap's grid systems, components, and styles to create responsive websites and applications.
