Building a Real-Time Chat App with React and Firebase
This tutorial demonstrates building a real-time chat application using React and Firebase, focusing on user authentication. We'll integrate third-party authentication providers (Google, GitHub) and leverage Firebase's Realtime Database for real-time data synchronization. While built with React, the core concepts are transferable to other frameworks.
Understanding Firebase
Firebase, Google's app development platform, offers a comprehensive suite of tools, including authentication, a real-time NoSQL database, cloud functions, static hosting, and cloud storage. Its generous free tier is perfect for this project, providing authentication (email/password, Google, GitHub) and Realtime Database access (with limitations on concurrent connections and storage).
Key Firebase features used in this application:
- Authentication: Secure user login via email/password and single sign-on (SSO) with Google and GitHub.
- Realtime Database: A NoSQL database enabling real-time data updates.
The Chatty Application
Our application, "Chatty," allows authenticated users to send and receive messages. Users can register with email/password or use Google/GitHub SSO. The source code is available for reference.
Setup and Configuration
- Firebase Project: Create a new project in the Firebase console. Name it (e.g., "Chatty"). You can optionally enable analytics.
- Web App Setup: In the Firebase console, navigate to the "Web" section and register your app. Record the configuration details (API key, auth domain, etc.) – you'll need these later.
-
Authentication Providers: Enable email/password, Google, and GitHub sign-in methods in the Firebase Authentication settings. Remember to add your web app's domain (e.g.,
localhost
) for authorized redirects.
Development Environment
- Node.js and npm (or yarn): Ensure Node.js and a package manager (npm or yarn) are installed.
-
Create React App: Bootstrap a new React project:
npx create-react-app chatty
-
Install Dependencies: Install React Router and Firebase:
yarn add react-router-dom firebase
(or usenpm install
if using npm). - Project Structure: Organize your project with folders for components, helpers, pages, and services.
Integrating Firebase
-
Firebase Configuration (
src/services/firebase.js
): Import Firebase and initialize it using your configuration details from step 2 of the setup. Export theauth
anddatabase
modules. -
App Setup (
src/App.js
): Import necessary modules (React, React Router, Firebase). CreatePrivateRoute
andPublicRoute
higher-order components (HOCs) to handle authentication-based routing. These HOCs will check the authentication state and redirect users accordingly. -
Authentication State (
src/App.js
): Useauth().onAuthStateChanged
to monitor authentication changes and update the app's state (authenticated
,loading
). -
Authentication Helpers (
src/helpers/auth.js
): Create functions for signup (signup
), signin (signin
), Google sign-in (signInWithGoogle
), and GitHub sign-in (signInWithGitHub
). These functions will interact with the Firebase authentication service. -
Signup and Login Pages (
src/pages/Signup.js
,src/pages/Login.js
): Create forms for user registration and login, using the helper functions fromsrc/helpers/auth.js
. Handle form submissions and display error messages. -
Chat Page (
src/pages/Chat.js
): Usedb.ref("chats").on("value", ...)
to listen for real-time updates from the Firebase Realtime Database. Render the chat messages and provide a form for sending new messages. Usedb.ref("chats").push(...)
to add new messages to the database. Implement error handling for both reading and writing data. -
Database Rules: Configure Firebase Realtime Database rules to restrict access to authenticated users only.
Testing and Deployment
Once completed, test the application thoroughly. Deployment will depend on your chosen hosting provider.
This detailed guide provides a comprehensive walkthrough of building a secure and real-time chat application using React and Firebase. Remember to consult the Firebase documentation for more advanced features and options.
The above is the detailed content of Building a Real-Time Chat App with React and Firebase. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
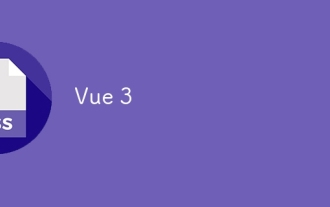
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
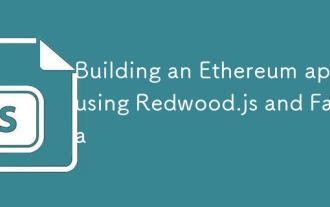
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
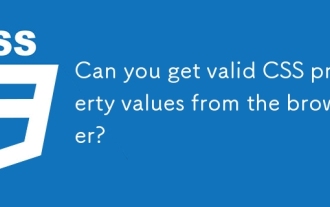
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
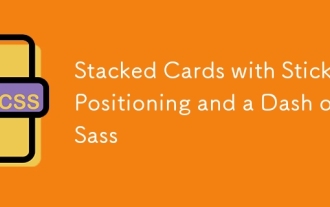
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
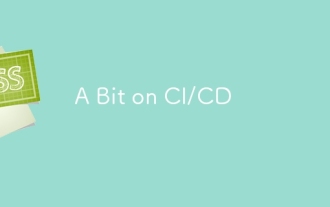
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
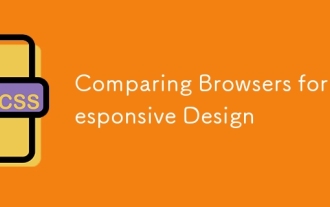
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
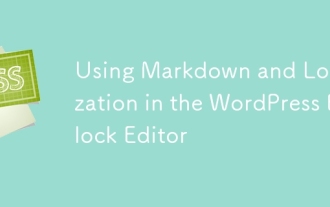
If we need to show documentation to the user directly in the WordPress editor, what is the best way to do it?
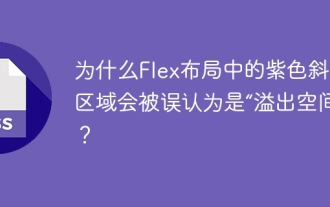
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
