Dijkstra Algorithm in Python
Mastering Dijkstra's Algorithm in Python: Finding the Shortest Path
This tutorial guides you through implementing Dijkstra's Algorithm in Python to efficiently find the shortest paths in a weighted graph. Understanding this algorithm is crucial for various applications, from GPS navigation to network routing.
Key Learning Objectives:
- Grasp the core principles of Dijkstra's Algorithm.
- Implement Dijkstra's Algorithm effectively in Python.
- Manage weighted graphs and compute shortest paths between nodes.
- Optimize the algorithm for enhanced performance in Python.
- Apply your knowledge by solving a practical shortest path problem.
Table of Contents:
- What is Dijkstra's Algorithm?
- Fundamental Concepts of Dijkstra's Algorithm
- Implementing Dijkstra's Algorithm
- Optimizing Dijkstra's Algorithm
- Real-World Applications
- Avoiding Common Pitfalls
- Frequently Asked Questions
What is Dijkstra's Algorithm?
Dijkstra's Algorithm is a greedy algorithm that determines the shortest path from a single source node to all other nodes in a graph with non-negative edge weights. It iteratively expands the set of nodes with known shortest distances from the source, selecting the node with the minimum distance at each step.
Here's a simplified explanation:
- Assign a tentative distance to each node: 0 for the source, infinity for others.
- Mark the source node as current. Mark all other nodes as unvisited.
- For the current node, examine all unvisited neighbors. Calculate their tentative distances via the current node. If this distance is shorter than the existing tentative distance, update it.
- Mark the current node as visited.
- Select the unvisited node with the smallest tentative distance as the new current node. Repeat steps 3-5 until all nodes are visited or the shortest distance to the target node is found.
Fundamental Concepts:
- Graph Representation: Nodes and edges represent the graph. Each edge has a non-negative weight (distance or cost).
-
Priority Queue: A priority queue (like Python's
heapq
) efficiently selects the node with the minimum tentative distance. - Greedy Approach: The algorithm expands the set of nodes with known shortest distances by selecting the nearest unvisited node.
Implementing Dijkstra's Algorithm:
We'll represent the graph as a dictionary: keys are nodes, values are lists of (neighbor, weight) tuples.
Step 1: Graph Initialization
graph = { 'A': [('B', 1), ('C', 4)], 'B': [('A', 1), ('C', 2), ('D', 5)], 'C': [('A', 4), ('B', 2), ('D', 1)], 'D': [('B', 5), ('C', 1)] }
Step 2: Algorithm Implementation
import heapq def dijkstra(graph, start): distances = {node: float('inf') for node in graph} distances[start] = 0 pq = [(0, start)] while pq: current_distance, current_node = heapq.heappop(pq) if current_distance > distances[current_node]: continue for neighbor, weight in graph[current_node]: distance = current_distance weight if distance <p><strong>Step 3: Running the Algorithm</strong></p> <pre class="brush:php;toolbar:false">start_node = 'A' shortest_paths = dijkstra(graph, start_node) print(f"Shortest paths from {start_node}: {shortest_paths}")
Step 4: Understanding the Output
The output shows the shortest distance from the starting node ('A') to all other nodes.
Example of Dijkstra's Algorithm:
This example visually demonstrates the step-by-step process, showing how the algorithm iteratively finds the shortest paths.
Optimizing Dijkstra's Algorithm:
- Early Stopping: Stop when the target node's shortest distance is found.
- Bidirectional Search: Run Dijkstra's from both source and destination simultaneously.
- Efficient Data Structures: Use Fibonacci heaps for extremely large graphs.
Real-World Applications:
- GPS Navigation: Finding optimal routes.
- Network Routing: Determining efficient data packet paths.
- Robotics: Path planning for robots.
- Game Development: NPC pathfinding.
Avoiding Common Pitfalls:
- Negative Edge Weights: Dijkstra's doesn't work with negative weights. Use Bellman-Ford instead.
-
Inefficient Priority Queue: Use
heapq
or Fibonacci heaps. - Memory Overhead: Optimize graph representation for large graphs.
Conclusion:
Dijkstra's Algorithm is a powerful tool for solving shortest path problems in graphs with non-negative weights. This tutorial provides a solid foundation for understanding and implementing this algorithm in Python.
Frequently Asked Questions:
Q1: What graph types does Dijkstra's handle? A: Graphs with non-negative edge weights.
Q2: Does it work with directed graphs? A: Yes.
Q3: Time complexity? A: O((V E) log V) with a binary heap.
Q4: Is it a greedy algorithm? A: Yes.
The above is the detailed content of Dijkstra Algorithm in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
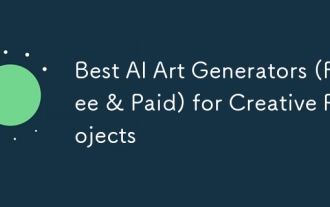
The article reviews top AI art generators, discussing their features, suitability for creative projects, and value. It highlights Midjourney as the best value for professionals and recommends DALL-E 2 for high-quality, customizable art.
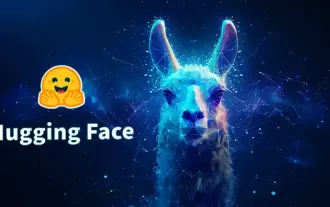
Meta's Llama 3.2: A Leap Forward in Multimodal and Mobile AI Meta recently unveiled Llama 3.2, a significant advancement in AI featuring powerful vision capabilities and lightweight text models optimized for mobile devices. Building on the success o

The article compares top AI chatbots like ChatGPT, Gemini, and Claude, focusing on their unique features, customization options, and performance in natural language processing and reliability.
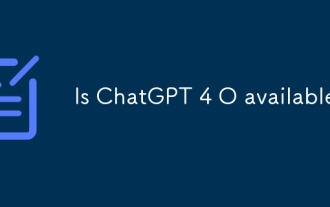
ChatGPT 4 is currently available and widely used, demonstrating significant improvements in understanding context and generating coherent responses compared to its predecessors like ChatGPT 3.5. Future developments may include more personalized interactions and real-time data processing capabilities, further enhancing its potential for various applications.
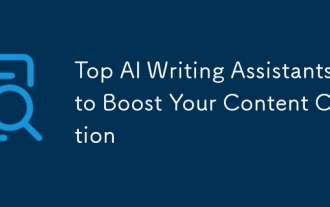
The article discusses top AI writing assistants like Grammarly, Jasper, Copy.ai, Writesonic, and Rytr, focusing on their unique features for content creation. It argues that Jasper excels in SEO optimization, while AI tools help maintain tone consist
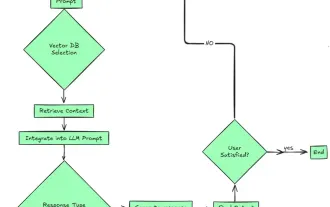
2024 witnessed a shift from simply using LLMs for content generation to understanding their inner workings. This exploration led to the discovery of AI Agents – autonomous systems handling tasks and decisions with minimal human intervention. Buildin
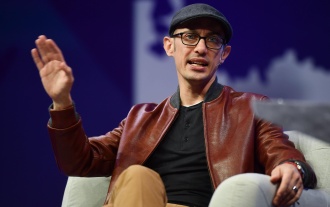
Shopify CEO Tobi Lütke's recent memo boldly declares AI proficiency a fundamental expectation for every employee, marking a significant cultural shift within the company. This isn't a fleeting trend; it's a new operational paradigm integrated into p
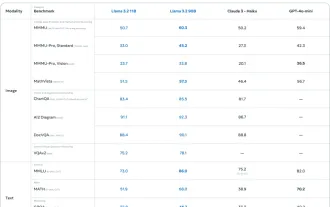
This week's AI landscape: A whirlwind of advancements, ethical considerations, and regulatory debates. Major players like OpenAI, Google, Meta, and Microsoft have unleashed a torrent of updates, from groundbreaking new models to crucial shifts in le
