Tips for Writing Animation Code Efficiently
I've been working on web animation coding for years and helping others do the same. However, I haven't seen a clean list of tips that focus on how to build animations efficiently , so let's share it now!
I will use GreenSock Animation Platform (GSAP). It provides a simple and easy-to-read API and addresses cross-browser inconsistencies so that you can focus on animation production. Even if you have never used GSAP, the code and concepts should be easy to understand. If you want to familiarize yourself with the basics of GSAP first, in order to make the most of this article, the best starting point is the entry page for GSAP (including videos).
Tips #1: Use the animation library
Some developers think using animation libraries is a waste because they can do the same with native browser technologies such as CSS transitions, CSS animations, or Web Animations API (WAAPI) without loading the library. In some cases, this is correct. However, here are some other factors to consider:
- Browser Errors, Inconsistencies, and Compatibility: Animation libraries like GSAP solve these problems for you and have universal compatibility. You can even use motion paths in IE9! There are many problem areas when it comes to cross-browser issues, including handling transform-origin on SVG elements, path stroke measurements, 3D origins in Safari, and more issues we don't have space to list.
- Animation workflow: It is much faster and more fun to build medium-complex animations using tools like GSAP. You can modularly animation, nest them as needed, and automatically adjust their timing. This makes experiments much easier. Trust me: Once you try to build an animation sequence with CSS and then build with GSAP, you will understand what I mean. A world of difference! Future editing will also be faster.
- Animation beyond DOM: Canvas, WebGL, common objects, and complex strings cannot be animated using native techniques. It is easier to use a consistent tool for all animations.
- Runtime Control: Using a good animation library allows you to pause, resume, invert, search, or even gradually change the speed of the entire animation sequence. You can independently control each transform component (rotate, zoom, x, y, tilt, etc.). You can also retrieve these values at any time. JavaScript animations provide you with the greatest flexibility.
- Easing options (rebound, elastic, etc.): CSS only provides you with two easing control points. GSAP's CustomEase allows you to create any easing effect you can imagine.
- Hysteresis Smoothing: If the CPU load is too heavy, GSAP can prioritize absolute timing or dynamic adjustments to avoid jumps.
- Advanced features: Using GSAP, you can easily deform SVG, add physics/inertia, edit motion paths directly in the browser, use position-aware interleaving, and more.
Most of the top animators in the industry use tools like GSAP because they have learned about these same things over the years. Once you go beyond very basic animations, the JavaScript library will make your life much easier and open up entirely new possibilities.
Tips #2: Use the Timeline
A good animation library will provide some ways to create a single animation (called tween ), as well as ways to sort the animations in the timeline . Think of the timeline as a container of tween where you can locate them according to each other's position.
Most of the time, if you need animations to run in order, you should use the timeline.
const tl = gsap.timeline(); tl.to(".box", { duration: 1, x: 100 }) .to(".box", { duration: 1, backgroundColor: "#f38630" }, " =0.5") .to(".box", { duration: 1, x: 0, rotation: -360 }, " =0.5")
In GSAP, by default, tween added to the timeline will wait for the previous tween to complete before running. =0.5 also adds an additional offset or delay of half a second, so no matter how long the first tween lasts, the second tween will start after 0.5 seconds after the first tween is completed.
To increase the time between tween to 1 second, you just need to change =0.5 to =1! Very simple. With this approach, you can iterate through the animation quickly without worrying about calculating the duration before the combination.
Tips #3: Use Relative Values
"Relative value" refers to three things:
- Animate the value relative to its current value. GSAP recognizes the = and -= prefixes. So x: "=200" will add 200 units (usually pixels) to the current x. And x: "-=200" will subtract 200 from the current value. This is also useful in GSAP position parameters, when tweens are positioned relative to each other.
- When the value needs to respond to viewport size changes, relative units (such as vw, vh, and % in some cases) are used.
- Use the .to() and .from() methods whenever possible (rather than .fromTo()) so that the start or end values are dynamically filled from their current value. This way, you don't need to declare the start and end values in each tween. Yes, typing less! For example, if you have a bunch of elements of different colors, you can animate them all to black, for example gsap.to(".class", {backgroundColor: "black" }).
Tips #4: Use Keyframes
If you find yourself animate the same target repeatedly over and over again, this is the best time to use keyframes! You can do it like this:
gsap.to(".box", { keyframes: [ { duration: 1, x: 100 }, { duration: 1, backgroundColor: "#f38630", delay: 0.5 }, { duration: 1, x: 0, rotation: -360, delay: 0.5 } ]});
No timeline is needed! To space tween, we just need to use the delay property in each keyframe. (It can be a negative number to create overlap.)
Tips #5: Use smart defaults
GSAP has default values for properties such as ease ("power1.out") and duration (0.5 seconds). Therefore, the following is an effective tween that lasts the animation for half a second.
gsap.to(".box", { color: "black" })
To change the global default value of GSAP, use gsap.defaults():
// Use linear easing and duration 1 gsap.defaults({ ease: "none", duration: 1 });
This may be convenient, but it is more common to set default values for a specific timeline so that it only affects its children. For example, we can avoid typing duration: 1 for each child tween by setting the default value on the parent timeline:
const tl = gsap.timeline({ defaults: { duration: 1 } }); tl.to(".box", { x: 100 }) .to(".box", { backgroundColor: "#f38630" }, " =0.5") .to(".box", { x: 0, rotation: -360 }, " =0.5")
Tips #6: Animate multiple elements at once
We briefly mentioned this in the third trick, but it deserves its own trick.
If you have multiple elements that share the same .box class, the above code will animate all elements at the same time!
You can also use more complex selector strings to select multiple elements with different selectors:
gsap.to(".box, .circle", { ... });
Alternatively, you can pass an array of variable references as long as the elements are of the same type (selector string, variable reference, general object, etc):
var box = document.querySelector(".box"); var circle = document.querySelector(".circle"); // sometimes later… gsap.to([box, circle], { ... });
Tips #7: Use function-based values, interleaving, and/or loops
Function-based value
For almost any property, a function is used instead of a number/string, and GSAP will call that function once for each target when the first renders the tween. Also, it will use whatever the function returns as the property value! This is very convenient for creating many different animations with a single tween and adding differences.
GSAP passes the following parameters into the function:
- index
- Affected specific elements
- Array of all elements affected by tween
For example, you can set the direction of movement based on the index:
Or you can select items from the array:
staggered
Make your animation look more dynamic and interesting by using interleaving to offset the start time. For simple interleaved offsets in a single tween, just use stagger: 0.2 to add 0.2 seconds between the start time of each animation.
You can also pass an object to get more complex interleaving effects, including interleaving effects that diverge outward from the center of the mesh or randomization time:
For more information about GSAP interleaving, check out the interleaving documentation.
cycle
It can be useful to loop through the list of elements to create or apply animations, especially if they are based on certain events (such as user interactions (I will discuss later).
The easiest way to loop through the project list is to use .forEach(). But since this is not supported on elements selected using .querySelectorAll() in IE, you can use GSAP's utils.toArray() function instead.
In the example below, we are looping through each container to add scope to the animation of the container to its child elements.
Tips #8: Modularize your animations
Modularity is one of the key principles of programming. It allows you to build small, easy to understand blocks that you can combine into larger works while keeping the code concise, reusable and easy to modify. It also allows you to use parameters and function scopes, thereby improving the reusability of your code.
function
Use the function to return to the tween or timeline and insert them into the main timeline:
function doAnimation() { // Do something, such as calculation, maybe using parameters passed into the function // Return a tween, maybe using the above calculation return gsap.to(".myElem", { duration: 1, color: "red"}); } tl.add( doAnimation() );
Nested timelines can really change the way you animations. It allows you to easily sort very complex animations while keeping your code modular and readable.
function doAnimation() { const tl = gsap.timeline(); tl.to(...); tl.to(...); // ...as many animations as you'd like! // When you complete all operations, return to the timeline return tl; } const master = gsap.timeline(); master.add( doAnimation() ); master.add( doAnotherAnimation() ); // Add more timelines!
Here is a modified practical use case from Carl Schoolff's "Writing Smarter Animated Code" post.
Here is a more complex demonstration that demonstrates the same technique using Craig Roblewsky's Star Wars theme:
Wrapping an animation build routine in a function also makes it easy to recreate animations (for example, after resizing)!
var tl; // Keep accessible reference to the timeline function buildAnimation() { var time = tl ? tl.time() : 0; // Save the old time (if it exists) // If present, close the old timeline if (tl) { tl.kill(); } // Create a new timeline tl = gsap.timeline(); tl.to(...) .to(...); // Execute the animation tl.time(time); // Set the playhead to match the previous} buildAnimation(); // Start window.addEventListener("resize", buildAnimation); // Handle resize
If you find yourself repeating the same code and swapping one variable for another, this usually means you should create a general function or use a loop instead to keep the code DRY (don't repeat yourself).
Effect
With effects, you can convert custom animations to named effects, which can be called at any time using new targets and configurations. This is especially useful when you have standards for animations or want to call the same animation from different contexts.
Here is a super simple "fade out" effect to demonstrate the concept:
// Use GSAP to register the effect: gsap.registerEffect({ name: "fade", defaults: {duration: 2}, // The default value is applied to the "config" object passed to the effect: (targets, config) => { return gsap.to(targets, {duration: config.duration, opacity:0}); } }); // Now we can use it like this: gsap.effects.fade(".box"); // Or override the default value: gsap.effects.fade(".box", {duration: 1});
Tips #9: Use Control Methods
GSAP provides many ways to control the state of the tween or timeline. They include .play(), .pause(), .reverse(), .progress(), .seek(), .restart(), .timeScale(), and some other methods.
Using control methods can make transitions between animations smoother (e.g. able to reverse midway) and perform better (by reusing the same tween/timeline instead of creating a new instance every time). And it also helps debugging by giving you more granular control of the state of the animation.
Here is a simple example:
An amazing use case is to adjust the timeScale of the timeline!
Use case: Interactive events that trigger animation
In the event listener of user interaction events, we can use control methods to finely control the playback status of the animation.
In the example below, we create a timeline for each element (so that it does not trigger the same animation for all instances), append the reference to that timeline to the element itself, and then play the relevant timeline when the element is hovered, and reverse it when the mouse leaves.
Use case: Animate between multiple states in the timeline
You might want a set of animations to affect the same properties of the same element, but only in some sequences (e.g. active/inactive state, each with a mouseover/mouse-out state). It can be tricky to manage. We can simplify it by using the state of the timeline and controlling events.
Use case: Animation based on scroll position
We can easily trigger animations based on scroll position by using control methods. For example, this demonstration plays the full animation after reaching the scroll position:
You can also attach the progress of the animation to the scroll position for a more refined scrolling effect!
However, if you want to do this, it is better to limit the scroll listener for performance reasons:
However, setting this operation is cumbersome every time you need to animate while scrolling. You can do this for you (and more) using GreenSock's official scrolling plugin ScrollTrigger!
Additional tips: Plugins, utility methods and helper functions using GSAP
The GSAP plugin adds extra functionality to the core of GSAP. Some plugins make it easier to use rendering libraries such as PixiJS or EaselJS, while others add superpowers such as deformation SVG, drag and drop capabilities. This keeps the GSAP core relatively small and can add functionality when needed.
Plugin
ScrollTrigger creates amazing scroll-based animations with minimal code. Or trigger any scroll-related event, even if it has nothing to do with the animation.
MorphSVG deforms between any two SVG shapes, regardless of the number of points, and allows you to finely control how the shape is deformed.
DrawSVG step by step displays (or hides) the strokes of the SVG element so that it looks like it is being drawn. It resolves various browser errors that affect typical stroke-dashoffset animations.
MotionPath animations any content (SVG, DOM, canvas, general objects, etc.) along the motion path in any browser. You can even use MotionPathHelper to edit the path in your browser!
GSDevTools provides you with a visual UI for interacting and debugging with GSAP animations, including advanced playback controls, keyboard shortcuts, global synchronization, and more.
Draggable provides a very simple way to make almost any DOM element draggable, rotate, throwable, and even scroll quickly using a mouse or touch event. Draggable is perfectly integrated with InertiaPlugin (optional), so users can flick and smoothly decelerate their movements according to momentum.
CustomEase (along with CustomBounce and CustomWiggle) enhances GSAP's already extensive easing features by enabling you to register any easing effect you want.
SplitText is an easy-to-use JavaScript utility that allows you to split HTML text into characters, words, and lines. It is easy to use, very flexible, goes back to IE9 and handles special characters for you.
ScrambleText uses random characters to scramble text in DOM elements, refreshing new random characters at regular intervals, while gradually displaying new text (or original text) during the tween process. Visually, it looks like a computer is decoding a string of text.
Physics2D allows you to animate the position of an element based on speed and acceleration, rather than going to a specific value. PhysicsProps is similar, but works for any property, not just two-dimensional coordinates.
Utility Methods
GSAP has built-in utility methods that can make certain common tasks easier. Most focus on manipulating values in a specific way, which is especially useful when generating or modifying animation values. I often use .wrap(), .random, .interpolate(), .distribute(), .pipe(), and .unitize(), but there are many other ways you might find useful.
Helper functions
Similarly, but not built into the core of GSAP, it is some helper functions that GreenSock has created over the years to handle specific use cases. These functions make it easy to flip animations easily, return random numbers based on easing curves, mix two easing curves, and more. I highly recommend you check it out!
in conclusion
You're done! Hopefully you have learned something along the way and this article will continue to be your resource for years to come.
As always, if you have any questions about GSAP, feel free to visit the GreenSock forum. They are very helpful and welcoming! As a GreenSock employee, I often hang out there; I love helping people solve challenges related to animation!
The above is the detailed content of Tips for Writing Animation Code Efficiently. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


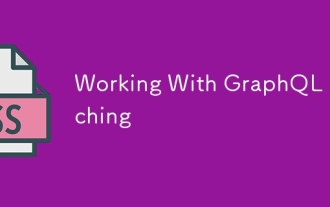
If you’ve recently started working with GraphQL, or reviewed its pros and cons, you’ve no doubt heard things like “GraphQL doesn’t support caching” or
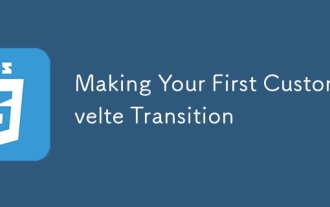
The Svelte transition API provides a way to animate components when they enter or leave the document, including custom Svelte transitions.
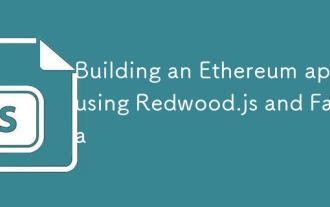
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
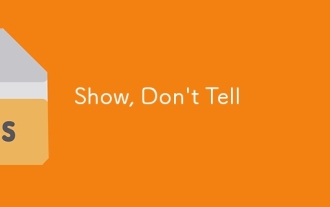
How much time do you spend designing the content presentation for your websites? When you write a new blog post or create a new page, are you thinking about
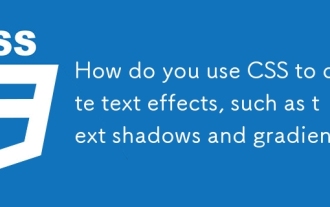
The article discusses using CSS for text effects like shadows and gradients, optimizing them for performance, and enhancing user experience. It also lists resources for beginners.(159 characters)
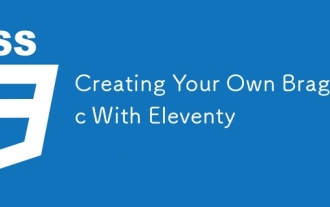
No matter what stage you’re at as a developer, the tasks we complete—whether big or small—make a huge impact in our personal and professional growth.
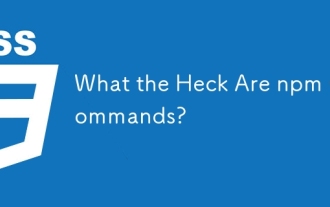
npm commands run various tasks for you, either as a one-off or a continuously running process for things like starting a server or compiling code.
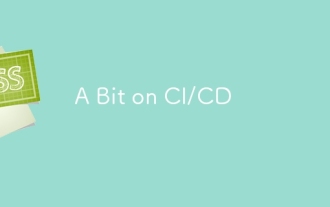
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
