Modern C Design Patterns: Building Scalable and Maintainable Software
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
introduction
In today's world of software development, C remains the preferred language in many fields, especially in scenarios where high performance and low-level control are required. However, as the complexity of software systems continues to increase, how to design scalable and easy-to-maintain software has become a key challenge. This article will dive into modern C design patterns to help you build more scalable and maintainable software. By reading this article, you will learn how to leverage the modern nature of C to implement classic and emerging design patterns and gain practical experience and insights from it.
Review of basic knowledge
Before we dive into the design pattern, let's review some of the key features of C that are crucial when implementing the design pattern. C provides rich language features, such as classes and objects, templates, metaprogramming, smart pointers, etc., which are the basis for building design patterns. For example, templates can help us implement generic programming, while smart pointers can simplify memory management and reduce the risk of memory leaks.
Core concept or function analysis
The definition and function of modern C design patterns
Modern C design pattern refers to a design pattern implemented using new features introduced in C 11 and later versions. These patterns not only inherit the advantages of classic design patterns, but also take advantage of the modern characteristics of C, such as lambda expressions, auto keywords, mobile semantics, etc., making the code more concise and expressive. Their role is to help developers build more flexible and efficient software systems.
For example, consider a simple observer pattern implementation:
#include <iostream> #include <vector> #include <functional> class Subject { public: void attach(std::function<void()> observer) { observers.push_back(observer); } void notify() { for (auto& observer : observers) { observer(); } } private: std::vector<std::function<void()>> observers; }; int main() { Subject subject; subject.attach([]() { std::cout << "Observer 1 notified\n"; }); subject.attach([]() { std::cout << "Observer 2 notified\n"; }); subject.notify(); return 0; }
In this example, we use lambda expressions and std::function
to implement the observer pattern, making the code more concise and flexible.
How it works
The working principle of modern C design patterns relies on the new features of C. For example, using moving semantics can reduce unnecessary copy operations and improve performance; using lambda expressions can simplify the definition and use of callback functions; using auto
keywords can reduce type declarations and improve code readability.
When implementing a design pattern, we need to consider the following aspects:
- Type safety : Use C's strong type system to ensure the type safety of the code.
- Performance optimization : Use mobile semantics, perfect forwarding and other features to optimize the performance of the code.
- Code simplicity : Use lambda expressions, auto keywords and other features to simplify code and improve readability.
Example of usage
Basic usage
Let's look at a simple factory model implementation:
#include <memory> #include <string> class Product { public: virtual ~Product() = default; virtual std::string getName() const = 0; }; class ConcreteProductA : public Product { public: std::string getName() const override { return "Product A"; } }; class ConcreteProductB : public Product { public: std::string getName() const override { return "Product B"; } }; class Factory { public: static std::unique_ptr<Product> createProduct(const std::string& type) { if (type == "A") { return std::make_unique<ConcreteProductA>(); } else if (type == "B") { return std::make_unique<ConcreteProductB>(); } return nullptr; } }; int main() { auto productA = Factory::createProduct("A"); auto productB = Factory::createProduct("B"); if (productA) std::cout << productA->getName() << std::endl; if (productB) std::cout << productB->getName() << std::endl; return 0; }
In this example, we use std::unique_ptr
to manage the life cycle of the object, ensuring the safe release of resources.
Advanced Usage
Now let's look at a more complex example using policy patterns to implement different sorting algorithms:
#include <vector> #include <algorithm> #include <functional> template<typename T> class SortStrategy { public: virtual void sort(std::vector<T>& data) = 0; virtual ~SortStrategy() = default; }; template<typename T> class BubbleSort : public SortStrategy<T> { public: void sort(std::vector<T>& data) override { for (size_t i = 0; i < data.size(); i) { for (size_t j = 0; j < data.size() - 1 - i; j) { if (data[j] > data[j 1]) { std::swap(data[j], data[j 1]); } } } } }; template<typename T> class QuickSort : public SortStrategy<T> { public: void sort(std::vector<T>& data) override { std::sort(data.begin(), data.end()); } }; template<typename T> class Sorter { public: void setStrategy(std::unique_ptr<SortStrategy<T>> strategy) { this->strategy = std::move(strategy); } void sort(std::vector<T>& data) { if (strategy) { strategy->sort(data); } } private: std::unique_ptr<SortStrategy<T>> strategy; }; int main() { std::vector<int> data = {5, 2, 8, 1, 9}; Sorter<int> sorter; sorter.setStrategy(std::make_unique<BubbleSort<int>>()); sorter.sort(data); for (auto& num : data) std::cout << num << " "; std::cout << std::endl; data = {5, 2, 8, 1, 9}; sorter.setStrategy(std::make_unique<QuickSort<int>>()); sorter.sort(data); for (auto& num : data) std::cout << num << " "; std::cout << std::endl; return 0; }
In this example, we use templates and smart pointers to implement policy patterns, making the code more flexible and type-safe.
Common Errors and Debugging Tips
Common errors when using modern C design patterns include:
- Memory Leaks : While smart pointers can help us manage memory, if used improperly, it can still lead to memory leaks. For example, in factory mode, forgetting to use
std::unique_ptr
, may result in memory leaks. - Type mismatch : When using templates, if the type mismatch, it may result in a compilation error or a runtime error. For example, in policy mode, if the type passed in does not match the template parameter, it may result in a compilation error.
Methods to debug these problems include:
- Using memory checking tools such as Valgrind or AddressSanitizer can help us detect memory leaks and memory access errors.
- Static code analysis : Using static code analysis tools such as Clang Static Analyzer can help us detect potential type errors and code problems.
Performance optimization and best practices
When using modern C design patterns, we need to consider performance optimization and best practices. For example, when implementing observer mode, we can use std::vector
instead of std::list
because std::vector
performs better in most cases. At the same time, we can use std::move
to optimize the moving operations of objects and reduce unnecessary copies.
When writing code, we should follow the following best practices:
- Code readability : Use clear naming and comments to ensure that the code is easy to understand and maintain.
- Code reusability : Try to reuse existing code to reduce the writing of duplicate code.
- Test-driven development : Use unit tests to verify the correctness of the code and ensure the reliability of the code.
In short, the modern C design pattern provides us with a powerful tool to help us build more scalable and maintainable software. By rationally leveraging the modern features of C, we can write more efficient and easier to maintain code. I hope this article can provide you with valuable insights and practical experience to help you go further on the road of C programming.
The above is the detailed content of Modern C Design Patterns: Building Scalable and Maintainable Software. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


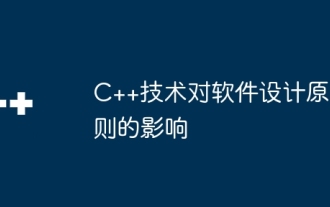
The impact of C++ on software design principles: encapsulation, data hiding: encapsulating data to improve security. Polymorphism, inheritance: object behavior changes according to type, code scalability. Synthesis and aggregation: ownership and inclusion relationships between objects to improve reusability. Dependency inversion: Reduce class coupling and achieve loose coupling through interfaces and injection.
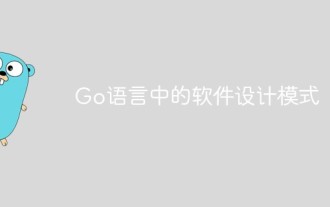
Go language is an efficient programming language that has developed rapidly in recent years. It is characterized by simplicity, efficiency, safety and ease of learning. The Go language provides a series of features and language structures that allow developers to write more robust software systems in a more efficient way. Software design patterns are designed to allow us to better design and implement high-quality codes and systems. This article will introduce commonly used software design patterns in the Go language. Factory Pattern Factory pattern is a pattern for creating objects. In the factory pattern, we can use a common interface or abstract class
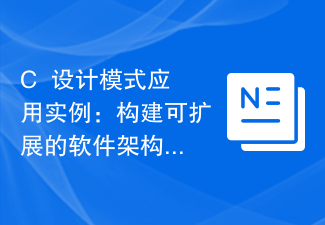
Application Example of C++ Design Pattern: Building a Scalable Software Architecture Introduction: As the scale of software systems continues to grow, the scalability of software architecture becomes particularly important. A good software architecture can meet changes in system requirements, reduce dependence on and modification of existing code, and provide development efficiency and code reusability. C++ design patterns have become an important tool for building scalable software architecture due to their flexibility and scalability. This article takes factory mode, observer mode and strategy mode as examples to analyze their application in actual projects and demonstrate C++ design
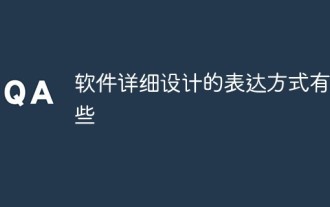
The expressions of detailed software design include: 1. Program flow chart, also known as program block diagram, which is a graphical representation that uses uniformly prescribed standard symbols to describe the specific steps of program operation; 2. PAD diagram (Problem Analysis Diagram), which is an algorithm description tool , is also a commonly used graphical tool in detailed design (software design); 3. Process design language, which is a language used to describe module algorithm design and processing details; 4. Box diagram, a diagram that forces the use of structured constructs Tool that can easily determine the scope of local and global data, and easily express nested relationships and hierarchical relationships of templates.
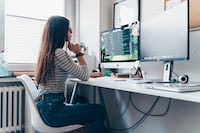
What are PHP design patterns? PHP design patterns are a set of general programming solutions for solving common software development problems. They provide a structured approach to solving common challenges such as creating reusable code, handling object interactions, and managing complexity. Types of PHP design patterns PHP design patterns are divided into three categories: Creation patterns: used to create objects, such as singleton pattern, factory method pattern and builder pattern. Structural patterns: used to organize and combine objects, such as adapter pattern, decorator pattern and composition pattern. Behavioral patterns: used to coordinate object interaction, such as command pattern, strategy pattern and observer pattern. Creational pattern example: Factory method pattern interfaceShapeInterfac
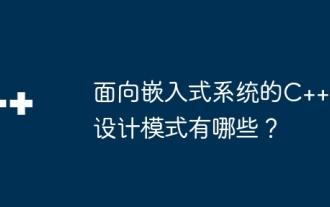
Embedded C++ design patterns can be used to create efficient and reliable code, suitable for resource-constrained environments: Singleton pattern: ensures that there is only one instance of a specific class used to manage resources. Observer Pattern: Allows objects to subscribe to other objects and receive notifications of state changes. Factory Method Pattern: Creates an object based on a type without specifying the exact class. Practical case: The task scheduling system uses these modes to achieve efficient task scheduling and ensure the reliable execution of key tasks.
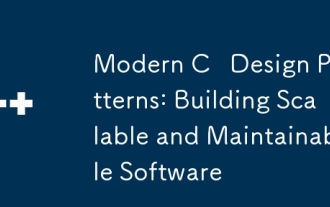
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
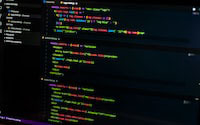
In the world of software development, MVC architecture has become a model for building maintainable and scalable applications. For PHP developers, the MVC architecture provides a structural and organizational framework that enables them to write reusable, testable, and maintainable code. Principles of MVC Architecture MVC architecture (Model-View-Controller) is a design pattern that decomposes the logic of an application into three independent components: Model: represents the data and business logic of the application. View: Responsible for displaying the application's data. Controller: Coordinates the interaction between the model and the view and manages user requests. MVC Architecture in PHP When implementing MVC architecture in PHP, the following structure is usually followed: //Model class class
