Demystifying JavaScript: What It Does and Why It Matters
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
introduction
Have you ever wondered how web pages become so vivid and interactive? The answer is likely to be JavaScript, which is the cornerstone of modern web development. This article will unveil JavaScript and explore what it does and why it is so important. By reading this article, you will learn about the basic concepts of JavaScript, its practical application, and why it is indispensable in today's online world.
The basics of JavaScript
JavaScript, referred to as JS, is a high-level and dynamic programming language that is mainly used to add interactive functions on web pages. It was originally developed by Brendan Eich in 1995 to enable web pages to be more than just static text and pictures, but to respond to user actions. The core of JavaScript is that it can manipulate HTML and CSS to achieve dynamic page effects.
For example, suppose you want to add a button on the web page and when the user clicks it, a welcome message pops up. You can use JavaScript like this:
document.getElementById('myButton').addEventListener('click', function() { alert('Welcome to JavaScript!'); });
This simple example shows how JavaScript interacts with HTML elements and performs specific tasks based on user actions.
The core features of JavaScript
Event-driven programming
One of the core of JavaScript is event-driven programming. Every user interaction on a web page, such as clicking, scrolling, or entering text, can be considered an event. JavaScript allows developers to listen to these events and execute corresponding code when the event occurs. This enables the web page to change dynamically according to the user's actions.
For example, when a user clicks a button, you can use JavaScript to change the text content on the page:
document.getElementById('changeTextButton').addEventListener('click', function() { document.getElementById('displayText').textContent = 'Text changed!'; });
Dynamic content generation
JavaScript can dynamically generate and modify web page content, which means developers can adjust page display based on user input or other conditions. For example, display different content based on the options selected by the user:
function showContent(selection) { let content; switch(selection) { case 'option1': content = 'You selected option 1'; break; case 'option2': content = 'You selected option 2'; break; default: content = 'Please select an option'; } document.getElementById('contentDisplay').textContent = content; }
Asynchronous programming
Another important feature of JavaScript is asynchronous programming, which allows it to handle time-consuming operations without blocking the user interface. For example, when fetching data from a server, JavaScript can use asynchronous requests (such as AJAX) to ensure that the user can still interact with the page without waiting for the data to load:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { document.getElementById('dataDisplay').textContent = JSON.stringify(data); }) .catch(error => console.error('Error:', error));
The practical application of JavaScript
Web Interaction
JavaScript has a wide range of applications in web interactions, from simple form verification to complex user interface design. For example, form validation can prevent users from submitting incomplete or incorrect form data:
document.getElementById('submitButton').addEventListener('click', function(event) { let email = document.getElementById('emailInput').value; if (!email.includes('@')) { alert('Please enter a valid email address'); event.preventDefault(); } });
Single Page Application (SPA)
JavaScript is also the core technology of single-page applications (SPA). SPAs provide a smooth user experience as they can update content without reloading the entire page. Frameworks such as React, Vue, and Angular all rely on JavaScript to build SPAs.
Server-side JavaScript
With the advent of Node.js, JavaScript is no longer limited to the browser side. It can run on the server side, allowing developers to develop on the front-end and back-end using the same language, thus simplifying the development process. For example, using Node.js can create a simple web server:
const http = require('http'); const server = http.createServer((req, res) => { res.writeHead(200, {'Content-Type': 'text/plain'}); res.end('Hello World\n'); }); server.listen(3000, () => { console.log('Server running on port 3000'); });
The importance of JavaScript
Improve user experience
The widespread use of JavaScript has greatly improved the user experience. It enables web pages to respond dynamically according to user actions, providing a smoother and more personalized experience. For example, Google Maps is a classic example of relying on JavaScript to provide an interactive map experience.
Cross-platform development
JavaScript's flexibility makes it an ideal choice for cross-platform development. Whether it is a mobile application, desktop application or server application, JavaScript is competent. For example, the Electron framework uses JavaScript and Node.js to build cross-platform desktop applications.
Ecosystems and communities
JavaScript has a huge and active community and ecosystem. This means developers can easily find a variety of libraries, frameworks, and tools to speed up the development process. For example, npm (Node Package Manager) offers millions of packages, covering from simple tools to complex frameworks.
Performance optimization and best practices
Performance optimization
Performance optimization is key when using JavaScript. Avoiding blocking user interface operations, using asynchronous programming to handle time-consuming tasks, and reducing unnecessary DOM operations are all effective ways to improve performance. For example, use requestAnimationFrame
to optimize animation effects:
function animate() { // Animation logic requestAnimationFrame(animate); } animate();
Best Practices
Following best practices can improve the readability and maintainability of your code. For example, use modular development to organize your code, use new features of ES6 such as let
and const
to avoid variable scope issues, and use strict patterns to catch common errors:
'use strict'; const PI = 3.14159; function calculateArea(radius) { return PI * radius * radius; } export { calculateArea };
in conclusion
JavaScript is not only a core technology of web development, but also an indispensable part of modern software development. By understanding the functions and applications of JavaScript, you can better utilize it to build dynamic, interactive applications. Whether you are a beginner or an experienced developer, mastering JavaScript will bring great value to your career.
The above is the detailed content of Demystifying JavaScript: What It Does and Why It Matters. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


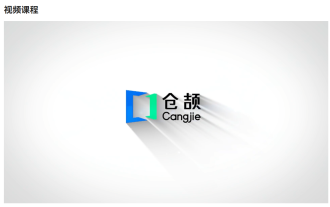
According to news from this site on June 24, at the keynote speech of the HDC2024 Huawei Developer Conference on June 21, Gong Ti, President of Huawei Terminal BG Software Department, officially announced Huawei’s self-developed Cangjie programming language. This language has been developed for 5 years and is now available for developer preview. Huawei's official developer website has now launched the official introductory tutorial video of Cangjie programming language to facilitate developers to get started and understand it. This tutorial will take users to experience Cangjie, learn Cangjie, and apply Cangjie, including using Cangjie language to estimate pi, calculate the stem and branch rules for each month of 2024, see N ways of expressing binary trees in Cangjie language, and use enumeration types to implement Algebraic calculations, signal system simulation using interfaces and extensions, and new syntax using Cangjie macros, etc. This site has tutorial access address: ht
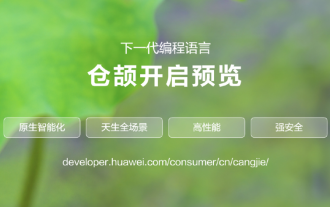
This site reported on June 21 that at the HDC2024 Huawei Developer Conference this afternoon, Gong Ti, President of Huawei Terminal BG Software Department, officially announced Huawei’s self-developed Cangjie programming language and released a developer preview version of HarmonyOSNEXT Cangjie language. This is the first time Huawei has publicly released the Cangjie programming language. Gong Ti said: "In 2019, the Cangjie programming language project was born at Huawei. After 5 years of R&D accumulation and heavy R&D investment, it finally meets global developers today. Cangjie programming language integrates modern language features, comprehensive compilation optimization and Runtime implementation and out-of-the-box IDE tool chain support create a friendly development experience and excellent program performance for developers. "According to reports, Cangjie programming language is an all-scenario intelligence tool.
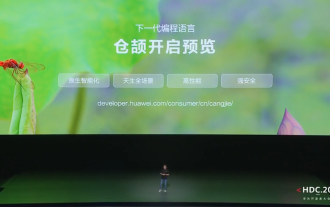
According to news from this site on June 21, Huawei’s self-developed Cangjie programming language was officially unveiled today, and the official announced the launch of HarmonyOSNEXT Cangjie language developer preview version Beta recruitment. This upgrade is an early adopter upgrade to the developer preview version, which provides Cangjie language SDK, developer guides and related DevEcoStudio plug-ins for developers to use Cangjie language to develop, debug and run HarmonyOSNext applications. Registration period: June 21, 2024 - October 21, 2024 Application requirements: This HarmonyOSNEXT Cangjie Language Developer Preview Beta recruitment event is only open to the following developers: 1) Real names have been completed in the Huawei Developer Alliance Certification; 2) Complete H
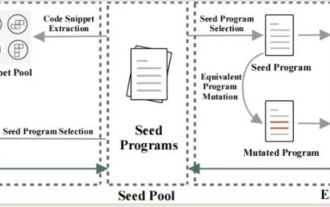
According to news from this site on June 22, Huawei yesterday introduced Huawei’s self-developed programming language-Cangjie to developers around the world. This is the first public appearance of Cangjie programming language. According to inquiries on this site, Tianjin University and Beijing University of Aeronautics and Astronautics were deeply involved in the research and development of Huawei’s “Cangjie”. Tianjin University: Cangjie Programming Language Compiler The software engineering team of the Department of Intelligence and Computing of Tianjin University joined hands with the Huawei Cangjie team to deeply participate in the quality assurance research of the Cangjie programming language compiler. According to reports, the Cangjie compiler is the basic software that is symbiotic with the Cangjie programming language. In the preparatory stage of the Cangjie programming language, a high-quality compiler that matches it became one of the core goals. As the Cangjie programming language evolves, the Cangjie compiler is constantly being upgraded and improved. In the past five years, Tianjin University
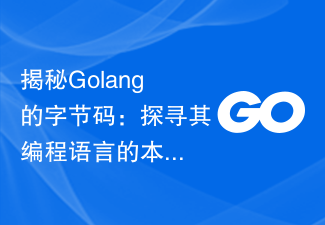
Golang (also known as Go language) is an open source programming language developed by Google and first released in 2007 to improve the productivity and development efficiency of engineers. Golang aims to simplify the complexity of programming languages and provide efficient execution speed while taking into account ease of use. This article will deeply explore the characteristics of Golang, analyze its bytecode mechanism, and reveal its working principle through specific code examples. 1. The characteristics and advantages of Golang are simple and efficient: Golang has a concise grammatical structure and rich
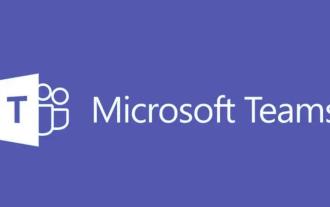
There are many languages to choose from in Microsoft Teams, so how to switch languages? Users need to click the menu, then find Settings, select General there, then click Language, select the language and save it. This introduction to switching language methods can tell you the specific content. The following is a detailed introduction. Take a look. Bar! How to switch language in Microsoft Teams Answer: Select the specific process in Settings-General-Language: 1. First, click the three dots next to the avatar to enter the settings. 2. Then click on the general options inside. 3. Then click on the language and scroll down to see more languages. 4. Finally, click Save and Restart.
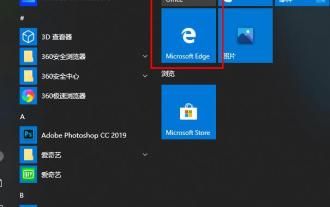
Recently, many friends have asked the editor what to do if the Microsoft Edge browser does not display images. Next, let us learn how to solve the problem of Microsoft Edge browser not displaying images. I hope it can help everyone. 1. First click on the lower left corner to start, and right-click on "Microsoft Edge Browser", as shown in the figure below. 2. Then select "More" and click "App Settings", as shown in the figure below. 3. Then scroll down to find "Pictures", as shown in the picture below. 4. Finally, turn on the switch below the picture, as shown in the picture below. The above is all the content that the editor brings to you on what to do if the Microsoft Edge browser does not display pictures. I hope it can be helpful to you.
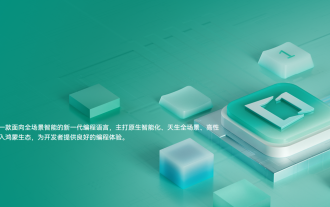
According to news from this site on June 21, before the HDC2024 Huawei Developer Conference, Huawei’s self-developed Cangjie programming language was officially unveiled, and the Cangjie official website is now online. The official website introduction shows that Cangjie programming language is a new generation programming language for all-scenario intelligence, focusing on "native intelligence, natural all-scenarios, high performance, and strong security." Integrate into the Hongmeng ecosystem to provide developers with a good programming experience. The official website attached to this site introduces as follows: Native intelligent programming framework embedded with AgentDSL, organic integration of natural language & programming language; multi-Agent collaboration, simplified symbolic expression, free combination of patterns, supporting the development of various intelligent applications. Innately lightweight and scalable runtime for all scenes, modular layered design, no matter how small the memory is, it can be accommodated; all-scenario domain expansion
