MySQL: A User-Friendly Introduction to Databases
The installation and basic operations of MySQL include: 1. Download and install MySQL and set the root user password; 2. Use SQL commands to create databases and tables, such as CREATE DATABASE and CREATE TABLE; 3. Execute CRUD operations, use INSERT, SELECT, UPDATE, DELETE commands; 4. Create indexes and stored procedures to optimize performance and implement complex logic. With these steps, you can build and manage MySQL databases from scratch.
introduction
In the modern digital world, databases are at the heart of any application system. Whether you are first exposed to programming or have been working in the industry for many years, mastering the basic knowledge of databases is indispensable. Today, we will dive into MySQL, the popular open source relational database management system. I will take you to start from scratch and gradually understand the basic operations and concepts of MySQL, helping you build a solid database foundation.
By reading this article, you will learn how to install MySQL, how to create and manage databases, and how to perform basic data operations. More importantly, you will understand why MySQL is so popular and its advantages and challenges in practical applications.
Review of basic knowledge
Before diving into MySQL, let's review the basics of databases. A database is essentially a system that organizes, stores and retrieves data, while a relational database organizes data through tables, where a table consists of rows and columns. MySQL, as a relational database management system (RDBMS), allows users to manage and operate databases using structured query language (SQL).
SQL is a powerful language that allows you to create, read, update and delete data, referred to as CRUD operations for short. Understanding SQL is the key to using MySQL, as it is the main tool for interacting with databases.
Core concept or function analysis
MySQL installation and configuration
Installing MySQL is the first step to getting in touch with this database. Whether you are using Windows, macOS or Linux, there are corresponding installation packages and detailed installation guides. You can download the latest version from the official MySQL website and follow the guide to install it. During the installation process, you need to set a root user password, which will be the highest permissions user of the database.
Configuring MySQL includes setting the character set of the database, adjusting server parameters to optimize performance, etc. Beginners can use the default configuration, but as you gain more knowledge about MySQL, you will find that tweaking configurations can significantly improve the performance of your database.
Database and table creation
In MySQL, creating databases and tables is the basic operation. With SQL commands, you can easily complete these tasks.
-- Create a database named 'mydb' CREATE DATABASE mydb; -- Use the database USE mydb just created; -- Create a table named 'users' containing 'id' and 'name' fields CREATE TABLE users ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(100) NOT NULL );
This simple example shows how to create databases and tables. As you can see, SQL commands are intuitive and easy to understand, which is also a major reason why MySQL is user-friendly.
Data Operation Language (DML)
What makes MySQL powerful is its Data Operation Language (DML), which allows you to perform CRUD operations. Here are a few basic DML command examples:
-- Insert data INSERT INTO users (name) VALUES ('John Doe'); -- Query data SELECT * FROM users; -- Update data UPDATE users SET name = 'Jane Doe' WHERE id = 1; -- Delete data DELETE FROM users WHERE id = 1;
These commands show how to perform basic data manipulation in MySQL. Understanding these commands is the basis for data management using MySQL.
Example of usage
Basic usage
Let's show the basic usage of MySQL with a simple example. We will create a book management system that contains the books and authors’ tables.
--Create TABLE books ( id INT AUTO_INCREMENT PRIMARY KEY, title VARCHAR(255) NOT NULL, author_id INT, FOREIGN KEY (author_id) REFERENCES authors(id) ); --Create authors CREATE TABLE authors ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(100) NOT NULL ); -- Insert data INSERT INTO authors (name) VALUES ('JK Rowling'); INSERT INTO books (title, author_id) VALUES ('Harry Potter', 1); -- Query data SELECT books.title, authors.name FROM books JOIN authors ON books.author_id = authors.id;
This example shows how to create tables, insert data, and perform simple query operations. With these basic operations, you can start building your own database application.
Advanced Usage
As your understanding of MySQL deepens, you can start exploring more advanced features. For example, indexes can significantly improve query performance, while stored procedures and triggers can help you implement complex business logic.
-- Create index CREATE INDEX idx_author_name ON authors(name); -- Create stored procedure DELIMITER // CREATE PROCEDURE GetBooksByAuthor(IN authorName VARCHAR(100)) BEGIN SELECT books.title FROM books JOIN authors ON books.author_id = authors.id WHERE authors.name = authorName; END // DELIMITER ; -- Call stored procedure CALL GetBooksByAuthor('JK Rowling');
These advanced features can help you manage and manipulate data more effectively, but require more learning and practice to master.
Common Errors and Debugging Tips
You may encounter some common errors when using MySQL. For example, forgetting to use the WHERE clause in a query can lead to unexpected data updates or deletion. Here are some common errors and their solutions:
- Forgot WHERE clause : When performing UPDATE or DELETE operations, make sure to use the WHERE clause to specify the data row to operate.
- SQL syntax error : Double check your SQL statements to make sure the syntax is correct. You can use MySQL's command line tools or graphical interface to help you discover and correct errors.
- Permissions Issue : Make sure you have enough permissions to perform the operation. If you encounter permission issues, contact the database administrator or use a user account with the appropriate permissions.
Performance optimization and best practices
In practical applications, it is crucial to optimize the performance of MySQL. Here are some recommendations for optimization and best practices:
- Using Indexes : Indexes can significantly improve query performance, but be careful not to overuse indexes, as this may increase the overhead of insertion and update operations.
- Optimize query : Avoid using SELECT * and select only the fields you need. Use the EXPLAIN command to analyze the execution plan of the query and find out the performance bottlenecks.
- Table and partition : For large-scale data, you can consider using table and partitioning techniques to improve query and insert performance.
- Regular maintenance : Regular database backup and optimization operations to ensure the health and performance of the database.
When writing SQL code, it is also very important to keep the code readable and maintainable. Using comments to interpret complex queries and consistently named conventions to name tables and fields can help you and your team manage your database more effectively.
In short, MySQL is a powerful and user-friendly database system. Through learning and practice, you can master its basic operations and advanced functions, thereby building efficient and reliable database applications. I hope this article can provide you with a good starting point and help you go further on the learning path of MySQL.
The above is the detailed content of MySQL: A User-Friendly Introduction to Databases. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


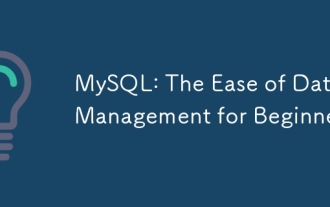
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
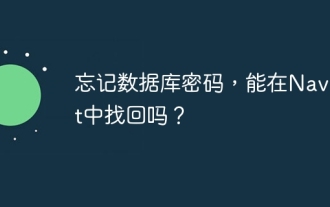
Navicat itself does not store the database password, and can only retrieve the encrypted password. Solution: 1. Check the password manager; 2. Check Navicat's "Remember Password" function; 3. Reset the database password; 4. Contact the database administrator.
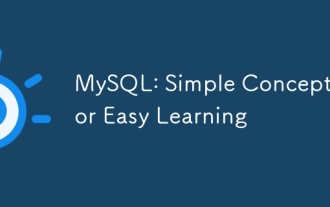
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
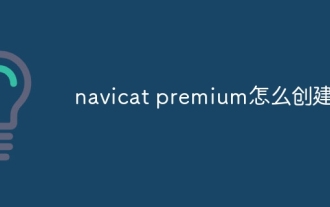
Create a database using Navicat Premium: Connect to the database server and enter the connection parameters. Right-click on the server and select Create Database. Enter the name of the new database and the specified character set and collation. Connect to the new database and create the table in the Object Browser. Right-click on the table and select Insert Data to insert the data.
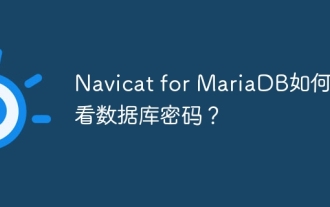
Navicat for MariaDB cannot view the database password directly because the password is stored in encrypted form. To ensure the database security, there are three ways to reset your password: reset your password through Navicat and set a complex password. View the configuration file (not recommended, high risk). Use system command line tools (not recommended, you need to be proficient in command line tools).
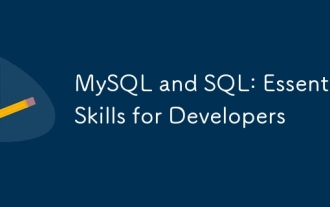
MySQL and SQL are essential skills for developers. 1.MySQL is an open source relational database management system, and SQL is the standard language used to manage and operate databases. 2.MySQL supports multiple storage engines through efficient data storage and retrieval functions, and SQL completes complex data operations through simple statements. 3. Examples of usage include basic queries and advanced queries, such as filtering and sorting by condition. 4. Common errors include syntax errors and performance issues, which can be optimized by checking SQL statements and using EXPLAIN commands. 5. Performance optimization techniques include using indexes, avoiding full table scanning, optimizing JOIN operations and improving code readability.
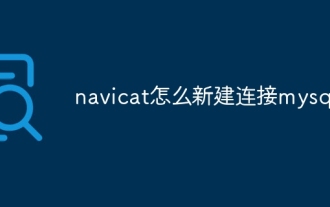
You can create a new MySQL connection in Navicat by following the steps: Open the application and select New Connection (Ctrl N). Select "MySQL" as the connection type. Enter the hostname/IP address, port, username, and password. (Optional) Configure advanced options. Save the connection and enter the connection name.
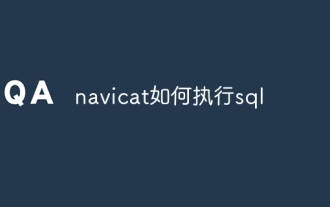
Steps to perform SQL in Navicat: Connect to the database. Create a SQL Editor window. Write SQL queries or scripts. Click the Run button to execute a query or script. View the results (if the query is executed).
