


What is the Null Coalescing Operator (??) and Null Coalescing Assignment Operator (??=)?
Null values can be handled in JavaScript using Null Coalescing Operator (??) and Null Coalescing Assignment Operator (??=). 1. ?? Returns the first non-null or non-undefined operand. 2. ??= Assign the variable to the value of the right operand, provided that the variable is null or undefined. These operators simplify code logic, improve readability and performance.
introduction
Have you ever encountered a situation when programming that needs to deal with null values? In JavaScript, Null Coalescing Operator (??) and Null Coalescing Assignment Operator (??=) are designed to solve this problem. The purpose of this article is to explore in-depth the usage and principles of these two operators, allowing you to flexibly apply them in real projects. After reading this article, you will understand how to use ?? and ??= to simplify the code logic and avoid the tedious operations when dealing with null values.
Review of basic knowledge
In JavaScript, handling null values has always been a headache. Traditionally, we use conditional statements or logical operators to handle null or undefined, but this often makes the code verbose and difficult to maintain. With this background knowledge, we can better understand why ?? and ??= is so important.
In JavaScript, null and undefined are two ways to represent "no value". They need to be handled specifically in many cases to prevent program errors. Traditional methods of handling are usually using if statements or ternary operators, but these methods tend to become complicated when dealing with multiple nested logic.
Core concept or function analysis
Null Coalescing Operator (??)
Null Coalescing Operator (??) is used to return the first non-null or non-undefined operand. It provides a neat way to handle default values. For example:
const name = null ?? 'John'; console.log(name); // Output 'John'
The function of this operator is to return the right operand when the left operand is null or undefined; otherwise, return the left operand. It avoids the use of lengthy if statements or ternary operators, making the code more concise and easy to read.
In terms of working principle, the ?? operator will first check the left operand, and if the left operand is null or undefined, it will return the right operand. Otherwise, the left operand is returned directly. This checking process is short-circuited, that is, if the left operand is not null or undefined, the right operand will not be evaluated.
Null Coalescing Assignment Operator (??=)
Null Coalescing Assignment Operator (??=) is the assignment version of the ?? operator. It is used to assign a variable to the value of the right operand, provided that the variable is currently null or undefined. For example:
let name; name ??= 'John'; console.log(name); // Output 'John'
The purpose of this operator is that when the variable is null or undefined, it is assigned to the value of the right operand. Otherwise, no operation is performed.
In terms of working principle, the ??= operator will first check the left operand. If the left operand is null or undefined, the value of the right operand is assigned to the left operand. Otherwise, no operation is performed. This assignment operation is also short-circuited, that is, if the left operand is not null or undefined, the right operand will not be evaluated.
Example of usage
Basic usage
Let's see the basic usage of ?? and ??=:
// Null Coalescing Operator (??) const userInput = null; const defaultValue = 'Default Value'; const result = userInput ?? defaultValue; console.log(result); // Output 'Default Value' // Null Coalescing Assignment Operator (??=) let userName = null; userName ??= 'Guest'; console.log(userName); // Output 'Guest'
In these examples, both ?? and ??= simplify the logic of handling null values, making the code more concise.
Advanced Usage
In more complex scenarios, ?? and ??= can be used in conjunction with other operators. For example:
// Use ?? to combine with logical operators const a = null; const b = false; const c = 0; const result = a ?? b || c; console.log(result); // Output false // Use ??= to process object attribute let user = { name: null, age: 30 }; user.name ??= 'Anonymous'; console.log(user.name); // Output 'Anonymous'
These advanced usages demonstrate the flexibility and simplicity of ?? and ??= when dealing with complex logic.
Common Errors and Debugging Tips
Common errors when using ?? and ??= include the difference between misuse and logical or operator (||). ?? The operator returns the right operand only when the left operand is null or undefined, while the || operator returns the right operand when the left operand is a false value. When debugging, you can use console.log or debugging tools to check the value of operands to ensure that both operators are used correctly.
Performance optimization and best practices
In terms of performance, the ?? and ??= operators are generally better than traditional if statements or ternary operators because they use short-circuit evaluation, reducing unnecessary calculations. Best practices when using ?? and ??= include:
- Try to avoid using ?? and ??= in complex logic to maintain the readability of the code.
- When working with object properties, use ??= to simplify the settings of default values.
- Note the difference between ?? and || to make sure they are used in the correct situation.
Through these practices, you can better utilize ?? and ??= to simplify code logic and improve code maintainability and performance.
In short, Null Coalescing Operator (??) and Null Coalescing Assignment Operator (??=) are powerful tools for handling null values in JavaScript. Through the explanation of this article, you should have mastered their usage and principles and be able to flexibly apply them in actual projects. I hope this knowledge can help you become more handy in the programming process.
The above is the detailed content of What is the Null Coalescing Operator (??) and Null Coalescing Assignment Operator (??=)?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


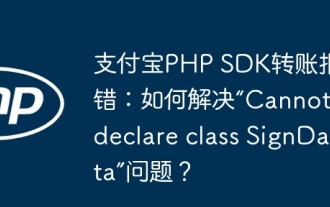
Alipay PHP...
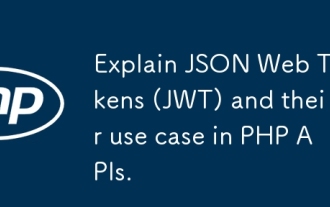
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
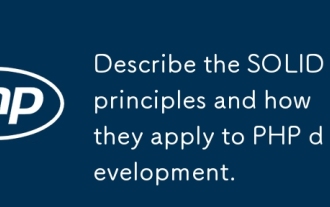
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
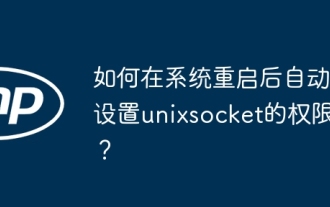
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
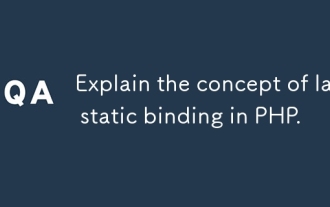
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
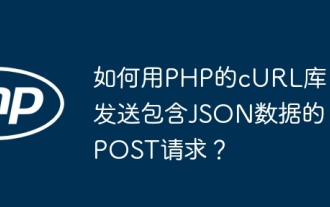
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
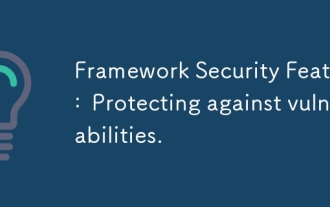
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
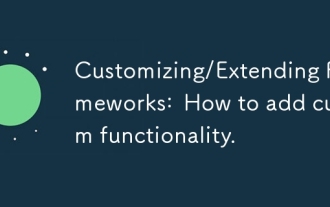
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
